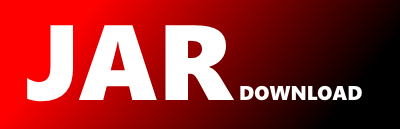
com.citrix.sharefile.api.interfaces.ISFQuery Maven / Gradle / Ivy
package com.citrix.sharefile.api.interfaces;
import com.citrix.sharefile.api.constants.SFKeywords;
import com.citrix.sharefile.api.enumerations.SFSafeEnum;
import com.citrix.sharefile.api.enumerations.SFV3ElementType;
import com.citrix.sharefile.api.exceptions.SFInvalidStateException;
import com.citrix.sharefile.api.exceptions.SFNotAuthorizedException;
import com.citrix.sharefile.api.exceptions.SFOAuthTokenRenewException;
import com.citrix.sharefile.api.exceptions.SFOtherException;
import com.citrix.sharefile.api.exceptions.SFServerException;
import com.citrix.sharefile.api.models.SFODataObject;
import java.io.UnsupportedEncodingException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
public interface ISFQuery
{
ISFQuery setApiClient(ISFApiClient apiClient);
ISFQuery setFrom(String string);
ISFQuery setHttpMethod(String string);
ISFQuery addIds(URI url);
ISFQuery setAction(String string);
ISFQuery setBody(SFODataObject sfoDataObject);
ISFQuery setBody(ArrayList> sfoDataObjectsFeed);
ISFQuery setBody(Object object);
ISFQuery addQueryString(String string, Object type);
ISFQuery addActionIds(String id);
ISFQuery addActionIds(Integer id);
ISFQuery addQueryString(String string, ArrayList ids);
ISFQuery addSubAction(String string);
ISFQuery addSubAction(String subaction, SFSafeEnum extras);
URI getLink();
String getUserName();
String getPassword();
String getHttpMethod();
String getBody();
boolean constructDownloadSpec();
String buildQueryUrlString(String server) throws UnsupportedEncodingException;
ISFQuery setLink(String string) throws URISyntaxException;
/**
* This implies that the query parameters need to be appended by the buildQuery
* function before executing the query.
*/
ISFQuery setLink(URI uri);
/**
* This implies that the query parameters are included in the URI and no more parameters more
* needs to be added before executing the query.
* Generally we get such URI from Redirection object.
*/
ISFQuery setFullyParametrizedLink(URI uri);
boolean canReNewTokenInternally();
ISFQuery setCredentials(String userName, String password);
/**
* For certain calls like create symbolic link we want to disable readahead done by the
* SDK. This function allows to set the flag to explicity false if required..
*/
ISFQuery allowRedirection(boolean value);
boolean reDirectionAllowed();
ISFQuery expect(Class clazz);
/**
* This will append the query paremeters from previuos query to the new link. use this only
* when re-executing the query for a redirected object.
* Also , this will ignore the previous params if new query already has some params
* @throws URISyntaxException
* @throws UnsupportedEncodingException
*/
ISFQuery setLinkAndAppendPreviousParameters(URI uri) throws URISyntaxException, UnsupportedEncodingException;
/**
* This will append the query paremeters from previuos query to the new link. use this only
* when re-executing the query for a redirected object.
* Also , this will ignore the previous params if new query already has some params
* @throws URISyntaxException
* @throws UnsupportedEncodingException
*/
ISFQuery setLinkAndAppendPreviousParameters(String string) throws URISyntaxException, UnsupportedEncodingException;;
/**
* simplifies the adding of expansion parameters to the query.
*/
ISFQuery expand(String expansionParameter);
ISFQuery top(int topItems);
ISFQuery skip(int skipItems);
ISFQuery orderBy(String orderParameter,SFKeywords.DIRECTION direction);
ISFQuery filter(String filterValue);
ISFQuery is(SFV3ElementType type);
ISFQuery select(String name);
/**
This function takes any uri and stores only its base part along with the provider
example if you pass: https://szqatest2.sharefiletest.com/cifs/v3/Capabilities
this function will store baseLink as : https://szqatest2.sharefiletest.com/cifs/v3/
*/
ISFQuery setBaseLink(URI uri) throws URISyntaxException;
T execute() throws SFInvalidStateException, SFServerException,
SFNotAuthorizedException,SFOAuthTokenRenewException, SFOtherException;
void executeAsync(ISFApiResultCallback callback);
/**
* This will allow the consumer apps to preserve identification information related to queries.
* The SDK itself never uses this.
*/
void setTag(Object tag);
/**
* Get tag set by the setTag function.
*/
Object getTag();
String getStringResponse();
void setStringResponse(String response);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy