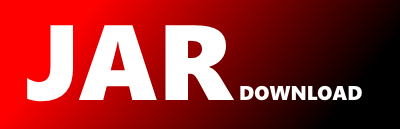
com.cinchapi.impromptu.server.util.MappedCollections Maven / Gradle / Ivy
/*
* Copyright (c) 2013-2017 Cinchapi Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.cinchapi.impromptu.server.util;
import java.util.Collection;
import java.util.Iterator;
import java.util.Map;
/**
* Utility functions for {@link Map Maps} that contain {@link Collection
* Collections} for values.
*
* @author Jeff Nelson
*/
public final class MappedCollections {
/**
* Return the first item in the {@link Collection} mapped from the
* {@code key} within the {@code data}.
*
* @param data the dataset
* @param key the lookup key
* @return the first item in the collection mapped from the {@code key} or
* {@code null} if there is no mapping from {@code key}.
*/
public static V getFirst(Map> data, K key) {
Collection values = data.get(key);
if(values == null || values.isEmpty()) {
return null;
}
else {
return getIterableFirstOrDefault(values, null);
}
}
/**
* Return the first item in the {@link Collection} mapped from the
* {@code key} within the {@code data} or {@code theDefault} value if no
* mapping from {@code key} exists.
*
* @param data the dataset
* @param key the lookup key
* @return the first item in the collection mapped from the {@code key} or
* {@code theDefault} if there is no mapping from {@code key}.
*/
public static V getFirstOrDefault(Map> data, K key,
V theDefault) {
Collection values = data.get(key);
if(values == null || values.isEmpty()) {
return theDefault;
}
else {
return getIterableFirstOrDefault(values, theDefault);
}
}
/**
* Return the first item in the {@code iterable} or {@code theDefault} if
* the {@code iterable} is empty.
*
* @param iterable the iterable from which the first item is returned
* @param theDefault the default value to return if the iterable is empty
* @return the first or default item
*/
private static T getIterableFirstOrDefault(Iterable iterable,
T theDefault) {
Iterator it = iterable.iterator();
if(it.hasNext()) {
return it.next();
}
else {
return theDefault;
}
}
private MappedCollections() {/* no-op */}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy