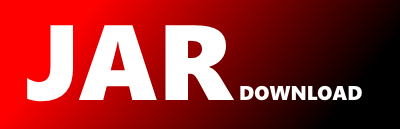
com.citrix.sharefile.api.entities.SFMetadataEntity Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.entities;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.entities.*;
import com.citrix.sharefile.api.models.*;
import com.citrix.sharefile.api.SFApiQuery;
import com.citrix.sharefile.api.interfaces.ISFQuery;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.enumerations.SFSafeEnum;
import com.citrix.sharefile.api.enumerations.SFSafeEnumFlags;
import com.citrix.sharefile.api.interfaces.ISFApiClient;
import com.citrix.sharefile.api.exceptions.InvalidOrMissingParameterException;
public class SFMetadataEntity extends SFEntitiesBase
{
public SFMetadataEntity(ISFApiClient client) {
super(client);
}
/**
* Get Metadata by ID
* Retrieves a single Metadata entry that has a given Name for a given Item.
* Note:
* 'GET https://account.sf-api.com/sf/v3/Items(parentid)/Metadata(id)' is unsupported.
* Current routing doesn't support the URI to retrieve a single Metadata enntry since it is aliased with the GetByItem feed.
* So for now we support only the same syntax as for AccessControls, i.e. .../Metadata(name=name,itemid=itemid)
* @return A single Metadata object matching the query
*/
public ISFQuery get(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Metadata List By Item
* Retrieves the Metadata List for a given Item.
* @param url
* @return The Metadata list of the given object ID.
*/
public ISFQuery> getByItem(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Items");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create Metadata
* {
* "Name":"metadata name",
* "Value":"metadata value"
* }
* Creates a single Metadata entry that has a specified Name for a given Item. Fails if an entry with the given name already exists for this Item.
* @param url
* @return The created Metadata object
*/
public ISFQuery createByItem(URI url, SFMetadata metadata) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (metadata == null) {
throw new InvalidOrMissingParameterException("metadata");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Items");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setBody(metadata);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Update Metadata
* {
* "Value":"metadata value"
* }
* Updates a single Metadata entry that has a specified Name for a given Item. Fails if an entry with the given name doesn't exist for this Item.
* @param url
* @return The updated Metadata object
*/
public ISFQuery updateByItem(URI url, String metadataId, SFMetadata metadata) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (metadataId == null) {
throw new InvalidOrMissingParameterException("metadataId");
}
if (metadata == null) {
throw new InvalidOrMissingParameterException("metadata");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Items");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.addActionIds(metadataId);
sfApiQuery.setBody(metadata);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Update Metadata
* {
* "Value":"metadata value"
* }
* Updates a single Metadata entry that has a specified Name for a given Item. Fails if an entry with the given name doesn't exist for this Item.
* @return The updated Metadata object
*/
public ISFQuery update(URI url, SFMetadata metadata) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (metadata == null) {
throw new InvalidOrMissingParameterException("metadata");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setBody(metadata);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Update Item Metadata
* [{
* "Value":"metadata value"
* },{
* "Value":"metadata value"
* },
* ...]
* Update the item corresponding to the supplied id with the provided key-value Metadata models.
* If a metadata key is not already associated with the item, the item is updated with the new key-value pair.
* @param url
* @return The updated Metadata list for the given object ID.
*/
public ISFQuery> updateItem(URI url, ArrayList metadata) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (metadata == null) {
throw new InvalidOrMissingParameterException("metadata");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Items");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setBody(metadata);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Delete Metadata
* Deletes a single Metadata entry that has a specified Name for a given Item. Fails if an entry with the given name doesn't exist for this Item.
* @param url
* @return (no data)
*/
public ISFQuery deleteByItem(URI url, String metadataId) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (metadataId == null) {
throw new InvalidOrMissingParameterException("metadataId");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Items");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.addActionIds(metadataId);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete Metadata
* Deletes a single Metadata entry that has a specified Name for a given Item. Fails if an entry with the given name doesn't exist for this Item.
* @return (no data)
*/
public ISFQuery delete(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy