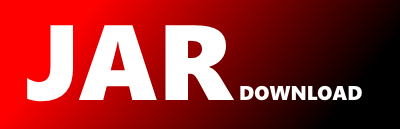
com.chutneytesting.admin.infra.gitbackup.ChutneyCampaignContent Maven / Gradle / Ivy
package com.chutneytesting.admin.infra.gitbackup;
import com.chutneytesting.admin.domain.gitbackup.ChutneyContent;
import com.chutneytesting.admin.domain.gitbackup.ChutneyContentCategory;
import com.chutneytesting.admin.domain.gitbackup.ChutneyContentProvider;
import com.chutneytesting.design.domain.campaign.Campaign;
import com.chutneytesting.design.domain.campaign.CampaignRepository;
import com.chutneytesting.tools.file.FileUtils;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
import java.util.stream.Stream;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.stereotype.Component;
@Component
public class ChutneyCampaignContent implements ChutneyContentProvider {
private final CampaignRepository repository;
private final ObjectMapper mapper;
public ChutneyCampaignContent(CampaignRepository repository, @Qualifier("gitObjectMapper") ObjectMapper mapper) {
this.repository = repository;
this.mapper = mapper;
}
@Override
public String provider() {
return "campaign";
}
@Override
public ChutneyContentCategory category() {
return ChutneyContentCategory.CAMPAIGN;
}
@Override
public Stream getContent() {
return repository.findAll().stream()
.map(c -> {
ChutneyContent.ChutneyContentBuilder builder = ChutneyContent.builder()
.withProvider(provider())
.withCategory(category())
.withName("[" + c.id + "]-" + c.title);
try {
builder
.withContent(mapper.writeValueAsString(c))
.withFormat("json");
} catch (JsonProcessingException e) {
throw new RuntimeException(e.getMessage(), e);
}
return builder.build();
});
}
@Override
public void importDefaultFolder(Path workingDirectory) {
importFolder(providerFolder(workingDirectory));
}
public void importFolder(Path folderPath) {
List campaigns = FileUtils.listFiles(folderPath);
campaigns.forEach(this::importFile);
}
public void importFile(Path filePath) {
if (Files.exists(filePath)) {
try {
byte[] bytes = Files.readAllBytes(filePath);
try {
Campaign campaign = mapper.readValue(bytes, Campaign.class);
repository.createOrUpdate(campaign);
} catch (IOException e) {
throw new UnsupportedOperationException("Cannot deserialize dataset file : " + filePath, e);
}
} catch (IOException e) {
throw new UnsupportedOperationException("Cannot read dataset file : " + filePath, e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy