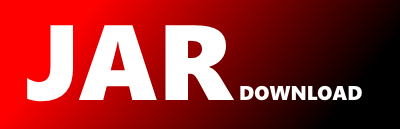
com.chutneytesting.execution.domain.compiler.ComposedTestCaseParametersResolutionPreProcessor Maven / Gradle / Ivy
package com.chutneytesting.execution.domain.compiler;
import static java.util.Collections.emptyMap;
import static java.util.Optional.ofNullable;
import static java.util.function.Function.identity;
import static java.util.stream.Collectors.toMap;
import static org.apache.commons.lang3.StringUtils.isBlank;
import com.chutneytesting.design.domain.globalvar.GlobalvarRepository;
import com.chutneytesting.design.domain.scenario.TestCaseMetadata;
import com.chutneytesting.design.domain.scenario.TestCaseMetadataImpl;
import com.chutneytesting.design.domain.scenario.compose.Strategy;
import com.chutneytesting.execution.domain.ExecutionRequest;
import com.chutneytesting.execution.domain.scenario.composed.ExecutableComposedScenario;
import com.chutneytesting.execution.domain.scenario.composed.ExecutableComposedStep;
import com.chutneytesting.execution.domain.scenario.composed.ExecutableComposedTestCase;
import com.chutneytesting.execution.domain.scenario.composed.StepImplementation;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.IOException;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.stream.Collectors;
import org.apache.commons.text.StringEscapeUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ComposedTestCaseParametersResolutionPreProcessor implements TestCasePreProcessor {
private static final Logger LOGGER = LoggerFactory.getLogger(ComposedTestCaseParametersResolutionPreProcessor.class);
private final GlobalvarRepository globalvarRepository;
private final ObjectMapper objectMapper;
ComposedTestCaseParametersResolutionPreProcessor(GlobalvarRepository globalvarRepository, ObjectMapper objectMapper) {
this.globalvarRepository = globalvarRepository;
this.objectMapper = objectMapper;
}
@Override
public ExecutableComposedTestCase apply(ExecutionRequest executionRequest) {
return this.apply((ExecutableComposedTestCase) executionRequest.testCase, executionRequest.environment);
}
private ExecutableComposedTestCase apply(ExecutableComposedTestCase testCase, String environment) {
Map globalVariables = initGlobalVariables(globalvarRepository);
makeEnvironmentNameAsGlobalVariable(globalVariables, environment);
ExecutableComposedTestCase newTestCase = this.applyOnStrategy(testCase, globalVariables);
return new ExecutableComposedTestCase(
applyToMetadata(newTestCase.metadata, newTestCase.executionParameters, globalVariables),
applyToScenario(newTestCase.composedScenario, newTestCase.executionParameters, globalVariables),
newTestCase.executionParameters);
}
public ExecutableComposedTestCase applyOnStrategy(ExecutableComposedTestCase testCase, Map globalVariable) {
Map testCaseDataSet = applyOnCurrentStepDataSet(testCase.executionParameters, emptyMap(), globalVariable);
return new ExecutableComposedTestCase(
testCase.metadata,
applyOnStrategy(testCase.composedScenario, testCaseDataSet, globalVariable),
testCaseDataSet
);
}
private TestCaseMetadata applyToMetadata(TestCaseMetadata metadata, Map dataSet, Map globalVariable) {
return TestCaseMetadataImpl.TestCaseMetadataBuilder
.from(metadata)
.withTitle(replaceParams(metadata.title(), globalVariable, dataSet))
.withDescription(replaceParams(metadata.description(), globalVariable, dataSet))
.build();
}
private ExecutableComposedScenario applyToScenario(ExecutableComposedScenario composedScenario, Map testCaseDataSet, Map globalVariable) {
return ExecutableComposedScenario.builder()
.withComposedSteps(
composedScenario.composedSteps.stream()
.map(step -> applyToComposedStep(step, testCaseDataSet, globalVariable))
.collect(Collectors.toList())
)
.withParameters(composedScenario.parameters)
.build();
}
private ExecutableComposedStep applyToComposedStep(ExecutableComposedStep composedStep, Map parentDataset, Map globalVariable) {
Map scopedDataset = applyOnCurrentStepDataSet(composedStep.executionParameters, parentDataset, globalVariable);
List subSteps = composedStep.steps;
// Preprocess substeps - Recurse
return ExecutableComposedStep.builder()
.withName(replaceParams(composedStep.name, globalVariable, scopedDataset))
.withSteps(
subSteps.stream()
.map(f -> applyToComposedStep(f, scopedDataset, globalVariable))
.collect(Collectors.toList())
)
.withImplementation(composedStep.stepImplementation.flatMap(si -> applyToImplementation(si, scopedDataset, globalVariable)))
.withStrategy(composedStep.strategy)
.withExecutionParameters(scopedDataset)
.build();
}
private Optional applyToImplementation(StepImplementation stepImplementation, Map scopedDataset, Map globalVariable) {
try {
String blob = replaceParams(objectMapper.writeValueAsString(stepImplementation), globalVariable, scopedDataset, StringEscapeUtils::escapeJson);
StepImplementation impl = objectMapper.readValue(blob, StepImplementation.class);
return ofNullable(impl);
} catch (IOException e) {
LOGGER.error("Error reading step implementation", e);
return ofNullable(stepImplementation);
}
}
private Map applyOnCurrentStepDataSet(Map currentStepDataset, Map parentDataset, Map globalVariables) {
Map scopedDataset = new HashMap<>();
Map>> splitDataSet = currentStepDataset.entrySet().stream().collect(Collectors.groupingBy(o -> isBlank(o.getValue())));
ofNullable(splitDataSet.get(true))
.ifPresent(l -> l.forEach(e ->
scopedDataset.put(e.getKey(), ofNullable(parentDataset.get(e.getKey())).orElse(""))
));
ofNullable(splitDataSet.get(false))
.ifPresent(l -> l.forEach(e ->
scopedDataset.put(e.getKey(), replaceParams(e.getValue(), globalVariables, parentDataset))
));
return scopedDataset;
}
private ExecutableComposedScenario applyOnStrategy(ExecutableComposedScenario composedScenario, Map testCaseDataSet, Map globalVariable) {
return ExecutableComposedScenario.builder()
.withComposedSteps(
composedScenario.composedSteps.stream()
.map(step -> applyOnStepStrategy(step, testCaseDataSet, globalVariable))
.collect(Collectors.toList())
)
.withParameters(composedScenario.parameters)
.build();
}
private ExecutableComposedStep applyOnStepStrategy(ExecutableComposedStep composedStep, Map parentDataset, Map globalVariable) {
Map scopedDataset = applyOnCurrentStepDataSet(composedStep.executionParameters, parentDataset, globalVariable);
return ExecutableComposedStep.builder()
.withName(composedStep.name)
.withSteps(
composedStep.steps.stream()
.map(f -> applyOnStepStrategy(f, scopedDataset, globalVariable))
.collect(Collectors.toList())
)
.withImplementation(composedStep.stepImplementation)
.withStrategy(applyToStrategy(composedStep.strategy, scopedDataset, globalVariable))
.withExecutionParameters(composedStep.executionParameters)
.build();
}
private Strategy applyToStrategy(Strategy strategy, Map scopedDataset, Map globalVariable) {
Map parameters = new HashMap<>();
strategy.parameters.forEach((key, value) -> parameters.put(key, replaceParams(value.toString(), scopedDataset, globalVariable)));
return new Strategy(strategy.type, parameters);
}
private Map initGlobalVariables(GlobalvarRepository globalvarRepository) {
Map flatMap = globalvarRepository.getFlatMap();
Map collect = resolveMapValues(flatMap);
while (!collect.equals(flatMap)) {
flatMap = collect;
collect = resolveMapValues(flatMap);
}
return collect;
}
private Map resolveMapValues(Map map) {
return map.entrySet().stream()
.collect(toMap(Map.Entry::getKey, e -> replaceParams(map, e.getValue(), identity())));
}
private void makeEnvironmentNameAsGlobalVariable(Map globalVariable, String environment) {
if (environment != null && !environment.isEmpty()) {
globalVariable.put("environment", environment);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy