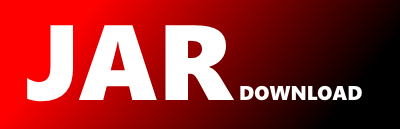
com.cinchapi.concourse.util.PrettyLinkedTableMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of concourse-driver-java Show documentation
Show all versions of concourse-driver-java Show documentation
Concourse is a self-tuning database that is designed for both ad hoc analytics and high volume transactions at scale. Developers use Concourse to quickly build mission critical software while also benefiting from real time insight into their most important data. With Concourse, end-to-end data management requires no extra infrastructure, no prior configuration and no additional coding–all of which greatly reduce costs and allow developers to focus on core business problems.
/*
* Copyright (c) 2013-2019 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.concourse.util;
import java.util.LinkedHashMap;
import java.util.Map;
import javax.annotation.Nullable;
import com.google.common.base.Strings;
import com.google.common.collect.Maps;
/**
* A special {@link LinkedHashMap} that simulates a sparse table where a row key
* maps to a set of columns and each column maps to a value (e.g. Map[R, ->
* Map[C -> V]]). This object has pretty {@link #toString()} output that is
* formatted as such:
*
*
* +-----------------------------------------------------------+
* | Record | name | gender | joinedBy |
* +-----------------------------------------------------------+
* | 1416242356271000 | Deary Hudson | UNSPECIFIED | EMAIL |
* | 1416242356407000 | Jeff Nelson | UNSPECIFIED | EMAIL |
* | 1416242356436000 | Morgan Debaun | UNSPECIFIED | EMAIL |
* +-----------------------------------------------------------+
*
*
* A {@link PrettyLinkedTableMap} is suitable for displaying information about
* multiple records or documents.
*
*
* @author Jeff Nelson
*/
@SuppressWarnings("serial")
public class PrettyLinkedTableMap extends LinkedHashMap> {
/**
* The minimum column length. Is set equal to the size of the string "null"
* for consistent spacing when values don't exist.
*/
private static int MIN_COLUMN_LENGTH = "null".length();
/**
* Return an empty {@link PrettyLinkedTableMap} with the default row name.
*
* @return the PrettyLinkedTableMap
*/
public static PrettyLinkedTableMap newPrettyLinkedTableMap() {
return new PrettyLinkedTableMap(null);
}
/**
* Return an empty {@link PrettyLinkedTableMap} with the specified
* {@code rowName}.
*
* @param rowName
* @return the PrettyLinkedTableMap
*/
public static PrettyLinkedTableMap newPrettyLinkedTableMap(
String rowName) {
return new PrettyLinkedTableMap(rowName);
}
private String rowName = "Row";
private int rowLength = rowName.length();
/**
* A mapping from column to display length, which is equal to the greater of
* the length of the largest value that will be displayed in the column and
* the value of the column's toString() value.
*/
private final Map columns = Maps.newLinkedHashMap();
/**
* Construct a new instance.
*
* @param rowName
*/
private PrettyLinkedTableMap(@Nullable String rowName) {
if(!Strings.isNullOrEmpty(rowName)) {
setRowName(rowName);
}
}
/**
* Insert {@code value} under {@code column} in {@code row}.
*
* @param row
* @param column
* @param value
* @return the previous value located at the intersection of {@code row} and
* {@code column} or {@code null} if one did not previously exist.
*/
public V put(R row, C column, V value) {
Map rowdata = super.get(row);
if(rowdata == null) {
rowdata = Maps.newLinkedHashMap();
super.put(row, rowdata);
}
rowLength = Math.max(row.toString().length(), rowLength);
int current = columns.containsKey(column) ? columns.get(column)
: MIN_COLUMN_LENGTH;
columns.put(column, Math.max(current, Math
.max(column.toString().length(), value.toString().length())));
return rowdata.put(column, value);
}
/**
*
* THIS METHOD ALWAYS RETURNS {@code NULL}.
*
* {@inheritDoc}
*/
@Override
public Map put(R key, Map value) {
for (Map.Entry entry : value.entrySet()) {
put(key, entry.getKey(), entry.getValue());
}
return null;
}
/**
* Set the rowName to {@code name}.
*
* @param name
* @return this
*/
public PrettyLinkedTableMap setRowName(String name) {
rowName = name;
rowLength = Math.max(name.length(), rowLength);
return this;
}
@Override
public String toString() {
int total = 0;
Object[] header = new Object[columns.size() + 1];
header[0] = rowName;
String format = "| %-" + rowLength + "s | ";
int i = 1;
for (java.util.Map.Entry entry : columns.entrySet()) {
format += "%-" + entry.getValue() + "s | ";
total += entry.getValue() + 3;
header[i] = entry.getKey();
++i;
}
format += "%n";
String hr = Strings.padEnd("+", rowLength + 3 + total, '-');
hr += "+" + System.getProperty("line.separator");
StringBuilder sb = new StringBuilder();
sb.append(System.getProperty("line.separator"));
sb.append(hr);
sb.append(String.format(format, header));
sb.append(hr);
for (R row : keySet()) {
Object[] rowdata = new Object[columns.size() + 1];
rowdata[0] = row;
i = 1;
for (C column : columns.keySet()) {
rowdata[i] = get(row).get(column);
++i;
}
sb.append(String.format(format, rowdata));
}
sb.append(hr);
return sb.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy