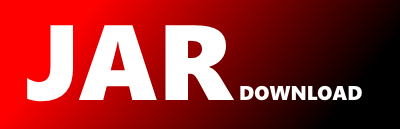
annotations.com.chutneytesting.scenario.api.raw.dto.ImmutableGwtTestCaseMetadataDto Maven / Gradle / Ivy
package com.chutneytesting.scenario.api.raw.dto;
import com.chutneytesting.execution.api.ExecutionSummaryDto;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.time.Instant;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link GwtTestCaseMetadataDto}.
*
* Use the builder to create immutable instances:
* {@code ImmutableGwtTestCaseMetadataDto.builder()}.
*/
@Generated(from = "GwtTestCaseMetadataDto", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableGwtTestCaseMetadataDto
implements GwtTestCaseMetadataDto {
private final @Nullable String id;
private final String title;
private final @Nullable String description;
private final @Nullable String repositorySource;
private final ImmutableList tags;
private final ImmutableList executions;
private final Instant creationDate;
private final Instant updateDate;
private ImmutableGwtTestCaseMetadataDto(ImmutableGwtTestCaseMetadataDto.Builder builder) {
this.id = builder.id;
this.title = builder.title;
this.description = builder.description;
this.repositorySource = builder.repositorySource;
this.tags = builder.tags.build();
this.executions = builder.executions.build();
if (builder.creationDate != null) {
initShim.creationDate(builder.creationDate);
}
if (builder.updateDate != null) {
initShim.updateDate(builder.updateDate);
}
this.creationDate = initShim.creationDate();
this.updateDate = initShim.updateDate();
this.initShim = null;
}
private ImmutableGwtTestCaseMetadataDto(
@Nullable String id,
String title,
@Nullable String description,
@Nullable String repositorySource,
ImmutableList tags,
ImmutableList executions,
Instant creationDate,
Instant updateDate) {
this.id = id;
this.title = title;
this.description = description;
this.repositorySource = repositorySource;
this.tags = tags;
this.executions = executions;
this.creationDate = creationDate;
this.updateDate = updateDate;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "GwtTestCaseMetadataDto", generator = "Immutables")
private final class InitShim {
private byte creationDateBuildStage = STAGE_UNINITIALIZED;
private Instant creationDate;
Instant creationDate() {
if (creationDateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (creationDateBuildStage == STAGE_UNINITIALIZED) {
creationDateBuildStage = STAGE_INITIALIZING;
this.creationDate = Objects.requireNonNull(creationDateInitialize(), "creationDate");
creationDateBuildStage = STAGE_INITIALIZED;
}
return this.creationDate;
}
void creationDate(Instant creationDate) {
this.creationDate = creationDate;
creationDateBuildStage = STAGE_INITIALIZED;
}
private byte updateDateBuildStage = STAGE_UNINITIALIZED;
private Instant updateDate;
Instant updateDate() {
if (updateDateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (updateDateBuildStage == STAGE_UNINITIALIZED) {
updateDateBuildStage = STAGE_INITIALIZING;
this.updateDate = Objects.requireNonNull(updateDateInitialize(), "updateDate");
updateDateBuildStage = STAGE_INITIALIZED;
}
return this.updateDate;
}
void updateDate(Instant updateDate) {
this.updateDate = updateDate;
updateDateBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (creationDateBuildStage == STAGE_INITIALIZING) attributes.add("creationDate");
if (updateDateBuildStage == STAGE_INITIALIZING) attributes.add("updateDate");
return "Cannot build GwtTestCaseMetadataDto, attribute initializers form cycle " + attributes;
}
}
private Instant creationDateInitialize() {
return GwtTestCaseMetadataDto.super.creationDate();
}
private Instant updateDateInitialize() {
return GwtTestCaseMetadataDto.super.updateDate();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public Optional id() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty("title")
@Override
public String title() {
return title;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public Optional description() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code repositorySource} attribute
*/
@JsonProperty("repositorySource")
@Override
public Optional repositorySource() {
return Optional.ofNullable(repositorySource);
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public ImmutableList tags() {
return tags;
}
/**
* @return The value of the {@code executions} attribute
*/
@JsonProperty("executions")
@Override
public ImmutableList executions() {
return executions;
}
/**
* @return The value of the {@code creationDate} attribute
*/
@JsonProperty("creationDate")
@Override
public Instant creationDate() {
InitShim shim = this.initShim;
return shim != null
? shim.creationDate()
: this.creationDate;
}
/**
* @return The value of the {@code updateDate} attribute
*/
@JsonProperty("updateDate")
@Override
public Instant updateDate() {
InitShim shim = this.initShim;
return shim != null
? shim.updateDate()
: this.updateDate;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseMetadataDto#id() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return new ImmutableGwtTestCaseMetadataDto(
newValue,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseMetadataDto#id() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return new ImmutableGwtTestCaseMetadataDto(
value,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseMetadataDto#title() title} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for title
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withTitle(String value) {
String newValue = Objects.requireNonNull(value, "title");
if (this.title.equals(newValue)) return this;
return new ImmutableGwtTestCaseMetadataDto(
this.id,
newValue,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseMetadataDto#description() description} attribute.
* @param value The value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withDescription(String value) {
String newValue = Objects.requireNonNull(value, "description");
if (Objects.equals(this.description, newValue)) return this;
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
newValue,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseMetadataDto#description() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
value,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseMetadataDto#repositorySource() repositorySource} attribute.
* @param value The value for repositorySource
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withRepositorySource(String value) {
String newValue = Objects.requireNonNull(value, "repositorySource");
if (Objects.equals(this.repositorySource, newValue)) return this;
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
newValue,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseMetadataDto#repositorySource() repositorySource} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for repositorySource
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withRepositorySource(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.repositorySource, value)) return this;
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
value,
this.tags,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseMetadataDto#tags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withTags(String... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
this.repositorySource,
newValue,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseMetadataDto#tags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withTags(Iterable elements) {
if (this.tags == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
this.repositorySource,
newValue,
this.executions,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseMetadataDto#executions() executions}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withExecutions(ExecutionSummaryDto... elements) {
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
newValue,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseMetadataDto#executions() executions}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of executions elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withExecutions(Iterable extends ExecutionSummaryDto> elements) {
if (this.executions == elements) return this;
ImmutableList newValue = ImmutableList.copyOf(elements);
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
newValue,
this.creationDate,
this.updateDate);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseMetadataDto#creationDate() creationDate} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for creationDate
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withCreationDate(Instant value) {
if (this.creationDate == value) return this;
Instant newValue = Objects.requireNonNull(value, "creationDate");
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
newValue,
this.updateDate);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseMetadataDto#updateDate() updateDate} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for updateDate
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseMetadataDto withUpdateDate(Instant value) {
if (this.updateDate == value) return this;
Instant newValue = Objects.requireNonNull(value, "updateDate");
return new ImmutableGwtTestCaseMetadataDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableGwtTestCaseMetadataDto} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableGwtTestCaseMetadataDto
&& equalTo(0, (ImmutableGwtTestCaseMetadataDto) another);
}
private boolean equalTo(int synthetic, ImmutableGwtTestCaseMetadataDto another) {
return Objects.equals(id, another.id)
&& title.equals(another.title)
&& Objects.equals(description, another.description)
&& Objects.equals(repositorySource, another.repositorySource)
&& tags.equals(another.tags)
&& executions.equals(another.executions)
&& creationDate.equals(another.creationDate)
&& updateDate.equals(another.updateDate);
}
/**
* Computes a hash code from attributes: {@code id}, {@code title}, {@code description}, {@code repositorySource}, {@code tags}, {@code executions}, {@code creationDate}, {@code updateDate}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + title.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + Objects.hashCode(repositorySource);
h += (h << 5) + tags.hashCode();
h += (h << 5) + executions.hashCode();
h += (h << 5) + creationDate.hashCode();
h += (h << 5) + updateDate.hashCode();
return h;
}
/**
* Prints the immutable value {@code GwtTestCaseMetadataDto} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("GwtTestCaseMetadataDto")
.omitNullValues()
.add("id", id)
.add("title", title)
.add("description", description)
.add("repositorySource", repositorySource)
.add("tags", tags)
.add("executions", executions)
.add("creationDate", creationDate)
.add("updateDate", updateDate)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "GwtTestCaseMetadataDto", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements GwtTestCaseMetadataDto {
@Nullable Optional id = Optional.empty();
@Nullable String title;
@Nullable Optional description = Optional.empty();
@Nullable Optional repositorySource = Optional.empty();
@Nullable List tags = ImmutableList.of();
@Nullable List executions = ImmutableList.of();
@Nullable Instant creationDate;
@Nullable Instant updateDate;
@JsonProperty("id")
public void setId(Optional id) {
this.id = id;
}
@JsonProperty("title")
public void setTitle(String title) {
this.title = title;
}
@JsonProperty("description")
public void setDescription(Optional description) {
this.description = description;
}
@JsonProperty("repositorySource")
public void setRepositorySource(Optional repositorySource) {
this.repositorySource = repositorySource;
}
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
}
@JsonProperty("executions")
public void setExecutions(List executions) {
this.executions = executions;
}
@JsonProperty("creationDate")
public void setCreationDate(Instant creationDate) {
this.creationDate = creationDate;
}
@JsonProperty("updateDate")
public void setUpdateDate(Instant updateDate) {
this.updateDate = updateDate;
}
@Override
public Optional id() { throw new UnsupportedOperationException(); }
@Override
public String title() { throw new UnsupportedOperationException(); }
@Override
public Optional description() { throw new UnsupportedOperationException(); }
@Override
public Optional repositorySource() { throw new UnsupportedOperationException(); }
@Override
public List tags() { throw new UnsupportedOperationException(); }
@Override
public List executions() { throw new UnsupportedOperationException(); }
@Override
public Instant creationDate() { throw new UnsupportedOperationException(); }
@Override
public Instant updateDate() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableGwtTestCaseMetadataDto fromJson(Json json) {
ImmutableGwtTestCaseMetadataDto.Builder builder = ImmutableGwtTestCaseMetadataDto.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.title != null) {
builder.title(json.title);
}
if (json.description != null) {
builder.description(json.description);
}
if (json.repositorySource != null) {
builder.repositorySource(json.repositorySource);
}
if (json.tags != null) {
builder.addAllTags(json.tags);
}
if (json.executions != null) {
builder.addAllExecutions(json.executions);
}
if (json.creationDate != null) {
builder.creationDate(json.creationDate);
}
if (json.updateDate != null) {
builder.updateDate(json.updateDate);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link GwtTestCaseMetadataDto} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable GwtTestCaseMetadataDto instance
*/
public static ImmutableGwtTestCaseMetadataDto copyOf(GwtTestCaseMetadataDto instance) {
if (instance instanceof ImmutableGwtTestCaseMetadataDto) {
return (ImmutableGwtTestCaseMetadataDto) instance;
}
return ImmutableGwtTestCaseMetadataDto.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableGwtTestCaseMetadataDto ImmutableGwtTestCaseMetadataDto}.
*
* ImmutableGwtTestCaseMetadataDto.builder()
* .id(String) // optional {@link GwtTestCaseMetadataDto#id() id}
* .title(String) // required {@link GwtTestCaseMetadataDto#title() title}
* .description(String) // optional {@link GwtTestCaseMetadataDto#description() description}
* .repositorySource(String) // optional {@link GwtTestCaseMetadataDto#repositorySource() repositorySource}
* .addTags|addAllTags(String) // {@link GwtTestCaseMetadataDto#tags() tags} elements
* .addExecutions|addAllExecutions(com.chutneytesting.execution.api.ExecutionSummaryDto) // {@link GwtTestCaseMetadataDto#executions() executions} elements
* .creationDate(java.time.Instant) // optional {@link GwtTestCaseMetadataDto#creationDate() creationDate}
* .updateDate(java.time.Instant) // optional {@link GwtTestCaseMetadataDto#updateDate() updateDate}
* .build();
*
* @return A new ImmutableGwtTestCaseMetadataDto builder
*/
public static ImmutableGwtTestCaseMetadataDto.Builder builder() {
return new ImmutableGwtTestCaseMetadataDto.Builder();
}
/**
* Builds instances of type {@link ImmutableGwtTestCaseMetadataDto ImmutableGwtTestCaseMetadataDto}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "GwtTestCaseMetadataDto", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TITLE = 0x1L;
private long initBits = 0x1L;
private @Nullable String id;
private @Nullable String title;
private @Nullable String description;
private @Nullable String repositorySource;
private ImmutableList.Builder tags = ImmutableList.builder();
private ImmutableList.Builder executions = ImmutableList.builder();
private @Nullable Instant creationDate;
private @Nullable Instant updateDate;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code GwtTestCaseMetadataDto} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(GwtTestCaseMetadataDto instance) {
Objects.requireNonNull(instance, "instance");
Optional idOptional = instance.id();
if (idOptional.isPresent()) {
id(idOptional);
}
title(instance.title());
Optional descriptionOptional = instance.description();
if (descriptionOptional.isPresent()) {
description(descriptionOptional);
}
Optional repositorySourceOptional = instance.repositorySource();
if (repositorySourceOptional.isPresent()) {
repositorySource(repositorySourceOptional);
}
addAllTags(instance.tags());
addAllExecutions(instance.executions());
creationDate(instance.creationDate());
updateDate(instance.updateDate());
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseMetadataDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseMetadataDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final Builder id(Optional id) {
this.id = id.orElse(null);
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseMetadataDto#title() title} attribute.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("title")
public final Builder title(String title) {
this.title = Objects.requireNonNull(title, "title");
initBits &= ~INIT_BIT_TITLE;
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseMetadataDto#description() description} to description.
* @param description The value for description
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder description(String description) {
this.description = Objects.requireNonNull(description, "description");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseMetadataDto#description() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
public final Builder description(Optional description) {
this.description = description.orElse(null);
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseMetadataDto#repositorySource() repositorySource} to repositorySource.
* @param repositorySource The value for repositorySource
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder repositorySource(String repositorySource) {
this.repositorySource = Objects.requireNonNull(repositorySource, "repositorySource");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseMetadataDto#repositorySource() repositorySource} to repositorySource.
* @param repositorySource The value for repositorySource
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("repositorySource")
public final Builder repositorySource(Optional repositorySource) {
this.repositorySource = repositorySource.orElse(null);
return this;
}
/**
* Adds one element to {@link GwtTestCaseMetadataDto#tags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String element) {
this.tags.add(element);
return this;
}
/**
* Adds elements to {@link GwtTestCaseMetadataDto#tags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String... elements) {
this.tags.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link GwtTestCaseMetadataDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
public final Builder tags(Iterable elements) {
this.tags = ImmutableList.builder();
return addAllTags(elements);
}
/**
* Adds elements to {@link GwtTestCaseMetadataDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable elements) {
this.tags.addAll(elements);
return this;
}
/**
* Adds one element to {@link GwtTestCaseMetadataDto#executions() executions} list.
* @param element A executions element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addExecutions(ExecutionSummaryDto element) {
this.executions.add(element);
return this;
}
/**
* Adds elements to {@link GwtTestCaseMetadataDto#executions() executions} list.
* @param elements An array of executions elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addExecutions(ExecutionSummaryDto... elements) {
this.executions.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link GwtTestCaseMetadataDto#executions() executions} list.
* @param elements An iterable of executions elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("executions")
public final Builder executions(Iterable extends ExecutionSummaryDto> elements) {
this.executions = ImmutableList.builder();
return addAllExecutions(elements);
}
/**
* Adds elements to {@link GwtTestCaseMetadataDto#executions() executions} list.
* @param elements An iterable of executions elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllExecutions(Iterable extends ExecutionSummaryDto> elements) {
this.executions.addAll(elements);
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseMetadataDto#creationDate() creationDate} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link GwtTestCaseMetadataDto#creationDate() creationDate}.
* @param creationDate The value for creationDate
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("creationDate")
public final Builder creationDate(Instant creationDate) {
this.creationDate = Objects.requireNonNull(creationDate, "creationDate");
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseMetadataDto#updateDate() updateDate} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GwtTestCaseMetadataDto#updateDate() updateDate}.
* @param updateDate The value for updateDate
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("updateDate")
public final Builder updateDate(Instant updateDate) {
this.updateDate = Objects.requireNonNull(updateDate, "updateDate");
return this;
}
/**
* Builds a new {@link ImmutableGwtTestCaseMetadataDto ImmutableGwtTestCaseMetadataDto}.
* @return An immutable instance of GwtTestCaseMetadataDto
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableGwtTestCaseMetadataDto build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableGwtTestCaseMetadataDto(this);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TITLE) != 0) attributes.add("title");
return "Cannot build GwtTestCaseMetadataDto, some of required attributes are not set " + attributes;
}
}
}