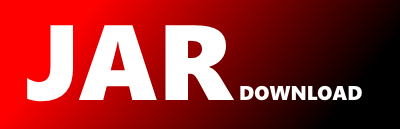
com.citrix.sharefile.api.models.SFAccessControl Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFAccessControl extends SFODataObject {
@SerializedName("Item")
private SFItem Item;
@SerializedName("Principal")
private SFPrincipal Principal;
@SerializedName("CanUpload")
private Boolean CanUpload;
@SerializedName("CanDownload")
private Boolean CanDownload;
@SerializedName("CanView")
private Boolean CanView;
@SerializedName("CanDelete")
private Boolean CanDelete;
@SerializedName("CanManagePermissions")
private Boolean CanManagePermissions;
@SerializedName("NotifyOnUpload")
private Boolean NotifyOnUpload;
@SerializedName("NotifyOnDownload")
private Boolean NotifyOnDownload;
@SerializedName("IsOwner")
private Boolean IsOwner;
/**
* Item that was given permission through this rule
*/
public SFItem getItem() {
return this.Item;
}
/**
* Item that was given permission through this rule
*/
public void setItem(SFItem item) {
this.Item = item;
}
/**
* Principal - User or Group - that has been granted permissions through this rule
*/
public SFPrincipal getPrincipal() {
return this.Principal;
}
/**
* Principal - User or Group - that has been granted permissions through this rule
*/
public void setPrincipal(SFPrincipal principal) {
this.Principal = principal;
}
/**
* Defines whether the principal can add files (upload) into the Item
*/
public Boolean getCanUpload() {
return this.CanUpload;
}
/**
* Defines whether the principal can add files (upload) into the Item
*/
public void setCanUpload(Boolean canupload) {
this.CanUpload = canupload;
}
/**
* Defines whether the principal can read file content (download) from this Item
*/
public Boolean getCanDownload() {
return this.CanDownload;
}
/**
* Defines whether the principal can read file content (download) from this Item
*/
public void setCanDownload(Boolean candownload) {
this.CanDownload = candownload;
}
/**
* Defines whether the principal can view items (browse) from this Item
*/
public Boolean getCanView() {
return this.CanView;
}
/**
* Defines whether the principal can view items (browse) from this Item
*/
public void setCanView(Boolean canview) {
this.CanView = canview;
}
/**
* Defines whether the principal can remove items from this Item
*/
public Boolean getCanDelete() {
return this.CanDelete;
}
/**
* Defines whether the principal can remove items from this Item
*/
public void setCanDelete(Boolean candelete) {
this.CanDelete = candelete;
}
/**
* Defines whether the principal can configure Access Controls in this Item
*/
public Boolean getCanManagePermissions() {
return this.CanManagePermissions;
}
/**
* Defines whether the principal can configure Access Controls in this Item
*/
public void setCanManagePermissions(Boolean canmanagepermissions) {
this.CanManagePermissions = canmanagepermissions;
}
/**
* Defines the notification preference for upload events. If set, the principal will receive
* notifications when new files are uploaded into this Item
*/
public Boolean getNotifyOnUpload() {
return this.NotifyOnUpload;
}
/**
* Defines the notification preference for upload events. If set, the principal will receive
* notifications when new files are uploaded into this Item
*/
public void setNotifyOnUpload(Boolean notifyonupload) {
this.NotifyOnUpload = notifyonupload;
}
/**
* Defines the notification preference for download events. If set, the principal will receive
* notifiation when items are downloaded from this Item.
*/
public Boolean getNotifyOnDownload() {
return this.NotifyOnDownload;
}
/**
* Defines the notification preference for download events. If set, the principal will receive
* notifiation when items are downloaded from this Item.
*/
public void setNotifyOnDownload(Boolean notifyondownload) {
this.NotifyOnDownload = notifyondownload;
}
/**
* Defines whether the principal is the owner of this Item
*/
public Boolean getIsOwner() {
return this.IsOwner;
}
/**
* Defines whether the principal is the owner of this Item
*/
public void setIsOwner(Boolean isowner) {
this.IsOwner = isowner;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy