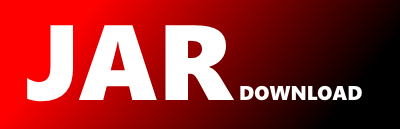
com.citrix.sharefile.api.entities.SFZonesEntity Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.entities;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.entities.*;
import com.citrix.sharefile.api.models.*;
import com.citrix.sharefile.api.SFApiQuery;
import com.citrix.sharefile.api.interfaces.ISFQuery;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.enumerations.SFSafeEnum;
import com.citrix.sharefile.api.enumerations.SFSafeEnumFlags;
import com.citrix.sharefile.api.interfaces.ISFApiClient;
import com.citrix.sharefile.api.exceptions.InvalidOrMissingParameterException;
public class SFZonesEntity extends SFEntitiesBase
{
public SFZonesEntity(ISFApiClient client) {
super(client);
}
/**
* Get List of Zones
* Retrieve the list of Zones accessible to the authenticated user
* This method will concatenate the list of private zones in the user's account and the
* list of public zones accessible to this account. Any user can see the list of zones.
* @param services (default: StorageZone)
* @param includeDisabled (default: false)
* @return The list of public and private zones accessible to this user
*/
public ISFQuery> get(SFSafeEnumFlags services, Boolean includeDisabled) throws InvalidOrMissingParameterException {
if (services == null) {
throw new InvalidOrMissingParameterException("services");
}
if (includeDisabled == null) {
throw new InvalidOrMissingParameterException("includeDisabled");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addQueryString("services", services);
sfApiQuery.addQueryString("includeDisabled", includeDisabled);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Zones
* Retrieve the list of Zones accessible to the authenticated user
* This method will concatenate the list of private zones in the user's account and the
* list of public zones accessible to this account. Any user can see the list of zones.
* @param services (default: StorageZone)
* @return The list of public and private zones accessible to this user
*/
public ISFQuery> get(SFSafeEnumFlags services) throws InvalidOrMissingParameterException {
if (services == null) {
throw new InvalidOrMissingParameterException("services");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addQueryString("services", services);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Zones
* Retrieve the list of Zones accessible to the authenticated user
* This method will concatenate the list of private zones in the user's account and the
* list of public zones accessible to this account. Any user can see the list of zones.
* @return The list of public and private zones accessible to this user
*/
public ISFQuery> get() {
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Zones
* Retrieve the list of Zones accessible to the authenticated user
* This method will concatenate the list of private zones in the user's account and the
* list of public zones accessible to this account. Any user can see the list of zones.
* @return The list of public and private zones accessible to this user
*/
public ISFQuery get(URI url, Boolean secret) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (secret == null) {
throw new InvalidOrMissingParameterException("secret");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("secret", secret);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Get List of Zones
* Retrieve the list of Zones accessible to the authenticated user
* This method will concatenate the list of private zones in the user's account and the
* list of public zones accessible to this account. Any user can see the list of zones.
* @return The list of public and private zones accessible to this user
*/
public ISFQuery get(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create Zone
* {
* "Name":"Name",
* "HeartbeatTolerance":10,
* "ZoneServices":"StorageZone, SharepointConnector, NetworkShareConnector"
* }
* Creates a new Zone.
* @return the created zone
*/
public ISFQuery create(SFZone zone) throws InvalidOrMissingParameterException {
if (zone == null) {
throw new InvalidOrMissingParameterException("zone");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setBody(zone);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Update Zone
* {
* "Name":"Name",
* "HeartbeatTolerance":10,
* "ZoneServices":"StorageZone, SharepointConnector, NetworkShareConnector"
* }
* Updates an existing zone
* @param url
* @param zone
* @return The modified zone
*/
public ISFQuery update(URI url, SFZone zone) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (zone == null) {
throw new InvalidOrMissingParameterException("zone");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addIds(url);
sfApiQuery.setBody(zone);
sfApiQuery.setHttpMethod("PATCH");
return sfApiQuery;
}
/**
* Delete Zone
* Removes an existing zone
* @param url
* @param force (default: false)
* @param newDefaultZoneId (default: null)
*/
public ISFQuery delete(URI url, Boolean force, String newDefaultZoneId) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (force == null) {
throw new InvalidOrMissingParameterException("force");
}
if (newDefaultZoneId == null) {
throw new InvalidOrMissingParameterException("newDefaultZoneId");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("force", force);
sfApiQuery.addQueryString("newDefaultZoneId", newDefaultZoneId);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete Zone
* Removes an existing zone
* @param url
* @param force (default: false)
*/
public ISFQuery delete(URI url, Boolean force) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (force == null) {
throw new InvalidOrMissingParameterException("force");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("force", force);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Delete Zone
* Removes an existing zone
* @param url
*/
public ISFQuery delete(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Reset Zone Secret
* Resets the current Zone Secret to a new Random value
* Caution! This Call will invalidate all Storage Center communications until the Storage Center Zone secret
* is also updated.
* User must be a Zone admin to perform this action
* @param url
* @return The modified Zone object
*/
public ISFQuery resetSecret(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("ResetSecret");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Get the tenants of a multi-tenant zone
* @param parentUrl
* @return List of tenant accounts, not including the zone admin account.
*/
public ISFQuery> getTenants(URI parentUrl) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Tenants");
sfApiQuery.addIds(parentUrl);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Add a tenant account to a multi-tenant zone
* @param parentUrl
* @param accountId
*/
public ISFQuery createTenants(URI parentUrl, String accountId) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (accountId == null) {
throw new InvalidOrMissingParameterException("accountId");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Tenants");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addQueryString("accountId", accountId);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Remove a tenant from a multi-tenant zone
* @param parentUrl
* @param id
* @param newDefaultZoneId
* @param expireItems (default: false)
*/
public ISFQuery deleteTenants(URI parentUrl, String id, String newDefaultZoneId, Boolean expireItems) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
if (newDefaultZoneId == null) {
throw new InvalidOrMissingParameterException("newDefaultZoneId");
}
if (expireItems == null) {
throw new InvalidOrMissingParameterException("expireItems");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Tenants");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addActionIds(id);
sfApiQuery.addQueryString("newDefaultZoneId", newDefaultZoneId);
sfApiQuery.addQueryString("expireItems", expireItems);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Remove a tenant from a multi-tenant zone
* @param parentUrl
* @param id
* @param newDefaultZoneId
*/
public ISFQuery deleteTenants(URI parentUrl, String id, String newDefaultZoneId) throws InvalidOrMissingParameterException {
if (parentUrl == null) {
throw new InvalidOrMissingParameterException("parentUrl");
}
if (id == null) {
throw new InvalidOrMissingParameterException("id");
}
if (newDefaultZoneId == null) {
throw new InvalidOrMissingParameterException("newDefaultZoneId");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Tenants");
sfApiQuery.addIds(parentUrl);
sfApiQuery.addActionIds(id);
sfApiQuery.addQueryString("newDefaultZoneId", newDefaultZoneId);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
/**
* Get Zone Metadata
* Gets metadata associated with the specified zone
* @param url
* @return the zone metadata feed
*/
public ISFQuery> getMetadata(URI url) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setHttpMethod("GET");
return sfApiQuery;
}
/**
* Create or update Zone Metadata
* [
* {"Name":"metadataName1", "Value":"metadataValue1", "IsPublic":"true"},
* {"Name":"metadataName2", "Value":"metadataValue2", "IsPublic":"false"},
* ...
* ]
* Creates or updates Metadata entries associated with the specified zone
* @param url
* @param metadata
* @return the zone metadata feed
*/
public ISFQuery> createMetadata(URI url, ArrayList metadata) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (metadata == null) {
throw new InvalidOrMissingParameterException("metadata");
}
SFApiQuery> sfApiQuery = new SFApiQuery>(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.setBody(metadata);
sfApiQuery.setHttpMethod("POST");
return sfApiQuery;
}
/**
* Delete Zone Metadata
* Delete the Metadata entry associated with the specified zone
* @param url
* @param name
* @return no data on success
*/
public ISFQuery deleteMetadata(URI url, String name) throws InvalidOrMissingParameterException {
if (url == null) {
throw new InvalidOrMissingParameterException("url");
}
if (name == null) {
throw new InvalidOrMissingParameterException("name");
}
SFApiQuery sfApiQuery = new SFApiQuery(this.client);
sfApiQuery.setFrom("Zones");
sfApiQuery.setAction("Metadata");
sfApiQuery.addIds(url);
sfApiQuery.addQueryString("name", name);
sfApiQuery.setHttpMethod("DELETE");
return sfApiQuery;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy