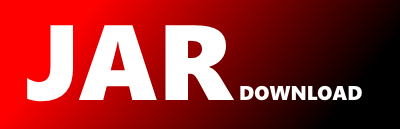
com.chutneytesting.design.api.scenario.compose.dto.ImmutableComposableStepDto Maven / Gradle / Ivy
package com.chutneytesting.design.api.scenario.compose.dto;
import com.chutneytesting.tools.ui.KeyValue;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ComposableStepDto}.
*
* Use the builder to create immutable instances:
* {@code ImmutableComposableStepDto.builder()}.
*/
@Generated(from = "ComposableStepDto", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableComposableStepDto
implements ComposableStepDto {
private final @Nullable String id;
private final String name;
private final Strategy strategy;
private final ComposableStepDto.StepUsage usage;
private final @Nullable String task;
private final List steps;
private final List defaultParameters;
private final List executionParameters;
private final List tags;
private ImmutableComposableStepDto(ImmutableComposableStepDto.Builder builder) {
this.id = builder.id;
this.name = builder.name;
this.task = builder.task;
if (builder.strategy != null) {
initShim.strategy(builder.strategy);
}
if (builder.usage != null) {
initShim.usage(builder.usage);
}
if (builder.stepsIsSet()) {
initShim.steps(createUnmodifiableList(true, builder.steps));
}
if (builder.defaultParametersIsSet()) {
initShim.defaultParameters(createUnmodifiableList(true, builder.defaultParameters));
}
if (builder.executionParametersIsSet()) {
initShim.executionParameters(createUnmodifiableList(true, builder.executionParameters));
}
if (builder.tagsIsSet()) {
initShim.tags(createUnmodifiableList(true, builder.tags));
}
this.strategy = initShim.strategy();
this.usage = initShim.usage();
this.steps = initShim.steps();
this.defaultParameters = initShim.defaultParameters();
this.executionParameters = initShim.executionParameters();
this.tags = initShim.tags();
this.initShim = null;
}
private ImmutableComposableStepDto(
@Nullable String id,
String name,
Strategy strategy,
ComposableStepDto.StepUsage usage,
@Nullable String task,
List steps,
List defaultParameters,
List executionParameters,
List tags) {
this.id = id;
this.name = name;
this.strategy = strategy;
this.usage = usage;
this.task = task;
this.steps = steps;
this.defaultParameters = defaultParameters;
this.executionParameters = executionParameters;
this.tags = tags;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "ComposableStepDto", generator = "Immutables")
private final class InitShim {
private byte strategyBuildStage = STAGE_UNINITIALIZED;
private Strategy strategy;
Strategy strategy() {
if (strategyBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (strategyBuildStage == STAGE_UNINITIALIZED) {
strategyBuildStage = STAGE_INITIALIZING;
this.strategy = Objects.requireNonNull(strategyInitialize(), "strategy");
strategyBuildStage = STAGE_INITIALIZED;
}
return this.strategy;
}
void strategy(Strategy strategy) {
this.strategy = strategy;
strategyBuildStage = STAGE_INITIALIZED;
}
private byte usageBuildStage = STAGE_UNINITIALIZED;
private ComposableStepDto.StepUsage usage;
ComposableStepDto.StepUsage usage() {
if (usageBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (usageBuildStage == STAGE_UNINITIALIZED) {
usageBuildStage = STAGE_INITIALIZING;
this.usage = Objects.requireNonNull(usageInitialize(), "usage");
usageBuildStage = STAGE_INITIALIZED;
}
return this.usage;
}
void usage(ComposableStepDto.StepUsage usage) {
this.usage = usage;
usageBuildStage = STAGE_INITIALIZED;
}
private byte stepsBuildStage = STAGE_UNINITIALIZED;
private List steps;
List steps() {
if (stepsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (stepsBuildStage == STAGE_UNINITIALIZED) {
stepsBuildStage = STAGE_INITIALIZING;
this.steps = createUnmodifiableList(false, createSafeList(stepsInitialize(), true, false));
stepsBuildStage = STAGE_INITIALIZED;
}
return this.steps;
}
void steps(List steps) {
this.steps = steps;
stepsBuildStage = STAGE_INITIALIZED;
}
private byte defaultParametersBuildStage = STAGE_UNINITIALIZED;
private List defaultParameters;
List defaultParameters() {
if (defaultParametersBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (defaultParametersBuildStage == STAGE_UNINITIALIZED) {
defaultParametersBuildStage = STAGE_INITIALIZING;
this.defaultParameters = createUnmodifiableList(false, createSafeList(defaultParametersInitialize(), true, false));
defaultParametersBuildStage = STAGE_INITIALIZED;
}
return this.defaultParameters;
}
void defaultParameters(List defaultParameters) {
this.defaultParameters = defaultParameters;
defaultParametersBuildStage = STAGE_INITIALIZED;
}
private byte executionParametersBuildStage = STAGE_UNINITIALIZED;
private List executionParameters;
List executionParameters() {
if (executionParametersBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (executionParametersBuildStage == STAGE_UNINITIALIZED) {
executionParametersBuildStage = STAGE_INITIALIZING;
this.executionParameters = createUnmodifiableList(false, createSafeList(executionParametersInitialize(), true, false));
executionParametersBuildStage = STAGE_INITIALIZED;
}
return this.executionParameters;
}
void executionParameters(List executionParameters) {
this.executionParameters = executionParameters;
executionParametersBuildStage = STAGE_INITIALIZED;
}
private byte tagsBuildStage = STAGE_UNINITIALIZED;
private List tags;
List tags() {
if (tagsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (tagsBuildStage == STAGE_UNINITIALIZED) {
tagsBuildStage = STAGE_INITIALIZING;
this.tags = createUnmodifiableList(false, createSafeList(tagsInitialize(), true, false));
tagsBuildStage = STAGE_INITIALIZED;
}
return this.tags;
}
void tags(List tags) {
this.tags = tags;
tagsBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (strategyBuildStage == STAGE_INITIALIZING) attributes.add("strategy");
if (usageBuildStage == STAGE_INITIALIZING) attributes.add("usage");
if (stepsBuildStage == STAGE_INITIALIZING) attributes.add("steps");
if (defaultParametersBuildStage == STAGE_INITIALIZING) attributes.add("defaultParameters");
if (executionParametersBuildStage == STAGE_INITIALIZING) attributes.add("executionParameters");
if (tagsBuildStage == STAGE_INITIALIZING) attributes.add("tags");
return "Cannot build ComposableStepDto, attribute initializers form cycle " + attributes;
}
}
private Strategy strategyInitialize() {
return ComposableStepDto.super.strategy();
}
private ComposableStepDto.StepUsage usageInitialize() {
return ComposableStepDto.super.usage();
}
private List stepsInitialize() {
return ComposableStepDto.super.steps();
}
private List defaultParametersInitialize() {
return ComposableStepDto.super.defaultParameters();
}
private List executionParametersInitialize() {
return ComposableStepDto.super.executionParameters();
}
private List tagsInitialize() {
return ComposableStepDto.super.tags();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public Optional id() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public String name() {
return name;
}
/**
* @return The value of the {@code strategy} attribute
*/
@JsonProperty("strategy")
@Override
public Strategy strategy() {
InitShim shim = this.initShim;
return shim != null
? shim.strategy()
: this.strategy;
}
/**
* @return The value of the {@code usage} attribute
*/
@JsonProperty("usage")
@Override
public ComposableStepDto.StepUsage usage() {
InitShim shim = this.initShim;
return shim != null
? shim.usage()
: this.usage;
}
/**
* @return The value of the {@code task} attribute
*/
@JsonProperty("task")
@Override
public Optional task() {
return Optional.ofNullable(task);
}
/**
* @return The value of the {@code steps} attribute
*/
@JsonProperty("steps")
@Override
public List steps() {
InitShim shim = this.initShim;
return shim != null
? shim.steps()
: this.steps;
}
/**
* @return The value of the {@code defaultParameters} attribute
*/
@JsonProperty("parameters")
@Override
public List defaultParameters() {
InitShim shim = this.initShim;
return shim != null
? shim.defaultParameters()
: this.defaultParameters;
}
/**
* @return The value of the {@code executionParameters} attribute
*/
@JsonProperty("computedParameters")
@Override
public List executionParameters() {
InitShim shim = this.initShim;
return shim != null
? shim.executionParameters()
: this.executionParameters;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public List tags() {
InitShim shim = this.initShim;
return shim != null
? shim.tags()
: this.tags;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ComposableStepDto#id() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withId(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return new ImmutableComposableStepDto(
newValue,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ComposableStepDto#id() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return new ImmutableComposableStepDto(
value,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object by setting a value for the {@link ComposableStepDto#name() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableComposableStepDto withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableComposableStepDto(
this.id,
newValue,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object by setting a value for the {@link ComposableStepDto#strategy() strategy} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for strategy
* @return A modified copy of the {@code this} object
*/
public final ImmutableComposableStepDto withStrategy(Strategy value) {
if (this.strategy == value) return this;
Strategy newValue = Objects.requireNonNull(value, "strategy");
return new ImmutableComposableStepDto(
this.id,
this.name,
newValue,
this.usage,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object by setting a value for the {@link ComposableStepDto#usage() usage} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for usage
* @return A modified copy of the {@code this} object
*/
public final ImmutableComposableStepDto withUsage(ComposableStepDto.StepUsage value) {
if (this.usage == value) return this;
ComposableStepDto.StepUsage newValue = Objects.requireNonNull(value, "usage");
if (this.usage.equals(newValue)) return this;
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
newValue,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ComposableStepDto#task() task} attribute.
* @param value The value for task
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withTask(String value) {
@Nullable String newValue = Objects.requireNonNull(value, "task");
if (Objects.equals(this.task, newValue)) return this;
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
newValue,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ComposableStepDto#task() task} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for task
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withTask(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.task, value)) return this;
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
value,
this.steps,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#steps() steps}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withSteps(ComposableStepDto... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
newValue,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#steps() steps}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of steps elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withSteps(Iterable extends ComposableStepDto> elements) {
if (this.steps == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
newValue,
this.defaultParameters,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#defaultParameters() defaultParameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withDefaultParameters(KeyValue... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
newValue,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#defaultParameters() defaultParameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of defaultParameters elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withDefaultParameters(Iterable extends KeyValue> elements) {
if (this.defaultParameters == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
newValue,
this.executionParameters,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#executionParameters() executionParameters}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withExecutionParameters(KeyValue... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
newValue,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#executionParameters() executionParameters}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of executionParameters elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withExecutionParameters(Iterable extends KeyValue> elements) {
if (this.executionParameters == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
newValue,
this.tags);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#tags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withTags(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link ComposableStepDto#tags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableComposableStepDto withTags(Iterable elements) {
if (this.tags == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableComposableStepDto(
this.id,
this.name,
this.strategy,
this.usage,
this.task,
this.steps,
this.defaultParameters,
this.executionParameters,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableComposableStepDto} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableComposableStepDto
&& equalTo((ImmutableComposableStepDto) another);
}
private boolean equalTo(ImmutableComposableStepDto another) {
return Objects.equals(id, another.id)
&& name.equals(another.name)
&& strategy.equals(another.strategy)
&& usage.equals(another.usage)
&& Objects.equals(task, another.task)
&& steps.equals(another.steps)
&& defaultParameters.equals(another.defaultParameters)
&& executionParameters.equals(another.executionParameters)
&& tags.equals(another.tags);
}
/**
* Computes a hash code from attributes: {@code id}, {@code name}, {@code strategy}, {@code usage}, {@code task}, {@code steps}, {@code defaultParameters}, {@code executionParameters}, {@code tags}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + name.hashCode();
h += (h << 5) + strategy.hashCode();
h += (h << 5) + usage.hashCode();
h += (h << 5) + Objects.hashCode(task);
h += (h << 5) + steps.hashCode();
h += (h << 5) + defaultParameters.hashCode();
h += (h << 5) + executionParameters.hashCode();
h += (h << 5) + tags.hashCode();
return h;
}
/**
* Prints the immutable value {@code ComposableStepDto} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("ComposableStepDto{");
if (id != null) {
builder.append("id=").append(id);
}
if (builder.length() > 18) builder.append(", ");
builder.append("name=").append(name);
builder.append(", ");
builder.append("strategy=").append(strategy);
builder.append(", ");
builder.append("usage=").append(usage);
if (task != null) {
builder.append(", ");
builder.append("task=").append(task);
}
builder.append(", ");
builder.append("steps=").append(steps);
builder.append(", ");
builder.append("defaultParameters=").append(defaultParameters);
builder.append(", ");
builder.append("executionParameters=").append(executionParameters);
builder.append(", ");
builder.append("tags=").append(tags);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "ComposableStepDto", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements ComposableStepDto {
@Nullable Optional id = Optional.empty();
@Nullable String name;
@Nullable Strategy strategy;
@Nullable ComposableStepDto.StepUsage usage;
@Nullable Optional task = Optional.empty();
@Nullable List steps = Collections.emptyList();
boolean stepsIsSet;
@Nullable List defaultParameters = Collections.emptyList();
boolean defaultParametersIsSet;
@Nullable List executionParameters = Collections.emptyList();
boolean executionParametersIsSet;
@Nullable List tags = Collections.emptyList();
boolean tagsIsSet;
@JsonProperty("id")
public void setId(Optional id) {
this.id = id;
}
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
@JsonProperty("strategy")
public void setStrategy(Strategy strategy) {
this.strategy = strategy;
}
@JsonProperty("usage")
public void setUsage(ComposableStepDto.StepUsage usage) {
this.usage = usage;
}
@JsonProperty("task")
public void setTask(Optional task) {
this.task = task;
}
@JsonProperty("steps")
public void setSteps(List steps) {
this.steps = steps;
this.stepsIsSet = true;
}
@JsonProperty("parameters")
public void setDefaultParameters(List defaultParameters) {
this.defaultParameters = defaultParameters;
this.defaultParametersIsSet = true;
}
@JsonProperty("computedParameters")
public void setExecutionParameters(List executionParameters) {
this.executionParameters = executionParameters;
this.executionParametersIsSet = true;
}
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
this.tagsIsSet = true;
}
@Override
public Optional id() { throw new UnsupportedOperationException(); }
@Override
public String name() { throw new UnsupportedOperationException(); }
@Override
public Strategy strategy() { throw new UnsupportedOperationException(); }
@Override
public ComposableStepDto.StepUsage usage() { throw new UnsupportedOperationException(); }
@Override
public Optional task() { throw new UnsupportedOperationException(); }
@Override
public List steps() { throw new UnsupportedOperationException(); }
@Override
public List defaultParameters() { throw new UnsupportedOperationException(); }
@Override
public List executionParameters() { throw new UnsupportedOperationException(); }
@Override
public List tags() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableComposableStepDto fromJson(Json json) {
ImmutableComposableStepDto.Builder builder = ImmutableComposableStepDto.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.name != null) {
builder.name(json.name);
}
if (json.strategy != null) {
builder.strategy(json.strategy);
}
if (json.usage != null) {
builder.usage(json.usage);
}
if (json.task != null) {
builder.task(json.task);
}
if (json.stepsIsSet) {
builder.addAllSteps(json.steps);
}
if (json.defaultParametersIsSet) {
builder.addAllDefaultParameters(json.defaultParameters);
}
if (json.executionParametersIsSet) {
builder.addAllExecutionParameters(json.executionParameters);
}
if (json.tagsIsSet) {
builder.addAllTags(json.tags);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link ComposableStepDto} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ComposableStepDto instance
*/
public static ImmutableComposableStepDto copyOf(ComposableStepDto instance) {
if (instance instanceof ImmutableComposableStepDto) {
return (ImmutableComposableStepDto) instance;
}
return ImmutableComposableStepDto.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableComposableStepDto ImmutableComposableStepDto}.
*
* ImmutableComposableStepDto.builder()
* .id(String) // optional {@link ComposableStepDto#id() id}
* .name(String) // required {@link ComposableStepDto#name() name}
* .strategy(com.chutneytesting.design.api.scenario.compose.dto.Strategy) // optional {@link ComposableStepDto#strategy() strategy}
* .usage(com.chutneytesting.design.api.scenario.compose.dto.ComposableStepDto.StepUsage) // optional {@link ComposableStepDto#usage() usage}
* .task(String) // optional {@link ComposableStepDto#task() task}
* .addSteps|addAllSteps(com.chutneytesting.design.api.scenario.compose.dto.ComposableStepDto) // {@link ComposableStepDto#steps() steps} elements
* .addDefaultParameters|addAllDefaultParameters(com.chutneytesting.tools.ui.KeyValue) // {@link ComposableStepDto#defaultParameters() defaultParameters} elements
* .addExecutionParameters|addAllExecutionParameters(com.chutneytesting.tools.ui.KeyValue) // {@link ComposableStepDto#executionParameters() executionParameters} elements
* .addTags|addAllTags(String) // {@link ComposableStepDto#tags() tags} elements
* .build();
*
* @return A new ImmutableComposableStepDto builder
*/
public static ImmutableComposableStepDto.Builder builder() {
return new ImmutableComposableStepDto.Builder();
}
/**
* Builds instances of type {@link ImmutableComposableStepDto ImmutableComposableStepDto}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ComposableStepDto", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long OPT_BIT_STEPS = 0x1L;
private static final long OPT_BIT_DEFAULT_PARAMETERS = 0x2L;
private static final long OPT_BIT_EXECUTION_PARAMETERS = 0x4L;
private static final long OPT_BIT_TAGS = 0x8L;
private long initBits = 0x1L;
private long optBits;
private @Nullable String id;
private @Nullable String name;
private @Nullable Strategy strategy;
private @Nullable ComposableStepDto.StepUsage usage;
private @Nullable String task;
private List steps = new ArrayList();
private List defaultParameters = new ArrayList();
private List executionParameters = new ArrayList();
private List tags = new ArrayList();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code ComposableStepDto} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ComposableStepDto instance) {
Objects.requireNonNull(instance, "instance");
Optional idOptional = instance.id();
if (idOptional.isPresent()) {
id(idOptional);
}
name(instance.name());
strategy(instance.strategy());
usage(instance.usage());
Optional taskOptional = instance.task();
if (taskOptional.isPresent()) {
task(taskOptional);
}
addAllSteps(instance.steps());
addAllDefaultParameters(instance.defaultParameters());
addAllExecutionParameters(instance.executionParameters());
addAllTags(instance.tags());
return this;
}
/**
* Initializes the optional value {@link ComposableStepDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return this;
}
/**
* Initializes the optional value {@link ComposableStepDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final Builder id(Optional id) {
this.id = id.orElse(null);
return this;
}
/**
* Initializes the value for the {@link ComposableStepDto#name() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link ComposableStepDto#strategy() strategy} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link ComposableStepDto#strategy() strategy}.
* @param strategy The value for strategy
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("strategy")
public final Builder strategy(Strategy strategy) {
this.strategy = Objects.requireNonNull(strategy, "strategy");
return this;
}
/**
* Initializes the value for the {@link ComposableStepDto#usage() usage} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link ComposableStepDto#usage() usage}.
* @param usage The value for usage
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("usage")
public final Builder usage(ComposableStepDto.StepUsage usage) {
this.usage = Objects.requireNonNull(usage, "usage");
return this;
}
/**
* Initializes the optional value {@link ComposableStepDto#task() task} to task.
* @param task The value for task
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder task(String task) {
this.task = Objects.requireNonNull(task, "task");
return this;
}
/**
* Initializes the optional value {@link ComposableStepDto#task() task} to task.
* @param task The value for task
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("task")
public final Builder task(Optional task) {
this.task = task.orElse(null);
return this;
}
/**
* Adds one element to {@link ComposableStepDto#steps() steps} list.
* @param element A steps element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSteps(ComposableStepDto element) {
this.steps.add(Objects.requireNonNull(element, "steps element"));
optBits |= OPT_BIT_STEPS;
return this;
}
/**
* Adds elements to {@link ComposableStepDto#steps() steps} list.
* @param elements An array of steps elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addSteps(ComposableStepDto... elements) {
for (ComposableStepDto element : elements) {
this.steps.add(Objects.requireNonNull(element, "steps element"));
}
optBits |= OPT_BIT_STEPS;
return this;
}
/**
* Sets or replaces all elements for {@link ComposableStepDto#steps() steps} list.
* @param elements An iterable of steps elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("steps")
public final Builder steps(Iterable extends ComposableStepDto> elements) {
this.steps.clear();
return addAllSteps(elements);
}
/**
* Adds elements to {@link ComposableStepDto#steps() steps} list.
* @param elements An iterable of steps elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllSteps(Iterable extends ComposableStepDto> elements) {
for (ComposableStepDto element : elements) {
this.steps.add(Objects.requireNonNull(element, "steps element"));
}
optBits |= OPT_BIT_STEPS;
return this;
}
/**
* Adds one element to {@link ComposableStepDto#defaultParameters() defaultParameters} list.
* @param element A defaultParameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addDefaultParameters(KeyValue element) {
this.defaultParameters.add(Objects.requireNonNull(element, "defaultParameters element"));
optBits |= OPT_BIT_DEFAULT_PARAMETERS;
return this;
}
/**
* Adds elements to {@link ComposableStepDto#defaultParameters() defaultParameters} list.
* @param elements An array of defaultParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addDefaultParameters(KeyValue... elements) {
for (KeyValue element : elements) {
this.defaultParameters.add(Objects.requireNonNull(element, "defaultParameters element"));
}
optBits |= OPT_BIT_DEFAULT_PARAMETERS;
return this;
}
/**
* Sets or replaces all elements for {@link ComposableStepDto#defaultParameters() defaultParameters} list.
* @param elements An iterable of defaultParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("parameters")
public final Builder defaultParameters(Iterable extends KeyValue> elements) {
this.defaultParameters.clear();
return addAllDefaultParameters(elements);
}
/**
* Adds elements to {@link ComposableStepDto#defaultParameters() defaultParameters} list.
* @param elements An iterable of defaultParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllDefaultParameters(Iterable extends KeyValue> elements) {
for (KeyValue element : elements) {
this.defaultParameters.add(Objects.requireNonNull(element, "defaultParameters element"));
}
optBits |= OPT_BIT_DEFAULT_PARAMETERS;
return this;
}
/**
* Adds one element to {@link ComposableStepDto#executionParameters() executionParameters} list.
* @param element A executionParameters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addExecutionParameters(KeyValue element) {
this.executionParameters.add(Objects.requireNonNull(element, "executionParameters element"));
optBits |= OPT_BIT_EXECUTION_PARAMETERS;
return this;
}
/**
* Adds elements to {@link ComposableStepDto#executionParameters() executionParameters} list.
* @param elements An array of executionParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addExecutionParameters(KeyValue... elements) {
for (KeyValue element : elements) {
this.executionParameters.add(Objects.requireNonNull(element, "executionParameters element"));
}
optBits |= OPT_BIT_EXECUTION_PARAMETERS;
return this;
}
/**
* Sets or replaces all elements for {@link ComposableStepDto#executionParameters() executionParameters} list.
* @param elements An iterable of executionParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("computedParameters")
public final Builder executionParameters(Iterable extends KeyValue> elements) {
this.executionParameters.clear();
return addAllExecutionParameters(elements);
}
/**
* Adds elements to {@link ComposableStepDto#executionParameters() executionParameters} list.
* @param elements An iterable of executionParameters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllExecutionParameters(Iterable extends KeyValue> elements) {
for (KeyValue element : elements) {
this.executionParameters.add(Objects.requireNonNull(element, "executionParameters element"));
}
optBits |= OPT_BIT_EXECUTION_PARAMETERS;
return this;
}
/**
* Adds one element to {@link ComposableStepDto#tags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String element) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
optBits |= OPT_BIT_TAGS;
return this;
}
/**
* Adds elements to {@link ComposableStepDto#tags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String... elements) {
for (String element : elements) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
}
optBits |= OPT_BIT_TAGS;
return this;
}
/**
* Sets or replaces all elements for {@link ComposableStepDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
public final Builder tags(Iterable elements) {
this.tags.clear();
return addAllTags(elements);
}
/**
* Adds elements to {@link ComposableStepDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable elements) {
for (String element : elements) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
}
optBits |= OPT_BIT_TAGS;
return this;
}
/**
* Builds a new {@link ImmutableComposableStepDto ImmutableComposableStepDto}.
* @return An immutable instance of ComposableStepDto
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableComposableStepDto build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableComposableStepDto(this);
}
private boolean stepsIsSet() {
return (optBits & OPT_BIT_STEPS) != 0;
}
private boolean defaultParametersIsSet() {
return (optBits & OPT_BIT_DEFAULT_PARAMETERS) != 0;
}
private boolean executionParametersIsSet() {
return (optBits & OPT_BIT_EXECUTION_PARAMETERS) != 0;
}
private boolean tagsIsSet() {
return (optBits & OPT_BIT_TAGS) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
return "Cannot build ComposableStepDto, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>();
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}