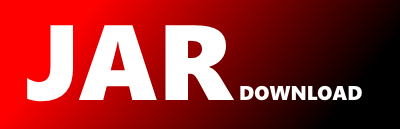
com.citrix.sharefile.api.entities.SFUsersEntity Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.entities;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.entities.*;
import com.citrix.sharefile.api.models.*;
import com.citrix.sharefile.api.SFApiQuery;
import com.citrix.sharefile.api.interfaces.ISFQuery;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.enumerations.SFSafeEnum;
import com.citrix.sharefile.api.enumerations.SFSafeEnumFlags;
import com.citrix.sharefile.api.interfaces.ISFApiClient;
import com.citrix.sharefile.api.exceptions.InvalidOrMissingParameterException;
public class SFUsersEntity extends SFEntitiesBase
{
public SFUsersEntity(ISFApiClient client) {
super(client);
}
/**
* Get User
* Retrieve a single user, by ID or email, or the currently authenticated user.
* @param id (default: null)
* @param emailAddress (default: null)
* @return the requested User object
*/
public ISFQuery get(String id, String emailAddress) throws InvalidOrMissingParameterException {
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
if (emailAddress == null) {
throw new InvalidOrMissingParameterException("emailAddress");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("id", id);
sfApiQuery.addQueryString("emailAddress", emailAddress);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get User
* Retrieve a single user, by ID or email, or the currently authenticated user.
* @param id (default: null)
* @return the requested User object
*/
public ISFQuery get(String id) throws InvalidOrMissingParameterException {
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("id", id);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get User
* Retrieve a single user, by ID or email, or the currently authenticated user.
* @return the requested User object
*/
public ISFQuery get() {
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create Client User
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* }
* }
* Creates a new Client User and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, Preferences.CanResetPassword and Preferences.CanViewMySettingsOther parameters are ignored
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @param notify (default: false)
* @param ifNecessary (default: false)
* @param addPersonal (default: false)
* @return The new user
*/
public ISFQuery create(SFUser user, Boolean pushCreatorDefaultSettings, Boolean addshared, Boolean notify, Boolean ifNecessary, Boolean addPersonal) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (ifNecessary == null) {
throw new InvalidOrMissingParameterException("ifNecessary");
}
if (addPersonal == null) {
throw new InvalidOrMissingParameterException("addPersonal");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("ifNecessary", ifNecessary);
sfApiQuery.addQueryString("addPersonal", addPersonal);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Client User
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* }
* }
* Creates a new Client User and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, Preferences.CanResetPassword and Preferences.CanViewMySettingsOther parameters are ignored
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @param notify (default: false)
* @param ifNecessary (default: false)
* @return The new user
*/
public ISFQuery create(SFUser user, Boolean pushCreatorDefaultSettings, Boolean addshared, Boolean notify, Boolean ifNecessary) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (ifNecessary == null) {
throw new InvalidOrMissingParameterException("ifNecessary");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("ifNecessary", ifNecessary);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Client User
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* }
* }
* Creates a new Client User and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, Preferences.CanResetPassword and Preferences.CanViewMySettingsOther parameters are ignored
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @param notify (default: false)
* @return The new user
*/
public ISFQuery create(SFUser user, Boolean pushCreatorDefaultSettings, Boolean addshared, Boolean notify) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Client User
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* }
* }
* Creates a new Client User and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, Preferences.CanResetPassword and Preferences.CanViewMySettingsOther parameters are ignored
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @return The new user
*/
public ISFQuery create(SFUser user, Boolean pushCreatorDefaultSettings, Boolean addshared) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Client User
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* }
* }
* Creates a new Client User and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, Preferences.CanResetPassword and Preferences.CanViewMySettingsOther parameters are ignored
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @return The new user
*/
public ISFQuery create(SFUser user, Boolean pushCreatorDefaultSettings) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Client User
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* }
* }
* Creates a new Client User and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, Preferences.CanResetPassword and Preferences.CanViewMySettingsOther parameters are ignored
* @param user
* @return The new user
*/
public ISFQuery create(SFUser user) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Employee
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "StorageQuotaLimitGB":50,
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* },
* "IsAdministrator": false,
* "CanCreateFolders": false,
* "CanUseFileBox": true,
* "CanManageUsers": false,
* "Roles": [
* "CanChangePassword", "CanManageMySettings",
* "CanUseFileBox", "CanManageUsers", "CanCreateFolders", "CanUseDropBox", "CanSelectFolderZone",
* "AdminAccountPolicies", "AdminBilling", "AdminBranding", "AdminChangePlan", "AdminFileBoxAccess",
* "AdminManageEmployees", "AdminRemoteUploadForms", "AdminReporting", "AdminSharedDistGroups",
* "AdminSharedAddressBook", "AdminViewReceipts", "AdminDelegate", "AdminManageFolderTemplates",
* "AdminEmailMessages", "AdminSSO", "AdminSuperGroup", "AdminZones", "AdminCreateSharedGroups", "AdminConnectors"
* ]
* }
* Creates a new Employee User (AccountUser) and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, IsEmployee, IsAdministrator, CanCreateFolders, CanUseFileBox, CanManageUsers,
* Preferences.CanResetPassword and Preferences.CanViewMySettings.
* Other parameters are ignoredStorageQuotaLimitGB parameter is optional. If not specified or equal to -1 the account default storage quota value will be set for the User.
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @param notify (default: false)
* @param ifNecessary (default: false)
* @param addPersonal (default: false)
* @return The new employee user
*/
public ISFQuery createAccountUser(SFAccountUser user, Boolean pushCreatorDefaultSettings, Boolean addshared, Boolean notify, Boolean ifNecessary, Boolean addPersonal) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (ifNecessary == null) {
throw new InvalidOrMissingParameterException("ifNecessary");
}
if (addPersonal == null) {
throw new InvalidOrMissingParameterException("addPersonal");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("ifNecessary", ifNecessary);
sfApiQuery.addQueryString("addPersonal", addPersonal);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Employee
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "StorageQuotaLimitGB":50,
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* },
* "IsAdministrator": false,
* "CanCreateFolders": false,
* "CanUseFileBox": true,
* "CanManageUsers": false,
* "Roles": [
* "CanChangePassword", "CanManageMySettings",
* "CanUseFileBox", "CanManageUsers", "CanCreateFolders", "CanUseDropBox", "CanSelectFolderZone",
* "AdminAccountPolicies", "AdminBilling", "AdminBranding", "AdminChangePlan", "AdminFileBoxAccess",
* "AdminManageEmployees", "AdminRemoteUploadForms", "AdminReporting", "AdminSharedDistGroups",
* "AdminSharedAddressBook", "AdminViewReceipts", "AdminDelegate", "AdminManageFolderTemplates",
* "AdminEmailMessages", "AdminSSO", "AdminSuperGroup", "AdminZones", "AdminCreateSharedGroups", "AdminConnectors"
* ]
* }
* Creates a new Employee User (AccountUser) and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, IsEmployee, IsAdministrator, CanCreateFolders, CanUseFileBox, CanManageUsers,
* Preferences.CanResetPassword and Preferences.CanViewMySettings.
* Other parameters are ignoredStorageQuotaLimitGB parameter is optional. If not specified or equal to -1 the account default storage quota value will be set for the User.
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @param notify (default: false)
* @param ifNecessary (default: false)
* @return The new employee user
*/
public ISFQuery createAccountUser(SFAccountUser user, Boolean pushCreatorDefaultSettings, Boolean addshared, Boolean notify, Boolean ifNecessary) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
if (ifNecessary == null) {
throw new InvalidOrMissingParameterException("ifNecessary");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.addQueryString("ifNecessary", ifNecessary);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Employee
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "StorageQuotaLimitGB":50,
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* },
* "IsAdministrator": false,
* "CanCreateFolders": false,
* "CanUseFileBox": true,
* "CanManageUsers": false,
* "Roles": [
* "CanChangePassword", "CanManageMySettings",
* "CanUseFileBox", "CanManageUsers", "CanCreateFolders", "CanUseDropBox", "CanSelectFolderZone",
* "AdminAccountPolicies", "AdminBilling", "AdminBranding", "AdminChangePlan", "AdminFileBoxAccess",
* "AdminManageEmployees", "AdminRemoteUploadForms", "AdminReporting", "AdminSharedDistGroups",
* "AdminSharedAddressBook", "AdminViewReceipts", "AdminDelegate", "AdminManageFolderTemplates",
* "AdminEmailMessages", "AdminSSO", "AdminSuperGroup", "AdminZones", "AdminCreateSharedGroups", "AdminConnectors"
* ]
* }
* Creates a new Employee User (AccountUser) and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, IsEmployee, IsAdministrator, CanCreateFolders, CanUseFileBox, CanManageUsers,
* Preferences.CanResetPassword and Preferences.CanViewMySettings.
* Other parameters are ignoredStorageQuotaLimitGB parameter is optional. If not specified or equal to -1 the account default storage quota value will be set for the User.
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @param notify (default: false)
* @return The new employee user
*/
public ISFQuery createAccountUser(SFAccountUser user, Boolean pushCreatorDefaultSettings, Boolean addshared, Boolean notify) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Employee
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "StorageQuotaLimitGB":50,
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* },
* "IsAdministrator": false,
* "CanCreateFolders": false,
* "CanUseFileBox": true,
* "CanManageUsers": false,
* "Roles": [
* "CanChangePassword", "CanManageMySettings",
* "CanUseFileBox", "CanManageUsers", "CanCreateFolders", "CanUseDropBox", "CanSelectFolderZone",
* "AdminAccountPolicies", "AdminBilling", "AdminBranding", "AdminChangePlan", "AdminFileBoxAccess",
* "AdminManageEmployees", "AdminRemoteUploadForms", "AdminReporting", "AdminSharedDistGroups",
* "AdminSharedAddressBook", "AdminViewReceipts", "AdminDelegate", "AdminManageFolderTemplates",
* "AdminEmailMessages", "AdminSSO", "AdminSuperGroup", "AdminZones", "AdminCreateSharedGroups", "AdminConnectors"
* ]
* }
* Creates a new Employee User (AccountUser) and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, IsEmployee, IsAdministrator, CanCreateFolders, CanUseFileBox, CanManageUsers,
* Preferences.CanResetPassword and Preferences.CanViewMySettings.
* Other parameters are ignoredStorageQuotaLimitGB parameter is optional. If not specified or equal to -1 the account default storage quota value will be set for the User.
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @param addshared (default: false)
* @return The new employee user
*/
public ISFQuery createAccountUser(SFAccountUser user, Boolean pushCreatorDefaultSettings, Boolean addshared) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
if (addshared == null) {
throw new InvalidOrMissingParameterException("addshared");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.addQueryString("addshared", addshared);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Employee
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "StorageQuotaLimitGB":50,
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* },
* "IsAdministrator": false,
* "CanCreateFolders": false,
* "CanUseFileBox": true,
* "CanManageUsers": false,
* "Roles": [
* "CanChangePassword", "CanManageMySettings",
* "CanUseFileBox", "CanManageUsers", "CanCreateFolders", "CanUseDropBox", "CanSelectFolderZone",
* "AdminAccountPolicies", "AdminBilling", "AdminBranding", "AdminChangePlan", "AdminFileBoxAccess",
* "AdminManageEmployees", "AdminRemoteUploadForms", "AdminReporting", "AdminSharedDistGroups",
* "AdminSharedAddressBook", "AdminViewReceipts", "AdminDelegate", "AdminManageFolderTemplates",
* "AdminEmailMessages", "AdminSSO", "AdminSuperGroup", "AdminZones", "AdminCreateSharedGroups", "AdminConnectors"
* ]
* }
* Creates a new Employee User (AccountUser) and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, IsEmployee, IsAdministrator, CanCreateFolders, CanUseFileBox, CanManageUsers,
* Preferences.CanResetPassword and Preferences.CanViewMySettings.
* Other parameters are ignoredStorageQuotaLimitGB parameter is optional. If not specified or equal to -1 the account default storage quota value will be set for the User.
* @param user
* @param pushCreatorDefaultSettings (default: false)
* @return The new employee user
*/
public ISFQuery createAccountUser(SFAccountUser user, Boolean pushCreatorDefaultSettings) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
if (pushCreatorDefaultSettings == null) {
throw new InvalidOrMissingParameterException("pushCreatorDefaultSettings");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.addQueryString("pushCreatorDefaultSettings", pushCreatorDefaultSettings);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create Employee
* {
* "Email":"[email protected]",
* "FirstName":"Name",
* "LastName":"Last Name",
* "Company":"Company",
* "Password":"password",
* "StorageQuotaLimitGB":50,
* "Preferences":
* {
* "CanResetPassword":true,
* "CanViewMySettings":true
* },
* "DefaultZone":
* {
* "Id":"zoneid"
* },
* "IsAdministrator": false,
* "CanCreateFolders": false,
* "CanUseFileBox": true,
* "CanManageUsers": false,
* "Roles": [
* "CanChangePassword", "CanManageMySettings",
* "CanUseFileBox", "CanManageUsers", "CanCreateFolders", "CanUseDropBox", "CanSelectFolderZone",
* "AdminAccountPolicies", "AdminBilling", "AdminBranding", "AdminChangePlan", "AdminFileBoxAccess",
* "AdminManageEmployees", "AdminRemoteUploadForms", "AdminReporting", "AdminSharedDistGroups",
* "AdminSharedAddressBook", "AdminViewReceipts", "AdminDelegate", "AdminManageFolderTemplates",
* "AdminEmailMessages", "AdminSSO", "AdminSuperGroup", "AdminZones", "AdminCreateSharedGroups", "AdminConnectors"
* ]
* }
* Creates a new Employee User (AccountUser) and associates it to an Account
* The following parameters from the input object are used: Email, FirstName, LastName, Company,
* DefaultZone, Password, IsEmployee, IsAdministrator, CanCreateFolders, CanUseFileBox, CanManageUsers,
* Preferences.CanResetPassword and Preferences.CanViewMySettings.
* Other parameters are ignoredStorageQuotaLimitGB parameter is optional. If not specified or equal to -1 the account default storage quota value will be set for the User.
* @param user
* @return The new employee user
*/
public ISFQuery createAccountUser(SFAccountUser user) throws InvalidOrMissingParameterException {
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Update User
* {
* "FirstName":"FirstName",
* "LastName":"LastName",
* "Company":"Company",
* "Email":"[email protected]",
* "Security":
* {
* "IsDisabled":false
* },
* "DefaultZone":
* {
* "Id":"newzoneid"
* }
* }
* Modifies an existing user object
* The following parameters can be modified through this call: FirstName, LastName, Company,
* Email, IsDisabled, DefaultZone Id
* @param url
* @param user
* @return a modified user record
*/
public ISFQuery update(URI url, SFUser user) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addIds(url);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Add Roles
* {
* "Roles" : [ "CanManageUsers", "CanSelectFolderZone" ]
* }
* Modifies an existing user object to ADD a new role
* The following roles can be added to the user through this call (depending on User type):
* CanCreateFolders,
* CanSelectFolderZone,
* CanUseFileBox,
* CanManageUsers,
* AdminSharedAddressBook,
* CanChangePassword,
* CanManageMySettings,
* AdminManageDropBox
* @param parentUrl
* @param user
* @return a modified user record
*/
public ISFQuery updateRoles(URI parentUrl, SFUser user) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Roles");
sfApiQuery.addIds(parentUrl);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Set Roles
* {
* "Roles" : [ "CanManageUsers", "CanSelectFolderZone" ]
* }
* Sets the roles for a user (roles not provided will be disabled.)
* The following roles can be set to the user through this call (depending on User type):
* CanCreateFolders,
* CanSelectFolderZone,
* CanUseFileBox,
* CanManageUsers,
* AdminSharedAddressBook,
* CanChangePassword,
* CanManageMySettings,
* AdminManageDropBox
* @param parentUrl
* @param user
* @return a modified user record
*/
public ISFQuery patchRoles(URI parentUrl, SFUser user) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Roles");
sfApiQuery.addIds(parentUrl);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("PUT");
return sfApiQuery;
}
/**
* Remove Roles
*
* [ "CanManageUsers", "CanSelectFolderZone" ]
* Removes the roles for user.
* The following roles can be removed from user through this call (depending on User type):
* CanCreateFolders,
* CanSelectFolderZone,
* CanUseFileBox,
* CanManageUsers,
* AdminSharedAddressBook,
* CanChangePassword,
* CanManageMySettings
* @param userUrl
* @param userRoles
*/
public ISFQuery removeRoles(URI userUrl, ArrayList> userRoles) throws InvalidOrMissingParameterException {
if (userUrl == null) {
throw new InvalidOrMissingParameterException("userUrl");
}
if (userRoles == null) {
throw new InvalidOrMissingParameterException("userRoles");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("RemoveRoles");
sfApiQuery.addIds(userUrl);
sfApiQuery.setBody(userRoles);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Update Employee or Promote Customer
* {
* "FirstName":"FirstName",
* "LastName":"LastName",
* "Company":"Company",
* "Email":"[email protected]",
* "StorageQuotaLimitGB":100,
* "Security":
* {
* "IsDisabled":false
* },
* "DefaultZone":
* {
* "Id":"newzoneid"
* }
* }
* Modifies an existing user object
* The following parameters can be modified through this call: FirstName, LastName, Company,
* Email, IsEmployee, IsDisabled, DefaultZone Id, StorageQuotaLimitGB.During a promotion (the user id points to Customer), the following parameters can be
* modified: CanCreateFolders, CanUseFileBox, CanManageUsers. StorageQuotaLimitGB equal to -1 sets the user quota to the account default storage quota value.
* @param id
* @param user
* @return a modified user record
*/
public ISFQuery updateAccountUser(String id, SFAccountUser user) throws InvalidOrMissingParameterException {
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
if (user == null) {
throw new InvalidOrMissingParameterException("user");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AccountUser");
sfApiQuery.addActionIds(id);
sfApiQuery.setBody(user);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Get HomeFolder
* Returns a user's home folder
* @param url
* @return A folder record representing the requesting user home folder
*/
public ISFQuery getHomeFolder(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("HomeFolder");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get User's top Folder
* @param url
* @return User's Top Folders
*/
public ISFQuery> getTopFolders(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("TopFolders");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get User's FileBox children
* @param url
* @return User's FileBox children
*/
public ISFQuery> box(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Box");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get User's FileBox folder
* @param url
* @return User's FileBox
*/
public ISFQuery fileBox(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("FileBox");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get User Preferences
* Retrieves the User preferences record - all user-selected prefernces, such as timezone,
* time format, sort preferences, etc.
* @param url
* @return the user selected preferences
*/
public ISFQuery getPreferences(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Preferences");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Update User Preferences
* {
* "EnableFlashUpload":"true",
* "EnableJavaUpload":"true"
* .
* .
* .
* }
* @param parentUrl
* @param preferences
*/
public ISFQuery updatePreferences(URI parentUrl, SFUserPreferences preferences) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (preferences == null) {
throw new InvalidOrMissingParameterException("preferences");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Preferences");
sfApiQuery.addIds(parentUrl);
sfApiQuery.setBody(preferences);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Get User Security
* Retrieve the user security record - current state of the user regarding
* security and password settings.
* @param url
* @return the user security status
*/
public ISFQuery getSecurity(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Security");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Reset Password
* {
* "NewPassword":"new password",
* "OldPassword":"old password"
* }
* {
* "NewPassword":"new password",
* "OldPassword":"old password"
* }
* Resets a user password. A user can reset his own password providing the old and new
* passwords. Administrators can issue this call without providing the old password.
* @param url
* @param properties
* @param notify (default: false)
* @return The modified user record
*/
public ISFQuery resetPassword(URI url, SFODataObject properties, Boolean notify) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (properties == null) {
throw new InvalidOrMissingParameterException("properties");
}
if (notify == null) {
throw new InvalidOrMissingParameterException("notify");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("ResetPassword");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("notify", notify);
sfApiQuery.setBody(properties);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Reset Password
* {
* "NewPassword":"new password",
* "OldPassword":"old password"
* }
* {
* "NewPassword":"new password",
* "OldPassword":"old password"
* }
* Resets a user password. A user can reset his own password providing the old and new
* passwords. Administrators can issue this call without providing the old password.
* @param url
* @param properties
* @return The modified user record
*/
public ISFQuery resetPassword(URI url, SFODataObject properties) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (properties == null) {
throw new InvalidOrMissingParameterException("properties");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("ResetPassword");
sfApiQuery.addIds(url);
sfApiQuery.setBody(properties);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Forgot Password
* Triggers a reset password email
* @param email
* @param resetOnMobile (default: false)
*/
public ISFQuery forgotPassword(String email, Boolean resetOnMobile) throws InvalidOrMissingParameterException {
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
if (resetOnMobile == null) {
throw new InvalidOrMissingParameterException("resetOnMobile");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("ForgotPassword");
sfApiQuery.addQueryString("email", email);
sfApiQuery.addQueryString("resetOnMobile", resetOnMobile);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Forgot Password
* Triggers a reset password email
* @param email
*/
public ISFQuery forgotPassword(String email) throws InvalidOrMissingParameterException {
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("ForgotPassword");
sfApiQuery.addQueryString("email", email);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Send Welcome Email
* Resends the 'welcome' email to the given user
* @param url
* @param customMessage (default: null)
*/
public ISFQuery resendWelcome(URI url, String customMessage) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (customMessage == null) {
throw new InvalidOrMissingParameterException("customMessage");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("ResendWelcome");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("customMessage", customMessage);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Send Welcome Email
* Resends the 'welcome' email to the given user
* @param url
*/
public ISFQuery resendWelcome(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("ResendWelcome");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Delete User
* Removes an user
* @param url
* @param completely (default: false)
* @param itemsReassignTo (default: null)
* @param groupsReassignTo (default: null)
*/
public ISFQuery delete(URI url, Boolean completely, String itemsReassignTo, String groupsReassignTo) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (completely == null) {
throw new InvalidOrMissingParameterException("completely");
}
if (itemsReassignTo == null) {
throw new InvalidOrMissingParameterException("itemsReassignTo");
}
if (groupsReassignTo == null) {
throw new InvalidOrMissingParameterException("groupsReassignTo");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("completely", completely);
sfApiQuery.addQueryString("itemsReassignTo", itemsReassignTo);
sfApiQuery.addQueryString("groupsReassignTo", groupsReassignTo);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete User
* Removes an user
* @param url
* @param completely (default: false)
* @param itemsReassignTo (default: null)
*/
public ISFQuery delete(URI url, Boolean completely, String itemsReassignTo) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (completely == null) {
throw new InvalidOrMissingParameterException("completely");
}
if (itemsReassignTo == null) {
throw new InvalidOrMissingParameterException("itemsReassignTo");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("completely", completely);
sfApiQuery.addQueryString("itemsReassignTo", itemsReassignTo);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete User
* Removes an user
* @param url
* @param completely (default: false)
*/
public ISFQuery delete(URI url, Boolean completely) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (completely == null) {
throw new InvalidOrMissingParameterException("completely");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("completely", completely);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete User
* Removes an user
* @param url
*/
public ISFQuery delete(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete multiple client users
*
* ["id1","id2",...]
* Removes a list of client users. The list should not exceed 100 client user ids.
* @param clientIds
*/
public ISFQuery deleteClients(ArrayList clientIds) throws InvalidOrMissingParameterException {
if (clientIds == null) {
throw new InvalidOrMissingParameterException("clientIds");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Clients");
sfApiQuery.setBody(clientIds);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete multiple client users
* {
* UserIds: ["id1", "id2", ...]
* }
* Removes a list of client users. The list should not exceed 100 client user ids.
* @param deleteRequest
* @return 204 if successful
*/
public ISFQuery bulkDeleteClients(SFUserBulkOperationRequest deleteRequest) throws InvalidOrMissingParameterException {
if (deleteRequest == null) {
throw new InvalidOrMissingParameterException("deleteRequest");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Clients");
sfApiQuery.addSubAction("BulkDelete");
sfApiQuery.setBody(deleteRequest);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Downgrade multiple employee users to clients
* {
* UserIds: ["id1", "id2", ...],
* ReassignItemsToId: "id3",
* ReassignGroupsToId: "id3"
* }
* Downgrades a list of employee users to clients. The list should not exceed 100 employee user ids.
* @param downgradeRequest
* @return 204 if successful
*/
public ISFQuery downgradeEmployees(SFUserBulkDowngradeRequest downgradeRequest) throws InvalidOrMissingParameterException {
if (downgradeRequest == null) {
throw new InvalidOrMissingParameterException("downgradeRequest");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Employees");
sfApiQuery.addSubAction("Downgrade");
sfApiQuery.setBody(downgradeRequest);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get List of User Shared Folders
* Retrieve the list of shared folders the authenticated user has access to
* @return A list of Folder objects, representing shared folders of an user
*/
public ISFQuery> getAllSharedFolders() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("AllSharedFolders");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of User Shared Folders
* Retrieve the top-level folders for this user. This method requires the account to be
* in the new UI model of "My Files", "Shared", "Connectors", "Favorites" tab - otherwise
* it will return an empty list (new UX model is the default since May 2013).
* @return A list of Folder objects, representing shared folders of an user
*/
public ISFQuery> getTopFolders() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("TopFolders");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of User Shared Folders
* Retrieve the connector folders that are associated with a network share service
* @return A list of Folder objects, representing network shared connector folders of an user
*/
public ISFQuery> networkShareConnectors() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("NetworkShareConnectors");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of User Shared Folders
* Retrieve the connector folders that are associated with a sharepoint service
* @return A list of Folder objects, representing sharepoint folders of an user
*/
public ISFQuery> sharepointConnectors() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("SharepointConnectors");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Confirm the current user logging in for the first time
* {
* "FirstName":"",
* "LastName":"",
* "Company":"",
* "Password":"",
* "SecurityQuestion":"",
* "SecurityQuestionAnswer":"",
* "DayLightName":"",
* "UTCOffset":"",
* "DateFormat":"",
* "TimeFormat":"",
* "EmailInterval":0,
* "UserNotificationLocale":"Spanish"
* }
* @param settings
* @return no data on success
*/
public ISFQuery confirm(SFUserConfirmationSettings settings) throws InvalidOrMissingParameterException {
if (settings == null) {
throw new InvalidOrMissingParameterException("settings");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Confirm");
sfApiQuery.setBody(settings);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get Current User Info
* @return UserInfo
*/
public ISFQuery getInfo() {
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Info");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Delete the email address from user
* @param email
* @return User
*/
public ISFQuery deleteEmailAddress(String email) throws InvalidOrMissingParameterException {
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("DeleteEmailAddress");
sfApiQuery.addQueryString("email", email);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Set email address as the primary email address for CURRENT user
* @param email
* @return User
*/
public ISFQuery makePrimary(String email) throws InvalidOrMissingParameterException {
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("MakePrimary");
sfApiQuery.addQueryString("email", email);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Send notification email address to this email address for verification
* @param email
* @return User
*/
public ISFQuery sendConfirmationEmail(String email) throws InvalidOrMissingParameterException {
if (email == null) {
throw new InvalidOrMissingParameterException("email");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("SendConfirmationEmail");
sfApiQuery.addQueryString("email", email);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create a one-time use login Uri for the Web App.
* @return Redirection populated with link in Uri field
*/
public ISFQuery webAppLink() {
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("WebAppLink");
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get Inbox Metadata
* Returns metadata of the inbox.User identifier
* @return Inbox metadata
*/
public ISFQuery inboxMetadata(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("InboxMetadata");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.User identifier
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox(URI url, SFSafeEnum type, Boolean archived) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (type == null) {
throw new InvalidOrMissingParameterException("type");
}
if (archived == null) {
throw new InvalidOrMissingParameterException("archived");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Inbox");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("type", type);
sfApiQuery.addQueryString("archived", archived);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.User identifier
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox(URI url, SFSafeEnum type) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (type == null) {
throw new InvalidOrMissingParameterException("type");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Inbox");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("type", type);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Inbox for Recipient
* Retrieve all outstanding Shares in the inbox.User identifier
* @return List of Shares created by the authenticated user
*/
public ISFQuery> getInbox(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("Inbox");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get Sent Messages
* Returns sent messages for the given user.User identifier
* @return Feed of Shares
*/
public ISFQuery> sentMessages(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("SentMessages");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create a one-time use login Uri for the Web App.
* @param url
* @return Redirection populated with link in Uri field
*/
public ISFQuery webAppManageUser(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("WebAppManageUser");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create a one-time use login Uri for the Web App.
* @return Redirection populated with link in Uri field
*/
public ISFQuery webAppManageUsers() {
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("WebAppManageUsers");
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Create a one-time use login Uri for the Web App.
* @return Redirection populated with link in Uri field
*/
public ISFQuery webAppAddEmployee() {
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Users");
sfApiQuery.setAction("WebAppAddEmployee");
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy