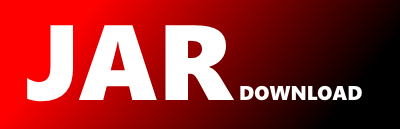
com.chutneytesting.execution.api.ImmutableExecutionSummaryDto Maven / Gradle / Ivy
package com.chutneytesting.execution.api;
import com.chutneytesting.server.core.domain.dataset.DataSet;
import com.chutneytesting.server.core.domain.execution.history.ExecutionHistory;
import com.chutneytesting.server.core.domain.execution.report.ServerReportStatus;
import com.chutneytesting.server.core.domain.scenario.campaign.CampaignExecution;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.Longs;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.time.LocalDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.Set;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link ExecutionSummaryDto}.
*
* Use the builder to create immutable instances:
* {@code ImmutableExecutionSummaryDto.builder()}.
*/
@Generated(from = "ExecutionSummaryDto", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableExecutionSummaryDto
implements ExecutionSummaryDto {
private final LocalDateTime time;
private final long duration;
private final ServerReportStatus status;
private final @Nullable String info;
private final @Nullable String error;
private final String testCaseTitle;
private final String environment;
private final @Nullable DataSet dataset;
private final String user;
private final @Nullable CampaignExecution campaignReport;
private final @Nullable Set tags;
private final Long executionId;
private final String scenarioId;
private ImmutableExecutionSummaryDto(
LocalDateTime time,
long duration,
ServerReportStatus status,
@Nullable String info,
@Nullable String error,
String testCaseTitle,
String environment,
@Nullable DataSet dataset,
String user,
@Nullable CampaignExecution campaignReport,
@Nullable Set tags,
Long executionId,
String scenarioId) {
this.time = time;
this.duration = duration;
this.status = status;
this.info = info;
this.error = error;
this.testCaseTitle = testCaseTitle;
this.environment = environment;
this.dataset = dataset;
this.user = user;
this.campaignReport = campaignReport;
this.tags = tags;
this.executionId = executionId;
this.scenarioId = scenarioId;
}
/**
* @return The value of the {@code time} attribute
*/
@JsonProperty("time")
@Override
public LocalDateTime time() {
return time;
}
/**
* @return The value of the {@code duration} attribute
*/
@JsonProperty("duration")
@Override
public long duration() {
return duration;
}
/**
* @return The value of the {@code status} attribute
*/
@JsonProperty("status")
@Override
public ServerReportStatus status() {
return status;
}
/**
* @return The value of the {@code info} attribute
*/
@JsonProperty("info")
@Override
public Optional info() {
return Optional.ofNullable(info);
}
/**
* @return The value of the {@code error} attribute
*/
@JsonProperty("error")
@Override
public Optional error() {
return Optional.ofNullable(error);
}
/**
* @return The value of the {@code testCaseTitle} attribute
*/
@JsonProperty("testCaseTitle")
@Override
public String testCaseTitle() {
return testCaseTitle;
}
/**
* @return The value of the {@code environment} attribute
*/
@JsonProperty("environment")
@Override
public String environment() {
return environment;
}
/**
* @return The value of the {@code dataset} attribute
*/
@JsonProperty("dataset")
@Override
public Optional dataset() {
return Optional.ofNullable(dataset);
}
/**
* @return The value of the {@code user} attribute
*/
@JsonProperty("user")
@Override
public String user() {
return user;
}
/**
* @return The value of the {@code campaignReport} attribute
*/
@JsonProperty("campaignReport")
@Override
public Optional campaignReport() {
return Optional.ofNullable(campaignReport);
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public Optional> tags() {
return Optional.ofNullable(tags);
}
/**
* @return The value of the {@code executionId} attribute
*/
@JsonProperty("executionId")
@Override
public Long executionId() {
return executionId;
}
/**
* @return The value of the {@code scenarioId} attribute
*/
@JsonProperty("scenarioId")
@Override
public String scenarioId() {
return scenarioId;
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#time() time} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for time
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withTime(LocalDateTime value) {
if (this.time == value) return this;
LocalDateTime newValue = Objects.requireNonNull(value, "time");
return new ImmutableExecutionSummaryDto(
newValue,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#duration() duration} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for duration
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withDuration(long value) {
if (this.duration == value) return this;
return new ImmutableExecutionSummaryDto(
this.time,
value,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#status() status} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for status
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withStatus(ServerReportStatus value) {
ServerReportStatus newValue = Objects.requireNonNull(value, "status");
if (this.status == newValue) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
newValue,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ExecutionSummaryDto#info() info} attribute.
* @param value The value for info
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withInfo(String value) {
String newValue = Objects.requireNonNull(value, "info");
if (Objects.equals(this.info, newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
newValue,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ExecutionSummaryDto#info() info} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for info
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withInfo(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.info, value)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
value,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ExecutionSummaryDto#error() error} attribute.
* @param value The value for error
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withError(String value) {
String newValue = Objects.requireNonNull(value, "error");
if (Objects.equals(this.error, newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
newValue,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ExecutionSummaryDto#error() error} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for error
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withError(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.error, value)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
value,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#testCaseTitle() testCaseTitle} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for testCaseTitle
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withTestCaseTitle(String value) {
String newValue = Objects.requireNonNull(value, "testCaseTitle");
if (this.testCaseTitle.equals(newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
newValue,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#environment() environment} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for environment
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withEnvironment(String value) {
String newValue = Objects.requireNonNull(value, "environment");
if (this.environment.equals(newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
newValue,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ExecutionSummaryDto#dataset() dataset} attribute.
* @param value The value for dataset
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withDataset(DataSet value) {
DataSet newValue = Objects.requireNonNull(value, "dataset");
if (this.dataset == newValue) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
newValue,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ExecutionSummaryDto#dataset() dataset} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for dataset
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableExecutionSummaryDto withDataset(Optional extends DataSet> optional) {
@Nullable DataSet value = optional.orElse(null);
if (this.dataset == value) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
value,
this.user,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#user() user} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for user
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withUser(String value) {
String newValue = Objects.requireNonNull(value, "user");
if (this.user.equals(newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
newValue,
this.campaignReport,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ExecutionSummaryDto#campaignReport() campaignReport} attribute.
* @param value The value for campaignReport
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withCampaignReport(CampaignExecution value) {
CampaignExecution newValue = Objects.requireNonNull(value, "campaignReport");
if (this.campaignReport == newValue) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
newValue,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ExecutionSummaryDto#campaignReport() campaignReport} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for campaignReport
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableExecutionSummaryDto withCampaignReport(Optional extends CampaignExecution> optional) {
@Nullable CampaignExecution value = optional.orElse(null);
if (this.campaignReport == value) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
value,
this.tags,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link ExecutionSummaryDto#tags() tags} attribute.
* @param value The value for tags
* @return A modified copy of {@code this} object
*/
public final ImmutableExecutionSummaryDto withTags(Set value) {
Set newValue = Objects.requireNonNull(value, "tags");
if (this.tags == newValue) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
newValue,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting an optional value for the {@link ExecutionSummaryDto#tags() tags} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for tags
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableExecutionSummaryDto withTags(Optional extends Set> optional) {
@Nullable Set value = optional.orElse(null);
if (this.tags == value) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
value,
this.executionId,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#executionId() executionId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for executionId
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withExecutionId(Long value) {
Long newValue = Objects.requireNonNull(value, "executionId");
if (this.executionId.equals(newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
newValue,
this.scenarioId);
}
/**
* Copy the current immutable object by setting a value for the {@link ExecutionSummaryDto#scenarioId() scenarioId} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for scenarioId
* @return A modified copy of the {@code this} object
*/
public final ImmutableExecutionSummaryDto withScenarioId(String value) {
String newValue = Objects.requireNonNull(value, "scenarioId");
if (this.scenarioId.equals(newValue)) return this;
return new ImmutableExecutionSummaryDto(
this.time,
this.duration,
this.status,
this.info,
this.error,
this.testCaseTitle,
this.environment,
this.dataset,
this.user,
this.campaignReport,
this.tags,
this.executionId,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableExecutionSummaryDto} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableExecutionSummaryDto
&& equalTo(0, (ImmutableExecutionSummaryDto) another);
}
private boolean equalTo(int synthetic, ImmutableExecutionSummaryDto another) {
return time.equals(another.time)
&& duration == another.duration
&& status.equals(another.status)
&& Objects.equals(info, another.info)
&& Objects.equals(error, another.error)
&& testCaseTitle.equals(another.testCaseTitle)
&& environment.equals(another.environment)
&& Objects.equals(dataset, another.dataset)
&& user.equals(another.user)
&& Objects.equals(campaignReport, another.campaignReport)
&& Objects.equals(tags, another.tags)
&& executionId.equals(another.executionId)
&& scenarioId.equals(another.scenarioId);
}
/**
* Computes a hash code from attributes: {@code time}, {@code duration}, {@code status}, {@code info}, {@code error}, {@code testCaseTitle}, {@code environment}, {@code dataset}, {@code user}, {@code campaignReport}, {@code tags}, {@code executionId}, {@code scenarioId}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + time.hashCode();
h += (h << 5) + Longs.hashCode(duration);
h += (h << 5) + status.hashCode();
h += (h << 5) + Objects.hashCode(info);
h += (h << 5) + Objects.hashCode(error);
h += (h << 5) + testCaseTitle.hashCode();
h += (h << 5) + environment.hashCode();
h += (h << 5) + Objects.hashCode(dataset);
h += (h << 5) + user.hashCode();
h += (h << 5) + Objects.hashCode(campaignReport);
h += (h << 5) + Objects.hashCode(tags);
h += (h << 5) + executionId.hashCode();
h += (h << 5) + scenarioId.hashCode();
return h;
}
/**
* Prints the immutable value {@code ExecutionSummaryDto} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("ExecutionSummaryDto")
.omitNullValues()
.add("time", time)
.add("duration", duration)
.add("status", status)
.add("info", info)
.add("error", error)
.add("testCaseTitle", testCaseTitle)
.add("environment", environment)
.add("dataset", dataset)
.add("user", user)
.add("campaignReport", campaignReport)
.add("tags", tags)
.add("executionId", executionId)
.add("scenarioId", scenarioId)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "ExecutionSummaryDto", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements ExecutionSummaryDto {
@Nullable LocalDateTime time;
long duration;
boolean durationIsSet;
@Nullable ServerReportStatus status;
@Nullable Optional info = Optional.empty();
@Nullable Optional error = Optional.empty();
@Nullable String testCaseTitle;
@Nullable String environment;
@Nullable Optional dataset = Optional.empty();
@Nullable String user;
@Nullable Optional campaignReport = Optional.empty();
@Nullable Optional> tags = Optional.empty();
@Nullable Long executionId;
@Nullable String scenarioId;
@JsonProperty("time")
public void setTime(LocalDateTime time) {
this.time = time;
}
@JsonProperty("duration")
public void setDuration(long duration) {
this.duration = duration;
this.durationIsSet = true;
}
@JsonProperty("status")
public void setStatus(ServerReportStatus status) {
this.status = status;
}
@JsonProperty("info")
public void setInfo(Optional info) {
this.info = info;
}
@JsonProperty("error")
public void setError(Optional error) {
this.error = error;
}
@JsonProperty("testCaseTitle")
public void setTestCaseTitle(String testCaseTitle) {
this.testCaseTitle = testCaseTitle;
}
@JsonProperty("environment")
public void setEnvironment(String environment) {
this.environment = environment;
}
@JsonProperty("dataset")
public void setDataset(Optional dataset) {
this.dataset = dataset;
}
@JsonProperty("user")
public void setUser(String user) {
this.user = user;
}
@JsonProperty("campaignReport")
public void setCampaignReport(Optional campaignReport) {
this.campaignReport = campaignReport;
}
@JsonProperty("tags")
public void setTags(Optional> tags) {
this.tags = tags;
}
@JsonProperty("executionId")
public void setExecutionId(Long executionId) {
this.executionId = executionId;
}
@JsonProperty("scenarioId")
public void setScenarioId(String scenarioId) {
this.scenarioId = scenarioId;
}
@Override
public LocalDateTime time() { throw new UnsupportedOperationException(); }
@Override
public long duration() { throw new UnsupportedOperationException(); }
@Override
public ServerReportStatus status() { throw new UnsupportedOperationException(); }
@Override
public Optional info() { throw new UnsupportedOperationException(); }
@Override
public Optional error() { throw new UnsupportedOperationException(); }
@Override
public String testCaseTitle() { throw new UnsupportedOperationException(); }
@Override
public String environment() { throw new UnsupportedOperationException(); }
@Override
public Optional dataset() { throw new UnsupportedOperationException(); }
@Override
public String user() { throw new UnsupportedOperationException(); }
@Override
public Optional campaignReport() { throw new UnsupportedOperationException(); }
@Override
public Optional> tags() { throw new UnsupportedOperationException(); }
@Override
public Long executionId() { throw new UnsupportedOperationException(); }
@Override
public String scenarioId() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableExecutionSummaryDto fromJson(Json json) {
ImmutableExecutionSummaryDto.Builder builder = ImmutableExecutionSummaryDto.builder();
if (json.time != null) {
builder.time(json.time);
}
if (json.durationIsSet) {
builder.duration(json.duration);
}
if (json.status != null) {
builder.status(json.status);
}
if (json.info != null) {
builder.info(json.info);
}
if (json.error != null) {
builder.error(json.error);
}
if (json.testCaseTitle != null) {
builder.testCaseTitle(json.testCaseTitle);
}
if (json.environment != null) {
builder.environment(json.environment);
}
if (json.dataset != null) {
builder.dataset(json.dataset);
}
if (json.user != null) {
builder.user(json.user);
}
if (json.campaignReport != null) {
builder.campaignReport(json.campaignReport);
}
if (json.tags != null) {
builder.tags(json.tags);
}
if (json.executionId != null) {
builder.executionId(json.executionId);
}
if (json.scenarioId != null) {
builder.scenarioId(json.scenarioId);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link ExecutionSummaryDto} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable ExecutionSummaryDto instance
*/
public static ImmutableExecutionSummaryDto copyOf(ExecutionSummaryDto instance) {
if (instance instanceof ImmutableExecutionSummaryDto) {
return (ImmutableExecutionSummaryDto) instance;
}
return ImmutableExecutionSummaryDto.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableExecutionSummaryDto ImmutableExecutionSummaryDto}.
*
* ImmutableExecutionSummaryDto.builder()
* .time(java.time.LocalDateTime) // required {@link ExecutionSummaryDto#time() time}
* .duration(long) // required {@link ExecutionSummaryDto#duration() duration}
* .status(com.chutneytesting.server.core.domain.execution.report.ServerReportStatus) // required {@link ExecutionSummaryDto#status() status}
* .info(String) // optional {@link ExecutionSummaryDto#info() info}
* .error(String) // optional {@link ExecutionSummaryDto#error() error}
* .testCaseTitle(String) // required {@link ExecutionSummaryDto#testCaseTitle() testCaseTitle}
* .environment(String) // required {@link ExecutionSummaryDto#environment() environment}
* .dataset(com.chutneytesting.server.core.domain.dataset.DataSet) // optional {@link ExecutionSummaryDto#dataset() dataset}
* .user(String) // required {@link ExecutionSummaryDto#user() user}
* .campaignReport(com.chutneytesting.server.core.domain.scenario.campaign.CampaignExecution) // optional {@link ExecutionSummaryDto#campaignReport() campaignReport}
* .tags(Set<String>) // optional {@link ExecutionSummaryDto#tags() tags}
* .executionId(Long) // required {@link ExecutionSummaryDto#executionId() executionId}
* .scenarioId(String) // required {@link ExecutionSummaryDto#scenarioId() scenarioId}
* .build();
*
* @return A new ImmutableExecutionSummaryDto builder
*/
public static ImmutableExecutionSummaryDto.Builder builder() {
return new ImmutableExecutionSummaryDto.Builder();
}
/**
* Builds instances of type {@link ImmutableExecutionSummaryDto ImmutableExecutionSummaryDto}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "ExecutionSummaryDto", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TIME = 0x1L;
private static final long INIT_BIT_DURATION = 0x2L;
private static final long INIT_BIT_STATUS = 0x4L;
private static final long INIT_BIT_TEST_CASE_TITLE = 0x8L;
private static final long INIT_BIT_ENVIRONMENT = 0x10L;
private static final long INIT_BIT_USER = 0x20L;
private static final long INIT_BIT_EXECUTION_ID = 0x40L;
private static final long INIT_BIT_SCENARIO_ID = 0x80L;
private long initBits = 0xffL;
private @Nullable LocalDateTime time;
private long duration;
private @Nullable ServerReportStatus status;
private @Nullable String info;
private @Nullable String error;
private @Nullable String testCaseTitle;
private @Nullable String environment;
private @Nullable DataSet dataset;
private @Nullable String user;
private @Nullable CampaignExecution campaignReport;
private @Nullable Set tags;
private @Nullable Long executionId;
private @Nullable String scenarioId;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.chutneytesting.server.core.domain.execution.history.ExecutionHistory.ExecutionProperties} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ExecutionHistory.ExecutionProperties instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.chutneytesting.server.core.domain.execution.history.ExecutionHistory.WithScenario} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ExecutionHistory.WithScenario instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.chutneytesting.server.core.domain.execution.history.ExecutionHistory.Attached} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ExecutionHistory.Attached instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.chutneytesting.execution.api.ExecutionSummaryDto} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ExecutionSummaryDto instance) {
Objects.requireNonNull(instance, "instance");
from((short) 0, (Object) instance);
return this;
}
private void from(short _unused, Object object) {
@Var long bits = 0;
if (object instanceof ExecutionHistory.ExecutionProperties) {
ExecutionHistory.ExecutionProperties instance = (ExecutionHistory.ExecutionProperties) object;
if ((bits & 0x4L) == 0) {
duration(instance.duration());
bits |= 0x4L;
}
if ((bits & 0x10L) == 0) {
environment(instance.environment());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
time(instance.time());
bits |= 0x20L;
}
if ((bits & 0x1L) == 0) {
Optional errorOptional = instance.error();
if (errorOptional.isPresent()) {
error(errorOptional);
}
bits |= 0x1L;
}
if ((bits & 0x40L) == 0) {
testCaseTitle(instance.testCaseTitle());
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
Optional datasetOptional = instance.dataset();
if (datasetOptional.isPresent()) {
dataset(datasetOptional);
}
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
user(instance.user());
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
Optional campaignReportOptional = instance.campaignReport();
if (campaignReportOptional.isPresent()) {
campaignReport(campaignReportOptional);
}
bits |= 0x200L;
}
if ((bits & 0x2L) == 0) {
Optional> tagsOptional = instance.tags();
if (tagsOptional.isPresent()) {
tags(tagsOptional);
}
bits |= 0x2L;
}
if ((bits & 0x800L) == 0) {
status(instance.status());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
Optional infoOptional = instance.info();
if (infoOptional.isPresent()) {
info(infoOptional);
}
bits |= 0x1000L;
}
}
if (object instanceof ExecutionHistory.WithScenario) {
ExecutionHistory.WithScenario instance = (ExecutionHistory.WithScenario) object;
if ((bits & 0x400L) == 0) {
scenarioId(instance.scenarioId());
bits |= 0x400L;
}
}
if (object instanceof ExecutionHistory.Attached) {
ExecutionHistory.Attached instance = (ExecutionHistory.Attached) object;
if ((bits & 0x8L) == 0) {
executionId(instance.executionId());
bits |= 0x8L;
}
}
if (object instanceof ExecutionSummaryDto) {
ExecutionSummaryDto instance = (ExecutionSummaryDto) object;
if ((bits & 0x1L) == 0) {
Optional errorOptional = instance.error();
if (errorOptional.isPresent()) {
error(errorOptional);
}
bits |= 0x1L;
}
if ((bits & 0x2L) == 0) {
Optional> tagsOptional = instance.tags();
if (tagsOptional.isPresent()) {
tags(tagsOptional);
}
bits |= 0x2L;
}
if ((bits & 0x4L) == 0) {
duration(instance.duration());
bits |= 0x4L;
}
if ((bits & 0x8L) == 0) {
executionId(instance.executionId());
bits |= 0x8L;
}
if ((bits & 0x10L) == 0) {
environment(instance.environment());
bits |= 0x10L;
}
if ((bits & 0x20L) == 0) {
time(instance.time());
bits |= 0x20L;
}
if ((bits & 0x40L) == 0) {
testCaseTitle(instance.testCaseTitle());
bits |= 0x40L;
}
if ((bits & 0x80L) == 0) {
Optional datasetOptional = instance.dataset();
if (datasetOptional.isPresent()) {
dataset(datasetOptional);
}
bits |= 0x80L;
}
if ((bits & 0x100L) == 0) {
user(instance.user());
bits |= 0x100L;
}
if ((bits & 0x200L) == 0) {
Optional campaignReportOptional = instance.campaignReport();
if (campaignReportOptional.isPresent()) {
campaignReport(campaignReportOptional);
}
bits |= 0x200L;
}
if ((bits & 0x400L) == 0) {
scenarioId(instance.scenarioId());
bits |= 0x400L;
}
if ((bits & 0x800L) == 0) {
status(instance.status());
bits |= 0x800L;
}
if ((bits & 0x1000L) == 0) {
Optional infoOptional = instance.info();
if (infoOptional.isPresent()) {
info(infoOptional);
}
bits |= 0x1000L;
}
}
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#time() time} attribute.
* @param time The value for time
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("time")
public final Builder time(LocalDateTime time) {
this.time = Objects.requireNonNull(time, "time");
initBits &= ~INIT_BIT_TIME;
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#duration() duration} attribute.
* @param duration The value for duration
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("duration")
public final Builder duration(long duration) {
this.duration = duration;
initBits &= ~INIT_BIT_DURATION;
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#status() status} attribute.
* @param status The value for status
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("status")
public final Builder status(ServerReportStatus status) {
this.status = Objects.requireNonNull(status, "status");
initBits &= ~INIT_BIT_STATUS;
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#info() info} to info.
* @param info The value for info
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder info(String info) {
this.info = Objects.requireNonNull(info, "info");
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#info() info} to info.
* @param info The value for info
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("info")
public final Builder info(Optional info) {
this.info = info.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#error() error} to error.
* @param error The value for error
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder error(String error) {
this.error = Objects.requireNonNull(error, "error");
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#error() error} to error.
* @param error The value for error
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("error")
public final Builder error(Optional error) {
this.error = error.orElse(null);
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#testCaseTitle() testCaseTitle} attribute.
* @param testCaseTitle The value for testCaseTitle
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("testCaseTitle")
public final Builder testCaseTitle(String testCaseTitle) {
this.testCaseTitle = Objects.requireNonNull(testCaseTitle, "testCaseTitle");
initBits &= ~INIT_BIT_TEST_CASE_TITLE;
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#environment() environment} attribute.
* @param environment The value for environment
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("environment")
public final Builder environment(String environment) {
this.environment = Objects.requireNonNull(environment, "environment");
initBits &= ~INIT_BIT_ENVIRONMENT;
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#dataset() dataset} to dataset.
* @param dataset The value for dataset
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder dataset(DataSet dataset) {
this.dataset = Objects.requireNonNull(dataset, "dataset");
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#dataset() dataset} to dataset.
* @param dataset The value for dataset
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("dataset")
public final Builder dataset(Optional extends DataSet> dataset) {
this.dataset = dataset.orElse(null);
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#user() user} attribute.
* @param user The value for user
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("user")
public final Builder user(String user) {
this.user = Objects.requireNonNull(user, "user");
initBits &= ~INIT_BIT_USER;
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#campaignReport() campaignReport} to campaignReport.
* @param campaignReport The value for campaignReport
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder campaignReport(CampaignExecution campaignReport) {
this.campaignReport = Objects.requireNonNull(campaignReport, "campaignReport");
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#campaignReport() campaignReport} to campaignReport.
* @param campaignReport The value for campaignReport
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("campaignReport")
public final Builder campaignReport(Optional extends CampaignExecution> campaignReport) {
this.campaignReport = campaignReport.orElse(null);
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#tags() tags} to tags.
* @param tags The value for tags
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder tags(Set tags) {
this.tags = Objects.requireNonNull(tags, "tags");
return this;
}
/**
* Initializes the optional value {@link ExecutionSummaryDto#tags() tags} to tags.
* @param tags The value for tags
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
public final Builder tags(Optional extends Set> tags) {
this.tags = tags.orElse(null);
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#executionId() executionId} attribute.
* @param executionId The value for executionId
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("executionId")
public final Builder executionId(Long executionId) {
this.executionId = Objects.requireNonNull(executionId, "executionId");
initBits &= ~INIT_BIT_EXECUTION_ID;
return this;
}
/**
* Initializes the value for the {@link ExecutionSummaryDto#scenarioId() scenarioId} attribute.
* @param scenarioId The value for scenarioId
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("scenarioId")
public final Builder scenarioId(String scenarioId) {
this.scenarioId = Objects.requireNonNull(scenarioId, "scenarioId");
initBits &= ~INIT_BIT_SCENARIO_ID;
return this;
}
/**
* Builds a new {@link ImmutableExecutionSummaryDto ImmutableExecutionSummaryDto}.
* @return An immutable instance of ExecutionSummaryDto
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableExecutionSummaryDto build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableExecutionSummaryDto(
time,
duration,
status,
info,
error,
testCaseTitle,
environment,
dataset,
user,
campaignReport,
tags,
executionId,
scenarioId);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TIME) != 0) attributes.add("time");
if ((initBits & INIT_BIT_DURATION) != 0) attributes.add("duration");
if ((initBits & INIT_BIT_STATUS) != 0) attributes.add("status");
if ((initBits & INIT_BIT_TEST_CASE_TITLE) != 0) attributes.add("testCaseTitle");
if ((initBits & INIT_BIT_ENVIRONMENT) != 0) attributes.add("environment");
if ((initBits & INIT_BIT_USER) != 0) attributes.add("user");
if ((initBits & INIT_BIT_EXECUTION_ID) != 0) attributes.add("executionId");
if ((initBits & INIT_BIT_SCENARIO_ID) != 0) attributes.add("scenarioId");
return "Cannot build ExecutionSummaryDto, some of required attributes are not set " + attributes;
}
}
}