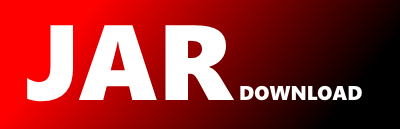
com.circleci.client.v2.model.PipelineVcs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-client Show documentation
Show all versions of java-client Show documentation
Client for CircleCI's API v2
/*
* CircleCI API
* This describes the resources that make up the CircleCI API v2. API v2 is currently in Preview. Additional documentation for this API can be found in the [API Preview Docs](https://github.com/CircleCI-Public/api-preview-docs/tree/master/docs). Breaking changes to the API will be announced in the [Breaking Changes log](https://github.com/CircleCI-Public/api-preview-docs/blob/master/docs/breaking.md).
*
* The version of the OpenAPI document: v2
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.circleci.client.v2.model;
import java.util.Objects;
import java.util.Arrays;
import com.circleci.client.v2.model.PipelineVcsCommit;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
/**
* VCS information for the pipeline.
*/
@ApiModel(description = "VCS information for the pipeline.")
public class PipelineVcs {
public static final String JSON_PROPERTY_PROVIDER_NAME = "provider_name";
@JsonProperty(JSON_PROPERTY_PROVIDER_NAME)
private String providerName;
public static final String JSON_PROPERTY_ORIGIN_REPOSITORY_URL = "origin_repository_url";
@JsonProperty(JSON_PROPERTY_ORIGIN_REPOSITORY_URL)
private String originRepositoryUrl;
public static final String JSON_PROPERTY_TARGET_REPOSITORY_URL = "target_repository_url";
@JsonProperty(JSON_PROPERTY_TARGET_REPOSITORY_URL)
private String targetRepositoryUrl;
public static final String JSON_PROPERTY_REVISION = "revision";
@JsonProperty(JSON_PROPERTY_REVISION)
private String revision;
public static final String JSON_PROPERTY_BRANCH = "branch";
@JsonProperty(JSON_PROPERTY_BRANCH)
private String branch;
public static final String JSON_PROPERTY_TAG = "tag";
@JsonProperty(JSON_PROPERTY_TAG)
private String tag;
public static final String JSON_PROPERTY_COMMIT = "commit";
@JsonProperty(JSON_PROPERTY_COMMIT)
private PipelineVcsCommit commit = null;
public PipelineVcs providerName(String providerName) {
this.providerName = providerName;
return this;
}
/**
* Name of the VCS provider (e.g. GitHub, Bitbucket).
* @return providerName
**/
@ApiModelProperty(example = "GitHub", required = true, value = "Name of the VCS provider (e.g. GitHub, Bitbucket).")
public String getProviderName() {
return providerName;
}
public void setProviderName(String providerName) {
this.providerName = providerName;
}
public PipelineVcs originRepositoryUrl(String originRepositoryUrl) {
this.originRepositoryUrl = originRepositoryUrl;
return this;
}
/**
* URL for the repository where the trigger originated. For fork-PR pipelines, this is the URL to the fork. For other pipelines the `origin_` and `target_repository_url`s will be the same.
* @return originRepositoryUrl
**/
@ApiModelProperty(example = "https://github.com/CircleCI-Public/api-preview-docs", required = true, value = "URL for the repository where the trigger originated. For fork-PR pipelines, this is the URL to the fork. For other pipelines the `origin_` and `target_repository_url`s will be the same.")
public String getOriginRepositoryUrl() {
return originRepositoryUrl;
}
public void setOriginRepositoryUrl(String originRepositoryUrl) {
this.originRepositoryUrl = originRepositoryUrl;
}
public PipelineVcs targetRepositoryUrl(String targetRepositoryUrl) {
this.targetRepositoryUrl = targetRepositoryUrl;
return this;
}
/**
* URL for the repository the trigger targets (i.e. the repository where the PR will be merged). For fork-PR pipelines, this is the URL to the parent repo. For other pipelines, the `origin_` and `target_repository_url`s will be the same.
* @return targetRepositoryUrl
**/
@ApiModelProperty(example = "https://github.com/CircleCI-Public/api-preview-docs", required = true, value = "URL for the repository the trigger targets (i.e. the repository where the PR will be merged). For fork-PR pipelines, this is the URL to the parent repo. For other pipelines, the `origin_` and `target_repository_url`s will be the same.")
public String getTargetRepositoryUrl() {
return targetRepositoryUrl;
}
public void setTargetRepositoryUrl(String targetRepositoryUrl) {
this.targetRepositoryUrl = targetRepositoryUrl;
}
public PipelineVcs revision(String revision) {
this.revision = revision;
return this;
}
/**
* The code revision the pipeline ran.
* @return revision
**/
@ApiModelProperty(example = "f454a02b5d10fcccfd7d9dd7608a76d6493a98b4", required = true, value = "The code revision the pipeline ran.")
public String getRevision() {
return revision;
}
public void setRevision(String revision) {
this.revision = revision;
}
public PipelineVcs branch(String branch) {
this.branch = branch;
return this;
}
/**
* The branch where the pipeline ran. The HEAD commit on this branch was used for the pipeline. Note that `branch` and `tag` are mutually exclusive.
* @return branch
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "feature/design-new-api", value = "The branch where the pipeline ran. The HEAD commit on this branch was used for the pipeline. Note that `branch` and `tag` are mutually exclusive.")
public String getBranch() {
return branch;
}
public void setBranch(String branch) {
this.branch = branch;
}
public PipelineVcs tag(String tag) {
this.tag = tag;
return this;
}
/**
* The tag used by the pipeline. The commit that this tag points to was used for the pipeline. Note that `branch` and `tag` are mutually exclusive.
* @return tag
**/
@javax.annotation.Nullable
@ApiModelProperty(example = "v3.1.4159", value = "The tag used by the pipeline. The commit that this tag points to was used for the pipeline. Note that `branch` and `tag` are mutually exclusive.")
public String getTag() {
return tag;
}
public void setTag(String tag) {
this.tag = tag;
}
public PipelineVcs commit(PipelineVcsCommit commit) {
this.commit = commit;
return this;
}
/**
* Get commit
* @return commit
**/
@javax.annotation.Nullable
@ApiModelProperty(value = "")
public PipelineVcsCommit getCommit() {
return commit;
}
public void setCommit(PipelineVcsCommit commit) {
this.commit = commit;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PipelineVcs pipelineVcs = (PipelineVcs) o;
return Objects.equals(this.providerName, pipelineVcs.providerName) &&
Objects.equals(this.originRepositoryUrl, pipelineVcs.originRepositoryUrl) &&
Objects.equals(this.targetRepositoryUrl, pipelineVcs.targetRepositoryUrl) &&
Objects.equals(this.revision, pipelineVcs.revision) &&
Objects.equals(this.branch, pipelineVcs.branch) &&
Objects.equals(this.tag, pipelineVcs.tag) &&
Objects.equals(this.commit, pipelineVcs.commit);
}
@Override
public int hashCode() {
return Objects.hash(providerName, originRepositoryUrl, targetRepositoryUrl, revision, branch, tag, commit);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PipelineVcs {\n");
sb.append(" providerName: ").append(toIndentedString(providerName)).append("\n");
sb.append(" originRepositoryUrl: ").append(toIndentedString(originRepositoryUrl)).append("\n");
sb.append(" targetRepositoryUrl: ").append(toIndentedString(targetRepositoryUrl)).append("\n");
sb.append(" revision: ").append(toIndentedString(revision)).append("\n");
sb.append(" branch: ").append(toIndentedString(branch)).append("\n");
sb.append(" tag: ").append(toIndentedString(tag)).append("\n");
sb.append(" commit: ").append(toIndentedString(commit)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy