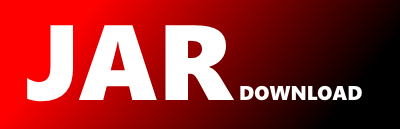
com.cinchapi.common.base.CheckedExceptions Maven / Gradle / Ivy
Show all versions of accent4j Show documentation
/*
* Copyright (c) 2015 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.common.base;
import com.cinchapi.common.reflect.Reflection;
/**
* Functions that eliminate the annoyance of checked {@link Exceptions}.
*
* The pros and cons of checked exceptions in Java is ripe for endless debate.
* In legitimate cases where you don't want to handle an exceptions in a method
* AND you don't want to force callers of a method to handle them either (e.g.
* by declaring that the method throws the exception), you can use this class to
* replace or wrap the exception with a {@link RuntimeException} type that is
* unchecked by the compiler.
*
*
* @author Jeff Nelson
*/
public final class CheckedExceptions {
/**
* Take a checked {@link Exception} and throw it as a
* {@link RuntimeException}.
*
* Unlike {@link #wrapAsRuntimeException(Exception)}, this method replaces
* {@code e} with a RuntimeException that has the same
* {@link Exception#getStackTrace() stack trace} as {@code e}.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.throwAsRuntimeException(e);
* }
*
*
* @param e the checked {@link Exception} to replace
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws RuntimeException
*/
public static RuntimeException throwAsRuntimeException(Exception e) {
return throwAsRuntimeException((Throwable) e);
}
/**
* Take a checked {@link Exception} and throw it as a
* {@link RuntimeException}.
*
* Unlike {@link #wrapAsRuntimeException(Exception, Class)}, this method
* replaces {@code e} with an instance of the {@code desiredType} that has
* the same {@link Exception#getStackTrace() stack trace} as {@code e}.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.throwAsRuntimeException(e,
* IllegalArgumentException.class);
* }
*
*
* @param e the checked {@link Exception} to replace
* @param desiredType the type of {@link RuntimeException} with which to
* wrap {@code e}
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws T
*/
public static T throwAsRuntimeException(Exception e, Class desiredType) {
return throwAsRuntimeException((Throwable) e, desiredType);
}
/**
* Take a checked {@link Exception} and throw it as a
* {@link RuntimeException}.
*
* Unlike {@link #wrapAsRuntimeException(Exception)}, this method replaces
* {@code e} with a RuntimeException that has the same
* {@link Exception#getStackTrace() stack trace} as {@code e}.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.throwAsRuntimeException(e);
* }
*
*
* @param e the checked {@link Exception} to replace
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws RuntimeException
*/
public static RuntimeException throwAsRuntimeException(Throwable e) {
if(e instanceof RuntimeException) {
throw (RuntimeException) e;
}
else {
RuntimeException re = new RuntimeException(AnyStrings
.format("{} {}", e.getClass().getName(), e.getMessage()));
re.setStackTrace(e.getStackTrace());
throw re;
}
}
/**
* Take a checked {@link Exception} and throw it as a
* {@link RuntimeException}.
*
* Unlike {@link #wrapAsRuntimeException(Exception, Class)}, this method
* replaces {@code e} with an instance of the {@code desiredType} that has
* the same {@link Exception#getStackTrace() stack trace} as {@code e}.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.throwAsRuntimeException(e,
* IllegalArgumentException.class);
* }
*
*
* @param e the checked {@link Exception} to replace
* @param desiredType the type of {@link RuntimeException} with which to
* wrap {@code e}
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws T
*/
@SuppressWarnings("unchecked")
public static T throwAsRuntimeException(
Throwable e, Class desiredType) {
if(e.getClass() == desiredType) {
throw (T) e;
}
else {
T re = Reflection.newInstance(desiredType);
re.setStackTrace(e.getStackTrace());
throw re;
}
}
/**
* Wrap a checked {@link Exception} within a {@link RuntimeException}.
*
* Unlike {@link #throwAsRuntimeException(Exception)} this method does not
* replace {@code e}. Instead it generates a new RuntimeException with
* {@code e} as the cause.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.wrapAsRuntimeException(e);
* }
*
*
* @param e the checked {@link Exception} to wrap
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws RuntimeException
*/
public static RuntimeException wrapAsRuntimeException(Exception e) {
return wrapAsRuntimeException((Throwable) e);
}
/**
* Wrap a checked {@link Exception} within a {@link RuntimeException}.
*
* Unlike {@link #throwAsRuntimeException(Exception, Class)} this method
* does not replace {@code e}. Instead it generates a new RuntimeException
* with {@code e} as the cause.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.wrapAsRuntimeException(e);
* }
*
*
* @param e the checked {@link Exception} to wrap
* @param desiredType the type of {@link RuntimeException} with which to
* wrap {@code e}
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws T
*/
public static T wrapAsRuntimeException(Exception e, Class desiredType) {
return wrapAsRuntimeException((Throwable) e, desiredType);
}
/**
* Wrap a checked {@link Exception} within a {@link RuntimeException}.
*
* Unlike {@link #throwAsRuntimeException(Exception)} this method does not
* replace {@code e}. Instead it generates a new RuntimeException with
* {@code e} as the cause.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.wrapAsRuntimeException(e);
* }
*
*
* @param e the checked {@link Exception} to wrap
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws RuntimeException
*/
public static RuntimeException wrapAsRuntimeException(Throwable e) {
if(e instanceof RuntimeException) {
throw (RuntimeException) e;
}
else {
RuntimeException re = new RuntimeException(e);
throw re;
}
}
/**
* Wrap a checked {@link Exception} within a {@link RuntimeException}.
*
* Unlike {@link #throwAsRuntimeException(Exception, Class)} this method
* does not replace {@code e}. Instead it generates a new RuntimeException
* with {@code e} as the cause.
*
* Example
*
*
* try {
* methodThatThrowsException();
* }
* catch (Exception e) {
* throw Exceptions.wrapAsRuntimeException(e);
* }
*
*
* @param e the checked {@link Exception} to wrap
* @param desiredType the type of {@link RuntimeException} with which to
* wrap {@code e}
* @return nothing, return type is only so that an invocation of this method
* can be chained with the throw keyword (see example
* above)
* @throws T
*/
@SuppressWarnings("unchecked")
public static T wrapAsRuntimeException(
Throwable e, Class desiredType) {
if(e.getClass() == desiredType) {
throw (T) e;
}
else {
T re = Reflection.newInstance(desiredType, e);
throw re;
}
}
private CheckedExceptions() {/* noinit */}
}