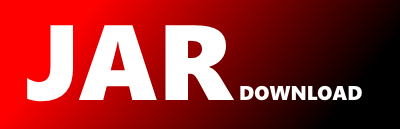
com.cinchapi.concourse.export.Exporter Maven / Gradle / Ivy
/*
* Copyright (c) 2013-2022 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.concourse.export;
import java.io.OutputStream;
import java.io.PrintStream;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import javax.annotation.concurrent.Immutable;
import com.cinchapi.common.base.AnyStrings;
import com.cinchapi.common.collect.Sequences;
import com.google.common.collect.Sets;
/**
* An {@link Exporter} can push objects (represented as a Map} to an
* {@link OutputStream}.
*
* @author Jeff Nelson
*/
@Immutable
public abstract class Exporter {
/**
* Return the union of all keys within the {@code data} as an
* {@link Iterable} that make up the headers for the data.
*
* @param data
* @return the headers
*/
protected static Iterable getHeaders(
Iterable
© 2015 - 2024 Weber Informatics LLC | Privacy Policy