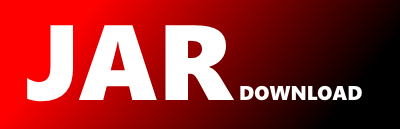
com.cinchapi.concourse.thrift.ComplexTObject Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of concourse-driver-java Show documentation
Show all versions of concourse-driver-java Show documentation
Concourse is a self-tuning database that is designed for both ad hoc analytics and high volume transactions at scale. Developers use Concourse to quickly build mission critical software while also benefiting from real time insight into their most important data. With Concourse, end-to-end data management requires no extra infrastructure, no prior configuration and no additional coding–all of which greatly reduce costs and allow developers to focus on core business problems.
/*
* Copyright (c) 2013-2019 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.concourse.thrift;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.BitSet;
import java.util.Collection;
import java.util.Collections;
import java.util.EnumMap;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.HashSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import javax.annotation.Generated;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import com.cinchapi.common.base.CheckedExceptions;
import com.cinchapi.concourse.util.ByteBuffers;
import com.cinchapi.concourse.util.Convert;
import com.google.common.base.MoreObjects;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
@SuppressWarnings({ "cast", "rawtypes", "serial", "unchecked" })
/**
* A recursive structure that encodes one or more {@link TObject TObjects}.
*
*
* The most basic {@link ComplexTObject} is a
* {@link ComplexTObjectType#SCALAR scalar}, which is just a wrapped
* {@link TObject}. Beyond that, complex collections can be represented as a
* {@link Set}, {@link List} or {@link Map} of
* {@link ComplexTObject ComplexTObjects}.
*
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.3)", date = "2016-09-19")
public class ComplexTObject implements
org.apache.thrift.TBase,
java.io.Serializable,
Cloneable,
Comparable {
/**
* Deserialize a {@link ComplexTObject} by reading its binary form from the
* {@code buffer}.
*
* @param buffer a {@link ByteBuffer} with the serialized content of a
* {@link ComplexTObject}
* @return the ComplexTObject
*/
public static ComplexTObject fromByteBuffer(ByteBuffer buffer) {
ComplexTObjectType type = ComplexTObjectType.values()[buffer.get()];
ComplexTObject obj = new ComplexTObject();
obj.type = type;
if(type == ComplexTObjectType.MAP) {
obj.tmap = Maps.newLinkedHashMap();
while (buffer.hasRemaining()) {
int keyLength = buffer.getInt();
ComplexTObject key = ComplexTObject
.fromByteBuffer(ByteBuffers.get(buffer, keyLength));
int valueLength = buffer.getInt();
ComplexTObject value = ComplexTObject
.fromByteBuffer(ByteBuffers.get(buffer, valueLength));
obj.tmap.put(key, value);
}
}
else if(type == ComplexTObjectType.LIST
|| type == ComplexTObjectType.SET) {
Collection collection = type == ComplexTObjectType.LIST
? (obj.tlist = Lists.newArrayList())
: (obj.tset = Sets.newLinkedHashSet());
while (buffer.hasRemaining()) {
int length = buffer.getInt();
ComplexTObject item = ComplexTObject
.fromByteBuffer(ByteBuffers.get(buffer, length));
collection.add(item);
}
}
else if(type == ComplexTObjectType.TCRITERIA) {
List symbols = Lists.newArrayList();
while (buffer.hasRemaining()) {
int length = buffer.getInt();
TSymbolType symbolType = TSymbolType.values()[buffer.get()];
String symbol = ByteBuffers
.getString(ByteBuffers.get(buffer, length));
symbols.add(new TSymbol(symbolType, symbol));
}
obj.tcriteria = new TCriteria(symbols);
}
else if(type == ComplexTObjectType.BINARY) {
obj.tbinary = ByteBuffers.get(buffer, buffer.remaining());
}
else {
Type ttype = Type.values()[buffer.get()];
TObject ref = new TObject(ByteBuffers.getRemaining(buffer), ttype);
if(type == ComplexTObjectType.SCALAR) {
obj.tscalar = ref;
}
else {
obj.tobject = ref;
}
}
return obj;
}
/**
* Create a new {@link ComplexTObject} from the specified java
* {@code object}.
* The original object can be retrieved using the {@link #getJavaObject()}
* method.
*
* @param object the object to wrap within the {@link ComplexTObject}.
* @return the ComplexTObject
*/
public static ComplexTObject fromJavaObject(T object) {
ComplexTObject complex = new ComplexTObject();
if(object instanceof Map) {
Map, ?> map = (Map, ?>) object;
complex.setType(ComplexTObjectType.MAP);
Map tmap = Maps.newLinkedHashMap();
for (Entry, ?> entry : map.entrySet()) {
tmap.put(fromJavaObject(entry.getKey()),
fromJavaObject(entry.getValue()));
}
complex.setTmap(tmap);
}
else if(object instanceof List) {
List> list = (List>) object;
complex.setType(ComplexTObjectType.LIST);
List tlist = Lists.newArrayList();
for (Object elt : list) {
tlist.add(fromJavaObject(elt));
}
complex.setTlist(tlist);
}
else if(object instanceof Set) {
Set> set = (Set>) object;
complex.setType(ComplexTObjectType.SET);
Set tset = Sets.newLinkedHashSet();
for (Object elt : set) {
tset.add(fromJavaObject(elt));
}
complex.setTset(tset);
}
else if(object instanceof TObject) {
complex.setType(ComplexTObjectType.TOBJECT);
complex.setTobject((TObject) object);
}
else if(object instanceof TCriteria) {
complex.setType(ComplexTObjectType.TCRITERIA);
complex.setTcriteria((TCriteria) object);
}
else if(object instanceof byte[] || object instanceof ByteBuffer) {
complex.setType(ComplexTObjectType.BINARY);
if(object instanceof ByteBuffer) {
complex.setTbinary((ByteBuffer) object);
}
else {
complex.setTbinary(ByteBuffer.wrap((byte[]) object));
}
}
else {
complex.setType(ComplexTObjectType.SCALAR);
complex.setTscalar(Convert.javaToThrift(object));
}
return complex;
}
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct(
"ComplexTObject");
private static final org.apache.thrift.protocol.TField TYPE_FIELD_DESC = new org.apache.thrift.protocol.TField(
"type", org.apache.thrift.protocol.TType.I32, (short) 1);
private static final org.apache.thrift.protocol.TField TSCALAR_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tscalar", org.apache.thrift.protocol.TType.STRUCT, (short) 2);
private static final org.apache.thrift.protocol.TField TMAP_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tmap", org.apache.thrift.protocol.TType.MAP, (short) 3);
private static final org.apache.thrift.protocol.TField TLIST_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tlist", org.apache.thrift.protocol.TType.LIST, (short) 4);
private static final org.apache.thrift.protocol.TField TSET_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tset", org.apache.thrift.protocol.TType.SET, (short) 5);
private static final org.apache.thrift.protocol.TField TOBJECT_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tobject", org.apache.thrift.protocol.TType.STRUCT, (short) 6);
private static final org.apache.thrift.protocol.TField TCRITERIA_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tcriteria", org.apache.thrift.protocol.TType.STRUCT, (short) 7);
private static final org.apache.thrift.protocol.TField TBINARY_FIELD_DESC = new org.apache.thrift.protocol.TField(
"tbinary", org.apache.thrift.protocol.TType.STRING, (short) 8);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class,
new ComplexTObjectStandardSchemeFactory());
schemes.put(TupleScheme.class, new ComplexTObjectTupleSchemeFactory());
}
/**
*
* @see ComplexTObjectType
*/
public ComplexTObjectType type; // required
public com.cinchapi.concourse.thrift.TObject tscalar; // optional
public Map tmap; // optional
public List tlist; // optional
public Set tset; // optional
public com.cinchapi.concourse.thrift.TObject tobject; // optional
public com.cinchapi.concourse.thrift.TCriteria tcriteria; // optional
public ByteBuffer tbinary; // optional
private transient Object cached;
/**
* The set of fields this struct contains, along with convenience methods
* for finding and manipulating them.
*/
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
/**
*
* @see ComplexTObjectType
*/
TYPE((short) 1, "type"), TSCALAR((short) 2, "tscalar"), TMAP((short) 3, "tmap"), TLIST((short) 4, "tlist"), TSET((short) 5, "tset"), TOBJECT((short) 6, "tobject"), TCRITERIA((short) 7, "tcriteria"), TBINARY((short) 8, "tbinary");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not
* found.
*/
public static _Fields findByThriftId(int fieldId) {
switch (fieldId) {
case 1: // TYPE
return TYPE;
case 2: // TSCALAR
return TSCALAR;
case 3: // TMAP
return TMAP;
case 4: // TLIST
return TLIST;
case 5: // TSET
return TSET;
case 6: // TOBJECT
return TOBJECT;
case 7: // TCRITERIA
return TCRITERIA;
case 8: // TBINARY
return TBINARY;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if(fields == null)
throw new IllegalArgumentException(
"Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not
* found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
@SuppressWarnings("unused")
private static final _Fields optionals[] = { _Fields.TSCALAR, _Fields.TMAP,
_Fields.TLIST, _Fields.TSET, _Fields.TOBJECT, _Fields.TCRITERIA,
_Fields.TBINARY };
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(
_Fields.class);
tmpMap.put(_Fields.TYPE,
new org.apache.thrift.meta_data.FieldMetaData("type",
org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.EnumMetaData(
org.apache.thrift.protocol.TType.ENUM,
ComplexTObjectType.class)));
tmpMap.put(_Fields.TSCALAR,
new org.apache.thrift.meta_data.FieldMetaData("tscalar",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(
org.apache.thrift.protocol.TType.STRUCT,
com.cinchapi.concourse.thrift.TObject.class)));
tmpMap.put(_Fields.TMAP,
new org.apache.thrift.meta_data.FieldMetaData("tmap",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.MapMetaData(
org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRUCT,
"ComplexTObject"),
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRUCT,
"ComplexTObject"))));
tmpMap.put(_Fields.TLIST,
new org.apache.thrift.meta_data.FieldMetaData("tlist",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.ListMetaData(
org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRUCT,
"ComplexTObject"))));
tmpMap.put(_Fields.TSET,
new org.apache.thrift.meta_data.FieldMetaData("tset",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.SetMetaData(
org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRUCT,
"ComplexTObject"))));
tmpMap.put(_Fields.TOBJECT,
new org.apache.thrift.meta_data.FieldMetaData("tobject",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(
org.apache.thrift.protocol.TType.STRUCT,
com.cinchapi.concourse.thrift.TObject.class)));
tmpMap.put(_Fields.TCRITERIA,
new org.apache.thrift.meta_data.FieldMetaData("tcriteria",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.StructMetaData(
org.apache.thrift.protocol.TType.STRUCT,
com.cinchapi.concourse.thrift.TCriteria.class)));
tmpMap.put(_Fields.TBINARY,
new org.apache.thrift.meta_data.FieldMetaData("tbinary",
org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRING,
true)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData
.addStructMetaDataMap(ComplexTObject.class, metaDataMap);
}
public ComplexTObject() {}
public ComplexTObject(ComplexTObjectType type) {
this();
this.type = type;
}
/**
* Performs a deep copy on other.
*/
public ComplexTObject(ComplexTObject other) {
if(other.isSetType()) {
this.type = other.type;
}
if(other.isSetTscalar()) {
this.tscalar = new com.cinchapi.concourse.thrift.TObject(
other.tscalar);
}
if(other.isSetTmap()) {
Map __this__tmap = new LinkedHashMap(
other.tmap.size());
for (Map.Entry other_element : other.tmap
.entrySet()) {
ComplexTObject other_element_key = other_element.getKey();
ComplexTObject other_element_value = other_element.getValue();
ComplexTObject __this__tmap_copy_key = other_element_key;
ComplexTObject __this__tmap_copy_value = other_element_value;
__this__tmap.put(__this__tmap_copy_key,
__this__tmap_copy_value);
}
this.tmap = __this__tmap;
}
if(other.isSetTlist()) {
List __this__tlist = new ArrayList(
other.tlist.size());
for (ComplexTObject other_element : other.tlist) {
__this__tlist.add(other_element);
}
this.tlist = __this__tlist;
}
if(other.isSetTset()) {
Set __this__tset = new HashSet(
other.tset.size());
for (ComplexTObject other_element : other.tset) {
__this__tset.add(other_element);
}
this.tset = __this__tset;
}
if(other.isSetTobject()) {
this.tobject = new com.cinchapi.concourse.thrift.TObject(
other.tobject);
}
if(other.isSetTcriteria()) {
this.tcriteria = new com.cinchapi.concourse.thrift.TCriteria(
other.tcriteria);
}
if(other.isSetTbinary()) {
this.tbinary = org.apache.thrift.TBaseHelper
.copyBinary(other.tbinary);
}
}
/**
* Return a {@link ByteBuffer} that contains a serialized representation of
* this {@link ComplexTObject}.
*
* @return the serialized form in a {@link ByteBuffer}
*/
public ByteBuffer toByteBuffer() {
ByteArrayOutputStream bytes = new ByteArrayOutputStream(); // TODO: use
// something
// else
// because
// ByteArrayOutputStream
// unnecessarily
// uses
// synchronization
ByteBuffer size = ByteBuffer.allocate(4);
bytes.write(type.ordinal());
if(type == ComplexTObjectType.MAP) {
tmap.entrySet().forEach(entry -> {
try {
byte[] key = entry.getKey().toByteBuffer().array();
bytes.write(
(byte[]) size.putInt(key.length).flip().array());
bytes.write(key);
byte[] value = entry.getValue().toByteBuffer().array();
bytes.write(
(byte[]) size.putInt(value.length).flip().array());
bytes.write(value);
}
catch (IOException e) {
throw CheckedExceptions.wrapAsRuntimeException(e);
}
});
}
else if(type == ComplexTObjectType.LIST
|| type == ComplexTObjectType.SET) {
Collection collection = type == ComplexTObjectType.LIST
? tlist : tset;
collection.forEach((item) -> {
try {
byte[] itemBytes = item.toByteBuffer().array();
bytes.write((byte[]) size.putInt(itemBytes.length).flip()
.array());
bytes.write(itemBytes);
}
catch (IOException e) {
throw CheckedExceptions.wrapAsRuntimeException(e);
}
});
}
else if(type == ComplexTObjectType.TCRITERIA) {
tcriteria.symbols.forEach((item) -> {
try {
byte[] symbolBytes = item.symbol
.getBytes(StandardCharsets.UTF_8);
int length = symbolBytes.length;
bytes.write((byte[]) size.putInt(length).flip().array());
bytes.write(item.type.ordinal());
bytes.write(symbolBytes);
}
catch (IOException e) {
throw CheckedExceptions.wrapAsRuntimeException(e);
}
});
}
else if(type == ComplexTObjectType.BINARY) {
try {
bytes.write(tbinary.array());
}
catch (IOException e) {
throw CheckedExceptions.wrapAsRuntimeException(e);
}
}
else {
TObject obj = MoreObjects.firstNonNull(tobject, tscalar);
try {
bytes.write(obj.type.ordinal());
bytes.write(obj.getData());
}
catch (IOException e) {
throw CheckedExceptions.wrapAsRuntimeException(e);
}
}
return ByteBuffer.wrap(bytes.toByteArray());
}
/**
* Return the canonical java object that is wrapped within this
* {@link ComplexTObject}.
*
* @return the wrapped java object
*/
public T getJavaObject() {
if(cached == null) {
if(type == ComplexTObjectType.MAP) {
Map tmap = getTmap();
Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy