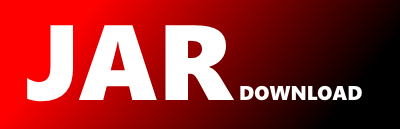
annotations.com.chutneytesting.dataset.api.ImmutableDataSetDto Maven / Gradle / Ivy
package com.chutneytesting.dataset.api;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.time.Instant;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link DataSetDto}.
*
* Use the builder to create immutable instances:
* {@code ImmutableDataSetDto.builder()}.
*/
@Generated(from = "DataSetDto", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableDataSetDto implements DataSetDto {
private final @Nullable String id;
private final String name;
private final String description;
private final Instant lastUpdated;
private final List tags;
private final List constants;
private final List> datatable;
private final List scenarioUsage;
private final List campaignUsage;
private final Map> scenarioInCampaignUsage;
private ImmutableDataSetDto(ImmutableDataSetDto.Builder builder) {
this.id = builder.id;
this.name = builder.name;
if (builder.description != null) {
initShim.description(builder.description);
}
if (builder.lastUpdated != null) {
initShim.lastUpdated(builder.lastUpdated);
}
if (builder.tagsIsSet()) {
initShim.tags(createUnmodifiableList(true, builder.tags));
}
if (builder.constantsIsSet()) {
initShim.constants(createUnmodifiableList(true, builder.constants));
}
if (builder.datatableIsSet()) {
initShim.datatable(createUnmodifiableList(true, builder.datatable));
}
if (builder.scenarioUsageIsSet()) {
initShim.scenarioUsage(createUnmodifiableList(true, builder.scenarioUsage));
}
if (builder.campaignUsageIsSet()) {
initShim.campaignUsage(createUnmodifiableList(true, builder.campaignUsage));
}
if (builder.scenarioInCampaignUsageIsSet()) {
initShim.scenarioInCampaignUsage(createUnmodifiableMap(false, false, builder.scenarioInCampaignUsage));
}
this.description = initShim.description();
this.lastUpdated = initShim.lastUpdated();
this.tags = initShim.tags();
this.constants = initShim.constants();
this.datatable = initShim.datatable();
this.scenarioUsage = initShim.scenarioUsage();
this.campaignUsage = initShim.campaignUsage();
this.scenarioInCampaignUsage = initShim.scenarioInCampaignUsage();
this.initShim = null;
}
private ImmutableDataSetDto(
@Nullable String id,
String name,
String description,
Instant lastUpdated,
List tags,
List constants,
List> datatable,
List scenarioUsage,
List campaignUsage,
Map> scenarioInCampaignUsage) {
this.id = id;
this.name = name;
this.description = description;
this.lastUpdated = lastUpdated;
this.tags = tags;
this.constants = constants;
this.datatable = datatable;
this.scenarioUsage = scenarioUsage;
this.campaignUsage = campaignUsage;
this.scenarioInCampaignUsage = scenarioInCampaignUsage;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "DataSetDto", generator = "Immutables")
private final class InitShim {
private byte descriptionBuildStage = STAGE_UNINITIALIZED;
private String description;
String description() {
if (descriptionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (descriptionBuildStage == STAGE_UNINITIALIZED) {
descriptionBuildStage = STAGE_INITIALIZING;
this.description = Objects.requireNonNull(descriptionInitialize(), "description");
descriptionBuildStage = STAGE_INITIALIZED;
}
return this.description;
}
void description(String description) {
this.description = description;
descriptionBuildStage = STAGE_INITIALIZED;
}
private byte lastUpdatedBuildStage = STAGE_UNINITIALIZED;
private Instant lastUpdated;
Instant lastUpdated() {
if (lastUpdatedBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (lastUpdatedBuildStage == STAGE_UNINITIALIZED) {
lastUpdatedBuildStage = STAGE_INITIALIZING;
this.lastUpdated = Objects.requireNonNull(lastUpdatedInitialize(), "lastUpdated");
lastUpdatedBuildStage = STAGE_INITIALIZED;
}
return this.lastUpdated;
}
void lastUpdated(Instant lastUpdated) {
this.lastUpdated = lastUpdated;
lastUpdatedBuildStage = STAGE_INITIALIZED;
}
private byte tagsBuildStage = STAGE_UNINITIALIZED;
private List tags;
List tags() {
if (tagsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (tagsBuildStage == STAGE_UNINITIALIZED) {
tagsBuildStage = STAGE_INITIALIZING;
this.tags = createUnmodifiableList(false, createSafeList(tagsInitialize(), true, false));
tagsBuildStage = STAGE_INITIALIZED;
}
return this.tags;
}
void tags(List tags) {
this.tags = tags;
tagsBuildStage = STAGE_INITIALIZED;
}
private byte constantsBuildStage = STAGE_UNINITIALIZED;
private List constants;
List constants() {
if (constantsBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (constantsBuildStage == STAGE_UNINITIALIZED) {
constantsBuildStage = STAGE_INITIALIZING;
this.constants = createUnmodifiableList(false, createSafeList(constantsInitialize(), true, false));
constantsBuildStage = STAGE_INITIALIZED;
}
return this.constants;
}
void constants(List constants) {
this.constants = constants;
constantsBuildStage = STAGE_INITIALIZED;
}
private byte datatableBuildStage = STAGE_UNINITIALIZED;
private List> datatable;
List> datatable() {
if (datatableBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (datatableBuildStage == STAGE_UNINITIALIZED) {
datatableBuildStage = STAGE_INITIALIZING;
this.datatable = createUnmodifiableList(false, createSafeList(datatableInitialize(), true, false));
datatableBuildStage = STAGE_INITIALIZED;
}
return this.datatable;
}
void datatable(List> datatable) {
this.datatable = datatable;
datatableBuildStage = STAGE_INITIALIZED;
}
private byte scenarioUsageBuildStage = STAGE_UNINITIALIZED;
private List scenarioUsage;
List scenarioUsage() {
if (scenarioUsageBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (scenarioUsageBuildStage == STAGE_UNINITIALIZED) {
scenarioUsageBuildStage = STAGE_INITIALIZING;
this.scenarioUsage = createUnmodifiableList(false, createSafeList(scenarioUsageInitialize(), true, false));
scenarioUsageBuildStage = STAGE_INITIALIZED;
}
return this.scenarioUsage;
}
void scenarioUsage(List scenarioUsage) {
this.scenarioUsage = scenarioUsage;
scenarioUsageBuildStage = STAGE_INITIALIZED;
}
private byte campaignUsageBuildStage = STAGE_UNINITIALIZED;
private List campaignUsage;
List campaignUsage() {
if (campaignUsageBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (campaignUsageBuildStage == STAGE_UNINITIALIZED) {
campaignUsageBuildStage = STAGE_INITIALIZING;
this.campaignUsage = createUnmodifiableList(false, createSafeList(campaignUsageInitialize(), true, false));
campaignUsageBuildStage = STAGE_INITIALIZED;
}
return this.campaignUsage;
}
void campaignUsage(List campaignUsage) {
this.campaignUsage = campaignUsage;
campaignUsageBuildStage = STAGE_INITIALIZED;
}
private byte scenarioInCampaignUsageBuildStage = STAGE_UNINITIALIZED;
private Map> scenarioInCampaignUsage;
Map> scenarioInCampaignUsage() {
if (scenarioInCampaignUsageBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (scenarioInCampaignUsageBuildStage == STAGE_UNINITIALIZED) {
scenarioInCampaignUsageBuildStage = STAGE_INITIALIZING;
this.scenarioInCampaignUsage = createUnmodifiableMap(true, false, scenarioInCampaignUsageInitialize());
scenarioInCampaignUsageBuildStage = STAGE_INITIALIZED;
}
return this.scenarioInCampaignUsage;
}
void scenarioInCampaignUsage(Map> scenarioInCampaignUsage) {
this.scenarioInCampaignUsage = scenarioInCampaignUsage;
scenarioInCampaignUsageBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (descriptionBuildStage == STAGE_INITIALIZING) attributes.add("description");
if (lastUpdatedBuildStage == STAGE_INITIALIZING) attributes.add("lastUpdated");
if (tagsBuildStage == STAGE_INITIALIZING) attributes.add("tags");
if (constantsBuildStage == STAGE_INITIALIZING) attributes.add("constants");
if (datatableBuildStage == STAGE_INITIALIZING) attributes.add("datatable");
if (scenarioUsageBuildStage == STAGE_INITIALIZING) attributes.add("scenarioUsage");
if (campaignUsageBuildStage == STAGE_INITIALIZING) attributes.add("campaignUsage");
if (scenarioInCampaignUsageBuildStage == STAGE_INITIALIZING) attributes.add("scenarioInCampaignUsage");
return "Cannot build DataSetDto, attribute initializers form cycle " + attributes;
}
}
private String descriptionInitialize() {
return DataSetDto.super.description();
}
private Instant lastUpdatedInitialize() {
return DataSetDto.super.lastUpdated();
}
private List tagsInitialize() {
return DataSetDto.super.tags();
}
private List constantsInitialize() {
return DataSetDto.super.constants();
}
private List> datatableInitialize() {
return DataSetDto.super.datatable();
}
private List scenarioUsageInitialize() {
return DataSetDto.super.scenarioUsage();
}
private List campaignUsageInitialize() {
return DataSetDto.super.campaignUsage();
}
private Map> scenarioInCampaignUsageInitialize() {
return DataSetDto.super.scenarioInCampaignUsage();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public Optional id() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public String name() {
return name;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public String description() {
InitShim shim = this.initShim;
return shim != null
? shim.description()
: this.description;
}
/**
* @return The value of the {@code lastUpdated} attribute
*/
@JsonProperty("lastUpdated")
@Override
public Instant lastUpdated() {
InitShim shim = this.initShim;
return shim != null
? shim.lastUpdated()
: this.lastUpdated;
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public List tags() {
InitShim shim = this.initShim;
return shim != null
? shim.tags()
: this.tags;
}
/**
* @return The value of the {@code constants} attribute
*/
@JsonProperty("uniqueValues")
@Override
public List constants() {
InitShim shim = this.initShim;
return shim != null
? shim.constants()
: this.constants;
}
/**
* @return The value of the {@code datatable} attribute
*/
@JsonProperty("multipleValues")
@Override
public List> datatable() {
InitShim shim = this.initShim;
return shim != null
? shim.datatable()
: this.datatable;
}
/**
* @return The value of the {@code scenarioUsage} attribute
*/
@JsonProperty("scenarioUsage")
@Override
public List scenarioUsage() {
InitShim shim = this.initShim;
return shim != null
? shim.scenarioUsage()
: this.scenarioUsage;
}
/**
* @return The value of the {@code campaignUsage} attribute
*/
@JsonProperty("campaignUsage")
@Override
public List campaignUsage() {
InitShim shim = this.initShim;
return shim != null
? shim.campaignUsage()
: this.campaignUsage;
}
/**
* @return The value of the {@code scenarioInCampaignUsage} attribute
*/
@JsonProperty("scenarioInCampaignUsage")
@Override
public Map> scenarioInCampaignUsage() {
InitShim shim = this.initShim;
return shim != null
? shim.scenarioInCampaignUsage()
: this.scenarioInCampaignUsage;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link DataSetDto#id() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return new ImmutableDataSetDto(
newValue,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object by setting an optional value for the {@link DataSetDto#id() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return new ImmutableDataSetDto(
value,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object by setting a value for the {@link DataSetDto#name() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableDataSetDto withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableDataSetDto(
this.id,
newValue,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object by setting a value for the {@link DataSetDto#description() description} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for description
* @return A modified copy of the {@code this} object
*/
public final ImmutableDataSetDto withDescription(String value) {
String newValue = Objects.requireNonNull(value, "description");
if (this.description.equals(newValue)) return this;
return new ImmutableDataSetDto(
this.id,
this.name,
newValue,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object by setting a value for the {@link DataSetDto#lastUpdated() lastUpdated} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for lastUpdated
* @return A modified copy of the {@code this} object
*/
public final ImmutableDataSetDto withLastUpdated(Instant value) {
if (this.lastUpdated == value) return this;
Instant newValue = Objects.requireNonNull(value, "lastUpdated");
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
newValue,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#tags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withTags(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
newValue,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#tags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withTags(Iterable elements) {
if (this.tags == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
newValue,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#constants() constants}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withConstants(KeyValue... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
newValue,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#constants() constants}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of constants elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withConstants(Iterable extends KeyValue> elements) {
if (this.constants == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
newValue,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#datatable() datatable}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
@SafeVarargs @SuppressWarnings("varargs")
public final ImmutableDataSetDto withDatatable(List... elements) {
List> newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
newValue,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#datatable() datatable}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of datatable elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withDatatable(Iterable extends List> elements) {
if (this.datatable == elements) return this;
List> newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
newValue,
this.scenarioUsage,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#scenarioUsage() scenarioUsage}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withScenarioUsage(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
newValue,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#scenarioUsage() scenarioUsage}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of scenarioUsage elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withScenarioUsage(Iterable elements) {
if (this.scenarioUsage == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
newValue,
this.campaignUsage,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#campaignUsage() campaignUsage}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withCampaignUsage(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
newValue,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object with elements that replace the content of {@link DataSetDto#campaignUsage() campaignUsage}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of campaignUsage elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withCampaignUsage(Iterable elements) {
if (this.campaignUsage == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
newValue,
this.scenarioInCampaignUsage);
}
/**
* Copy the current immutable object by replacing the {@link DataSetDto#scenarioInCampaignUsage() scenarioInCampaignUsage} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the scenarioInCampaignUsage map
* @return A modified copy of {@code this} object
*/
public final ImmutableDataSetDto withScenarioInCampaignUsage(Map> entries) {
if (this.scenarioInCampaignUsage == entries) return this;
Map> newValue = createUnmodifiableMap(true, false, entries);
return new ImmutableDataSetDto(
this.id,
this.name,
this.description,
this.lastUpdated,
this.tags,
this.constants,
this.datatable,
this.scenarioUsage,
this.campaignUsage,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableDataSetDto} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableDataSetDto
&& equalTo(0, (ImmutableDataSetDto) another);
}
private boolean equalTo(int synthetic, ImmutableDataSetDto another) {
return Objects.equals(id, another.id)
&& name.equals(another.name)
&& description.equals(another.description)
&& lastUpdated.equals(another.lastUpdated)
&& tags.equals(another.tags)
&& constants.equals(another.constants)
&& datatable.equals(another.datatable)
&& scenarioUsage.equals(another.scenarioUsage)
&& campaignUsage.equals(another.campaignUsage)
&& scenarioInCampaignUsage.equals(another.scenarioInCampaignUsage);
}
/**
* Computes a hash code from attributes: {@code id}, {@code name}, {@code description}, {@code lastUpdated}, {@code tags}, {@code constants}, {@code datatable}, {@code scenarioUsage}, {@code campaignUsage}, {@code scenarioInCampaignUsage}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + name.hashCode();
h += (h << 5) + description.hashCode();
h += (h << 5) + lastUpdated.hashCode();
h += (h << 5) + tags.hashCode();
h += (h << 5) + constants.hashCode();
h += (h << 5) + datatable.hashCode();
h += (h << 5) + scenarioUsage.hashCode();
h += (h << 5) + campaignUsage.hashCode();
h += (h << 5) + scenarioInCampaignUsage.hashCode();
return h;
}
/**
* Prints the immutable value {@code DataSetDto} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("DataSetDto{");
if (id != null) {
builder.append("id=").append(id);
}
if (builder.length() > 11) builder.append(", ");
builder.append("name=").append(name);
builder.append(", ");
builder.append("description=").append(description);
builder.append(", ");
builder.append("lastUpdated=").append(lastUpdated);
builder.append(", ");
builder.append("tags=").append(tags);
builder.append(", ");
builder.append("constants=").append(constants);
builder.append(", ");
builder.append("datatable=").append(datatable);
builder.append(", ");
builder.append("scenarioUsage=").append(scenarioUsage);
builder.append(", ");
builder.append("campaignUsage=").append(campaignUsage);
builder.append(", ");
builder.append("scenarioInCampaignUsage=").append(scenarioInCampaignUsage);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "DataSetDto", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements DataSetDto {
@Nullable Optional id = Optional.empty();
@Nullable String name;
@Nullable String description;
@Nullable Instant lastUpdated;
@Nullable List tags = Collections.emptyList();
boolean tagsIsSet;
@Nullable List constants = Collections.emptyList();
boolean constantsIsSet;
@Nullable List> datatable = Collections.emptyList();
boolean datatableIsSet;
@Nullable List scenarioUsage = Collections.emptyList();
boolean scenarioUsageIsSet;
@Nullable List campaignUsage = Collections.emptyList();
boolean campaignUsageIsSet;
@Nullable Map> scenarioInCampaignUsage = Collections.emptyMap();
boolean scenarioInCampaignUsageIsSet;
@JsonProperty("id")
public void setId(Optional id) {
this.id = id;
}
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
@JsonProperty("description")
public void setDescription(String description) {
this.description = description;
}
@JsonProperty("lastUpdated")
public void setLastUpdated(Instant lastUpdated) {
this.lastUpdated = lastUpdated;
}
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
this.tagsIsSet = null != tags;
}
@JsonProperty("uniqueValues")
public void setConstants(List constants) {
this.constants = constants;
this.constantsIsSet = null != constants;
}
@JsonProperty("multipleValues")
public void setDatatable(List> datatable) {
this.datatable = datatable;
this.datatableIsSet = null != datatable;
}
@JsonProperty("scenarioUsage")
public void setScenarioUsage(List scenarioUsage) {
this.scenarioUsage = scenarioUsage;
this.scenarioUsageIsSet = null != scenarioUsage;
}
@JsonProperty("campaignUsage")
public void setCampaignUsage(List campaignUsage) {
this.campaignUsage = campaignUsage;
this.campaignUsageIsSet = null != campaignUsage;
}
@JsonProperty("scenarioInCampaignUsage")
public void setScenarioInCampaignUsage(Map> scenarioInCampaignUsage) {
this.scenarioInCampaignUsage = scenarioInCampaignUsage;
this.scenarioInCampaignUsageIsSet = null != scenarioInCampaignUsage;
}
@Override
public Optional id() { throw new UnsupportedOperationException(); }
@Override
public String name() { throw new UnsupportedOperationException(); }
@Override
public String description() { throw new UnsupportedOperationException(); }
@Override
public Instant lastUpdated() { throw new UnsupportedOperationException(); }
@Override
public List tags() { throw new UnsupportedOperationException(); }
@Override
public List constants() { throw new UnsupportedOperationException(); }
@Override
public List> datatable() { throw new UnsupportedOperationException(); }
@Override
public List scenarioUsage() { throw new UnsupportedOperationException(); }
@Override
public List campaignUsage() { throw new UnsupportedOperationException(); }
@Override
public Map> scenarioInCampaignUsage() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableDataSetDto fromJson(Json json) {
ImmutableDataSetDto.Builder builder = ImmutableDataSetDto.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.name != null) {
builder.name(json.name);
}
if (json.description != null) {
builder.description(json.description);
}
if (json.lastUpdated != null) {
builder.lastUpdated(json.lastUpdated);
}
if (json.tagsIsSet) {
builder.addAllTags(json.tags);
}
if (json.constantsIsSet) {
builder.addAllConstants(json.constants);
}
if (json.datatableIsSet) {
builder.addAllDatatable(json.datatable);
}
if (json.scenarioUsageIsSet) {
builder.addAllScenarioUsage(json.scenarioUsage);
}
if (json.campaignUsageIsSet) {
builder.addAllCampaignUsage(json.campaignUsage);
}
if (json.scenarioInCampaignUsageIsSet) {
builder.putAllScenarioInCampaignUsage(json.scenarioInCampaignUsage);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link DataSetDto} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable DataSetDto instance
*/
public static ImmutableDataSetDto copyOf(DataSetDto instance) {
if (instance instanceof ImmutableDataSetDto) {
return (ImmutableDataSetDto) instance;
}
return ImmutableDataSetDto.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableDataSetDto ImmutableDataSetDto}.
*
* ImmutableDataSetDto.builder()
* .id(String) // optional {@link DataSetDto#id() id}
* .name(String) // required {@link DataSetDto#name() name}
* .description(String) // optional {@link DataSetDto#description() description}
* .lastUpdated(java.time.Instant) // optional {@link DataSetDto#lastUpdated() lastUpdated}
* .addTags|addAllTags(String) // {@link DataSetDto#tags() tags} elements
* .addConstants|addAllConstants(com.chutneytesting.dataset.api.KeyValue) // {@link DataSetDto#constants() constants} elements
* .addDatatable|addAllDatatable(List<com.chutneytesting.dataset.api.KeyValue>) // {@link DataSetDto#datatable() datatable} elements
* .addScenarioUsage|addAllScenarioUsage(String) // {@link DataSetDto#scenarioUsage() scenarioUsage} elements
* .addCampaignUsage|addAllCampaignUsage(String) // {@link DataSetDto#campaignUsage() campaignUsage} elements
* .putScenarioInCampaignUsage|putAllScenarioInCampaignUsage(String => Set<String>) // {@link DataSetDto#scenarioInCampaignUsage() scenarioInCampaignUsage} mappings
* .build();
*
* @return A new ImmutableDataSetDto builder
*/
public static ImmutableDataSetDto.Builder builder() {
return new ImmutableDataSetDto.Builder();
}
/**
* Builds instances of type {@link ImmutableDataSetDto ImmutableDataSetDto}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "DataSetDto", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long OPT_BIT_TAGS = 0x1L;
private static final long OPT_BIT_CONSTANTS = 0x2L;
private static final long OPT_BIT_DATATABLE = 0x4L;
private static final long OPT_BIT_SCENARIO_USAGE = 0x8L;
private static final long OPT_BIT_CAMPAIGN_USAGE = 0x10L;
private static final long OPT_BIT_SCENARIO_IN_CAMPAIGN_USAGE = 0x20L;
private long initBits = 0x1L;
private long optBits;
private @Nullable String id;
private @Nullable String name;
private @Nullable String description;
private @Nullable Instant lastUpdated;
private List tags = new ArrayList();
private List constants = new ArrayList();
private List> datatable = new ArrayList>();
private List scenarioUsage = new ArrayList();
private List campaignUsage = new ArrayList();
private Map> scenarioInCampaignUsage = new LinkedHashMap>();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code DataSetDto} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(DataSetDto instance) {
Objects.requireNonNull(instance, "instance");
Optional idOptional = instance.id();
if (idOptional.isPresent()) {
id(idOptional);
}
name(instance.name());
description(instance.description());
lastUpdated(instance.lastUpdated());
addAllTags(instance.tags());
addAllConstants(instance.constants());
addAllDatatable(instance.datatable());
addAllScenarioUsage(instance.scenarioUsage());
addAllCampaignUsage(instance.campaignUsage());
putAllScenarioInCampaignUsage(instance.scenarioInCampaignUsage());
return this;
}
/**
* Initializes the optional value {@link DataSetDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return this;
}
/**
* Initializes the optional value {@link DataSetDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final Builder id(Optional id) {
this.id = id.orElse(null);
return this;
}
/**
* Initializes the value for the {@link DataSetDto#name() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link DataSetDto#description() description} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link DataSetDto#description() description}.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
public final Builder description(String description) {
this.description = Objects.requireNonNull(description, "description");
return this;
}
/**
* Initializes the value for the {@link DataSetDto#lastUpdated() lastUpdated} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link DataSetDto#lastUpdated() lastUpdated}.
* @param lastUpdated The value for lastUpdated
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("lastUpdated")
public final Builder lastUpdated(Instant lastUpdated) {
this.lastUpdated = Objects.requireNonNull(lastUpdated, "lastUpdated");
return this;
}
/**
* Adds one element to {@link DataSetDto#tags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String element) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
optBits |= OPT_BIT_TAGS;
return this;
}
/**
* Adds elements to {@link DataSetDto#tags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String... elements) {
for (String element : elements) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
}
optBits |= OPT_BIT_TAGS;
return this;
}
/**
* Sets or replaces all elements for {@link DataSetDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
public final Builder tags(Iterable elements) {
this.tags.clear();
return addAllTags(elements);
}
/**
* Adds elements to {@link DataSetDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable elements) {
for (String element : elements) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
}
optBits |= OPT_BIT_TAGS;
return this;
}
/**
* Adds one element to {@link DataSetDto#constants() constants} list.
* @param element A constants element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addConstants(KeyValue element) {
this.constants.add(Objects.requireNonNull(element, "constants element"));
optBits |= OPT_BIT_CONSTANTS;
return this;
}
/**
* Adds elements to {@link DataSetDto#constants() constants} list.
* @param elements An array of constants elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addConstants(KeyValue... elements) {
for (KeyValue element : elements) {
this.constants.add(Objects.requireNonNull(element, "constants element"));
}
optBits |= OPT_BIT_CONSTANTS;
return this;
}
/**
* Sets or replaces all elements for {@link DataSetDto#constants() constants} list.
* @param elements An iterable of constants elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("uniqueValues")
public final Builder constants(Iterable extends KeyValue> elements) {
this.constants.clear();
return addAllConstants(elements);
}
/**
* Adds elements to {@link DataSetDto#constants() constants} list.
* @param elements An iterable of constants elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllConstants(Iterable extends KeyValue> elements) {
for (KeyValue element : elements) {
this.constants.add(Objects.requireNonNull(element, "constants element"));
}
optBits |= OPT_BIT_CONSTANTS;
return this;
}
/**
* Adds one element to {@link DataSetDto#datatable() datatable} list.
* @param element A datatable element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addDatatable(List element) {
this.datatable.add(Objects.requireNonNull(element, "datatable element"));
optBits |= OPT_BIT_DATATABLE;
return this;
}
/**
* Adds elements to {@link DataSetDto#datatable() datatable} list.
* @param elements An array of datatable elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@SafeVarargs @SuppressWarnings("varargs")
public final Builder addDatatable(List... elements) {
for (List element : elements) {
this.datatable.add(Objects.requireNonNull(element, "datatable element"));
}
optBits |= OPT_BIT_DATATABLE;
return this;
}
/**
* Sets or replaces all elements for {@link DataSetDto#datatable() datatable} list.
* @param elements An iterable of datatable elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("multipleValues")
public final Builder datatable(Iterable extends List> elements) {
this.datatable.clear();
return addAllDatatable(elements);
}
/**
* Adds elements to {@link DataSetDto#datatable() datatable} list.
* @param elements An iterable of datatable elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllDatatable(Iterable extends List> elements) {
for (List element : elements) {
this.datatable.add(Objects.requireNonNull(element, "datatable element"));
}
optBits |= OPT_BIT_DATATABLE;
return this;
}
/**
* Adds one element to {@link DataSetDto#scenarioUsage() scenarioUsage} list.
* @param element A scenarioUsage element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addScenarioUsage(String element) {
this.scenarioUsage.add(Objects.requireNonNull(element, "scenarioUsage element"));
optBits |= OPT_BIT_SCENARIO_USAGE;
return this;
}
/**
* Adds elements to {@link DataSetDto#scenarioUsage() scenarioUsage} list.
* @param elements An array of scenarioUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addScenarioUsage(String... elements) {
for (String element : elements) {
this.scenarioUsage.add(Objects.requireNonNull(element, "scenarioUsage element"));
}
optBits |= OPT_BIT_SCENARIO_USAGE;
return this;
}
/**
* Sets or replaces all elements for {@link DataSetDto#scenarioUsage() scenarioUsage} list.
* @param elements An iterable of scenarioUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("scenarioUsage")
public final Builder scenarioUsage(Iterable elements) {
this.scenarioUsage.clear();
return addAllScenarioUsage(elements);
}
/**
* Adds elements to {@link DataSetDto#scenarioUsage() scenarioUsage} list.
* @param elements An iterable of scenarioUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllScenarioUsage(Iterable elements) {
for (String element : elements) {
this.scenarioUsage.add(Objects.requireNonNull(element, "scenarioUsage element"));
}
optBits |= OPT_BIT_SCENARIO_USAGE;
return this;
}
/**
* Adds one element to {@link DataSetDto#campaignUsage() campaignUsage} list.
* @param element A campaignUsage element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addCampaignUsage(String element) {
this.campaignUsage.add(Objects.requireNonNull(element, "campaignUsage element"));
optBits |= OPT_BIT_CAMPAIGN_USAGE;
return this;
}
/**
* Adds elements to {@link DataSetDto#campaignUsage() campaignUsage} list.
* @param elements An array of campaignUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addCampaignUsage(String... elements) {
for (String element : elements) {
this.campaignUsage.add(Objects.requireNonNull(element, "campaignUsage element"));
}
optBits |= OPT_BIT_CAMPAIGN_USAGE;
return this;
}
/**
* Sets or replaces all elements for {@link DataSetDto#campaignUsage() campaignUsage} list.
* @param elements An iterable of campaignUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("campaignUsage")
public final Builder campaignUsage(Iterable elements) {
this.campaignUsage.clear();
return addAllCampaignUsage(elements);
}
/**
* Adds elements to {@link DataSetDto#campaignUsage() campaignUsage} list.
* @param elements An iterable of campaignUsage elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllCampaignUsage(Iterable elements) {
for (String element : elements) {
this.campaignUsage.add(Objects.requireNonNull(element, "campaignUsage element"));
}
optBits |= OPT_BIT_CAMPAIGN_USAGE;
return this;
}
/**
* Put one entry to the {@link DataSetDto#scenarioInCampaignUsage() scenarioInCampaignUsage} map.
* @param key The key in the scenarioInCampaignUsage map
* @param value The associated value in the scenarioInCampaignUsage map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putScenarioInCampaignUsage(String key, Set value) {
this.scenarioInCampaignUsage.put(
Objects.requireNonNull(key, "scenarioInCampaignUsage key"),
Objects.requireNonNull(value, value == null ? "scenarioInCampaignUsage value for key: " + key : null));
optBits |= OPT_BIT_SCENARIO_IN_CAMPAIGN_USAGE;
return this;
}
/**
* Put one entry to the {@link DataSetDto#scenarioInCampaignUsage() scenarioInCampaignUsage} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putScenarioInCampaignUsage(Map.Entry> entry) {
String k = entry.getKey();
Set v = entry.getValue();
this.scenarioInCampaignUsage.put(
Objects.requireNonNull(k, "scenarioInCampaignUsage key"),
Objects.requireNonNull(v, v == null ? "scenarioInCampaignUsage value for key: " + k : null));
optBits |= OPT_BIT_SCENARIO_IN_CAMPAIGN_USAGE;
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link DataSetDto#scenarioInCampaignUsage() scenarioInCampaignUsage} map. Nulls are not permitted
* @param entries The entries that will be added to the scenarioInCampaignUsage map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("scenarioInCampaignUsage")
public final Builder scenarioInCampaignUsage(Map> entries) {
this.scenarioInCampaignUsage.clear();
optBits |= OPT_BIT_SCENARIO_IN_CAMPAIGN_USAGE;
return putAllScenarioInCampaignUsage(entries);
}
/**
* Put all mappings from the specified map as entries to {@link DataSetDto#scenarioInCampaignUsage() scenarioInCampaignUsage} map. Nulls are not permitted
* @param entries The entries that will be added to the scenarioInCampaignUsage map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllScenarioInCampaignUsage(Map> entries) {
for (Map.Entry> e : entries.entrySet()) {
String k = e.getKey();
Set v = e.getValue();
this.scenarioInCampaignUsage.put(
Objects.requireNonNull(k, "scenarioInCampaignUsage key"),
Objects.requireNonNull(v, v == null ? "scenarioInCampaignUsage value for key: " + k : null));
}
optBits |= OPT_BIT_SCENARIO_IN_CAMPAIGN_USAGE;
return this;
}
/**
* Builds a new {@link ImmutableDataSetDto ImmutableDataSetDto}.
* @return An immutable instance of DataSetDto
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableDataSetDto build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableDataSetDto(this);
}
private boolean tagsIsSet() {
return (optBits & OPT_BIT_TAGS) != 0;
}
private boolean constantsIsSet() {
return (optBits & OPT_BIT_CONSTANTS) != 0;
}
private boolean datatableIsSet() {
return (optBits & OPT_BIT_DATATABLE) != 0;
}
private boolean scenarioUsageIsSet() {
return (optBits & OPT_BIT_SCENARIO_USAGE) != 0;
}
private boolean campaignUsageIsSet() {
return (optBits & OPT_BIT_CAMPAIGN_USAGE) != 0;
}
private boolean scenarioInCampaignUsageIsSet() {
return (optBits & OPT_BIT_SCENARIO_IN_CAMPAIGN_USAGE) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
return "Cannot build DataSetDto, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
private static Map createUnmodifiableMap(boolean checkNulls, boolean skipNulls, Map extends K, ? extends V> map) {
switch (map.size()) {
case 0: return Collections.emptyMap();
case 1: {
Map.Entry extends K, ? extends V> e = map.entrySet().iterator().next();
K k = e.getKey();
V v = e.getValue();
if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
if (skipNulls && (k == null || v == null)) {
return Collections.emptyMap();
}
return Collections.singletonMap(k, v);
}
default: {
Map linkedMap = new LinkedHashMap<>(map.size() * 4 / 3 + 1);
if (skipNulls || checkNulls) {
for (Map.Entry extends K, ? extends V> e : map.entrySet()) {
K k = e.getKey();
V v = e.getValue();
if (skipNulls) {
if (k == null || v == null) continue;
} else if (checkNulls) {
Objects.requireNonNull(k, "key");
Objects.requireNonNull(v, v == null ? "value for key: " + k : null);
}
linkedMap.put(k, v);
}
} else {
linkedMap.putAll(map);
}
return Collections.unmodifiableMap(linkedMap);
}
}
}
}