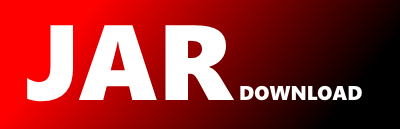
com.chutneytesting.scenario.api.raw.dto.ImmutableGwtTestCaseDto Maven / Gradle / Ivy
package com.chutneytesting.scenario.api.raw.dto;
import com.chutneytesting.execution.api.ExecutionSummaryDto;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.time.Instant;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link GwtTestCaseDto}.
*
* Use the builder to create immutable instances:
* {@code ImmutableGwtTestCaseDto.builder()}.
*/
@Generated(from = "GwtTestCaseDto", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutableGwtTestCaseDto implements GwtTestCaseDto {
private final @Nullable String id;
private final String title;
private final @Nullable String description;
private final @Nullable String repositorySource;
private final List tags;
private final List executions;
private final @Nullable Instant creationDate;
private final GwtScenarioDto scenario;
private final @Nullable String defaultDataset;
private final String author;
private final Instant updateDate;
private final Integer version;
private ImmutableGwtTestCaseDto(ImmutableGwtTestCaseDto.Builder builder) {
this.id = builder.id;
this.title = builder.title;
this.description = builder.description;
this.repositorySource = builder.repositorySource;
this.tags = createUnmodifiableList(true, builder.tags);
this.executions = createUnmodifiableList(true, builder.executions);
this.creationDate = builder.creationDate;
this.scenario = builder.scenario;
this.defaultDataset = builder.defaultDataset;
if (builder.author != null) {
initShim.author(builder.author);
}
if (builder.updateDate != null) {
initShim.updateDate(builder.updateDate);
}
if (builder.version != null) {
initShim.version(builder.version);
}
this.author = initShim.author();
this.updateDate = initShim.updateDate();
this.version = initShim.version();
this.initShim = null;
}
private ImmutableGwtTestCaseDto(
@Nullable String id,
String title,
@Nullable String description,
@Nullable String repositorySource,
List tags,
List executions,
@Nullable Instant creationDate,
GwtScenarioDto scenario,
@Nullable String defaultDataset,
String author,
Instant updateDate,
Integer version) {
this.id = id;
this.title = title;
this.description = description;
this.repositorySource = repositorySource;
this.tags = tags;
this.executions = executions;
this.creationDate = creationDate;
this.scenario = scenario;
this.defaultDataset = defaultDataset;
this.author = author;
this.updateDate = updateDate;
this.version = version;
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "GwtTestCaseDto", generator = "Immutables")
private final class InitShim {
private byte authorBuildStage = STAGE_UNINITIALIZED;
private String author;
String author() {
if (authorBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (authorBuildStage == STAGE_UNINITIALIZED) {
authorBuildStage = STAGE_INITIALIZING;
this.author = Objects.requireNonNull(authorInitialize(), "author");
authorBuildStage = STAGE_INITIALIZED;
}
return this.author;
}
void author(String author) {
this.author = author;
authorBuildStage = STAGE_INITIALIZED;
}
private byte updateDateBuildStage = STAGE_UNINITIALIZED;
private Instant updateDate;
Instant updateDate() {
if (updateDateBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (updateDateBuildStage == STAGE_UNINITIALIZED) {
updateDateBuildStage = STAGE_INITIALIZING;
this.updateDate = Objects.requireNonNull(updateDateInitialize(), "updateDate");
updateDateBuildStage = STAGE_INITIALIZED;
}
return this.updateDate;
}
void updateDate(Instant updateDate) {
this.updateDate = updateDate;
updateDateBuildStage = STAGE_INITIALIZED;
}
private byte versionBuildStage = STAGE_UNINITIALIZED;
private Integer version;
Integer version() {
if (versionBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (versionBuildStage == STAGE_UNINITIALIZED) {
versionBuildStage = STAGE_INITIALIZING;
this.version = Objects.requireNonNull(versionInitialize(), "version");
versionBuildStage = STAGE_INITIALIZED;
}
return this.version;
}
void version(Integer version) {
this.version = version;
versionBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (authorBuildStage == STAGE_INITIALIZING) attributes.add("author");
if (updateDateBuildStage == STAGE_INITIALIZING) attributes.add("updateDate");
if (versionBuildStage == STAGE_INITIALIZING) attributes.add("version");
return "Cannot build GwtTestCaseDto, attribute initializers form cycle " + attributes;
}
}
private String authorInitialize() {
return GwtTestCaseDto.super.author();
}
private Instant updateDateInitialize() {
return GwtTestCaseDto.super.updateDate();
}
private Integer versionInitialize() {
return GwtTestCaseDto.super.version();
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public Optional id() {
return Optional.ofNullable(id);
}
/**
* @return The value of the {@code title} attribute
*/
@JsonProperty("title")
@Override
public String title() {
return title;
}
/**
* @return The value of the {@code description} attribute
*/
@JsonProperty("description")
@Override
public Optional description() {
return Optional.ofNullable(description);
}
/**
* @return The value of the {@code repositorySource} attribute
*/
@JsonProperty("repositorySource")
@Override
public Optional repositorySource() {
return Optional.ofNullable(repositorySource);
}
/**
* @return The value of the {@code tags} attribute
*/
@JsonProperty("tags")
@Override
public List tags() {
return tags;
}
/**
* @return The value of the {@code executions} attribute
*/
@JsonProperty("executions")
@Override
public List executions() {
return executions;
}
/**
* @return The value of the {@code creationDate} attribute
*/
@JsonProperty("creationDate")
@Override
public Optional creationDate() {
return Optional.ofNullable(creationDate);
}
/**
* @return The value of the {@code scenario} attribute
*/
@JsonProperty("scenario")
@Override
public GwtScenarioDto scenario() {
return scenario;
}
/**
* @return The value of the {@code defaultDataset} attribute
*/
@JsonProperty("defaultDataset")
@Override
public Optional defaultDataset() {
return Optional.ofNullable(defaultDataset);
}
/**
* @return The value of the {@code author} attribute
*/
@JsonProperty("author")
@Override
public String author() {
InitShim shim = this.initShim;
return shim != null
? shim.author()
: this.author;
}
/**
* @return The value of the {@code updateDate} attribute
*/
@JsonProperty("updateDate")
@Override
public Instant updateDate() {
InitShim shim = this.initShim;
return shim != null
? shim.updateDate()
: this.updateDate;
}
/**
* @return The value of the {@code version} attribute
*/
@JsonProperty("version")
@Override
public Integer version() {
InitShim shim = this.initShim;
return shim != null
? shim.version()
: this.version;
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseDto#id() id} attribute.
* @param value The value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (Objects.equals(this.id, newValue)) return this;
return new ImmutableGwtTestCaseDto(
newValue,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseDto#id() id} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for id
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withId(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.id, value)) return this;
return new ImmutableGwtTestCaseDto(
value,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseDto#title() title} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for title
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseDto withTitle(String value) {
String newValue = Objects.requireNonNull(value, "title");
if (this.title.equals(newValue)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
newValue,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseDto#description() description} attribute.
* @param value The value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withDescription(String value) {
String newValue = Objects.requireNonNull(value, "description");
if (Objects.equals(this.description, newValue)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
newValue,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseDto#description() description} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for description
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withDescription(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.description, value)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
value,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseDto#repositorySource() repositorySource} attribute.
* @param value The value for repositorySource
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withRepositorySource(String value) {
String newValue = Objects.requireNonNull(value, "repositorySource");
if (Objects.equals(this.repositorySource, newValue)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
newValue,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseDto#repositorySource() repositorySource} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for repositorySource
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withRepositorySource(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.repositorySource, value)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
value,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseDto#tags() tags}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withTags(String... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
newValue,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseDto#tags() tags}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of tags elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withTags(Iterable elements) {
if (this.tags == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
newValue,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseDto#executions() executions}.
* @param elements The elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withExecutions(ExecutionSummaryDto... elements) {
List newValue = createUnmodifiableList(false, createSafeList(Arrays.asList(elements), true, false));
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
newValue,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object with elements that replace the content of {@link GwtTestCaseDto#executions() executions}.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param elements An iterable of executions elements to set
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withExecutions(Iterable extends ExecutionSummaryDto> elements) {
if (this.executions == elements) return this;
List newValue = createUnmodifiableList(false, createSafeList(elements, true, false));
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
newValue,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseDto#creationDate() creationDate} attribute.
* @param value The value for creationDate
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withCreationDate(Instant value) {
Instant newValue = Objects.requireNonNull(value, "creationDate");
if (this.creationDate == newValue) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
newValue,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseDto#creationDate() creationDate} attribute.
* A shallow reference equality check is used on unboxed optional value to prevent copying of the same value by returning {@code this}.
* @param optional A value for creationDate
* @return A modified copy of {@code this} object
*/
@SuppressWarnings("unchecked") // safe covariant cast
public final ImmutableGwtTestCaseDto withCreationDate(Optional extends Instant> optional) {
@Nullable Instant value = optional.orElse(null);
if (this.creationDate == value) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
value,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseDto#scenario() scenario} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for scenario
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseDto withScenario(GwtScenarioDto value) {
if (this.scenario == value) return this;
GwtScenarioDto newValue = Objects.requireNonNull(value, "scenario");
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
newValue,
this.defaultDataset,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link GwtTestCaseDto#defaultDataset() defaultDataset} attribute.
* @param value The value for defaultDataset
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withDefaultDataset(String value) {
String newValue = Objects.requireNonNull(value, "defaultDataset");
if (Objects.equals(this.defaultDataset, newValue)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
newValue,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting an optional value for the {@link GwtTestCaseDto#defaultDataset() defaultDataset} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for defaultDataset
* @return A modified copy of {@code this} object
*/
public final ImmutableGwtTestCaseDto withDefaultDataset(Optional optional) {
@Nullable String value = optional.orElse(null);
if (Objects.equals(this.defaultDataset, value)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
value,
this.author,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseDto#author() author} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for author
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseDto withAuthor(String value) {
String newValue = Objects.requireNonNull(value, "author");
if (this.author.equals(newValue)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
newValue,
this.updateDate,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseDto#updateDate() updateDate} attribute.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for updateDate
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseDto withUpdateDate(Instant value) {
if (this.updateDate == value) return this;
Instant newValue = Objects.requireNonNull(value, "updateDate");
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
newValue,
this.version);
}
/**
* Copy the current immutable object by setting a value for the {@link GwtTestCaseDto#version() version} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for version
* @return A modified copy of the {@code this} object
*/
public final ImmutableGwtTestCaseDto withVersion(Integer value) {
Integer newValue = Objects.requireNonNull(value, "version");
if (this.version.equals(newValue)) return this;
return new ImmutableGwtTestCaseDto(
this.id,
this.title,
this.description,
this.repositorySource,
this.tags,
this.executions,
this.creationDate,
this.scenario,
this.defaultDataset,
this.author,
this.updateDate,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableGwtTestCaseDto} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableGwtTestCaseDto
&& equalTo(0, (ImmutableGwtTestCaseDto) another);
}
private boolean equalTo(int synthetic, ImmutableGwtTestCaseDto another) {
return Objects.equals(id, another.id)
&& title.equals(another.title)
&& Objects.equals(description, another.description)
&& Objects.equals(repositorySource, another.repositorySource)
&& tags.equals(another.tags)
&& executions.equals(another.executions)
&& Objects.equals(creationDate, another.creationDate)
&& scenario.equals(another.scenario)
&& Objects.equals(defaultDataset, another.defaultDataset)
&& author.equals(another.author)
&& updateDate.equals(another.updateDate)
&& version.equals(another.version);
}
/**
* Computes a hash code from attributes: {@code id}, {@code title}, {@code description}, {@code repositorySource}, {@code tags}, {@code executions}, {@code creationDate}, {@code scenario}, {@code defaultDataset}, {@code author}, {@code updateDate}, {@code version}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Objects.hashCode(id);
h += (h << 5) + title.hashCode();
h += (h << 5) + Objects.hashCode(description);
h += (h << 5) + Objects.hashCode(repositorySource);
h += (h << 5) + tags.hashCode();
h += (h << 5) + executions.hashCode();
h += (h << 5) + Objects.hashCode(creationDate);
h += (h << 5) + scenario.hashCode();
h += (h << 5) + Objects.hashCode(defaultDataset);
h += (h << 5) + author.hashCode();
h += (h << 5) + updateDate.hashCode();
h += (h << 5) + version.hashCode();
return h;
}
/**
* Prints the immutable value {@code GwtTestCaseDto} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
StringBuilder builder = new StringBuilder("GwtTestCaseDto{");
if (id != null) {
builder.append("id=").append(id);
}
if (builder.length() > 15) builder.append(", ");
builder.append("title=").append(title);
if (description != null) {
builder.append(", ");
builder.append("description=").append(description);
}
if (repositorySource != null) {
builder.append(", ");
builder.append("repositorySource=").append(repositorySource);
}
builder.append(", ");
builder.append("tags=").append(tags);
builder.append(", ");
builder.append("executions=").append(executions);
if (creationDate != null) {
builder.append(", ");
builder.append("creationDate=").append(creationDate);
}
builder.append(", ");
builder.append("scenario=").append(scenario);
if (defaultDataset != null) {
builder.append(", ");
builder.append("defaultDataset=").append(defaultDataset);
}
builder.append(", ");
builder.append("author=").append(author);
builder.append(", ");
builder.append("updateDate=").append(updateDate);
builder.append(", ");
builder.append("version=").append(version);
return builder.append("}").toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "GwtTestCaseDto", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements GwtTestCaseDto {
@Nullable Optional id = Optional.empty();
@Nullable String title;
@Nullable Optional description = Optional.empty();
@Nullable Optional repositorySource = Optional.empty();
@Nullable List tags = Collections.emptyList();
@Nullable List executions = Collections.emptyList();
@Nullable Optional creationDate = Optional.empty();
@Nullable GwtScenarioDto scenario;
@Nullable Optional defaultDataset = Optional.empty();
@Nullable String author;
@Nullable Instant updateDate;
@Nullable Integer version;
@JsonProperty("id")
public void setId(Optional id) {
this.id = id;
}
@JsonProperty("title")
public void setTitle(String title) {
this.title = title;
}
@JsonProperty("description")
public void setDescription(Optional description) {
this.description = description;
}
@JsonProperty("repositorySource")
public void setRepositorySource(Optional repositorySource) {
this.repositorySource = repositorySource;
}
@JsonProperty("tags")
public void setTags(List tags) {
this.tags = tags;
}
@JsonProperty("executions")
public void setExecutions(List executions) {
this.executions = executions;
}
@JsonProperty("creationDate")
public void setCreationDate(Optional creationDate) {
this.creationDate = creationDate;
}
@JsonProperty("scenario")
public void setScenario(GwtScenarioDto scenario) {
this.scenario = scenario;
}
@JsonProperty("defaultDataset")
public void setDefaultDataset(Optional defaultDataset) {
this.defaultDataset = defaultDataset;
}
@JsonProperty("author")
public void setAuthor(String author) {
this.author = author;
}
@JsonProperty("updateDate")
public void setUpdateDate(Instant updateDate) {
this.updateDate = updateDate;
}
@JsonProperty("version")
public void setVersion(Integer version) {
this.version = version;
}
@Override
public Optional id() { throw new UnsupportedOperationException(); }
@Override
public String title() { throw new UnsupportedOperationException(); }
@Override
public Optional description() { throw new UnsupportedOperationException(); }
@Override
public Optional repositorySource() { throw new UnsupportedOperationException(); }
@Override
public List tags() { throw new UnsupportedOperationException(); }
@Override
public List executions() { throw new UnsupportedOperationException(); }
@Override
public Optional creationDate() { throw new UnsupportedOperationException(); }
@Override
public GwtScenarioDto scenario() { throw new UnsupportedOperationException(); }
@Override
public Optional defaultDataset() { throw new UnsupportedOperationException(); }
@Override
public String author() { throw new UnsupportedOperationException(); }
@Override
public Instant updateDate() { throw new UnsupportedOperationException(); }
@Override
public Integer version() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableGwtTestCaseDto fromJson(Json json) {
ImmutableGwtTestCaseDto.Builder builder = ImmutableGwtTestCaseDto.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.title != null) {
builder.title(json.title);
}
if (json.description != null) {
builder.description(json.description);
}
if (json.repositorySource != null) {
builder.repositorySource(json.repositorySource);
}
if (json.tags != null) {
builder.addAllTags(json.tags);
}
if (json.executions != null) {
builder.addAllExecutions(json.executions);
}
if (json.creationDate != null) {
builder.creationDate(json.creationDate);
}
if (json.scenario != null) {
builder.scenario(json.scenario);
}
if (json.defaultDataset != null) {
builder.defaultDataset(json.defaultDataset);
}
if (json.author != null) {
builder.author(json.author);
}
if (json.updateDate != null) {
builder.updateDate(json.updateDate);
}
if (json.version != null) {
builder.version(json.version);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link GwtTestCaseDto} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable GwtTestCaseDto instance
*/
public static ImmutableGwtTestCaseDto copyOf(GwtTestCaseDto instance) {
if (instance instanceof ImmutableGwtTestCaseDto) {
return (ImmutableGwtTestCaseDto) instance;
}
return ImmutableGwtTestCaseDto.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableGwtTestCaseDto ImmutableGwtTestCaseDto}.
*
* ImmutableGwtTestCaseDto.builder()
* .id(String) // optional {@link GwtTestCaseDto#id() id}
* .title(String) // required {@link GwtTestCaseDto#title() title}
* .description(String) // optional {@link GwtTestCaseDto#description() description}
* .repositorySource(String) // optional {@link GwtTestCaseDto#repositorySource() repositorySource}
* .addTags|addAllTags(String) // {@link GwtTestCaseDto#tags() tags} elements
* .addExecutions|addAllExecutions(com.chutneytesting.execution.api.ExecutionSummaryDto) // {@link GwtTestCaseDto#executions() executions} elements
* .creationDate(java.time.Instant) // optional {@link GwtTestCaseDto#creationDate() creationDate}
* .scenario(com.chutneytesting.scenario.api.raw.dto.GwtScenarioDto) // required {@link GwtTestCaseDto#scenario() scenario}
* .defaultDataset(String) // optional {@link GwtTestCaseDto#defaultDataset() defaultDataset}
* .author(String) // optional {@link GwtTestCaseDto#author() author}
* .updateDate(java.time.Instant) // optional {@link GwtTestCaseDto#updateDate() updateDate}
* .version(Integer) // optional {@link GwtTestCaseDto#version() version}
* .build();
*
* @return A new ImmutableGwtTestCaseDto builder
*/
public static ImmutableGwtTestCaseDto.Builder builder() {
return new ImmutableGwtTestCaseDto.Builder();
}
/**
* Builds instances of type {@link ImmutableGwtTestCaseDto ImmutableGwtTestCaseDto}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "GwtTestCaseDto", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_TITLE = 0x1L;
private static final long INIT_BIT_SCENARIO = 0x2L;
private long initBits = 0x3L;
private @Nullable String id;
private @Nullable String title;
private @Nullable String description;
private @Nullable String repositorySource;
private List tags = new ArrayList();
private List executions = new ArrayList();
private @Nullable Instant creationDate;
private @Nullable GwtScenarioDto scenario;
private @Nullable String defaultDataset;
private @Nullable String author;
private @Nullable Instant updateDate;
private @Nullable Integer version;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code GwtTestCaseDto} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(GwtTestCaseDto instance) {
Objects.requireNonNull(instance, "instance");
Optional idOptional = instance.id();
if (idOptional.isPresent()) {
id(idOptional);
}
title(instance.title());
Optional descriptionOptional = instance.description();
if (descriptionOptional.isPresent()) {
description(descriptionOptional);
}
Optional repositorySourceOptional = instance.repositorySource();
if (repositorySourceOptional.isPresent()) {
repositorySource(repositorySourceOptional);
}
addAllTags(instance.tags());
addAllExecutions(instance.executions());
Optional creationDateOptional = instance.creationDate();
if (creationDateOptional.isPresent()) {
creationDate(creationDateOptional);
}
scenario(instance.scenario());
Optional defaultDatasetOptional = instance.defaultDataset();
if (defaultDatasetOptional.isPresent()) {
defaultDataset(defaultDatasetOptional);
}
author(instance.author());
updateDate(instance.updateDate());
version(instance.version());
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#id() id} to id.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final Builder id(Optional id) {
this.id = id.orElse(null);
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseDto#title() title} attribute.
* @param title The value for title
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("title")
public final Builder title(String title) {
this.title = Objects.requireNonNull(title, "title");
initBits &= ~INIT_BIT_TITLE;
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#description() description} to description.
* @param description The value for description
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder description(String description) {
this.description = Objects.requireNonNull(description, "description");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#description() description} to description.
* @param description The value for description
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("description")
public final Builder description(Optional description) {
this.description = description.orElse(null);
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#repositorySource() repositorySource} to repositorySource.
* @param repositorySource The value for repositorySource
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder repositorySource(String repositorySource) {
this.repositorySource = Objects.requireNonNull(repositorySource, "repositorySource");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#repositorySource() repositorySource} to repositorySource.
* @param repositorySource The value for repositorySource
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("repositorySource")
public final Builder repositorySource(Optional repositorySource) {
this.repositorySource = repositorySource.orElse(null);
return this;
}
/**
* Adds one element to {@link GwtTestCaseDto#tags() tags} list.
* @param element A tags element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String element) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
return this;
}
/**
* Adds elements to {@link GwtTestCaseDto#tags() tags} list.
* @param elements An array of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addTags(String... elements) {
for (String element : elements) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link GwtTestCaseDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tags")
public final Builder tags(Iterable elements) {
this.tags.clear();
return addAllTags(elements);
}
/**
* Adds elements to {@link GwtTestCaseDto#tags() tags} list.
* @param elements An iterable of tags elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllTags(Iterable elements) {
for (String element : elements) {
this.tags.add(Objects.requireNonNull(element, "tags element"));
}
return this;
}
/**
* Adds one element to {@link GwtTestCaseDto#executions() executions} list.
* @param element A executions element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addExecutions(ExecutionSummaryDto element) {
this.executions.add(Objects.requireNonNull(element, "executions element"));
return this;
}
/**
* Adds elements to {@link GwtTestCaseDto#executions() executions} list.
* @param elements An array of executions elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addExecutions(ExecutionSummaryDto... elements) {
for (ExecutionSummaryDto element : elements) {
this.executions.add(Objects.requireNonNull(element, "executions element"));
}
return this;
}
/**
* Sets or replaces all elements for {@link GwtTestCaseDto#executions() executions} list.
* @param elements An iterable of executions elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("executions")
public final Builder executions(Iterable extends ExecutionSummaryDto> elements) {
this.executions.clear();
return addAllExecutions(elements);
}
/**
* Adds elements to {@link GwtTestCaseDto#executions() executions} list.
* @param elements An iterable of executions elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder addAllExecutions(Iterable extends ExecutionSummaryDto> elements) {
for (ExecutionSummaryDto element : elements) {
this.executions.add(Objects.requireNonNull(element, "executions element"));
}
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#creationDate() creationDate} to creationDate.
* @param creationDate The value for creationDate
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder creationDate(Instant creationDate) {
this.creationDate = Objects.requireNonNull(creationDate, "creationDate");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#creationDate() creationDate} to creationDate.
* @param creationDate The value for creationDate
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("creationDate")
public final Builder creationDate(Optional extends Instant> creationDate) {
this.creationDate = creationDate.orElse(null);
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseDto#scenario() scenario} attribute.
* @param scenario The value for scenario
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("scenario")
public final Builder scenario(GwtScenarioDto scenario) {
this.scenario = Objects.requireNonNull(scenario, "scenario");
initBits &= ~INIT_BIT_SCENARIO;
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#defaultDataset() defaultDataset} to defaultDataset.
* @param defaultDataset The value for defaultDataset
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder defaultDataset(String defaultDataset) {
this.defaultDataset = Objects.requireNonNull(defaultDataset, "defaultDataset");
return this;
}
/**
* Initializes the optional value {@link GwtTestCaseDto#defaultDataset() defaultDataset} to defaultDataset.
* @param defaultDataset The value for defaultDataset
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("defaultDataset")
public final Builder defaultDataset(Optional defaultDataset) {
this.defaultDataset = defaultDataset.orElse(null);
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseDto#author() author} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link GwtTestCaseDto#author() author}.
* @param author The value for author
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("author")
public final Builder author(String author) {
this.author = Objects.requireNonNull(author, "author");
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseDto#updateDate() updateDate} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GwtTestCaseDto#updateDate() updateDate}.
* @param updateDate The value for updateDate
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("updateDate")
public final Builder updateDate(Instant updateDate) {
this.updateDate = Objects.requireNonNull(updateDate, "updateDate");
return this;
}
/**
* Initializes the value for the {@link GwtTestCaseDto#version() version} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link GwtTestCaseDto#version() version}.
* @param version The value for version
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("version")
public final Builder version(Integer version) {
this.version = Objects.requireNonNull(version, "version");
return this;
}
/**
* Builds a new {@link ImmutableGwtTestCaseDto ImmutableGwtTestCaseDto}.
* @return An immutable instance of GwtTestCaseDto
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableGwtTestCaseDto build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableGwtTestCaseDto(this);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_TITLE) != 0) attributes.add("title");
if ((initBits & INIT_BIT_SCENARIO) != 0) attributes.add("scenario");
return "Cannot build GwtTestCaseDto, some of required attributes are not set " + attributes;
}
}
private static List createSafeList(Iterable extends T> iterable, boolean checkNulls, boolean skipNulls) {
ArrayList list;
if (iterable instanceof Collection>) {
int size = ((Collection>) iterable).size();
if (size == 0) return Collections.emptyList();
list = new ArrayList<>(size);
} else {
list = new ArrayList<>();
}
for (T element : iterable) {
if (skipNulls && element == null) continue;
if (checkNulls) Objects.requireNonNull(element, "element");
list.add(element);
}
return list;
}
private static List createUnmodifiableList(boolean clone, List list) {
switch(list.size()) {
case 0: return Collections.emptyList();
case 1: return Collections.singletonList(list.get(0));
default:
if (clone) {
return Collections.unmodifiableList(new ArrayList<>(list));
} else {
if (list instanceof ArrayList>) {
((ArrayList>) list).trimToSize();
}
return Collections.unmodifiableList(list);
}
}
}
}