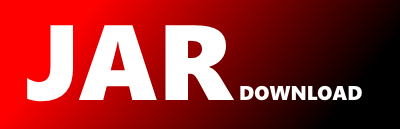
com.citrix.sharefile.api.entities.SFReportsEntity Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.entities;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.entities.*;
import com.citrix.sharefile.api.models.*;
import com.citrix.sharefile.api.SFApiQuery;
import com.citrix.sharefile.api.interfaces.ISFQuery;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.enumerations.SFSafeEnum;
import com.citrix.sharefile.api.enumerations.SFSafeEnumFlags;
import com.citrix.sharefile.api.interfaces.ISFApiClient;
import com.citrix.sharefile.api.exceptions.InvalidOrMissingParameterException;
public class SFReportsEntity extends SFEntitiesBase
{
public SFReportsEntity(ISFApiClient client) {
super(client);
}
/**
* Get Reports for Current Account
* Returns all the reports configured for the current account. By expanding the Records property, a list of all ReportRecords can be accessed as well.
* @return Reports for current account
*/
public ISFQuery> get() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Report by ID
* Returns a single report specified by id. The Records property is expandable.
* @param url
* @return Single Report
*/
public ISFQuery get(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get recent reports
* Returns the most recent reports run for the current account. 10 reports are returned unless otherwise specified.
* @param maxReports (default: 10)
* @return List of reports
*/
public ISFQuery> getRecent(Integer maxReports) throws InvalidOrMissingParameterException {
if (maxReports == null) {
throw new InvalidOrMissingParameterException("maxReports");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Recent");
sfApiQuery.addQueryString("maxReports", maxReports);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get recent reports
* Returns the most recent reports run for the current account. 10 reports are returned unless otherwise specified.
* @return List of reports
*/
public ISFQuery> getRecent() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Recent");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get recurring reports
* Returns all recurring reports for the current account.
* @return List of reports
*/
public ISFQuery> getRecurring() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Recurring");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Report Record by ID
* Returns a single record.
* @param id
* @return Single Record
*/
public ISFQuery getRecord(String id) throws InvalidOrMissingParameterException {
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Record");
sfApiQuery.addActionIds(id);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get all Records by Report ID
* Returns all records for a single report.
* @param url
* @return Records for a Report
*/
public ISFQuery> getRecords(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Records");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create Report
* {
* "Title": "Usage Report",
* "ReportType": "Activity",
* "ActivityTypes": "Login,Upload,Download,View",
* "ObjectType": "Account",
* "ObjectId": "accountId",
* "DateOption": "Last30Days",
* "SaveFormat": "Excel"
* }
* Creates a new Report. The sample above is for an Account Activity Report. For more options please refer to the Report
* model documentation.
* @param report
* @param runOnCreate
* @return Created Report
*/
public ISFQuery create(SFReport report, Boolean runOnCreate) throws InvalidOrMissingParameterException {
if (report == null) {
throw new InvalidOrMissingParameterException("report");
}
if (runOnCreate == null) {
throw new InvalidOrMissingParameterException("runOnCreate");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.addQueryString("runOnCreate", runOnCreate);
sfApiQuery.setBody(report);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Update Report
* {
* "Title": "Usage Report",
* "ReportType": "Activity",
* "ObjectType": "Account",
* "ObjectId": "a024f83e-b147-437e-9f28-e7d03634af42",
* "DateOption": "Last30Days",
* "Frequency": "Once"
* }
* Updates an existing report
* @param report
* @return the updated report
*/
public ISFQuery update(SFReport report) throws InvalidOrMissingParameterException {
if (report == null) {
throw new InvalidOrMissingParameterException("report");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setBody(report);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Delete Report
* Removes a report from the system
* @param url
*/
public ISFQuery delete(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Run Report
* Run a report and get the run id.
* @return ReportRecord
*/
public ISFQuery getRun(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Run");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get a preview location for the report
* @param reportUrl
*/
public ISFQuery preview(URI reportUrl) throws InvalidOrMissingParameterException {
if (reportUrl == null) {
throw new InvalidOrMissingParameterException("reportUrl");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Preview");
sfApiQuery.addIds(reportUrl);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get JSON Data
* Get the JSON data for a report
* @return JSON Formatted Report Results
*/
public ISFQuery getJsonData(String id) throws InvalidOrMissingParameterException {
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Records");
sfApiQuery.addActionIds(id);
sfApiQuery.addSubAction("JsonData");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Save a folder to a folder location
* @param reportUrl
* @param folderId
*/
public ISFQuery move(URI reportUrl, String folderId) throws InvalidOrMissingParameterException {
if (reportUrl == null) {
throw new InvalidOrMissingParameterException("reportUrl");
}
if (folderId == null) {
throw new InvalidOrMissingParameterException("folderId");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Move");
sfApiQuery.addIds(reportUrl);
sfApiQuery.addQueryString("folderId", folderId);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get spreadsheet data
* Get the spreadsheet data for a report
* @return Excel Formatted Report Results
*/
public ISFQuery downloadData(String id) throws InvalidOrMissingParameterException {
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Reports");
sfApiQuery.setAction("Records");
sfApiQuery.addActionIds(id);
sfApiQuery.addSubAction("DownloadData");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy