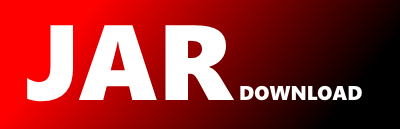
annotations.com.chutneytesting.agent.domain.configure.ImmutableNetworkConfiguration Maven / Gradle / Ivy
package com.chutneytesting.agent.domain.configure;
import com.chutneytesting.engine.domain.delegation.NamedHostAndPort;
import com.chutneytesting.environment.api.environment.dto.EnvironmentDto;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableSet;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.time.Instant;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Set;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link NetworkConfiguration}.
*
* Use the builder to create immutable instances:
* {@code ImmutableNetworkConfiguration.builder()}.
*/
@Generated(from = "NetworkConfiguration", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableNetworkConfiguration
implements NetworkConfiguration {
private final Instant creationDate;
private final NetworkConfiguration.AgentNetworkConfiguration agentNetworkConfiguration;
private final NetworkConfiguration.EnvironmentConfiguration environmentConfiguration;
private ImmutableNetworkConfiguration(
Instant creationDate,
NetworkConfiguration.AgentNetworkConfiguration agentNetworkConfiguration,
NetworkConfiguration.EnvironmentConfiguration environmentConfiguration) {
this.creationDate = creationDate;
this.agentNetworkConfiguration = agentNetworkConfiguration;
this.environmentConfiguration = environmentConfiguration;
}
/**
* Used to determine if a configuration have already been applied,
* and to avoid infinite-loop / dead-lock when propagating the configuration in the agent network.
*/
@Override
public Instant creationDate() {
return creationDate;
}
/**
* Set of {@link NamedHostAndPort} representing the agent network.
*/
@Override
public NetworkConfiguration.AgentNetworkConfiguration agentNetworkConfiguration() {
return agentNetworkConfiguration;
}
/**
* Set of {@link Environment} representing environment declared.
*/
@Override
public NetworkConfiguration.EnvironmentConfiguration environmentConfiguration() {
return environmentConfiguration;
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkConfiguration#creationDate() creationDate} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for creationDate
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkConfiguration withCreationDate(Instant value) {
if (this.creationDate == value) return this;
Instant newValue = Objects.requireNonNull(value, "creationDate");
return new ImmutableNetworkConfiguration(newValue, this.agentNetworkConfiguration, this.environmentConfiguration);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkConfiguration#agentNetworkConfiguration() agentNetworkConfiguration} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for agentNetworkConfiguration
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkConfiguration withAgentNetworkConfiguration(NetworkConfiguration.AgentNetworkConfiguration value) {
if (this.agentNetworkConfiguration == value) return this;
NetworkConfiguration.AgentNetworkConfiguration newValue = Objects.requireNonNull(value, "agentNetworkConfiguration");
return new ImmutableNetworkConfiguration(this.creationDate, newValue, this.environmentConfiguration);
}
/**
* Copy the current immutable object by setting a value for the {@link NetworkConfiguration#environmentConfiguration() environmentConfiguration} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for environmentConfiguration
* @return A modified copy of the {@code this} object
*/
public final ImmutableNetworkConfiguration withEnvironmentConfiguration(NetworkConfiguration.EnvironmentConfiguration value) {
if (this.environmentConfiguration == value) return this;
NetworkConfiguration.EnvironmentConfiguration newValue = Objects.requireNonNull(value, "environmentConfiguration");
return new ImmutableNetworkConfiguration(this.creationDate, this.agentNetworkConfiguration, newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableNetworkConfiguration} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableNetworkConfiguration
&& equalTo(0, (ImmutableNetworkConfiguration) another);
}
private boolean equalTo(int synthetic, ImmutableNetworkConfiguration another) {
return creationDate.equals(another.creationDate)
&& agentNetworkConfiguration.equals(another.agentNetworkConfiguration)
&& environmentConfiguration.equals(another.environmentConfiguration);
}
/**
* Computes a hash code from attributes: {@code creationDate}, {@code agentNetworkConfiguration}, {@code environmentConfiguration}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + creationDate.hashCode();
h += (h << 5) + agentNetworkConfiguration.hashCode();
h += (h << 5) + environmentConfiguration.hashCode();
return h;
}
/**
* Prints the immutable value {@code NetworkConfiguration} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("NetworkConfiguration")
.omitNullValues()
.add("creationDate", creationDate)
.add("agentNetworkConfiguration", agentNetworkConfiguration)
.add("environmentConfiguration", environmentConfiguration)
.toString();
}
/**
* Creates an immutable copy of a {@link NetworkConfiguration} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable NetworkConfiguration instance
*/
public static ImmutableNetworkConfiguration copyOf(NetworkConfiguration instance) {
if (instance instanceof ImmutableNetworkConfiguration) {
return (ImmutableNetworkConfiguration) instance;
}
return ImmutableNetworkConfiguration.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableNetworkConfiguration ImmutableNetworkConfiguration}.
*
* ImmutableNetworkConfiguration.builder()
* .creationDate(java.time.Instant) // required {@link NetworkConfiguration#creationDate() creationDate}
* .agentNetworkConfiguration(com.chutneytesting.agent.domain.configure.NetworkConfiguration.AgentNetworkConfiguration) // required {@link NetworkConfiguration#agentNetworkConfiguration() agentNetworkConfiguration}
* .environmentConfiguration(com.chutneytesting.agent.domain.configure.NetworkConfiguration.EnvironmentConfiguration) // required {@link NetworkConfiguration#environmentConfiguration() environmentConfiguration}
* .build();
*
* @return A new ImmutableNetworkConfiguration builder
*/
public static ImmutableNetworkConfiguration.Builder builder() {
return new ImmutableNetworkConfiguration.Builder();
}
/**
* Builds instances of type {@link ImmutableNetworkConfiguration ImmutableNetworkConfiguration}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "NetworkConfiguration", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_CREATION_DATE = 0x1L;
private static final long INIT_BIT_AGENT_NETWORK_CONFIGURATION = 0x2L;
private static final long INIT_BIT_ENVIRONMENT_CONFIGURATION = 0x4L;
private long initBits = 0x7L;
private @Nullable Instant creationDate;
private @Nullable NetworkConfiguration.AgentNetworkConfiguration agentNetworkConfiguration;
private @Nullable NetworkConfiguration.EnvironmentConfiguration environmentConfiguration;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code NetworkConfiguration} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(NetworkConfiguration instance) {
Objects.requireNonNull(instance, "instance");
creationDate(instance.creationDate());
agentNetworkConfiguration(instance.agentNetworkConfiguration());
environmentConfiguration(instance.environmentConfiguration());
return this;
}
/**
* Initializes the value for the {@link NetworkConfiguration#creationDate() creationDate} attribute.
* @param creationDate The value for creationDate
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder creationDate(Instant creationDate) {
this.creationDate = Objects.requireNonNull(creationDate, "creationDate");
initBits &= ~INIT_BIT_CREATION_DATE;
return this;
}
/**
* Initializes the value for the {@link NetworkConfiguration#agentNetworkConfiguration() agentNetworkConfiguration} attribute.
* @param agentNetworkConfiguration The value for agentNetworkConfiguration
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder agentNetworkConfiguration(NetworkConfiguration.AgentNetworkConfiguration agentNetworkConfiguration) {
this.agentNetworkConfiguration = Objects.requireNonNull(agentNetworkConfiguration, "agentNetworkConfiguration");
initBits &= ~INIT_BIT_AGENT_NETWORK_CONFIGURATION;
return this;
}
/**
* Initializes the value for the {@link NetworkConfiguration#environmentConfiguration() environmentConfiguration} attribute.
* @param environmentConfiguration The value for environmentConfiguration
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder environmentConfiguration(NetworkConfiguration.EnvironmentConfiguration environmentConfiguration) {
this.environmentConfiguration = Objects.requireNonNull(environmentConfiguration, "environmentConfiguration");
initBits &= ~INIT_BIT_ENVIRONMENT_CONFIGURATION;
return this;
}
/**
* Builds a new {@link ImmutableNetworkConfiguration ImmutableNetworkConfiguration}.
* @return An immutable instance of NetworkConfiguration
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableNetworkConfiguration build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableNetworkConfiguration(creationDate, agentNetworkConfiguration, environmentConfiguration);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_CREATION_DATE) != 0) attributes.add("creationDate");
if ((initBits & INIT_BIT_AGENT_NETWORK_CONFIGURATION) != 0) attributes.add("agentNetworkConfiguration");
if ((initBits & INIT_BIT_ENVIRONMENT_CONFIGURATION) != 0) attributes.add("environmentConfiguration");
return "Cannot build NetworkConfiguration, some of required attributes are not set " + attributes;
}
}
/**
* Immutable implementation of {@link NetworkConfiguration.AgentNetworkConfiguration}.
*
* Use the builder to create immutable instances:
* {@code ImmutableNetworkConfiguration.AgentNetworkConfiguration.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableNetworkConfiguration.AgentNetworkConfiguration.of()}.
*/
@Generated(from = "NetworkConfiguration.AgentNetworkConfiguration", generator = "Immutables")
@Immutable
@CheckReturnValue
public static final class AgentNetworkConfiguration
implements NetworkConfiguration.AgentNetworkConfiguration {
private final ImmutableSet agentInfos;
private AgentNetworkConfiguration(Iterable extends NamedHostAndPort> agentInfos) {
this.agentInfos = ImmutableSet.copyOf(agentInfos);
}
private AgentNetworkConfiguration(
ImmutableNetworkConfiguration.AgentNetworkConfiguration original,
ImmutableSet agentInfos) {
this.agentInfos = agentInfos;
}
/**
* @return The value of the {@code agentInfos} attribute
*/
@Override
public ImmutableSet agentInfos() {
return agentInfos;
}
/**
* Copy the current immutable object with elements that replace the content of {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkConfiguration.AgentNetworkConfiguration withAgentInfos(NamedHostAndPort... elements) {
ImmutableSet newValue = ImmutableSet.copyOf(elements);
return new ImmutableNetworkConfiguration.AgentNetworkConfiguration(this, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of agentInfos elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkConfiguration.AgentNetworkConfiguration withAgentInfos(Iterable extends NamedHostAndPort> elements) {
if (this.agentInfos == elements) return this;
ImmutableSet newValue = ImmutableSet.copyOf(elements);
return new ImmutableNetworkConfiguration.AgentNetworkConfiguration(this, newValue);
}
/**
* This instance is equal to all instances of {@code AgentNetworkConfiguration} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableNetworkConfiguration.AgentNetworkConfiguration
&& equalTo(0, (ImmutableNetworkConfiguration.AgentNetworkConfiguration) another);
}
private boolean equalTo(int synthetic, ImmutableNetworkConfiguration.AgentNetworkConfiguration another) {
return agentInfos.equals(another.agentInfos);
}
/**
* Computes a hash code from attributes: {@code agentInfos}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + agentInfos.hashCode();
return h;
}
/**
* Prints the immutable value {@code AgentNetworkConfiguration} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("AgentNetworkConfiguration")
.omitNullValues()
.add("agentInfos", agentInfos)
.toString();
}
/**
* Construct a new immutable {@code AgentNetworkConfiguration} instance.
* @param agentInfos The value for the {@code agentInfos} attribute
* @return An immutable AgentNetworkConfiguration instance
*/
public static ImmutableNetworkConfiguration.AgentNetworkConfiguration of(Set agentInfos) {
return of((Iterable extends NamedHostAndPort>) agentInfos);
}
/**
* Construct a new immutable {@code AgentNetworkConfiguration} instance.
* @param agentInfos The value for the {@code agentInfos} attribute
* @return An immutable AgentNetworkConfiguration instance
*/
public static ImmutableNetworkConfiguration.AgentNetworkConfiguration of(Iterable extends NamedHostAndPort> agentInfos) {
return new ImmutableNetworkConfiguration.AgentNetworkConfiguration(agentInfos);
}
/**
* Creates an immutable copy of a {@link NetworkConfiguration.AgentNetworkConfiguration} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable AgentNetworkConfiguration instance
*/
public static ImmutableNetworkConfiguration.AgentNetworkConfiguration copyOf(NetworkConfiguration.AgentNetworkConfiguration instance) {
if (instance instanceof ImmutableNetworkConfiguration.AgentNetworkConfiguration) {
return (ImmutableNetworkConfiguration.AgentNetworkConfiguration) instance;
}
return ImmutableNetworkConfiguration.AgentNetworkConfiguration.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableNetworkConfiguration.AgentNetworkConfiguration AgentNetworkConfiguration}.
*
* ImmutableNetworkConfiguration.AgentNetworkConfiguration.builder()
* .addAgentInfos|addAllAgentInfos(com.chutneytesting.engine.domain.delegation.NamedHostAndPort) // {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos} elements
* .build();
*
* @return A new AgentNetworkConfiguration builder
*/
public static ImmutableNetworkConfiguration.AgentNetworkConfiguration.Builder builder() {
return new ImmutableNetworkConfiguration.AgentNetworkConfiguration.Builder();
}
/**
* Builds instances of type {@link ImmutableNetworkConfiguration.AgentNetworkConfiguration AgentNetworkConfiguration}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "NetworkConfiguration.AgentNetworkConfiguration", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private ImmutableSet.Builder agentInfos = ImmutableSet.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code AgentNetworkConfiguration} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(NetworkConfiguration.AgentNetworkConfiguration instance) {
Objects.requireNonNull(instance, "instance");
addAllAgentInfos(instance.agentInfos());
return this;
}
/**
* Adds one element to {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos} set.
* @param element A agentInfos element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAgentInfos(NamedHostAndPort element) {
this.agentInfos.add(element);
return this;
}
/**
* Adds elements to {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos} set.
* @param elements An array of agentInfos elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAgentInfos(NamedHostAndPort... elements) {
this.agentInfos.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos} set.
* @param elements An iterable of agentInfos elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder agentInfos(Iterable extends NamedHostAndPort> elements) {
this.agentInfos = ImmutableSet.builder();
return addAllAgentInfos(elements);
}
/**
* Adds elements to {@link NetworkConfiguration.AgentNetworkConfiguration#agentInfos() agentInfos} set.
* @param elements An iterable of agentInfos elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllAgentInfos(Iterable extends NamedHostAndPort> elements) {
this.agentInfos.addAll(elements);
return this;
}
/**
* Builds a new {@link ImmutableNetworkConfiguration.AgentNetworkConfiguration AgentNetworkConfiguration}.
* @return An immutable instance of AgentNetworkConfiguration
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableNetworkConfiguration.AgentNetworkConfiguration build() {
return new ImmutableNetworkConfiguration.AgentNetworkConfiguration(null, agentInfos.build());
}
}
}
/**
* Immutable implementation of {@link NetworkConfiguration.EnvironmentConfiguration}.
*
* Use the builder to create immutable instances:
* {@code ImmutableNetworkConfiguration.EnvironmentConfiguration.builder()}.
* Use the static factory method to create immutable instances:
* {@code ImmutableNetworkConfiguration.EnvironmentConfiguration.of()}.
*/
@Generated(from = "NetworkConfiguration.EnvironmentConfiguration", generator = "Immutables")
@Immutable
@CheckReturnValue
public static final class EnvironmentConfiguration
implements NetworkConfiguration.EnvironmentConfiguration {
private final ImmutableSet environments;
private EnvironmentConfiguration(
Iterable extends EnvironmentDto> environments) {
this.environments = ImmutableSet.copyOf(environments);
}
private EnvironmentConfiguration(
ImmutableNetworkConfiguration.EnvironmentConfiguration original,
ImmutableSet environments) {
this.environments = environments;
}
/**
* @return The value of the {@code environments} attribute
*/
@Override
public ImmutableSet environments() {
return environments;
}
/**
* Copy the current immutable object with elements that replace the content of {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkConfiguration.EnvironmentConfiguration withEnvironments(EnvironmentDto... elements) {
ImmutableSet newValue = ImmutableSet.copyOf(elements);
return new ImmutableNetworkConfiguration.EnvironmentConfiguration(this, newValue);
}
/**
* Copy the current immutable object with elements that replace the content of {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of environments elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableNetworkConfiguration.EnvironmentConfiguration withEnvironments(Iterable extends EnvironmentDto> elements) {
if (this.environments == elements) return this;
ImmutableSet newValue = ImmutableSet.copyOf(elements);
return new ImmutableNetworkConfiguration.EnvironmentConfiguration(this, newValue);
}
/**
* This instance is equal to all instances of {@code EnvironmentConfiguration} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableNetworkConfiguration.EnvironmentConfiguration
&& equalTo(0, (ImmutableNetworkConfiguration.EnvironmentConfiguration) another);
}
private boolean equalTo(int synthetic, ImmutableNetworkConfiguration.EnvironmentConfiguration another) {
return environments.equals(another.environments);
}
/**
* Computes a hash code from attributes: {@code environments}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + environments.hashCode();
return h;
}
/**
* Prints the immutable value {@code EnvironmentConfiguration} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("EnvironmentConfiguration")
.omitNullValues()
.add("environments", environments)
.toString();
}
/**
* Construct a new immutable {@code EnvironmentConfiguration} instance.
* @param environments The value for the {@code environments} attribute
* @return An immutable EnvironmentConfiguration instance
*/
public static ImmutableNetworkConfiguration.EnvironmentConfiguration of(Set environments) {
return of((Iterable extends EnvironmentDto>) environments);
}
/**
* Construct a new immutable {@code EnvironmentConfiguration} instance.
* @param environments The value for the {@code environments} attribute
* @return An immutable EnvironmentConfiguration instance
*/
public static ImmutableNetworkConfiguration.EnvironmentConfiguration of(Iterable extends EnvironmentDto> environments) {
return new ImmutableNetworkConfiguration.EnvironmentConfiguration(environments);
}
/**
* Creates an immutable copy of a {@link NetworkConfiguration.EnvironmentConfiguration} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable EnvironmentConfiguration instance
*/
public static ImmutableNetworkConfiguration.EnvironmentConfiguration copyOf(NetworkConfiguration.EnvironmentConfiguration instance) {
if (instance instanceof ImmutableNetworkConfiguration.EnvironmentConfiguration) {
return (ImmutableNetworkConfiguration.EnvironmentConfiguration) instance;
}
return ImmutableNetworkConfiguration.EnvironmentConfiguration.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableNetworkConfiguration.EnvironmentConfiguration EnvironmentConfiguration}.
*
* ImmutableNetworkConfiguration.EnvironmentConfiguration.builder()
* .addEnvironments|addAllEnvironments(com.chutneytesting.environment.api.environment.dto.EnvironmentDto) // {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments} elements
* .build();
*
* @return A new EnvironmentConfiguration builder
*/
public static ImmutableNetworkConfiguration.EnvironmentConfiguration.Builder builder() {
return new ImmutableNetworkConfiguration.EnvironmentConfiguration.Builder();
}
/**
* Builds instances of type {@link ImmutableNetworkConfiguration.EnvironmentConfiguration EnvironmentConfiguration}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "NetworkConfiguration.EnvironmentConfiguration", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private ImmutableSet.Builder environments = ImmutableSet.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code EnvironmentConfiguration} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(NetworkConfiguration.EnvironmentConfiguration instance) {
Objects.requireNonNull(instance, "instance");
addAllEnvironments(instance.environments());
return this;
}
/**
* Adds one element to {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments} set.
* @param element A environments element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addEnvironments(EnvironmentDto element) {
this.environments.add(element);
return this;
}
/**
* Adds elements to {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments} set.
* @param elements An array of environments elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addEnvironments(EnvironmentDto... elements) {
this.environments.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments} set.
* @param elements An iterable of environments elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder environments(Iterable extends EnvironmentDto> elements) {
this.environments = ImmutableSet.builder();
return addAllEnvironments(elements);
}
/**
* Adds elements to {@link NetworkConfiguration.EnvironmentConfiguration#environments() environments} set.
* @param elements An iterable of environments elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllEnvironments(Iterable extends EnvironmentDto> elements) {
this.environments.addAll(elements);
return this;
}
/**
* Builds a new {@link ImmutableNetworkConfiguration.EnvironmentConfiguration EnvironmentConfiguration}.
* @return An immutable instance of EnvironmentConfiguration
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableNetworkConfiguration.EnvironmentConfiguration build() {
return new ImmutableNetworkConfiguration.EnvironmentConfiguration(null, environments.build());
}
}
}
}