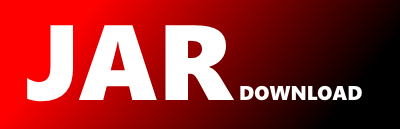
src.app.core.services.step.service.ts Maven / Gradle / Ivy
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { environment } from '@env/environment';
import { Observable } from 'rxjs';
import { isNullOrBlankString } from '@shared/tools';
@Injectable({
providedIn: 'root'
})
export class StepService {
private stepUrl = '/api/steps/v1';
constructor(private http: HttpClient) {
}
findAllSteps(start: number, limit: number, name: string, usage: string, sort: string): Observable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy