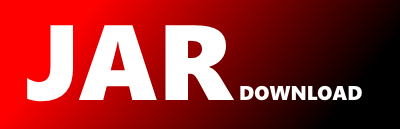
com.citrix.sharefile.api.entities.SFSharesEntity Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.entities;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.entities.*;
import com.citrix.sharefile.api.models.*;
import com.citrix.sharefile.api.SFApiQuery;
import com.citrix.sharefile.api.interfaces.ISFQuery;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.enumerations.SFSafeEnum;
import com.citrix.sharefile.api.enumerations.SFSafeEnumFlags;
import com.citrix.sharefile.api.interfaces.ISFApiClient;
import com.citrix.sharefile.api.exceptions.InvalidOrMissingParameterException;
public class SFSharesEntity extends SFEntitiesBase
{
public SFSharesEntity(ISFApiClient client) {
super(client);
}
/**
* Get List of Shares
* Retrieve all outstanding Shares of the authenticated user
* @param includeExpired (default: true)
* @return List of Shares created by the authenticated user
*/
public ISFQuery> get(Boolean includeExpired) throws InvalidOrMissingParameterException {
if (includeExpired == null) {
throw new InvalidOrMissingParameterException("includeExpired");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addQueryString("includeExpired", includeExpired);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Shares
* Retrieve all outstanding Shares of the authenticated user
* @return List of Shares created by the authenticated user
*/
public ISFQuery> get() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Shares
* Retrieve all outstanding Shares of the authenticated user
* @return List of Shares created by the authenticated user
*/
public ISFQuery get(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Recipients of a Share
* Retrieve the list of Recipients in the share. Recipients represent the target users of the Share, containing
* access information, such as number of times that user downloaded files from the share. Each Recipient is
* identified by an Alias, which is an unique ID given to each user - allowing tracking of downloads for
* non-authenticated users.
* @param url
* @return A feed of Share Aliases representing recipients of the Share
*/
public ISFQuery> getRecipients(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Recipient of a Share
* Retrieve a single Share Recipient identified by the alias id.
* @param parentUrl
* @param id
* @return A Share Alias representing a single recipient of the Share
*/
public ISFQuery getRecipients(URI parentUrl, String id) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addActionIds(id);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create Recipient for a Share
* Creates a Recipient User for a Share that requires user information
* @param parentUrl
* @param Email (default: null)
* @param FirstName (default: null)
* @param LastName (default: null)
* @param Company (default: null)
* @return A Share Alias representing a single recipient of the Share
*/
public ISFQuery createRecipients(URI parentUrl, String Email, String FirstName, String LastName, String Company) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
if (FirstName == null) {
throw new InvalidOrMissingParameterException("FirstName");
}
if (LastName == null) {
throw new InvalidOrMissingParameterException("LastName");
}
if (Company == null) {
throw new InvalidOrMissingParameterException("Company");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.addQueryString("FirstName", FirstName);
sfApiQuery.addQueryString("LastName", LastName);
sfApiQuery.addQueryString("Company", Company);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Recipient for a Share
* Creates a Recipient User for a Share that requires user information
* @param parentUrl
* @param Email (default: null)
* @param FirstName (default: null)
* @param LastName (default: null)
* @return A Share Alias representing a single recipient of the Share
*/
public ISFQuery createRecipients(URI parentUrl, String Email, String FirstName, String LastName) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
if (FirstName == null) {
throw new InvalidOrMissingParameterException("FirstName");
}
if (LastName == null) {
throw new InvalidOrMissingParameterException("LastName");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.addQueryString("FirstName", FirstName);
sfApiQuery.addQueryString("LastName", LastName);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Recipient for a Share
* Creates a Recipient User for a Share that requires user information
* @param parentUrl
* @param Email (default: null)
* @param FirstName (default: null)
* @return A Share Alias representing a single recipient of the Share
*/
public ISFQuery createRecipients(URI parentUrl, String Email, String FirstName) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
if (FirstName == null) {
throw new InvalidOrMissingParameterException("FirstName");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.addQueryString("FirstName", FirstName);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Recipient for a Share
* Creates a Recipient User for a Share that requires user information
* @param parentUrl
* @param Email (default: null)
* @return A Share Alias representing a single recipient of the Share
*/
public ISFQuery createRecipients(URI parentUrl, String Email) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Recipient for a Share
* Creates a Recipient User for a Share that requires user information
* @param parentUrl
* @return A Share Alias representing a single recipient of the Share
*/
public ISFQuery createRecipients(URI parentUrl) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(parentUrl);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get Items of a Share
* Retrieve the list of Items (files and folders) in the Send Share.
* @param url
* @return A feed of Items of the Share
*/
public ISFQuery> getItems(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Items");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Items of a Send Share
* Retrieve a single Item in the Send Share
* @param shareUrl
* @return An item in the Share
*/
public ISFQuery getItems(URI shareUrl, String itemid) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemid == null) {
throw new InvalidOrMissingParameterException("itemid");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Items");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(itemid);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Thumbnail of a Share Item
* Retrieve a thumbnail link for the specified Item in the Share.
* @param shareUrl
* @param size (default: 75)
* @param redirect (default: false)
* @return A 302 redirection to the Thumbnail link
*/
public ISFQuery thumbnail(URI shareUrl, String itemid, Integer size, Boolean redirect) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemid == null) {
throw new InvalidOrMissingParameterException("itemid");
}
if (size == null) {
throw new InvalidOrMissingParameterException("size");
}
if (redirect == null) {
throw new InvalidOrMissingParameterException("redirect");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Items");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(itemid);
sfApiQuery.addSubAction("Thumbnail");
sfApiQuery.addQueryString("size", size);
sfApiQuery.addQueryString("redirect", redirect);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Thumbnail of a Share Item
* Retrieve a thumbnail link for the specified Item in the Share.
* @param shareUrl
* @param size (default: 75)
* @return A 302 redirection to the Thumbnail link
*/
public ISFQuery thumbnail(URI shareUrl, String itemid, Integer size) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemid == null) {
throw new InvalidOrMissingParameterException("itemid");
}
if (size == null) {
throw new InvalidOrMissingParameterException("size");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Items");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(itemid);
sfApiQuery.addSubAction("Thumbnail");
sfApiQuery.addQueryString("size", size);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Thumbnail of a Share Item
* Retrieve a thumbnail link for the specified Item in the Share.
* @param shareUrl
* @return A 302 redirection to the Thumbnail link
*/
public ISFQuery thumbnail(URI shareUrl, String itemid) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemid == null) {
throw new InvalidOrMissingParameterException("itemid");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Items");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(itemid);
sfApiQuery.addSubAction("Thumbnail");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Protocol Links of a Share item
* @param shareUrl
* @return A list of protocol links depending on the input parameter 'platform', 404 (Not Found) if not supported by the item
*/
public ISFQuery> protocolLinks(URI shareUrl, String itemid, SFSafeEnum platform) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemid == null) {
throw new InvalidOrMissingParameterException("itemid");
}
if (platform == null) {
throw new InvalidOrMissingParameterException("platform");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Items");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(itemid);
sfApiQuery.addSubAction("ProtocolLinks", platform);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Downloads Share Items
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require authentication, make sure this request is authenticated. To download
* shares that require require user information, provide the Name, Email and Company parameters in the URI
* query. Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Share(id)/Items(id)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @param Name (default: null)
* @param Email (default: null)
* @param Company (default: null)
* @param redirect (default: true)
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery download(URI shareUrl, String itemId, String Name, String Email, String Company, Boolean redirect) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
if (Name == null) {
throw new InvalidOrMissingParameterException("Name");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
if (Company == null) {
throw new InvalidOrMissingParameterException("Company");
}
if (redirect == null) {
throw new InvalidOrMissingParameterException("redirect");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Download");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.addQueryString("Name", Name);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.addQueryString("Company", Company);
sfApiQuery.addQueryString("redirect", redirect);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Downloads Share Items
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require authentication, make sure this request is authenticated. To download
* shares that require require user information, provide the Name, Email and Company parameters in the URI
* query. Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Share(id)/Items(id)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @param Name (default: null)
* @param Email (default: null)
* @param Company (default: null)
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery download(URI shareUrl, String itemId, String Name, String Email, String Company) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
if (Name == null) {
throw new InvalidOrMissingParameterException("Name");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
if (Company == null) {
throw new InvalidOrMissingParameterException("Company");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Download");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.addQueryString("Name", Name);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.addQueryString("Company", Company);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Downloads Share Items
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require authentication, make sure this request is authenticated. To download
* shares that require require user information, provide the Name, Email and Company parameters in the URI
* query. Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Share(id)/Items(id)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @param Name (default: null)
* @param Email (default: null)
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery download(URI shareUrl, String itemId, String Name, String Email) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
if (Name == null) {
throw new InvalidOrMissingParameterException("Name");
}
if (Email == null) {
throw new InvalidOrMissingParameterException("Email");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Download");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.addQueryString("Name", Name);
sfApiQuery.addQueryString("Email", Email);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Downloads Share Items
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require authentication, make sure this request is authenticated. To download
* shares that require require user information, provide the Name, Email and Company parameters in the URI
* query. Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Share(id)/Items(id)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @param Name (default: null)
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery download(URI shareUrl, String itemId, String Name) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
if (Name == null) {
throw new InvalidOrMissingParameterException("Name");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Download");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.addQueryString("Name", Name);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Downloads Share Items
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require authentication, make sure this request is authenticated. To download
* shares that require require user information, provide the Name, Email and Company parameters in the URI
* query. Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Share(id)/Items(id)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery download(URI shareUrl, String itemId) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Download");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Downloads Share Items
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require authentication, make sure this request is authenticated. To download
* shares that require require user information, provide the Name, Email and Company parameters in the URI
* query. Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Share(id)/Items(id)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery download(URI shareUrl) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Download");
sfApiQuery.addIds(shareUrl);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Download Items from a Share for a Recipient
* GET https://account.sf-api.com/sf/v3/Shares(shareid)/Recipients(aliasid)/DownloadWithAlias?id=itemid
* GET https://account.sf-api.com/sf/v3/Shares(shareid)/Recipients(aliasid)/DownloadWithAlias(itemid)
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require user informaion ( Email, First Name, Last Name and Company), make sure
* to create an Recipient (alias)To download Shares that require authentication, make sure this request is authenticated.
* Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Shares(id)/Recipients(aliasid)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @param redirect (default: true)
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery downloadWithAlias(URI shareUrl, String aliasid, String itemId, Boolean redirect) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (aliasid == null) {
throw new InvalidOrMissingParameterException("aliasid");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
if (redirect == null) {
throw new InvalidOrMissingParameterException("redirect");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(aliasid);
sfApiQuery.addSubAction("DownloadWithAlias");
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.addQueryString("redirect", redirect);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Download Items from a Share for a Recipient
* GET https://account.sf-api.com/sf/v3/Shares(shareid)/Recipients(aliasid)/DownloadWithAlias?id=itemid
* GET https://account.sf-api.com/sf/v3/Shares(shareid)/Recipients(aliasid)/DownloadWithAlias(itemid)
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require user informaion ( Email, First Name, Last Name and Company), make sure
* to create an Recipient (alias)To download Shares that require authentication, make sure this request is authenticated.
* Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Shares(id)/Recipients(aliasid)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery downloadWithAlias(URI shareUrl, String aliasid, String itemId) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (aliasid == null) {
throw new InvalidOrMissingParameterException("aliasid");
}
if (itemId == null) {
throw new InvalidOrMissingParameterException("itemId");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(aliasid);
sfApiQuery.addSubAction("DownloadWithAlias");
sfApiQuery.addQueryString("id", itemId);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Download Items from a Share for a Recipient
* GET https://account.sf-api.com/sf/v3/Shares(shareid)/Recipients(aliasid)/DownloadWithAlias?id=itemid
* GET https://account.sf-api.com/sf/v3/Shares(shareid)/Recipients(aliasid)/DownloadWithAlias(itemid)
* Downloads items from the Share. The default action will download all Items in the Share.
* If a Share has a single item, the download attachment name
* will use the item name. Otherwise, the download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require user informaion ( Email, First Name, Last Name and Company), make sure
* to create an Recipient (alias)To download Shares that require authentication, make sure this request is authenticated.
* Anyone can download files from anonymous shares.You can also download individual Items in the Share. Use the Shares(id)/Recipients(aliasid)/Download action. The
* item ID must be a top-level item in the Share - i.e., you cannot download or address files contained inside
* a shared folder.
* @param shareUrl
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery downloadWithAlias(URI shareUrl, String aliasid) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (aliasid == null) {
throw new InvalidOrMissingParameterException("aliasid");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(aliasid);
sfApiQuery.addSubAction("DownloadWithAlias");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Download Multiple Items from a Share for a Recipient
* ["id1","id2",...]
* Download Multiple Items from a Share for a Recipient. The download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require user informaion ( Email, First Name, Last Name and Company), make sure
* to create an Recipient (alias) and pass in the alaisId.To download Shares that require authentication, make sure this request is authenticated.
* Anyone can download files from anonymous shares.
* @param shareUrl
* @param ids
* @param redirect (default: true)
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery bulkDownload(URI shareUrl, String aliasid, ArrayList ids, Boolean redirect) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (aliasid == null) {
throw new InvalidOrMissingParameterException("aliasid");
}
if (ids == null) {
throw new InvalidOrMissingParameterException("ids");
}
if (redirect == null) {
throw new InvalidOrMissingParameterException("redirect");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(aliasid);
sfApiQuery.addSubAction("BulkDownload");
sfApiQuery.addQueryString("redirect", redirect);
sfApiQuery.setBody(ids);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Download Multiple Items from a Share for a Recipient
* ["id1","id2",...]
* Download Multiple Items from a Share for a Recipient. The download will contain a ZIP archive containing all
* files and folders in the share, named Files.zip.To download Shares that require user informaion ( Email, First Name, Last Name and Company), make sure
* to create an Recipient (alias) and pass in the alaisId.To download Shares that require authentication, make sure this request is authenticated.
* Anyone can download files from anonymous shares.
* @param shareUrl
* @param ids
* @return Redirects the caller (302) to the download address for the share contents.
*/
public ISFQuery bulkDownload(URI shareUrl, String aliasid, ArrayList ids) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (aliasid == null) {
throw new InvalidOrMissingParameterException("aliasid");
}
if (ids == null) {
throw new InvalidOrMissingParameterException("ids");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(aliasid);
sfApiQuery.addSubAction("BulkDownload");
sfApiQuery.setBody(ids);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Share
* {
* "ShareType":"Send",
* "Title":"Sample Send Share",
* "Items": [ { "Id":"itemid" }, {...} ],
* "Recipients":[ { "User": { "Id":"userid" } }, { "User": { "Email": "user@email" } } ],
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "RequireUserInfo": false,
* "MaxDownloads": -1,
* "UsesStreamIDs": false
* }
* {
* "ShareType":"Request",
* "Title":"Sample Request Share",
* "Recipients":[ { "User": { "Id":"userid" } }, { "User": { "Email": "user@email" } } ],
* "Parent": { "Id":"folderid" },
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "RequireUserInfo": false,
* "TrackUntilDate": "2013-07-23",
* "SendFrequency": -1,
* "SendInterval": -1
* }
* Creates a new Send or Request Share.
* Expiration date:
* - if not specified the default is 30 days
* - "9999-12-31" disables share expiration.
* To use stream IDs as item IDs UsesStreamIDs needs to be set to true, and all the IDs in Items need to be specified
* as stream IDs.
* View Only:
* View Only share can be created by either setting "IsViewOnly = true" or "share.ShareAccessRight.ShareAccessRightType = ViewOnline"
* If both "share.IsViewOnly = true" and "share.ShareAccessRight.AccessRightType = FullControl" are passed to this method, then the "Full Control" permission takes higher priority
* and disables "ViewOnly" permission on the share.
* Only one of the two features(ViewOnly, IRM) can be enabled at a time. If you set both "IsViewOnly = true" and "share.ShareAccessRight.ShareAccessRightType = IRM", exception will be thrown
* @param share
* @param notify (default: false)
* @param direct (default: false)
* @return The new Share
*/
public ISFQuery create(SFShare share, Boolean notify, Boolean direct) throws InvalidOrMissingParameterException {
if (share == null) {
throw new InvalidOrMissingParameterException("share");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (direct == null) {
throw new InvalidOrMissingParameterException("direct");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("direct", direct);
sfApiQuery.setBody(share);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Share
* {
* "ShareType":"Send",
* "Title":"Sample Send Share",
* "Items": [ { "Id":"itemid" }, {...} ],
* "Recipients":[ { "User": { "Id":"userid" } }, { "User": { "Email": "user@email" } } ],
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "RequireUserInfo": false,
* "MaxDownloads": -1,
* "UsesStreamIDs": false
* }
* {
* "ShareType":"Request",
* "Title":"Sample Request Share",
* "Recipients":[ { "User": { "Id":"userid" } }, { "User": { "Email": "user@email" } } ],
* "Parent": { "Id":"folderid" },
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "RequireUserInfo": false,
* "TrackUntilDate": "2013-07-23",
* "SendFrequency": -1,
* "SendInterval": -1
* }
* Creates a new Send or Request Share.
* Expiration date:
* - if not specified the default is 30 days
* - "9999-12-31" disables share expiration.
* To use stream IDs as item IDs UsesStreamIDs needs to be set to true, and all the IDs in Items need to be specified
* as stream IDs.
* View Only:
* View Only share can be created by either setting "IsViewOnly = true" or "share.ShareAccessRight.ShareAccessRightType = ViewOnline"
* If both "share.IsViewOnly = true" and "share.ShareAccessRight.AccessRightType = FullControl" are passed to this method, then the "Full Control" permission takes higher priority
* and disables "ViewOnly" permission on the share.
* Only one of the two features(ViewOnly, IRM) can be enabled at a time. If you set both "IsViewOnly = true" and "share.ShareAccessRight.ShareAccessRightType = IRM", exception will be thrown
* @param share
* @param notify (default: false)
* @return The new Share
*/
public ISFQuery create(SFShare share, Boolean notify) throws InvalidOrMissingParameterException {
if (share == null) {
throw new InvalidOrMissingParameterException("share");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.setBody(share);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Share
* {
* "ShareType":"Send",
* "Title":"Sample Send Share",
* "Items": [ { "Id":"itemid" }, {...} ],
* "Recipients":[ { "User": { "Id":"userid" } }, { "User": { "Email": "user@email" } } ],
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "RequireUserInfo": false,
* "MaxDownloads": -1,
* "UsesStreamIDs": false
* }
* {
* "ShareType":"Request",
* "Title":"Sample Request Share",
* "Recipients":[ { "User": { "Id":"userid" } }, { "User": { "Email": "user@email" } } ],
* "Parent": { "Id":"folderid" },
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "RequireUserInfo": false,
* "TrackUntilDate": "2013-07-23",
* "SendFrequency": -1,
* "SendInterval": -1
* }
* Creates a new Send or Request Share.
* Expiration date:
* - if not specified the default is 30 days
* - "9999-12-31" disables share expiration.
* To use stream IDs as item IDs UsesStreamIDs needs to be set to true, and all the IDs in Items need to be specified
* as stream IDs.
* View Only:
* View Only share can be created by either setting "IsViewOnly = true" or "share.ShareAccessRight.ShareAccessRightType = ViewOnline"
* If both "share.IsViewOnly = true" and "share.ShareAccessRight.AccessRightType = FullControl" are passed to this method, then the "Full Control" permission takes higher priority
* and disables "ViewOnly" permission on the share.
* Only one of the two features(ViewOnly, IRM) can be enabled at a time. If you set both "IsViewOnly = true" and "share.ShareAccessRight.ShareAccessRightType = IRM", exception will be thrown
* @param share
* @return The new Share
*/
public ISFQuery create(SFShare share) throws InvalidOrMissingParameterException {
if (share == null) {
throw new InvalidOrMissingParameterException("share");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setBody(share);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Update Share
* {
* "Title": "New Title",
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "Items": [ { "Id":"itemid" }, {...} ],
* }
* Modifies an existing Share. If Items are specified they are added to the share by default. If appendItemsFeed is set to false, the specified Items will replace any existing ones instead.
*
* View Only:
* If a share is not IRM Classified, it can be updated to ViewOnline/ViewOnly share by passing either "IsViewOnly= true" or "Share.ShareAccessRight.AccessRightType = ViewOnline"
* If a share is IRM Classified, then it can be updated to ViewOnline/ViewOnly share only by passing "Share.ShareAccessRight = ViewOnline". This will remove the IRMClassification on this share.
* Only one of the two features(ViewOnly, IRM) can be enabled at a time.
*
* Full Control:
* Passing "Share.ShareAccessRight.AccessRightType = FullControl" will remove IRMClassification and ViewOnly features on the share. If you set both "IsViewOnly = true" and "share.ShareAccessRight.ShareAccessRightType = IRM", exception will be thrown
* @param url
* @param share
* @param appendItemsFeed (default: true)
* @return The modified Share
*/
public ISFQuery update(URI url, SFShare share, Boolean appendItemsFeed) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (share == null) {
throw new InvalidOrMissingParameterException("share");
}
if (appendItemsFeed == null) {
throw new InvalidOrMissingParameterException("appendItemsFeed");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("appendItemsFeed", appendItemsFeed);
sfApiQuery.setBody(share);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Update Share
* {
* "Title": "New Title",
* "ExpirationDate": "2013-07-23",
* "RequireLogin": false,
* "Items": [ { "Id":"itemid" }, {...} ],
* }
* Modifies an existing Share. If Items are specified they are added to the share by default. If appendItemsFeed is set to false, the specified Items will replace any existing ones instead.
*
* View Only:
* If a share is not IRM Classified, it can be updated to ViewOnline/ViewOnly share by passing either "IsViewOnly= true" or "Share.ShareAccessRight.AccessRightType = ViewOnline"
* If a share is IRM Classified, then it can be updated to ViewOnline/ViewOnly share only by passing "Share.ShareAccessRight = ViewOnline". This will remove the IRMClassification on this share.
* Only one of the two features(ViewOnly, IRM) can be enabled at a time.
*
* Full Control:
* Passing "Share.ShareAccessRight.AccessRightType = FullControl" will remove IRMClassification and ViewOnly features on the share. If you set both "IsViewOnly = true" and "share.ShareAccessRight.ShareAccessRightType = IRM", exception will be thrown
* @param url
* @param share
* @return The modified Share
*/
public ISFQuery update(URI url, SFShare share) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (share == null) {
throw new InvalidOrMissingParameterException("share");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addIds(url);
sfApiQuery.setBody(share);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Delete Share
* Removes an existing Share
* @param url
*/
public ISFQuery delete(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Create Share Alias
* Creates a share alias for the specified share ID and user email. If a user with the given email address does not
* exist it is created first.
* For shares requiring login an activation email is sent to the created user. If 'notify' is enabled, the user activation is
* included in the share notification email.
* @param url
* @param email
* @param notify (default: false)
* @return Share with the AliasID property set to the created alias ID
*/
public ISFQuery createAlias(URI url, String email, Boolean notify) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Alias");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("email", email);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Share Alias
* Creates a share alias for the specified share ID and user email. If a user with the given email address does not
* exist it is created first.
* For shares requiring login an activation email is sent to the created user. If 'notify' is enabled, the user activation is
* included in the share notification email.
* @param url
* @param email
* @return Share with the AliasID property set to the created alias ID
*/
public ISFQuery createAlias(URI url, String email) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Alias");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("email", email);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Deliver Send a File Email
* {
* "Items":["itemId1", "itemId2", ...],
* "Emails":["[email protected]", "[email protected]",...]
* "Subject": "Email Subject",
* "Body": "Email Message",
* "CcSender": false,
* "NotifyOnDownload": true,
* "RequireLogin": false,
* "MaxDownloads": 30,
* "ExpirationDays": -1
* }
* Sends an Email to the specified list of addresses, containing a link to the specified Items.
* The default number of expiration days is 30. Setting it to -1 disables share expiration. Note that the
* Emails and Items parameters expect an array of strings, rather than nested JSON objects.
* @param parameters
* @return Share object
*/
public ISFQuery createSend(SFShareSendParams parameters) throws InvalidOrMissingParameterException {
if (parameters == null) {
throw new InvalidOrMissingParameterException("parameters");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Send");
sfApiQuery.setBody(parameters);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Deliver Request a File Email
* {
* "FolderId":"folderId",
* "Emails":["[email protected]", "[email protected]",...]
* "Subject": "Email Subject",
* "Body": "Email Message",
* "CcSender": false,
* "NotifyOnUpload": true,
* "RequireLogin": false,
* "ExpirationDays": -1
* }
* Sends an Email to the specified list of addresses, containing a link to upload to the specified folder.
* The default number of expiration days is 30. Setting it to -1 disables share expiration. Note that the
* Emails parameter expectd an array of strings, rather than nested JSON objects.
*
* View Only:
* View Only share can be created by either setting "IsViewOnly = true" or "share.ShareAccessRight.ShareAccessRightType = ViewOnline"
* If both "share.IsViewOnly = true" and "share.ShareAccessRight.AccessRightType = FullControl" are passed to this method, then the "Full Control" permission takes higher priority
* and disables "ViewOnly" permission on the share.
* Only one of the two features(ViewOnly, IRM) can be enabled at a time. If you set both "IsViewOnly = true" and "share.ShareAccessRight.ShareAccessRightType = IRM", exception will be thrown
* @param parameters
* @return Share Object
*/
public ISFQuery createRequest(SFShareRequestParams parameters) throws InvalidOrMissingParameterException {
if (parameters == null) {
throw new InvalidOrMissingParameterException("parameters");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Request");
sfApiQuery.setBody(parameters);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Re-deliver an Existing Share Link
* {
* "Recipients":["[email protected]", "groupId",...]
* "Subject": "Email Subject",
* "Body": "Email Message",
* "CcSender": false,
* "NotifyOnUse": true,
* "ShareId": "shareId"
* }
* Resends an Email to the specified list of addresses, containing a link to a Send or Request Share
* @param parameters
* @return The updated Share
*/
public ISFQuery resend(SFShareResendParams parameters) throws InvalidOrMissingParameterException {
if (parameters == null) {
throw new InvalidOrMissingParameterException("parameters");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Resend");
sfApiQuery.setBody(parameters);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @param threadCount (default: 4)
* @param responseFormat (default: "json")
* @param notify (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid, Integer threadCount, String responseFormat, Boolean notify, Date clientCreatedDateUTC, Date clientModifiedDateUTC, Integer expirationDays) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
if (threadCount == null) {
throw new InvalidOrMissingParameterException("threadCount");
}
if (responseFormat == null) {
throw new InvalidOrMissingParameterException("responseFormat");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (clientCreatedDateUTC == null) {
throw new InvalidOrMissingParameterException("clientCreatedDateUTC");
}
if (clientModifiedDateUTC == null) {
throw new InvalidOrMissingParameterException("clientModifiedDateUTC");
}
if (expirationDays == null) {
throw new InvalidOrMissingParameterException("expirationDays");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.addQueryString("threadCount", threadCount);
sfApiQuery.addQueryString("responseFormat", responseFormat);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("clientCreatedDateUTC", clientCreatedDateUTC);
sfApiQuery.addQueryString("clientModifiedDateUTC", clientModifiedDateUTC);
sfApiQuery.addQueryString("expirationDays", expirationDays);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @param threadCount (default: 4)
* @param responseFormat (default: "json")
* @param notify (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid, Integer threadCount, String responseFormat, Boolean notify, Date clientCreatedDateUTC, Date clientModifiedDateUTC) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
if (threadCount == null) {
throw new InvalidOrMissingParameterException("threadCount");
}
if (responseFormat == null) {
throw new InvalidOrMissingParameterException("responseFormat");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (clientCreatedDateUTC == null) {
throw new InvalidOrMissingParameterException("clientCreatedDateUTC");
}
if (clientModifiedDateUTC == null) {
throw new InvalidOrMissingParameterException("clientModifiedDateUTC");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.addQueryString("threadCount", threadCount);
sfApiQuery.addQueryString("responseFormat", responseFormat);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("clientCreatedDateUTC", clientCreatedDateUTC);
sfApiQuery.addQueryString("clientModifiedDateUTC", clientModifiedDateUTC);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @param threadCount (default: 4)
* @param responseFormat (default: "json")
* @param notify (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid, Integer threadCount, String responseFormat, Boolean notify, Date clientCreatedDateUTC) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
if (threadCount == null) {
throw new InvalidOrMissingParameterException("threadCount");
}
if (responseFormat == null) {
throw new InvalidOrMissingParameterException("responseFormat");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (clientCreatedDateUTC == null) {
throw new InvalidOrMissingParameterException("clientCreatedDateUTC");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.addQueryString("threadCount", threadCount);
sfApiQuery.addQueryString("responseFormat", responseFormat);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("clientCreatedDateUTC", clientCreatedDateUTC);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @param threadCount (default: 4)
* @param responseFormat (default: "json")
* @param notify (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid, Integer threadCount, String responseFormat, Boolean notify) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
if (threadCount == null) {
throw new InvalidOrMissingParameterException("threadCount");
}
if (responseFormat == null) {
throw new InvalidOrMissingParameterException("responseFormat");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.addQueryString("threadCount", threadCount);
sfApiQuery.addQueryString("responseFormat", responseFormat);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @param threadCount (default: 4)
* @param responseFormat (default: "json")
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid, Integer threadCount, String responseFormat) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
if (threadCount == null) {
throw new InvalidOrMissingParameterException("threadCount");
}
if (responseFormat == null) {
throw new InvalidOrMissingParameterException("responseFormat");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.addQueryString("threadCount", threadCount);
sfApiQuery.addQueryString("responseFormat", responseFormat);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @param threadCount (default: 4)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid, Integer threadCount) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
if (threadCount == null) {
throw new InvalidOrMissingParameterException("threadCount");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.addQueryString("threadCount", threadCount);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @param opid (default: null)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid, String opid) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
if (opid == null) {
throw new InvalidOrMissingParameterException("opid");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.addQueryString("opid", opid);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @param sendGuid (default: null)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend, String sendGuid) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
if (sendGuid == null) {
throw new InvalidOrMissingParameterException("sendGuid");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.addQueryString("sendGuid", sendGuid);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @param isSend (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details, Boolean isSend) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
if (isSend == null) {
throw new InvalidOrMissingParameterException("isSend");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.addQueryString("isSend", isSend);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @param details (default: null)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title, String details) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
if (details == null) {
throw new InvalidOrMissingParameterException("details");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.addQueryString("details", details);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @param title (default: null)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite, String title) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
if (title == null) {
throw new InvalidOrMissingParameterException("title");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.addQueryString("title", title);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @param overwrite (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool, Boolean overwrite) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
if (overwrite == null) {
throw new InvalidOrMissingParameterException("overwrite");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.addQueryString("overwrite", overwrite);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @param tool (default: "apiv3")
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip, String tool) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
if (tool == null) {
throw new InvalidOrMissingParameterException("tool");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.addQueryString("tool", tool);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @param unzip (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver, Boolean unzip) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
if (unzip == null) {
throw new InvalidOrMissingParameterException("unzip");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.addQueryString("unzip", unzip);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @param startOver (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume, Boolean startOver) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
if (startOver == null) {
throw new InvalidOrMissingParameterException("startOver");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.addQueryString("startOver", startOver);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @param canResume (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast, Boolean canResume) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
if (canResume == null) {
throw new InvalidOrMissingParameterException("canResume");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.addQueryString("canResume", canResume);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @param batchLast (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId, Boolean batchLast) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
if (batchLast == null) {
throw new InvalidOrMissingParameterException("batchLast");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.addQueryString("batchLast", batchLast);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @param batchId (default: null)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize, String batchId) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
if (batchId == null) {
throw new InvalidOrMissingParameterException("batchId");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.addQueryString("batchId", batchId);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @param fileSize (default: 0)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName, Long fileSize) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
if (fileSize == null) {
throw new InvalidOrMissingParameterException("fileSize");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.addQueryString("fileSize", fileSize);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @param fileName (default: null)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw, String fileName) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
if (fileName == null) {
throw new InvalidOrMissingParameterException("fileName");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.addQueryString("fileName", fileName);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @param raw (default: false)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method, Boolean raw) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
if (raw == null) {
throw new InvalidOrMissingParameterException("raw");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.addQueryString("raw", raw);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @param method (default: Standard)
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url, SFSafeEnum method) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (method == null) {
throw new InvalidOrMissingParameterException("method");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("method", method);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Upload File to Request Share
* POST https://account.sf-api.com/sf/v3/Shares(id)/Upload2
* {
* "Method":"Method",
* "Raw": false,
* "FileName":"FileName"
* "FileLength": 123
* }
* Prepares the links for uploading files to the target Share.
* This method returns an Upload Specification object. The fields are
* populated based on the upload method, provider, and resume parameters passed to the
* upload call.
* The Method determines how the URLs must be called.
*
* Standard uploads use a single HTTP POST message to the ChunkUri address provided in
* the response. All other fields will be empty. Standard uploads do not support Resume.
*
* Streamed uploads use multiple HTTP POST calls to the ChunkUri address. The client must
* append the parameters index, offset and hash to the end of the ChunkUri address. Index
* is a sequential number representing the data block (zero-based); Offset represents the
* byte offset for the block; and hash contains the MD5 hash of the block. The last HTTP
* POST must also contain finish=true parameter.
*
* Threaded uploads use multiple HTTP POST calls to ChunkUri, and can have a number of
* threads issuing blocks in parallel. Clients must append index, offset and hash to
* the end of ChunkUri, as explained in Streamed. After all chunks were sent, the client
* must call the FinishUri provided in this spec.
*
* For all uploaders, the contents of the POST Body can either be "raw", if the "Raw" parameter
* was provided to the Uploader, or use MIME multi-part form encoding otherwise. Raw uploads
* simply put the block content in the POST body - Content-Length specifies the size. Multi-part
* form encoding has to pass the File as a Form parameter named "File1".
*
* For streamed and threaded, if Resume options were provided to the Upload call, then the
* fields IsResume, ResumeIndex, ResumeOffset and ResumeFileHash MAY be populated. If they are,
* it indicates that the server has identified a partial upload with that specification, and is
* ready to resume on the provided parameters. The clients can then verify the ResumeFileHash to
* ensure the file has not been modified; and continue issuing ChunkUri calls from the ResumeIndex
* ResumeOffset parameters. If the client decides to restart, it should simply ignore the resume
* parameters and send the blocks from Index 0.
*
* For all uploaders: the result code for the HTTP POST calls to Chunk and Finish Uri can either
* be a 401 - indicating authentication is required; 4xx/5xx indicating some kind of error; or
* 200 with a Content Body of format 'ERROR:message'. Successful calls will return either a 200
* response with no Body, or with Body of format 'OK'.
* @param url
* @return an Upload Specification element, containing the links for uploading, and the parameters for resume. The caller must know the protocol for sending the prepare, chunk and finish URLs returned in the spec; as well as negotiate the resume upload.
*/
public ISFQuery upload(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
public ISFQuery upload2(URI url, SFUploadRequestParams uploadParams, Integer expirationDays) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (uploadParams == null) {
throw new InvalidOrMissingParameterException("uploadParams");
}
if (expirationDays == null) {
throw new InvalidOrMissingParameterException("expirationDays");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload2");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("expirationDays", expirationDays);
sfApiQuery.setBody(uploadParams);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
public ISFQuery upload2(URI url, SFUploadRequestParams uploadParams) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (uploadParams == null) {
throw new InvalidOrMissingParameterException("uploadParams");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Upload2");
sfApiQuery.addIds(url);
sfApiQuery.setBody(uploadParams);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get Redirection endpoint Information
* Returns the redirection endpoint for this Share.
* @param url
* @return The Redirection endpoint Information
*/
public ISFQuery getRedirection(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Redirection");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox(String userId, SFSafeEnum type, Boolean archived) throws InvalidOrMissingParameterException {
if (userId == null) {
throw new InvalidOrMissingParameterException("userId");
}
if (type == null) {
throw new InvalidOrMissingParameterException("type");
}
if (archived == null) {
throw new InvalidOrMissingParameterException("archived");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Inbox");
sfApiQuery.addActionIds(userId);
sfApiQuery.addQueryString("type", type);
sfApiQuery.addQueryString("archived", archived);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox(String userId, SFSafeEnum type) throws InvalidOrMissingParameterException {
if (userId == null) {
throw new InvalidOrMissingParameterException("userId");
}
if (type == null) {
throw new InvalidOrMissingParameterException("type");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Inbox");
sfApiQuery.addActionIds(userId);
sfApiQuery.addQueryString("type", type);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox(String userId) throws InvalidOrMissingParameterException {
if (userId == null) {
throw new InvalidOrMissingParameterException("userId");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Inbox");
sfApiQuery.addActionIds(userId);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Inbox");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Sent Message Content by Share
* Returns sent message content.
* @param shareUrl
* @return Sent Message Content
*/
public ISFQuery message(URI shareUrl, String aliasId) throws InvalidOrMissingParameterException {
if (shareUrl == null) {
throw new InvalidOrMissingParameterException("shareUrl");
}
if (aliasId == null) {
throw new InvalidOrMissingParameterException("aliasId");
}
SFQueryStream sfApiQuery = new SFQueryStream(this.client);
sfApiQuery.setFrom("Shares");
sfApiQuery.setAction("Recipients");
sfApiQuery.addIds(shareUrl);
sfApiQuery.addActionIds(aliasId);
sfApiQuery.addSubAction("Message");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy