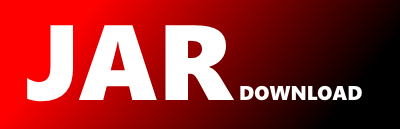
com.cinchapi.common.base.AnyObjects Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of accent4j Show documentation
Show all versions of accent4j Show documentation
Accent4J is a suite of libraries, helpers and data structures that make Java programming idioms more fluent.
/*
* Copyright (c) 2015 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.common.base;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import javax.annotation.Nullable;
import com.cinchapi.common.collect.Sequences;
import com.cinchapi.common.describe.Empty;
/**
* Helper functions
* (that are not already contained in {@link java.util.Objects
* Objects} or other common utility classes for the same purpose) that
* operate on any {@link Object}.
*
* @author Jeff Nelson
*/
public final class AnyObjects {
/**
* An {@link Empty} instance to implement empty checking methods.
*/
private static Empty EMPTY = Empty.is();
/**
* Check if {@code value} is {@code null} or semantically
* {@link #isNullOrEmpty(Object) empty}. If so, throw an
* {@link IllegalArgumentException}. Otherwise, return {@code value}.
*
* @param value the value to check
* @return {@code value} if it is not {@code null} or empty
*/
public static T checkNotNullOrEmpty(T value) {
return checkNotNullOrEmpty(value, null);
}
/**
* Check if {@code value} is {@code null} or semantically
* {@link #isNullOrEmpty(Object) empty}. If so, throw an
* {@link IllegalArgumentException} with {@code message}. Otherwise, return
* {@code value}.
*
* @param value the value to check
* @param message the message for the exception
* @return {@code value} if it is not {@code null} or empty
*/
public static T checkNotNullOrEmpty(T value, Object message) {
if(!isNullOrEmpty(value)) {
return value;
}
else {
throw message == null ? new IllegalArgumentException()
: new IllegalArgumentException(message.toString());
}
}
/**
* This function takes a generic {@code value} and a character as the
* delimiter.
*
*
* Firstly, if the generic is a sequence, then we iterate through each item
* and split the string representation, by the delimiter, of each item
* individually, trimming any whitespace away. We then convert this new
* sequence to a list and return that.
*
*
*
* Secondly, if the generic is not a sequence, then we simply convert the
* item to a String and split by the delimiter, again trimming the
* whitespace, and then we convert that new sequence to a list and return
* that.
*
*
* @param value The generic value
* @param delimiter The character that we use to specify the boundary
* between different parts of the text.
* @param The type of the generic value
* @return a list based on the {@code value} being split
*/
public static List split(T value, char delimiter) {
Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy