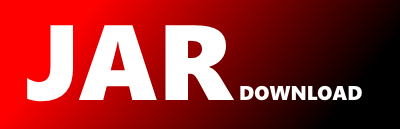
com.circleci.client.v2.model.JobDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Client for CircleCI's API v2
/*
* CircleCI API
* This describes the resources that make up the CircleCI API v2. API v2 is currently in Preview. Additional documentation for this API can be found in the [API Preview Docs](https://github.com/CircleCI-Public/api-preview-docs/tree/master/docs). Breaking changes to the API will be announced in the [Breaking Changes log](https://github.com/CircleCI-Public/api-preview-docs/blob/master/docs/breaking.md).
*
* The version of the OpenAPI document: v2
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.circleci.client.v2.model;
import java.util.Objects;
import java.util.Arrays;
import com.circleci.client.v2.model.JobDetailsContexts;
import com.circleci.client.v2.model.JobDetailsExecutor;
import com.circleci.client.v2.model.JobDetailsLatestWorkflow;
import com.circleci.client.v2.model.JobDetailsMessages;
import com.circleci.client.v2.model.JobDetailsOrganization;
import com.circleci.client.v2.model.JobDetailsParallelRuns;
import com.circleci.client.v2.model.JobDetailsPipeline;
import com.circleci.client.v2.model.JobDetailsProject;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
/**
* Job Details
*/
@ApiModel(description = "Job Details")
public class JobDetails {
public static final String JSON_PROPERTY_WEB_URL = "web_url";
@JsonProperty(JSON_PROPERTY_WEB_URL)
private String webUrl;
public static final String JSON_PROPERTY_PROJECT = "project";
@JsonProperty(JSON_PROPERTY_PROJECT)
private JobDetailsProject project = null;
public static final String JSON_PROPERTY_PARALLEL_RUNS = "parallel_runs";
@JsonProperty(JSON_PROPERTY_PARALLEL_RUNS)
private List parallelRuns = new ArrayList<>();
public static final String JSON_PROPERTY_STARTED_AT = "started_at";
@JsonProperty(JSON_PROPERTY_STARTED_AT)
private OffsetDateTime startedAt;
public static final String JSON_PROPERTY_LATEST_WORKFLOW = "latest_workflow";
@JsonProperty(JSON_PROPERTY_LATEST_WORKFLOW)
private JobDetailsLatestWorkflow latestWorkflow = null;
public static final String JSON_PROPERTY_NAME = "name";
@JsonProperty(JSON_PROPERTY_NAME)
private String name;
public static final String JSON_PROPERTY_EXECUTOR = "executor";
@JsonProperty(JSON_PROPERTY_EXECUTOR)
private JobDetailsExecutor executor = null;
public static final String JSON_PROPERTY_PARALLELISM = "parallelism";
@JsonProperty(JSON_PROPERTY_PARALLELISM)
private Long parallelism;
public static final String JSON_PROPERTY_STATUS = "status";
@JsonProperty(JSON_PROPERTY_STATUS)
private Object status = null;
public static final String JSON_PROPERTY_NUMBER = "number";
@JsonProperty(JSON_PROPERTY_NUMBER)
private Long number;
public static final String JSON_PROPERTY_PIPELINE = "pipeline";
@JsonProperty(JSON_PROPERTY_PIPELINE)
private JobDetailsPipeline pipeline = null;
public static final String JSON_PROPERTY_DURATION = "duration";
@JsonProperty(JSON_PROPERTY_DURATION)
private Long duration;
public static final String JSON_PROPERTY_CREATED_AT = "created_at";
@JsonProperty(JSON_PROPERTY_CREATED_AT)
private OffsetDateTime createdAt;
public static final String JSON_PROPERTY_MESSAGES = "messages";
@JsonProperty(JSON_PROPERTY_MESSAGES)
private List messages = new ArrayList<>();
public static final String JSON_PROPERTY_CONTEXTS = "contexts";
@JsonProperty(JSON_PROPERTY_CONTEXTS)
private List contexts = new ArrayList<>();
public static final String JSON_PROPERTY_ORGANIZATION = "organization";
@JsonProperty(JSON_PROPERTY_ORGANIZATION)
private JobDetailsOrganization organization = null;
public static final String JSON_PROPERTY_QUEUED_AT = "queued_at";
@JsonProperty(JSON_PROPERTY_QUEUED_AT)
private OffsetDateTime queuedAt;
public static final String JSON_PROPERTY_STOPPED_AT = "stopped_at";
@JsonProperty(JSON_PROPERTY_STOPPED_AT)
private OffsetDateTime stoppedAt;
public JobDetails webUrl(String webUrl) {
this.webUrl = webUrl;
return this;
}
/**
* URL of the job in CircleCI Web UI.
* @return webUrl
**/
@ApiModelProperty(required = true, value = "URL of the job in CircleCI Web UI.")
public String getWebUrl() {
return webUrl;
}
public void setWebUrl(String webUrl) {
this.webUrl = webUrl;
}
public JobDetails project(JobDetailsProject project) {
this.project = project;
return this;
}
/**
* Get project
* @return project
**/
@ApiModelProperty(required = true, value = "")
public JobDetailsProject getProject() {
return project;
}
public void setProject(JobDetailsProject project) {
this.project = project;
}
public JobDetails parallelRuns(List parallelRuns) {
this.parallelRuns = parallelRuns;
return this;
}
public JobDetails addParallelRunsItem(JobDetailsParallelRuns parallelRunsItem) {
this.parallelRuns.add(parallelRunsItem);
return this;
}
/**
* Info about parallels runs and their status.
* @return parallelRuns
**/
@ApiModelProperty(required = true, value = "Info about parallels runs and their status.")
public List getParallelRuns() {
return parallelRuns;
}
public void setParallelRuns(List parallelRuns) {
this.parallelRuns = parallelRuns;
}
public JobDetails startedAt(OffsetDateTime startedAt) {
this.startedAt = startedAt;
return this;
}
/**
* The date and time the job started.
* @return startedAt
**/
@ApiModelProperty(required = true, value = "The date and time the job started.")
public OffsetDateTime getStartedAt() {
return startedAt;
}
public void setStartedAt(OffsetDateTime startedAt) {
this.startedAt = startedAt;
}
public JobDetails latestWorkflow(JobDetailsLatestWorkflow latestWorkflow) {
this.latestWorkflow = latestWorkflow;
return this;
}
/**
* Get latestWorkflow
* @return latestWorkflow
**/
@ApiModelProperty(required = true, value = "")
public JobDetailsLatestWorkflow getLatestWorkflow() {
return latestWorkflow;
}
public void setLatestWorkflow(JobDetailsLatestWorkflow latestWorkflow) {
this.latestWorkflow = latestWorkflow;
}
public JobDetails name(String name) {
this.name = name;
return this;
}
/**
* The name of the job.
* @return name
**/
@ApiModelProperty(required = true, value = "The name of the job.")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public JobDetails executor(JobDetailsExecutor executor) {
this.executor = executor;
return this;
}
/**
* Get executor
* @return executor
**/
@ApiModelProperty(required = true, value = "")
public JobDetailsExecutor getExecutor() {
return executor;
}
public void setExecutor(JobDetailsExecutor executor) {
this.executor = executor;
}
public JobDetails parallelism(Long parallelism) {
this.parallelism = parallelism;
return this;
}
/**
* A number of parallel runs the job has.
* @return parallelism
**/
@ApiModelProperty(required = true, value = "A number of parallel runs the job has.")
public Long getParallelism() {
return parallelism;
}
public void setParallelism(Long parallelism) {
this.parallelism = parallelism;
}
public JobDetails status(Object status) {
this.status = status;
return this;
}
/**
* The current status of the job.
* @return status
**/
@ApiModelProperty(required = true, value = "The current status of the job.")
public Object getStatus() {
return status;
}
public void setStatus(Object status) {
this.status = status;
}
public JobDetails number(Long number) {
this.number = number;
return this;
}
/**
* The number of the job.
* @return number
**/
@ApiModelProperty(required = true, value = "The number of the job.")
public Long getNumber() {
return number;
}
public void setNumber(Long number) {
this.number = number;
}
public JobDetails pipeline(JobDetailsPipeline pipeline) {
this.pipeline = pipeline;
return this;
}
/**
* Get pipeline
* @return pipeline
**/
@ApiModelProperty(required = true, value = "")
public JobDetailsPipeline getPipeline() {
return pipeline;
}
public void setPipeline(JobDetailsPipeline pipeline) {
this.pipeline = pipeline;
}
public JobDetails duration(Long duration) {
this.duration = duration;
return this;
}
/**
* Duration of a job in milliseconds.
* @return duration
**/
@ApiModelProperty(required = true, value = "Duration of a job in milliseconds.")
public Long getDuration() {
return duration;
}
public void setDuration(Long duration) {
this.duration = duration;
}
public JobDetails createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* The time when the job was created.
* @return createdAt
**/
@ApiModelProperty(required = true, value = "The time when the job was created.")
public OffsetDateTime getCreatedAt() {
return createdAt;
}
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public JobDetails messages(List messages) {
this.messages = messages;
return this;
}
public JobDetails addMessagesItem(JobDetailsMessages messagesItem) {
this.messages.add(messagesItem);
return this;
}
/**
* Messages from CircleCI execution platform.
* @return messages
**/
@ApiModelProperty(required = true, value = "Messages from CircleCI execution platform.")
public List getMessages() {
return messages;
}
public void setMessages(List messages) {
this.messages = messages;
}
public JobDetails contexts(List contexts) {
this.contexts = contexts;
return this;
}
public JobDetails addContextsItem(JobDetailsContexts contextsItem) {
this.contexts.add(contextsItem);
return this;
}
/**
* List of contexts used by the job.
* @return contexts
**/
@ApiModelProperty(required = true, value = "List of contexts used by the job.")
public List getContexts() {
return contexts;
}
public void setContexts(List contexts) {
this.contexts = contexts;
}
public JobDetails organization(JobDetailsOrganization organization) {
this.organization = organization;
return this;
}
/**
* Get organization
* @return organization
**/
@ApiModelProperty(required = true, value = "")
public JobDetailsOrganization getOrganization() {
return organization;
}
public void setOrganization(JobDetailsOrganization organization) {
this.organization = organization;
}
public JobDetails queuedAt(OffsetDateTime queuedAt) {
this.queuedAt = queuedAt;
return this;
}
/**
* The time when the job was placed in a queue.
* @return queuedAt
**/
@ApiModelProperty(required = true, value = "The time when the job was placed in a queue.")
public OffsetDateTime getQueuedAt() {
return queuedAt;
}
public void setQueuedAt(OffsetDateTime queuedAt) {
this.queuedAt = queuedAt;
}
public JobDetails stoppedAt(OffsetDateTime stoppedAt) {
this.stoppedAt = stoppedAt;
return this;
}
/**
* The time when the job stopped.
* @return stoppedAt
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "The time when the job stopped.")
public OffsetDateTime getStoppedAt() {
return stoppedAt;
}
public void setStoppedAt(OffsetDateTime stoppedAt) {
this.stoppedAt = stoppedAt;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
JobDetails jobDetails = (JobDetails) o;
return Objects.equals(this.webUrl, jobDetails.webUrl) &&
Objects.equals(this.project, jobDetails.project) &&
Objects.equals(this.parallelRuns, jobDetails.parallelRuns) &&
Objects.equals(this.startedAt, jobDetails.startedAt) &&
Objects.equals(this.latestWorkflow, jobDetails.latestWorkflow) &&
Objects.equals(this.name, jobDetails.name) &&
Objects.equals(this.executor, jobDetails.executor) &&
Objects.equals(this.parallelism, jobDetails.parallelism) &&
Objects.equals(this.status, jobDetails.status) &&
Objects.equals(this.number, jobDetails.number) &&
Objects.equals(this.pipeline, jobDetails.pipeline) &&
Objects.equals(this.duration, jobDetails.duration) &&
Objects.equals(this.createdAt, jobDetails.createdAt) &&
Objects.equals(this.messages, jobDetails.messages) &&
Objects.equals(this.contexts, jobDetails.contexts) &&
Objects.equals(this.organization, jobDetails.organization) &&
Objects.equals(this.queuedAt, jobDetails.queuedAt) &&
Objects.equals(this.stoppedAt, jobDetails.stoppedAt);
}
@Override
public int hashCode() {
return Objects.hash(webUrl, project, parallelRuns, startedAt, latestWorkflow, name, executor, parallelism, status, number, pipeline, duration, createdAt, messages, contexts, organization, queuedAt, stoppedAt);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class JobDetails {\n");
sb.append(" webUrl: ").append(toIndentedString(webUrl)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" parallelRuns: ").append(toIndentedString(parallelRuns)).append("\n");
sb.append(" startedAt: ").append(toIndentedString(startedAt)).append("\n");
sb.append(" latestWorkflow: ").append(toIndentedString(latestWorkflow)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" executor: ").append(toIndentedString(executor)).append("\n");
sb.append(" parallelism: ").append(toIndentedString(parallelism)).append("\n");
sb.append(" status: ").append(toIndentedString(status)).append("\n");
sb.append(" number: ").append(toIndentedString(number)).append("\n");
sb.append(" pipeline: ").append(toIndentedString(pipeline)).append("\n");
sb.append(" duration: ").append(toIndentedString(duration)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" messages: ").append(toIndentedString(messages)).append("\n");
sb.append(" contexts: ").append(toIndentedString(contexts)).append("\n");
sb.append(" organization: ").append(toIndentedString(organization)).append("\n");
sb.append(" queuedAt: ").append(toIndentedString(queuedAt)).append("\n");
sb.append(" stoppedAt: ").append(toIndentedString(stoppedAt)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy