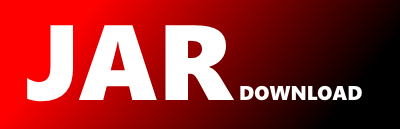
com.cinchapi.concourse.thrift.InvalidArgumentException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of concourse-driver-java Show documentation
Show all versions of concourse-driver-java Show documentation
Concourse is a self-tuning database that is designed for both ad hoc analytics and high volume transactions at scale. Developers use Concourse to quickly build mission critical software while also benefiting from real time insight into their most important data. With Concourse, end-to-end data management requires no extra infrastructure, no prior configuration and no additional coding–all of which greatly reduce costs and allow developers to focus on core business problems.
/*
* Copyright (c) 2013-2019 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.concourse.thrift;
import java.util.ArrayList;
import java.util.BitSet;
import java.util.Collections;
import java.util.EnumMap;
import java.util.EnumSet;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.annotation.Generated;
import org.apache.thrift.TException;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.server.AbstractNonblockingServer.*;
@SuppressWarnings({ "cast", "rawtypes", "serial", "unchecked", "unused" })
/**
* Signals that an invalid argument was submitted.
*/
@Generated(value = "Autogenerated by Thrift Compiler (0.9.2)", date = "2015-9-30")
public class InvalidArgumentException extends TException implements
org.apache.thrift.TBase,
java.io.Serializable,
Cloneable,
Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct(
"InvalidArgumentException");
private static final org.apache.thrift.protocol.TField MESSAGE_FIELD_DESC = new org.apache.thrift.protocol.TField(
"message", org.apache.thrift.protocol.TType.STRING, (short) 1);
private static final Map, SchemeFactory> schemes = new HashMap, SchemeFactory>();
static {
schemes.put(StandardScheme.class,
new InvalidArgumentExceptionStandardSchemeFactory());
schemes.put(TupleScheme.class,
new InvalidArgumentExceptionTupleSchemeFactory());
}
public String message; // required
/**
* The set of fields this struct contains, along with convenience methods
* for finding and manipulating them.
*/
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
MESSAGE((short) 1, "message");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not
* found.
*/
public static _Fields findByThriftId(int fieldId) {
switch (fieldId) {
case 1: // MESSAGE
return MESSAGE;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if(fields == null)
throw new IllegalArgumentException(
"Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not
* found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(
_Fields.class);
tmpMap.put(_Fields.MESSAGE,
new org.apache.thrift.meta_data.FieldMetaData("message",
org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(
org.apache.thrift.protocol.TType.STRING)));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(
InvalidArgumentException.class, metaDataMap);
}
public InvalidArgumentException() {}
public InvalidArgumentException(String message) {
this();
this.message = message;
}
/**
* Performs a deep copy on other.
*/
public InvalidArgumentException(InvalidArgumentException other) {
if(other.isSetMessage()) {
this.message = other.message;
}
}
public InvalidArgumentException deepCopy() {
return new InvalidArgumentException(this);
}
@Override
public void clear() {
this.message = null;
}
public String getMessage() {
return this.message;
}
public InvalidArgumentException setMessage(String message) {
this.message = message;
return this;
}
public void unsetMessage() {
this.message = null;
}
/**
* Returns true if field message is set (has been assigned a value) and
* false otherwise
*/
public boolean isSetMessage() {
return this.message != null;
}
public void setMessageIsSet(boolean value) {
if(!value) {
this.message = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case MESSAGE:
if(value == null) {
unsetMessage();
}
else {
setMessage((String) value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case MESSAGE:
return getMessage();
}
throw new IllegalStateException();
}
/**
* Returns true if field corresponding to fieldID is set (has been assigned
* a value) and false otherwise
*/
public boolean isSet(_Fields field) {
if(field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case MESSAGE:
return isSetMessage();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if(that == null)
return false;
if(that instanceof InvalidArgumentException)
return this.equals((InvalidArgumentException) that);
return false;
}
public boolean equals(InvalidArgumentException that) {
if(that == null)
return false;
boolean this_present_message = true && this.isSetMessage();
boolean that_present_message = true && that.isSetMessage();
if(this_present_message || that_present_message) {
if(!(this_present_message && that_present_message))
return false;
if(!this.message.equals(that.message))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy