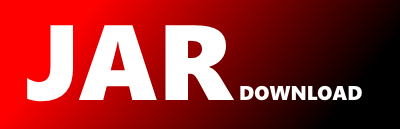
com.cinchapi.concourse.thrift.AccessToken Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of concourse-driver-java Show documentation
Show all versions of concourse-driver-java Show documentation
Concourse is a self-tuning database that is designed for both ad hoc analytics and high volume transactions at scale. Developers use Concourse to quickly build mission critical software while also benefiting from real time insight into their most important data. With Concourse, end-to-end data management requires no extra infrastructure, no prior configuration and no additional coding–all of which greatly reduce costs and allow developers to focus on core business problems.
/*
* Copyright (c) 2013-2019 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.concourse.thrift;
import java.nio.ByteBuffer;
import java.util.Arrays;
import java.util.Collections;
import java.util.EnumMap;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.Map;
import javax.annotation.Generated;
import org.apache.commons.codec.binary.Hex;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.server.AbstractNonblockingServer.*;
@SuppressWarnings({ "cast", "rawtypes", "serial", "unchecked", "unused" })
/**
* A temporary token that is returned by the
* {@link ConcourseService#login(String, String)} method to grant access
* to secure resources in place of raw credentials.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.2)", date = "2015-2-22")
public class AccessToken implements
org.apache.thrift.TBase,
java.io.Serializable,
Cloneable,
Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct(
"AccessToken");
private static final org.apache.thrift.protocol.TField DATA_FIELD_DESC = new org.apache.thrift.protocol.TField(
"data", org.apache.thrift.protocol.TType.STRING, (short) 1);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class,
new AccessTokenStandardSchemeFactory());
schemes.put(TupleScheme.class, new AccessTokenTupleSchemeFactory());
}
public ByteBuffer data; // required
/**
* The set of fields this struct contains, along with convenience methods
* for finding and manipulating them.
*/
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
DATA((short) 1, "data");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not
* found.
*/
public static _Fields findByThriftId(int fieldId) {
switch (fieldId) {
case 1: // DATA
return DATA;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if(fields == null)
throw new IllegalArgumentException(
"Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not
* found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(
_Fields.class);
tmpMap.put(_Fields.DATA,
new org.apache.thrift.meta_data.FieldMetaData("data",
org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRING,
true)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData
.addStructMetaDataMap(AccessToken.class, metaDataMap);
}
public AccessToken() {}
public AccessToken(ByteBuffer data) {
this();
this.data = data;
}
/**
* Performs a deep copy on other.
*/
public AccessToken(AccessToken other) {
if(other.isSetData()) {
this.data = org.apache.thrift.TBaseHelper.copyBinary(other.data);
}
}
public AccessToken deepCopy() {
return new AccessToken(this);
}
@Override
public void clear() {
this.data = null;
}
public byte[] getData() {
setData(org.apache.thrift.TBaseHelper.rightSize(data));
return data == null ? null : data.array();
}
public ByteBuffer bufferForData() {
return data;
}
public AccessToken setData(byte[] data) {
setData(data == null ? (ByteBuffer) null : ByteBuffer.wrap(data));
return this;
}
public AccessToken setData(ByteBuffer data) {
this.data = data;
return this;
}
public void unsetData() {
this.data = null;
}
/**
* Returns true if field data is set (has been assigned a value) and false
* otherwise
*/
public boolean isSetData() {
return this.data != null;
}
public void setDataIsSet(boolean value) {
if(!value) {
this.data = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case DATA:
if(value == null) {
unsetData();
}
else {
setData((ByteBuffer) value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case DATA:
return getData();
}
throw new IllegalStateException();
}
/**
* Returns true if field corresponding to fieldID is set (has been assigned
* a value) and false otherwise
*/
public boolean isSet(_Fields field) {
if(field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case DATA:
return isSetData();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object obj) {
if(obj instanceof AccessToken) {
AccessToken other = (AccessToken) obj;
return Arrays.equals(getData(), other.getData());
}
return false;
}
@Override
public int hashCode() {
return Arrays.hashCode(getData());
}
@Override
public int compareTo(AccessToken other) {
if(!getClass().equals(other.getClass())) {
return getClass().getName().compareTo(other.getClass().getName());
}
int lastComparison = 0;
lastComparison = Boolean.valueOf(isSetData())
.compareTo(other.isSetData());
if(lastComparison != 0) {
return lastComparison;
}
if(isSetData()) {
lastComparison = org.apache.thrift.TBaseHelper.compareTo(this.data,
other.data);
if(lastComparison != 0) {
return lastComparison;
}
}
return 0;
}
public _Fields fieldForId(int fieldId) {
return _Fields.findByThriftId(fieldId);
}
public void read(org.apache.thrift.protocol.TProtocol iprot)
throws org.apache.thrift.TException {
schemes.get(iprot.getScheme()).getScheme().read(iprot, this);
}
public void write(org.apache.thrift.protocol.TProtocol oprot)
throws org.apache.thrift.TException {
schemes.get(oprot.getScheme()).getScheme().write(oprot, this);
}
@Override
public String toString() {
return Hex.encodeHexString(getData());
}
public void validate() throws org.apache.thrift.TException {
// check for required fields
if(data == null) {
throw new org.apache.thrift.protocol.TProtocolException(
"Required field 'data' was not present! Struct: "
+ toString());
}
// check for sub-struct validity
}
private void writeObject(java.io.ObjectOutputStream out)
throws java.io.IOException {
try {
write(new org.apache.thrift.protocol.TCompactProtocol(
new org.apache.thrift.transport.TIOStreamTransport(out)));
}
catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private void readObject(java.io.ObjectInputStream in)
throws java.io.IOException, ClassNotFoundException {
try {
read(new org.apache.thrift.protocol.TCompactProtocol(
new org.apache.thrift.transport.TIOStreamTransport(in)));
}
catch (org.apache.thrift.TException te) {
throw new java.io.IOException(te);
}
}
private static class AccessTokenStandardSchemeFactory
implements SchemeFactory {
public AccessTokenStandardScheme getScheme() {
return new AccessTokenStandardScheme();
}
}
private static class AccessTokenStandardScheme
extends StandardScheme {
public void read(org.apache.thrift.protocol.TProtocol iprot,
AccessToken struct) throws org.apache.thrift.TException {
org.apache.thrift.protocol.TField schemeField;
iprot.readStructBegin();
while (true) {
schemeField = iprot.readFieldBegin();
if(schemeField.type == org.apache.thrift.protocol.TType.STOP) {
break;
}
switch (schemeField.id) {
case 1: // DATA
if(schemeField.type == org.apache.thrift.protocol.TType.STRING) {
struct.data = iprot.readBinary();
struct.setDataIsSet(true);
}
else {
org.apache.thrift.protocol.TProtocolUtil.skip(iprot,
schemeField.type);
}
break;
default:
org.apache.thrift.protocol.TProtocolUtil.skip(iprot,
schemeField.type);
}
iprot.readFieldEnd();
}
iprot.readStructEnd();
// check for required fields of primitive type, which can't be
// checked in the validate method
struct.validate();
}
public void write(org.apache.thrift.protocol.TProtocol oprot,
AccessToken struct) throws org.apache.thrift.TException {
struct.validate();
oprot.writeStructBegin(STRUCT_DESC);
if(struct.data != null) {
oprot.writeFieldBegin(DATA_FIELD_DESC);
oprot.writeBinary(struct.data);
oprot.writeFieldEnd();
}
oprot.writeFieldStop();
oprot.writeStructEnd();
}
}
private static class AccessTokenTupleSchemeFactory
implements SchemeFactory {
public AccessTokenTupleScheme getScheme() {
return new AccessTokenTupleScheme();
}
}
private static class AccessTokenTupleScheme
extends TupleScheme {
@Override
public void write(org.apache.thrift.protocol.TProtocol prot,
AccessToken struct) throws org.apache.thrift.TException {
TTupleProtocol oprot = (TTupleProtocol) prot;
oprot.writeBinary(struct.data);
}
@Override
public void read(org.apache.thrift.protocol.TProtocol prot,
AccessToken struct) throws org.apache.thrift.TException {
TTupleProtocol iprot = (TTupleProtocol) prot;
struct.data = iprot.readBinary();
struct.setDataIsSet(true);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy