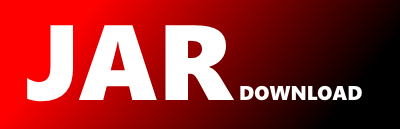
com.citrix.sharefile.api.models.SFCreditCard Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFCreditCard extends SFODataObject {
@SerializedName("FirstName")
private String FirstName;
@SerializedName("LastName")
private String LastName;
@SerializedName("CardType")
private String CardType;
@SerializedName("Number")
private String Number;
@SerializedName("Token")
private String Token;
@SerializedName("ExpirationMonth")
private String ExpirationMonth;
@SerializedName("ExpirationYear")
private String ExpirationYear;
@SerializedName("SecurityCode")
private String SecurityCode;
@SerializedName("BillingAddress")
private SFAddress BillingAddress;
/**
* First Name on the Credit Card
*/
public String getFirstName() {
return this.FirstName;
}
/**
* First Name on the Credit Card
*/
public void setFirstName(String firstname) {
this.FirstName = firstname;
}
/**
* Last Name on the Credit Card
*/
public String getLastName() {
return this.LastName;
}
/**
* Last Name on the Credit Card
*/
public void setLastName(String lastname) {
this.LastName = lastname;
}
/**
* Visa, MasterCard, Discover, etc.
*/
public String getCardType() {
return this.CardType;
}
/**
* Visa, MasterCard, Discover, etc.
*/
public void setCardType(String cardtype) {
this.CardType = cardtype;
}
/**
* Credit Card Number
*/
public String getNumber() {
return this.Number;
}
/**
* Credit Card Number
*/
public void setNumber(String number) {
this.Number = number;
}
/**
* The Card Number, tokenized
*/
public String getToken() {
return this.Token;
}
/**
* The Card Number, tokenized
*/
public void setToken(String token) {
this.Token = token;
}
/**
* Expiration Month's number, 1-12
*/
public String getExpirationMonth() {
return this.ExpirationMonth;
}
/**
* Expiration Month's number, 1-12
*/
public void setExpirationMonth(String expirationmonth) {
this.ExpirationMonth = expirationmonth;
}
/**
* Expiration Year, in YYYY format
*/
public String getExpirationYear() {
return this.ExpirationYear;
}
/**
* Expiration Year, in YYYY format
*/
public void setExpirationYear(String expirationyear) {
this.ExpirationYear = expirationyear;
}
/**
* Security Code in the back of the card
*/
public String getSecurityCode() {
return this.SecurityCode;
}
/**
* Security Code in the back of the card
*/
public void setSecurityCode(String securitycode) {
this.SecurityCode = securitycode;
}
/**
* Billing Address
*/
public SFAddress getBillingAddress() {
return this.BillingAddress;
}
/**
* Billing Address
*/
public void setBillingAddress(SFAddress billingaddress) {
this.BillingAddress = billingaddress;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy