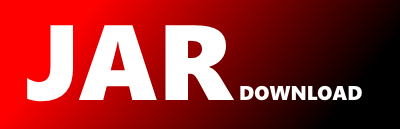
com.cinchapi.impromptu.server.api.automate.Action Maven / Gradle / Ivy
/*
* Cinchapi Inc. CONFIDENTIAL
* Copyright (c) 2017 Cinchapi Inc. All Rights Reserved.
*
* All information contained herein is, and remains the property of Cinchapi.
* The intellectual and technical concepts contained herein are proprietary to
* Cinchapi and may be covered by U.S. and Foreign Patents, patents in process,
* and are protected by trade secret or copyright law. Dissemination of this
* information or reproduction of this material is strictly forbidden unless
* prior written permission is obtained from Cinchapi. Access to the source code
* contained herein is hereby forbidden to anyone except current Cinchapi
* employees, managers or contractors who have executed Confidentiality and
* Non-disclosure agreements explicitly covering such access.
*
* The copyright notice above does not evidence any actual or intended
* publication or disclosure of this source code, which includes information
* that is confidential and/or proprietary, and is a trade secret, of Cinchapi.
*
* ANY REPRODUCTION, MODIFICATION, DISTRIBUTION, PUBLIC PERFORMANCE, OR PUBLIC
* DISPLAY OF OR THROUGH USE OF THIS SOURCE CODE WITHOUT THE EXPRESS WRITTEN
* CONSENT OF COMPANY IS STRICTLY PROHIBITED, AND IN VIOLATION OF APPLICABLE
* LAWS AND INTERNATIONAL TREATIES. THE RECEIPT OR POSSESSION OF THIS SOURCE
* CODE AND/OR RELATED INFORMATION DOES NOT CONVEY OR IMPLY ANY RIGHTS TO
* REPRODUCE, DISCLOSE OR DISTRIBUTE ITS CONTENTS, OR TO MANUFACTURE, USE, OR
* SELL ANYTHING THAT IT MAY DESCRIBE, IN WHOLE OR IN PART.
*/
package com.cinchapi.impromptu.server.api.automate;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Map;
import com.cinchapi.common.reflect.Reflection;
import com.google.common.collect.Maps;
/**
* A {@link Action} is a workflow component that responds to a data change
* event.
*
* The instance variables defined in a {@link Action} class are automatically
* sent
* to the client to be supplied by a user. When a {@link Action} is created with
* the supplied params, the variables are automatically populated with the
* values.
*
*
* By default, the assumption is that all the variables defined in the class are
* params that need to be defined by the user (even private variables). If a
* user is only needed for internal use, it can be marked as {@code transient}
* and it won't be included in the task serialization.
*
*
* @author Jeff Nelson
*/
public abstract class Action {
/**
* Every {@link Action} should be created reflectively using the
* {@link Actions#create(Map)} method.
*/
protected Action() {}
/**
* Execute the {@link Action} and returns a boolean that indicates whether
* the task has succeeded or failed.
*
* @return a boolean that indicates whether the task has succeeded or failed
*/
public abstract boolean exec();
/**
* Return the params that were provided by the user when this {@link Action}
* was created.
*
* @return the user provided params
*/
public final Map params() {
Map params = Maps.newHashMap();
for (Field field : Reflection.getAllDeclaredFields(this.getClass())) {
if(Modifier.isTransient(field.getModifiers())) {
continue;
}
String key = field.getName();
Object value = Reflection.get(field.getName(), this);
params.put(key, value);
}
return params;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy