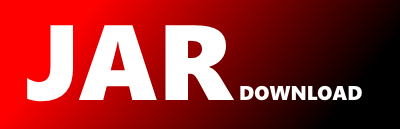
com.chutneytesting.design.domain.dataset.DataSet Maven / Gradle / Ivy
package com.chutneytesting.design.domain.dataset;
import static java.time.temporal.ChronoUnit.MILLIS;
import static java.util.Collections.emptyList;
import static java.util.Collections.emptyMap;
import static java.util.Optional.ofNullable;
import static java.util.stream.Collectors.toList;
import static org.apache.commons.lang3.StringUtils.isNotBlank;
import java.time.Instant;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.stream.Collectors;
import org.apache.commons.lang3.StringUtils;
public class DataSet {
public final String id;
public final String name;
public final String description;
public final Instant creationDate;
public final List tags;
public final Map constants;
public final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy