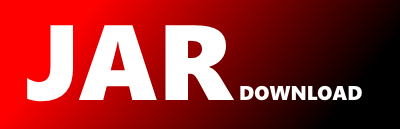
annotations.com.chutneytesting.execution.api.ScenarioExecutionReportMapperImpl Maven / Gradle / Ivy
package com.chutneytesting.execution.api;
import com.chutneytesting.server.core.domain.execution.report.ScenarioExecutionReport;
import com.chutneytesting.server.core.domain.execution.report.ServerReportStatus;
import com.chutneytesting.server.core.domain.execution.report.StepExecutionReportCore;
import java.time.Instant;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.annotation.processing.Generated;
import org.springframework.stereotype.Component;
@Generated(
value = "org.mapstruct.ap.MappingProcessor",
date = "2024-11-25T22:31:19+0000",
comments = "version: 1.6.2, compiler: javac, environment: Java 17.0.13 (Eclipse Adoptium)"
)
@Component
public class ScenarioExecutionReportMapperImpl implements ScenarioExecutionReportMapper {
@Override
public ScenarioExecutionReportDto toDto(ScenarioExecutionReport source) {
if ( source == null ) {
return null;
}
long executionId = 0L;
String scenarioName = null;
String environment = null;
String user = null;
Set tags = null;
Map contextVariables = null;
StepExecutionReportCoreDto report = null;
executionId = source.executionId;
scenarioName = source.scenarioName;
environment = source.environment;
user = source.user;
Set set = source.tags;
if ( set != null ) {
tags = new LinkedHashSet( set );
}
Map map = source.contextVariables;
if ( map != null ) {
contextVariables = new LinkedHashMap( map );
}
report = toStepDto( source.report );
ScenarioExecutionReportDto scenarioExecutionReportDto = new ScenarioExecutionReportDto( executionId, scenarioName, environment, user, tags, contextVariables, report );
return scenarioExecutionReportDto;
}
@Override
public StepExecutionReportCoreDto toStepDto(StepExecutionReportCore domain) {
if ( domain == null ) {
return null;
}
String name = null;
Long duration = null;
Instant startDate = null;
ServerReportStatus status = null;
List information = null;
List errors = null;
List steps = null;
String type = null;
String targetName = null;
String targetUrl = null;
String strategy = null;
Map evaluatedInputs = null;
Map stepOutputs = null;
name = domain.name;
duration = domain.duration;
startDate = domain.startDate;
status = domain.getStatus();
List list = domain.information;
if ( list != null ) {
information = new ArrayList( list );
}
List list1 = domain.errors;
if ( list1 != null ) {
errors = new ArrayList( list1 );
}
steps = stepExecutionReportCoreListToStepExecutionReportCoreDtoList( domain.steps );
type = domain.type;
targetName = domain.targetName;
targetUrl = domain.targetUrl;
strategy = domain.strategy;
Map map = domain.evaluatedInputs;
if ( map != null ) {
evaluatedInputs = new LinkedHashMap( map );
}
Map map1 = domain.stepOutputs;
if ( map1 != null ) {
stepOutputs = new LinkedHashMap( map1 );
}
StepExecutionReportCoreDto stepExecutionReportCoreDto = new StepExecutionReportCoreDto( name, duration, startDate, status, information, errors, steps, type, targetName, targetUrl, strategy, evaluatedInputs, stepOutputs );
return stepExecutionReportCoreDto;
}
protected List stepExecutionReportCoreListToStepExecutionReportCoreDtoList(List list) {
if ( list == null ) {
return null;
}
List list1 = new ArrayList( list.size() );
for ( StepExecutionReportCore stepExecutionReportCore : list ) {
list1.add( toStepDto( stepExecutionReportCore ) );
}
return list1;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy