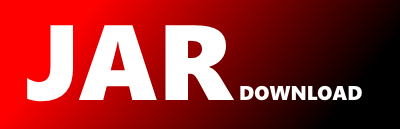
com.citrix.sharefile.api.models.SFBillingAddOn Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFBillingAddOn extends SFODataObject {
@SerializedName("Account")
private SFAccount Account;
@SerializedName("PlanAddOnsTypeCode")
private String PlanAddOnsTypeCode;
@SerializedName("PlanAddOnsCode")
private String PlanAddOnsCode;
@SerializedName("UnitPrice")
private String UnitPrice;
@SerializedName("LimitingFactor")
private String LimitingFactor;
@SerializedName("LowerLimit")
private Integer LowerLimit;
@SerializedName("UpperLimit")
private Integer UpperLimit;
@SerializedName("AddonField")
private String AddonField;
@SerializedName("IncreaseInterval")
private Integer IncreaseInterval;
@SerializedName("CreatedBy")
private SFUser CreatedBy;
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("UpdatedBy")
private SFUser UpdatedBy;
@SerializedName("UpdatedDate")
private Date UpdatedDate;
@SerializedName("PurchaseDate")
private Date PurchaseDate;
@SerializedName("ExpirationDate")
private Date ExpirationDate;
@SerializedName("IsActive")
private Boolean IsActive;
@SerializedName("NumberofLicenses")
private Integer NumberofLicenses;
@SerializedName("PlanAddonBundleId")
private Integer PlanAddonBundleId;
@SerializedName("ProductCodeName")
private String ProductCodeName;
public SFAccount getAccount() {
return this.Account;
}
public void setAccount(SFAccount account) {
this.Account = account;
}
public String getPlanAddOnsTypeCode() {
return this.PlanAddOnsTypeCode;
}
public void setPlanAddOnsTypeCode(String planaddonstypecode) {
this.PlanAddOnsTypeCode = planaddonstypecode;
}
public String getPlanAddOnsCode() {
return this.PlanAddOnsCode;
}
public void setPlanAddOnsCode(String planaddonscode) {
this.PlanAddOnsCode = planaddonscode;
}
public String getUnitPrice() {
return this.UnitPrice;
}
public void setUnitPrice(String unitprice) {
this.UnitPrice = unitprice;
}
public String getLimitingFactor() {
return this.LimitingFactor;
}
public void setLimitingFactor(String limitingfactor) {
this.LimitingFactor = limitingfactor;
}
public Integer getLowerLimit() {
return this.LowerLimit;
}
public void setLowerLimit(Integer lowerlimit) {
this.LowerLimit = lowerlimit;
}
public Integer getUpperLimit() {
return this.UpperLimit;
}
public void setUpperLimit(Integer upperlimit) {
this.UpperLimit = upperlimit;
}
public String getAddonField() {
return this.AddonField;
}
public void setAddonField(String addonfield) {
this.AddonField = addonfield;
}
public Integer getIncreaseInterval() {
return this.IncreaseInterval;
}
public void setIncreaseInterval(Integer increaseinterval) {
this.IncreaseInterval = increaseinterval;
}
public SFUser getCreatedBy() {
return this.CreatedBy;
}
public void setCreatedBy(SFUser createdby) {
this.CreatedBy = createdby;
}
public Date getCreationDate() {
return this.CreationDate;
}
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
public SFUser getUpdatedBy() {
return this.UpdatedBy;
}
public void setUpdatedBy(SFUser updatedby) {
this.UpdatedBy = updatedby;
}
public Date getUpdatedDate() {
return this.UpdatedDate;
}
public void setUpdatedDate(Date updateddate) {
this.UpdatedDate = updateddate;
}
public Date getPurchaseDate() {
return this.PurchaseDate;
}
public void setPurchaseDate(Date purchasedate) {
this.PurchaseDate = purchasedate;
}
public Date getExpirationDate() {
return this.ExpirationDate;
}
public void setExpirationDate(Date expirationdate) {
this.ExpirationDate = expirationdate;
}
public Boolean getIsActive() {
return this.IsActive;
}
public void setIsActive(Boolean isactive) {
this.IsActive = isactive;
}
public Integer getNumberofLicenses() {
return this.NumberofLicenses;
}
public void setNumberofLicenses(Integer numberoflicenses) {
this.NumberofLicenses = numberoflicenses;
}
public Integer getPlanAddonBundleId() {
return this.PlanAddonBundleId;
}
public void setPlanAddonBundleId(Integer planaddonbundleid) {
this.PlanAddonBundleId = planaddonbundleid;
}
/**
* Name of the product, e.g, RightSignature, ShareConnect, etc.
*/
public String getProductCodeName() {
return this.ProductCodeName;
}
/**
* Name of the product, e.g, RightSignature, ShareConnect, etc.
*/
public void setProductCodeName(String productcodename) {
this.ProductCodeName = productcodename;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy