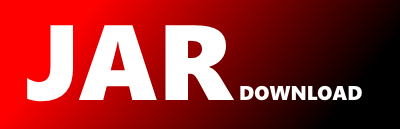
com.cisco.common.constructs.AbstractTree Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of device-package-maven-plugin Show documentation
Show all versions of device-package-maven-plugin Show documentation
Provides support for building Device Package related projects for various Cisco Network Management Products.
The newest version!
/******************************************************************************
* Copyright (c) 2011-2018 by Cisco Systems, Inc. and/or its affiliates.
* All rights reserved.
*
* This software is made available under the CISCO SAMPLE CODE LICENSE
* Version 1.1. See LICENSE.TXT at the root of this project for more information.
*
*****************************************************************************/
package com.cisco.common.constructs;
import java.util.ArrayList;
import java.util.Collection;
/**
* A basic tree implementation for storing data in a hierarchical fashion.
*
* @author danijoh2
*
* @param
* - The type of objects that will be stored in the tree.
*/
public abstract class AbstractTree implements ITree {
Collection> roots = new ArrayList>();
/**
* {@inheritDoc}
*/
public Collection> getRoots() {
return roots;
}
/**
* {@inheritDoc}
*/
public Node find(T data) {
for (Node root : roots) {
Node node = internalFind(root, data);
if (node != null) {
return node;
}
}
return null;
}
private Node internalFind(Node currentNode, T data) {
if (compareEqual(currentNode.getData(), data)) {
return currentNode;
}
for (Node node : currentNode.getChildren()) {
Node returnNode = internalFind(node, data);
if (returnNode != null) {
return returnNode;
}
}
return null;
}
/**
* {@inheritDoc}
*/
public Node addRoot(T data) {
Node node = new Node(data, null);
roots.add(node);
return node;
}
/**
* {@inheritDoc}
*/
public abstract boolean compareEqual(T a, T b);
/**
* {@inheritDoc}
*/
public void walkTree(final ITreeWalker callback) {
roots.stream().forEach(root -> walkSubTree(root, callback));
}
/**
* {@inheritDoc}
*/
public void walkSubTree(Node startNode, ITreeWalker callback) {
if (callback.processNode(startNode) && startNode.hasChildren()) {
startNode.getChildren().stream().forEach(child -> walkSubTree(child, callback));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy