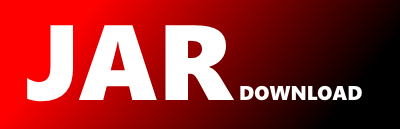
com.citrix.sharefile.api.models.SFAsyncOperation Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFAsyncOperation extends SFODataObject {
@SerializedName("Operation")
private SFSafeEnum Operation;
@SerializedName("Account")
private SFAccount Account;
@SerializedName("AuthorityZone")
private SFZone AuthorityZone;
@SerializedName("Source")
private SFItem Source;
@SerializedName("User")
private SFUser User;
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("State")
private SFSafeEnum State;
@SerializedName("UpdateDate")
private Date UpdateDate;
@SerializedName("Target")
private SFItem Target;
@SerializedName("BatchID")
private String BatchID;
@SerializedName("BatchSourceID")
private String BatchSourceID;
@SerializedName("BatchTargetID")
private String BatchTargetID;
@SerializedName("BatchProgress")
private Double BatchProgress;
@SerializedName("BatchState")
private SFSafeEnum BatchState;
@SerializedName("BatchTotal")
private Integer BatchTotal;
/**
* Operation type
*/
public SFSafeEnum getOperation() {
return this.Operation;
}
/**
* Operation type
*/
public void setOperation(SFSafeEnum operation) {
this.Operation = operation;
}
/**
* ShareFile Account
*/
public SFAccount getAccount() {
return this.Account;
}
/**
* ShareFile Account
*/
public void setAccount(SFAccount account) {
this.Account = account;
}
/**
* Represents the Zone that is driving the asynchronous operation process. It
* may be null if the operation is driven by the control plane.
*/
public SFZone getAuthorityZone() {
return this.AuthorityZone;
}
/**
* Represents the Zone that is driving the asynchronous operation process. It
* may be null if the operation is driven by the control plane.
*/
public void setAuthorityZone(SFZone authorityzone) {
this.AuthorityZone = authorityzone;
}
/**
* Source Item for the operation.
*/
public SFItem getSource() {
return this.Source;
}
/**
* Source Item for the operation.
*/
public void setSource(SFItem source) {
this.Source = source;
}
/**
* User that initiated the operation
*/
public SFUser getUser() {
return this.User;
}
/**
* User that initiated the operation
*/
public void setUser(SFUser user) {
this.User = user;
}
/**
* Operation creation date
*/
public Date getCreationDate() {
return this.CreationDate;
}
/**
* Operation creation date
*/
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
/**
* Operation state. States 'Created' and 'Scheduled' indicate the operation is
* in progress; States 'Success' and 'Failure' indicate the operatoin is finalized
*/
public SFSafeEnum getState() {
return this.State;
}
/**
* Operation state. States 'Created' and 'Scheduled' indicate the operation is
* in progress; States 'Success' and 'Failure' indicate the operatoin is finalized
*/
public void setState(SFSafeEnum state) {
this.State = state;
}
/**
* Last time the operation state was modified
*/
public Date getUpdateDate() {
return this.UpdateDate;
}
/**
* Last time the operation state was modified
*/
public void setUpdateDate(Date updatedate) {
this.UpdateDate = updatedate;
}
/**
* Target Item for the operation.
*/
public SFItem getTarget() {
return this.Target;
}
/**
* Target Item for the operation.
*/
public void setTarget(SFItem target) {
this.Target = target;
}
/**
* Batch Identifier for an asynchronous operation that includes multiple Items - for
* example, a recursive Copy will create a single AsyncOp instance per file, all sharing
* the same BatchID
*/
public String getBatchID() {
return this.BatchID;
}
/**
* Batch Identifier for an asynchronous operation that includes multiple Items - for
* example, a recursive Copy will create a single AsyncOp instance per file, all sharing
* the same BatchID
*/
public void setBatchID(String batchid) {
this.BatchID = batchid;
}
/**
* Item ID used as source for the Batch operation
*/
public String getBatchSourceID() {
return this.BatchSourceID;
}
/**
* Item ID used as source for the Batch operation
*/
public void setBatchSourceID(String batchsourceid) {
this.BatchSourceID = batchsourceid;
}
/**
* Item ID used as target for the Batch operation
*/
public String getBatchTargetID() {
return this.BatchTargetID;
}
/**
* Item ID used as target for the Batch operation
*/
public void setBatchTargetID(String batchtargetid) {
this.BatchTargetID = batchtargetid;
}
/**
* BatchProgress indicates the progress of the Batch operation
*/
public Double getBatchProgress() {
return this.BatchProgress;
}
/**
* BatchProgress indicates the progress of the Batch operation
*/
public void setBatchProgress(Double batchprogress) {
this.BatchProgress = batchprogress;
}
/**
* Batch Operation state. State 'Scheduled' indicate the operation is
* in progress; States 'Success' and 'Failure' indicate the operation is finalized
*/
public SFSafeEnum getBatchState() {
return this.BatchState;
}
/**
* Batch Operation state. State 'Scheduled' indicate the operation is
* in progress; States 'Success' and 'Failure' indicate the operation is finalized
*/
public void setBatchState(SFSafeEnum batchstate) {
this.BatchState = batchstate;
}
public Integer getBatchTotal() {
return this.BatchTotal;
}
public void setBatchTotal(Integer batchtotal) {
this.BatchTotal = batchtotal;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy