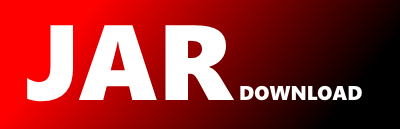
src.app.modules.scenarios.components.execution.execution.component.ts Maven / Gradle / Ivy
import { Component, OnDestroy, OnInit } from '@angular/core';
import { Location } from '@angular/common';
import { ActivatedRoute, Router } from '@angular/router';
import { Observable, Subscription } from 'rxjs';
import { debounceTime, delay, tap } from 'rxjs/internal/operators';
import { EventManagerService } from '@shared/event-manager.service';
import { Execution, GwtTestCase, ScenarioComponent, ScenarioExecutionReport, StepExecutionReport, TestCase, Authorization } from '@model';
import { ComponentService, ScenarioExecutionService, ScenarioService } from '@core/services';
@Component({
selector: 'chutney-execution',
providers: [Location],
templateUrl: './execution.component.html',
styleUrls: ['./execution.component.scss']
})
export class ScenarioExecutionComponent implements OnInit, OnDestroy {
scenarioComponent$: Observable = null;
scenarioGwt$: Observable = null;
parseError: String;
executionError: String;
currentScenarioId: string;
currentExecutionId: number;
scenarioExecutionReport: ScenarioExecutionReport;
lastExecutionRunning = false;
toggleScenarioDetails = true;
toggleScenarioInfo = true;
private isComposed = TestCase.isComposed;
private hasParameters: boolean = null;
private routeParamsSubscription: Subscription;
private scenarioExecutionAsyncSubscription: Subscription;
Authorization = Authorization;
constructor(
private eventManager: EventManagerService,
private scenarioService: ScenarioService,
private scenarioExecutionService: ScenarioExecutionService,
private componentService: ComponentService,
private route: ActivatedRoute,
private router: Router,
private location: Location,
) {
}
ngOnInit() {
this.routeParamsSubscription = this.route.params.subscribe((params) => {
this.currentScenarioId = params['id'];
if (params['execId'] && params['execId'] !== 'last') {
this.loadScenarioExecution(params['execId']);
}
this.loadScenario();
});
}
ngOnDestroy() {
this.eventManager.destroy(this.routeParamsSubscription);
this.unsubscribeScenarioExecutionAsyncSubscription();
}
loadScenario() {
if (this.isComposed(this.currentScenarioId)) {
this.scenarioComponent$ = this.componentService
.findComponentTestCaseWithoutDeserializeImpl(this.currentScenarioId).pipe(
tap(sc => {
this.hasParameters = (sc.computedParameters && sc.computedParameters.length > 0);
})
);
} else {
this.scenarioGwt$ = this.scenarioService
.findTestCase(this.currentScenarioId).pipe(
tap(gwt => {
this.hasParameters = (gwt.wrappedParams.params && gwt.wrappedParams.params.length > 0);
})
);
}
}
onlastStatusExecution(status: String) {
this.lastExecutionRunning = (status === 'RUNNING' || status === 'PAUSED');
}
onLastIdExecution(execution: Execution) {
if (Execution.NO_EXECUTION === execution) {
this.currentExecutionId = null;
} else if (this.currentExecutionId !== execution.executionId) {
this.currentExecutionId = execution.executionId;
if (!this.scenarioExecutionAsyncSubscription || this.scenarioExecutionAsyncSubscription.closed) {
if ('RUNNING' === execution.status) {
this.observeScenarioExecution(execution.executionId);
} else {
this.loadScenarioExecution(execution.executionId);
}
}
}
}
onSelectExecution(execution: Execution) {
if (execution != null) {
this.currentExecutionId = execution.executionId;
this.executionError = '';
this.unsubscribeScenarioExecutionAsyncSubscription();
if ('RUNNING' === execution.status) {
this.observeScenarioExecution(execution.executionId);
} else {
this.loadScenarioExecution(execution.executionId);
}
} else {
this.currentExecutionId = null;
this.executionError = '';
this.scenarioExecutionReport = null;
}
}
loadScenarioExecution(executionId: number) {
this.executionError = '';
this.currentExecutionId = executionId;
this.scenarioExecutionService.findExecutionReport(this.currentScenarioId, executionId)
.subscribe((scenarioExecutionReport: ScenarioExecutionReport) => {
this.updateLocation(executionId);
if (scenarioExecutionReport && scenarioExecutionReport.report.status === 'RUNNING') {
this.observeScenarioExecution(executionId);
} else {
this.toggleScenarioDetails = true;
this.scenarioExecutionReport = scenarioExecutionReport;
}
}, error => {
console.error(error.message);
this.executionError = 'Cannot find execution n°' + executionId;
this.scenarioExecutionReport = null;
});
}
executeScenario(env: string) {
if (this.hasParameters == null) {
let scenario$ : Observable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy