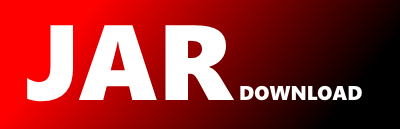
com.ch.report.upload.azure.AzureBlobStorageFileUploader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ch-upload Show documentation
Show all versions of ch-upload Show documentation
The Charles Hudson Java FileUploader to cloud storage.
The newest version!
package com.ch.report.upload.azure;
import java.io.File;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import java.util.TimeZone;
import java.util.logging.Logger;
import com.azure.storage.blob.BlobClient;
import com.azure.storage.blob.BlobContainerClient;
import com.azure.storage.blob.BlobContainerClientBuilder;
import com.ch.config.ConfigProperties;
import com.ch.model.ExecutionReport;
import com.ch.report.upload.FileUploader;
public class AzureBlobStorageFileUploader implements FileUploader {
private final static Logger LOGGER = Logger.getLogger(AzureBlobStorageFileUploader.class.getName());
@Override
public String upload(File file, ConfigProperties configProperties, ExecutionReport report) throws Exception {
if(file == null || report == null) {
return null;
}
if(!file.exists() && !file.isFile()) {
return null;
}
String sasUrl = configProperties.getProperty("CH_AZURE_BLOB_SAS_URL");
String timeZone = configProperties.getProperty("REPORT_TIMEZONE");
BlobContainerClient blobContainerClient = new BlobContainerClientBuilder()
.endpoint(sasUrl)
.buildClient();
Optional fileExt = getExtensionByStringHandling(file.getAbsolutePath());
String blobName = buildFileName("."+fileExt.orElse("html"),report, timeZone);
BlobClient blobClient = blobContainerClient.getBlobClient(blobName);
blobClient.uploadFromFile(file.getAbsolutePath(), true);
Map metadata = new HashMap();
metadata.put("EXECUTION_ID",report.getExecutionId());
metadata.put("SITE_ID",report.getSite());
blobClient.setMetadata(metadata);
LOGGER.info("Custom Report Upload to " + blobClient.getBlobUrl());
return blobClient.getBlobUrl();
}
public Optional getExtensionByStringHandling(String filename) {
return Optional.ofNullable(filename)
.filter(f -> f.contains("."))
.map(f -> f.substring(filename.lastIndexOf(".") + 1));
}
private String buildFileName(String extension, ExecutionReport report, String timeZone) {
String suiteName = report.getEnvironmentInfo().getSuite();
StringBuilder nameBuilder = new StringBuilder();
nameBuilder.append(report.getSite()).append("_");
nameBuilder.append(report.getEnvironmentInfo().getEnvironment()).append("_");
if(suiteName != null) {
nameBuilder.append(suiteName).append("_");
}
nameBuilder.append(report.getEnvironmentInfo().getBrowserName()).append("_");
nameBuilder.append(report.getEnvironmentInfo().getOs()).append("_");
String timeStr = getAppendableCurrentDate(timeZone);
nameBuilder.append(timeStr).append("_");
nameBuilder.append(report.getExecutionId());
nameBuilder.append(extension);
return nameBuilder.toString();
}
public String getAppendableCurrentDate(String timeZone) {
String format = "YYYYMMMdd_HHmmssSSz";
Date date = new Date();
DateFormat df = new SimpleDateFormat(format);
if(timeZone != null) {
df.setTimeZone(TimeZone.getTimeZone(timeZone));
} else {
df.setTimeZone(TimeZone.getTimeZone("America/New_York"));
}
String str = df.format(date);
return str;
}
public String removeFileExtension(String filename, boolean removeAllExtensions) {
if (filename == null || filename.isEmpty()) {
return filename;
}
String extPattern = "(?
© 2015 - 2025 Weber Informatics LLC | Privacy Policy