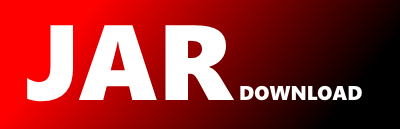
com.chutneytesting.design.api.scenario.v2_0.dto.ImmutableGwtStepImplementationDto Maven / Gradle / Ivy
package com.chutneytesting.design.api.scenario.v2_0.dto;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link GwtStepImplementationDto}.
*
* Use the builder to create immutable instances:
* {@code ImmutableGwtStepImplementationDto.builder()}.
*/
@Generated(from = "GwtStepImplementationDto", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableGwtStepImplementationDto
implements GwtStepImplementationDto {
private final String task;
private final String type;
private final String target;
private final String xRef;
private final Map inputs;
private final Map outputs;
private final Map validations;
private ImmutableGwtStepImplementationDto(ImmutableGwtStepImplementationDto.Builder builder) {
this.inputs = createUnmodifiableMap(false, false, builder.inputs);
this.outputs = createUnmodifiableMap(false, false, builder.outputs);
this.validations = createUnmodifiableMap(false, false, builder.validations);
if (builder.task != null) {
initShim.task(builder.task);
}
if (builder.type != null) {
initShim.type(builder.type);
}
if (builder.target != null) {
initShim.target(builder.target);
}
if (builder.xRef != null) {
initShim.xRef(builder.xRef);
}
this.task = initShim.task();
this.type = initShim.type();
this.target = initShim.target();
this.xRef = initShim.xRef();
this.initShim = null;
}
private ImmutableGwtStepImplementationDto(
String task,
String type,
String target,
String xRef,
Map inputs,
Map outputs,
Map validations) {
this.task = task;
this.type = type;
this.target = target;
this.xRef = xRef;
this.inputs = inputs;
this.outputs = outputs;
this.validations = validations;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "GwtStepImplementationDto", generator = "Immutables")
private final class InitShim {
private byte taskBuildStage = STAGE_UNINITIALIZED;
private String task;
String task() {
if (taskBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (taskBuildStage == STAGE_UNINITIALIZED) {
taskBuildStage = STAGE_INITIALIZING;
this.task = Objects.requireNonNull(taskInitialize(), "task");
taskBuildStage = STAGE_INITIALIZED;
}
return this.task;
}
void task(String task) {
this.task = task;
taskBuildStage = STAGE_INITIALIZED;
}
private byte typeBuildStage = STAGE_UNINITIALIZED;
private String type;
String type() {
if (typeBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (typeBuildStage == STAGE_UNINITIALIZED) {
typeBuildStage = STAGE_INITIALIZING;
this.type = Objects.requireNonNull(typeInitialize(), "type");
typeBuildStage = STAGE_INITIALIZED;
}
return this.type;
}
void type(String type) {
this.type = type;
typeBuildStage = STAGE_INITIALIZED;
}
private byte targetBuildStage = STAGE_UNINITIALIZED;
private String target;
String target() {
if (targetBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (targetBuildStage == STAGE_UNINITIALIZED) {
targetBuildStage = STAGE_INITIALIZING;
this.target = Objects.requireNonNull(targetInitialize(), "target");
targetBuildStage = STAGE_INITIALIZED;
}
return this.target;
}
void target(String target) {
this.target = target;
targetBuildStage = STAGE_INITIALIZED;
}
private byte xRefBuildStage = STAGE_UNINITIALIZED;
private String xRef;
String xRef() {
if (xRefBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (xRefBuildStage == STAGE_UNINITIALIZED) {
xRefBuildStage = STAGE_INITIALIZING;
this.xRef = Objects.requireNonNull(xRefInitialize(), "xRef");
xRefBuildStage = STAGE_INITIALIZED;
}
return this.xRef;
}
void xRef(String xRef) {
this.xRef = xRef;
xRefBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (taskBuildStage == STAGE_INITIALIZING) attributes.add("task");
if (typeBuildStage == STAGE_INITIALIZING) attributes.add("type");
if (targetBuildStage == STAGE_INITIALIZING) attributes.add("target");
if (xRefBuildStage == STAGE_INITIALIZING) attributes.add("xRef");
return "Cannot build GwtStepImplementationDto, attribute initializers form cycle " + attributes;
}
}
private String taskInitialize() {
return GwtStepImplementationDto.super.task();
}
private String typeInitialize() {
return GwtStepImplementationDto.super.type();
}
private String targetInitialize() {
return GwtStepImplementationDto.super.target();
}
private String xRefInitialize() {
return GwtStepImplementationDto.super.xRef();
}
/**
* @return The value of the {@code task} attribute
*/
@JsonProperty("task")
@Override
public String task() {
InitShim shim = this.initShim;
return shim != null
? shim.task()
: this.task;
}
/**
* @return The value of the {@code type} attribute
*/
@JsonProperty("type")
@Override
public String type() {
InitShim shim = this.initShim;
return shim != null
? shim.type()
: this.type;
}
/**
* @return The value of the {@code target} attribute
*/
@JsonProperty("target")
@Override
public String target() {
InitShim shim = this.initShim;
return shim != null
? shim.target()
: this.target;
}
/**
* @return The value of the {@code xRef} attribute
*/
@JsonProperty("x-$ref")
@Override
public String xRef() {
InitShim shim = this.initShim;
return shim != null
? shim.xRef()
: this.xRef;
}
/**
* @return The value of the {@code inputs} attribute
*/
@JsonProperty("inputs")
@Override
public Map inputs() {
return inputs;
}
/**
* @return The value of the {@code outputs} attribute
*/
@JsonProperty("outputs")
@Override
public Map outputs() {
return outputs;
}
/**
* @return The value of the {@code validations} attribute
*/
@JsonProperty("validations")
@Override
public Map validations() {
return validations;
}
/**
* Copy the current immutable object by setting a value for the {@link GwtStepImplementationDto#task() task} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for task
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtStepImplementationDto withTask(String value) {
String newValue = Objects.requireNonNull(value, "task");
if (this.task.equals(newValue)) return this;
return new ImmutableGwtStepImplementationDto(newValue, this.type, this.target, this.xRef, this.inputs, this.outputs, this.validations);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtStepImplementationDto#type() type} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for type
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtStepImplementationDto withType(String value) {
String newValue = Objects.requireNonNull(value, "type");
if (this.type.equals(newValue)) return this;
return new ImmutableGwtStepImplementationDto(this.task, newValue, this.target, this.xRef, this.inputs, this.outputs, this.validations);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtStepImplementationDto#target() target} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for target
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtStepImplementationDto withTarget(String value) {
String newValue = Objects.requireNonNull(value, "target");
if (this.target.equals(newValue)) return this;
return new ImmutableGwtStepImplementationDto(this.task, this.type, newValue, this.xRef, this.inputs, this.outputs, this.validations);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtStepImplementationDto#xRef() xRef} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for xRef
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtStepImplementationDto withXRef(String value) {
String newValue = Objects.requireNonNull(value, "xRef");
if (this.xRef.equals(newValue)) return this;
return new ImmutableGwtStepImplementationDto(this.task, this.type, this.target, newValue, this.inputs, this.outputs, this.validations);
}
/**
* Copy the current immutable object by replacing the {@link GwtStepImplementationDto#inputs() inputs} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the inputs map
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtStepImplementationDto withInputs(Map entries) {
if (this.inputs == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return new ImmutableGwtStepImplementationDto(this.task, this.type, this.target, this.xRef, newValue, this.outputs, this.validations);
}
/**
* Copy the current immutable object by replacing the {@link GwtStepImplementationDto#outputs() outputs} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the outputs map
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtStepImplementationDto withOutputs(Map entries) {
if (this.outputs == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return new ImmutableGwtStepImplementationDto(this.task, this.type, this.target, this.xRef, this.inputs, newValue, this.validations);
}
/**
* Copy the current immutable object by replacing the {@link GwtStepImplementationDto#validations() validations} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the validations map
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtStepImplementationDto withValidations(Map entries) {
if (this.validations == entries) return this;
Map newValue = createUnmodifiableMap(true, false, entries);
return new ImmutableGwtStepImplementationDto(this.task, this.type, this.target, this.xRef, this.inputs, this.outputs, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableGwtStepImplementationDto} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableGwtStepImplementationDto
&& equalTo((ImmutableGwtStepImplementationDto) another);
}
private boolean equalTo(ImmutableGwtStepImplementationDto another) {
return task.equals(another.task)
&& type.equals(another.type)
&& target.equals(another.target)
&& xRef.equals(another.xRef)
&& inputs.equals(another.inputs)
&& outputs.equals(another.outputs)
&& validations.equals(another.validations);
}
/**
* Computes a hash code from attributes: {@code task}, {@code type}, {@code target}, {@code xRef}, {@code inputs}, {@code outputs}, {@code validations}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + task.hashCode();
h += (h << 5) + type.hashCode();
h += (h << 5) + target.hashCode();
h += (h << 5) + xRef.hashCode();
h += (h << 5) + inputs.hashCode();
h += (h << 5) + outputs.hashCode();
h += (h << 5) + validations.hashCode();
return h;
}
/**
* Prints the immutable value {@code GwtStepImplementationDto} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return "GwtStepImplementationDto{"
+ "task=" + task
+ ", type=" + type
+ ", target=" + target
+ ", xRef=" + xRef
+ ", inputs=" + inputs
+ ", outputs=" + outputs
+ ", validations=" + validations
+ "}";
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "GwtStepImplementationDto", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements GwtStepImplementationDto {
@Nullable String task;
@Nullable String type;
@Nullable String target;
@Nullable String xRef;
@Nullable Map inputs = Collections.emptyMap();
@Nullable Map outputs = Collections.emptyMap();
@Nullable Map validations = Collections.emptyMap();
@JsonProperty("task")
public void setTask(String task) {
this.task = task;
}
@JsonProperty("type")
public void setType(String type) {
this.type = type;
}
@JsonProperty("target")
public void setTarget(String target) {
this.target = target;
}
@JsonProperty("x-$ref")
public void setXRef(String xRef) {
this.xRef = xRef;
}
@JsonProperty("inputs")
public void setInputs(Map inputs) {
this.inputs = inputs;
}
@JsonProperty("outputs")
public void setOutputs(Map outputs) {
this.outputs = outputs;
}
@JsonProperty("validations")
public void setValidations(Map validations) {
this.validations = validations;
}
@Override
public String task() { throw new UnsupportedOperationException(); }
@Override
public String type() { throw new UnsupportedOperationException(); }
@Override
public String target() { throw new UnsupportedOperationException(); }
@Override
public String xRef() { throw new UnsupportedOperationException(); }
@Override
public Map inputs() { throw new UnsupportedOperationException(); }
@Override
public Map outputs() { throw new UnsupportedOperationException(); }
@Override
public Map validations() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableGwtStepImplementationDto fromJson(Json json) {
ImmutableGwtStepImplementationDto.Builder builder = ImmutableGwtStepImplementationDto.builder();
if (json.task != null) {
builder.task(json.task);
}
if (json.type != null) {
builder.type(json.type);
}
if (json.target != null) {
builder.target(json.target);
}
if (json.xRef != null) {
builder.xRef(json.xRef);
}
if (json.inputs != null) {
builder.putAllInputs(json.inputs);
}
if (json.outputs != null) {
builder.putAllOutputs(json.outputs);
}
if (json.validations != null) {
builder.putAllValidations(json.validations);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link GwtStepImplementationDto} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable GwtStepImplementationDto instance
*/
public static ImmutableGwtStepImplementationDto copyOf(GwtStepImplementationDto instance) {
if (instance instanceof ImmutableGwtStepImplementationDto) {
return (ImmutableGwtStepImplementationDto) instance;
}
return ImmutableGwtStepImplementationDto.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableGwtStepImplementationDto ImmutableGwtStepImplementationDto}.
*
* ImmutableGwtStepImplementationDto.builder()
* .task(String) // optional {@link GwtStepImplementationDto#task() task}
* .type(String) // optional {@link GwtStepImplementationDto#type() type}
* .target(String) // optional {@link GwtStepImplementationDto#target() target}
* .xRef(String) // optional {@link GwtStepImplementationDto#xRef() xRef}
* .putInputs|putAllInputs(String => Object) // {@link GwtStepImplementationDto#inputs() inputs} mappings
* .putOutputs|putAllOutputs(String => Object) // {@link GwtStepImplementationDto#outputs() outputs} mappings
* .putValidations|putAllValidations(String => Object) // {@link GwtStepImplementationDto#validations() validations} mappings
* .build();
*
* @return A new ImmutableGwtStepImplementationDto builder
*/
public static ImmutableGwtStepImplementationDto.Builder builder() {
return new ImmutableGwtStepImplementationDto.Builder();
}
/**
* Builds instances of type {@link ImmutableGwtStepImplementationDto ImmutableGwtStepImplementationDto}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "GwtStepImplementationDto", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private @Nullable String task;
private @Nullable String type;
private @Nullable String target;
private @Nullable String xRef;
private Map inputs = new LinkedHashMap();
private Map outputs = new LinkedHashMap();
private Map validations = new LinkedHashMap();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code GwtStepImplementationDto} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(GwtStepImplementationDto instance) {
Objects.requireNonNull(instance, "instance");
task(instance.task());
type(instance.type());
target(instance.target());
xRef(instance.xRef());
putAllInputs(instance.inputs());
putAllOutputs(instance.outputs());
putAllValidations(instance.validations());
return this;
}
/**
* Initializes the value for the {@link GwtStepImplementationDto#task() task} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link GwtStepImplementationDto#task() task}.
* @param task The value for task
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("task")
public final Builder task(String task) {
this.task = Objects.requireNonNull(task, "task");
return this;
}
/**
* Initializes the value for the {@link GwtStepImplementationDto#type() type} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GwtStepImplementationDto#type() type}.
* @param type The value for type
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("type")
public final Builder type(String type) {
this.type = Objects.requireNonNull(type, "type");
return this;
}
/**
* Initializes the value for the {@link GwtStepImplementationDto#target() target} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GwtStepImplementationDto#target() target}.
* @param target The value for target
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("target")
public final Builder target(String target) {
this.target = Objects.requireNonNull(target, "target");
return this;
}
/**
* Initializes the value for the {@link GwtStepImplementationDto#xRef() xRef} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GwtStepImplementationDto#xRef() xRef}.
* @param xRef The value for xRef
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("x-$ref")
public final Builder xRef(String xRef) {
this.xRef = Objects.requireNonNull(xRef, "xRef");
return this;
}
/**
* Put one entry to the {@link GwtStepImplementationDto#inputs() inputs} map.
* @param key The key in the inputs map
* @param value The associated value in the inputs map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putInputs(String key, Object value) {
this.inputs.put(
Objects.requireNonNull(key, "inputs key"),
Objects.requireNonNull(value, "inputs value"));
return this;
}
/**
* Put one entry to the {@link GwtStepImplementationDto#inputs() inputs} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putInputs(Map.Entry entry) {
String k = entry.getKey();
Object v = entry.getValue();
this.inputs.put(
Objects.requireNonNull(k, "inputs key"),
Objects.requireNonNull(v, "inputs value"));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link GwtStepImplementationDto#inputs() inputs} map. Nulls are not permitted
* @param entries The entries that will be added to the inputs map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("inputs")
public final Builder inputs(Map entries) {
this.inputs.clear();
return putAllInputs(entries);
}
/**
* Put all mappings from the specified map as entries to {@link GwtStepImplementationDto#inputs() inputs} map. Nulls are not permitted
* @param entries The entries that will be added to the inputs map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllInputs(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
Object v = e.getValue();
this.inputs.put(
Objects.requireNonNull(k, "inputs key"),
Objects.requireNonNull(v, "inputs value"));
}
return this;
}
/**
* Put one entry to the {@link GwtStepImplementationDto#outputs() outputs} map.
* @param key The key in the outputs map
* @param value The associated value in the outputs map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putOutputs(String key, Object value) {
this.outputs.put(
Objects.requireNonNull(key, "outputs key"),
Objects.requireNonNull(value, "outputs value"));
return this;
}
/**
* Put one entry to the {@link GwtStepImplementationDto#outputs() outputs} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putOutputs(Map.Entry entry) {
String k = entry.getKey();
Object v = entry.getValue();
this.outputs.put(
Objects.requireNonNull(k, "outputs key"),
Objects.requireNonNull(v, "outputs value"));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link GwtStepImplementationDto#outputs() outputs} map. Nulls are not permitted
* @param entries The entries that will be added to the outputs map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("outputs")
public final Builder outputs(Map entries) {
this.outputs.clear();
return putAllOutputs(entries);
}
/**
* Put all mappings from the specified map as entries to {@link GwtStepImplementationDto#outputs() outputs} map. Nulls are not permitted
* @param entries The entries that will be added to the outputs map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllOutputs(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
Object v = e.getValue();
this.outputs.put(
Objects.requireNonNull(k, "outputs key"),
Objects.requireNonNull(v, "outputs value"));
}
return this;
}
/**
* Put one entry to the {@link GwtStepImplementationDto#validations() validations} map.
* @param key The key in the validations map
* @param value The associated value in the validations map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putValidations(String key, Object value) {
this.validations.put(
Objects.requireNonNull(key, "validations key"),
Objects.requireNonNull(value, "validations value"));
return this;
}
/**
* Put one entry to the {@link GwtStepImplementationDto#validations() validations} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putValidations(Map.Entry entry) {
String k = entry.getKey();
Object v = entry.getValue();
this.validations.put(
Objects.requireNonNull(k, "validations key"),
Objects.requireNonNull(v, "validations value"));
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link GwtStepImplementationDto#validations() validations} map. Nulls are not permitted
* @param entries The entries that will be added to the validations map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("validations")
public final Builder validations(Map entries) {
this.validations.clear();
return putAllValidations(entries);
}
/**
* Put all mappings from the specified map as entries to {@link GwtStepImplementationDto#validations() validations} map. Nulls are not permitted
* @param entries The entries that will be added to the validations map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllValidations(Map entries) {
for (Map.Entry e : entries.entrySet()) {
String k = e.getKey();
Object v = e.getValue();
this.validations.put(
Objects.requireNonNull(k, "validations key"),
Objects.requireNonNull(v, "validations value"));
}
return this;
}
/**
* Builds a new {@link ImmutableGwtStepImplementationDto ImmutableGwtStepImplementationDto}.
* @return An immutable instance of GwtStepImplementationDto
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableGwtStepImplementationDto build() {
return new ImmutableGwtStepImplementationDto(this);
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size());
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, "value");
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}