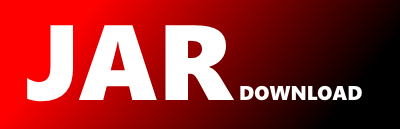
com.ch.manager.TestCaseManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ch-mongo Show documentation
Show all versions of ch-mongo Show documentation
Persistence services for mongo collections.
The newest version!
package com.ch.manager;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Logger;
import com.ch.config.ConfigProperties;
import com.ch.exception.DAOException;
import com.ch.exception.ValidationException;
import com.ch.model.BaseEntity;
import com.ch.model.Report;
import com.ch.model.TestReport;
import com.ch.mongo.TemplateDao;
import com.ch.validator.TestReportValidator;
public class TestCaseManager {
private static final Logger LOGGER = Logger.getLogger(TestCaseManager.class.getName());
private TemplateDao testCaseDao;
private TestCaseManager(TemplateDao testCaseDao) {
super();
this.testCaseDao = testCaseDao;
}
public static TestCaseManager getTestReportManager(String name, ConfigProperties configProperties)
throws NoSuchMethodException, SecurityException, ClassNotFoundException, InstantiationException, IllegalAccessException, IllegalArgumentException, InvocationTargetException {
Constructor> cons = Class.forName(name).getConstructor(ConfigProperties.class);
TemplateDao dao = (TemplateDao) cons.newInstance(configProperties);
LOGGER.finest("TestCaseDAO class loaded.");
return new TestCaseManager(dao);
}
public void createTestCase(BaseEntity testCase) throws ValidationException, DAOException {
TestReport testReport = (TestReport) testCase;
List reportList = new ArrayList();
reportList.add(testReport);
TestReportValidator.validate(reportList);
testCaseDao.saveEntity(testCase);
LOGGER.finest("Data saved successfully.");
}
public void createTestCase(Report report) throws ValidationException, DAOException {
List testReport = report.getTestReports();
try {
TestReportValidator.validate(testReport);
testCaseDao.saveBatchEntity(testReport);
LOGGER.finest("Data saved successfully.");
} catch (Exception e) {
throw new ValidationException(e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy