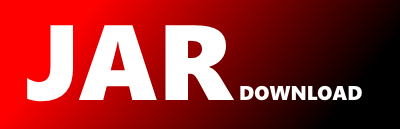
com.citahub.cita.protocol.Service Maven / Gradle / Ivy
The newest version!
package com.citahub.cita.protocol;
import java.io.IOException;
import java.io.InputStream;
import java.util.concurrent.Callable;
import java.util.concurrent.Future;
import com.citahub.cita.protocol.core.Request;
import com.citahub.cita.protocol.core.Response;
import com.fasterxml.jackson.databind.ObjectMapper;
import io.reactivex.Flowable;
import io.reactivex.Notification;
import com.citahub.cita.utils.Async;
/**
* Base service implementation.
*/
public abstract class Service implements CITAjService {
protected final ObjectMapper objectMapper;
public Service(boolean includeRawResponses) {
objectMapper = ObjectMapperFactory.getObjectMapper(includeRawResponses);
}
protected abstract InputStream performIO(String payload) throws IOException;
@Override
public T send(
Request request, Class responseType) throws IOException {
String payload = objectMapper.writeValueAsString(request);
InputStream result = null;
try {
result = performIO(payload);
if(result != null) {
return objectMapper.readValue(result, responseType);
}
return null;
}finally {
if(result != null)
result.close();
}
}
@Override
public Future sendAsync(
final Request jsonRpc20Request, final Class responseType) {
return Async.run(new Callable() {
@Override
public T call() throws Exception {
return Service.this.send(jsonRpc20Request, responseType);
}
});
}
@Override
public > Flowable subscribe(
Request request,
String unsubscribeMethod,
Class responseType) {
throw new UnsupportedOperationException(
String.format(
"Service %s does not support subscriptions",
this.getClass().getSimpleName()));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy