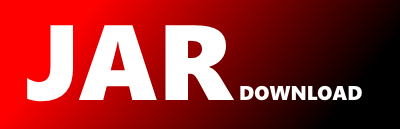
com.citrix.sharefile.api.models.SFDeviceUser Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFDeviceUser extends SFODataObject {
@SerializedName("Account")
private SFAccount Account;
@SerializedName("User")
private SFUser User;
@SerializedName("Device")
private SFDevice Device;
@SerializedName("IsOwner")
private Boolean IsOwner;
@SerializedName("FriendlyName")
private String FriendlyName;
@SerializedName("Wipe")
private Boolean Wipe;
@SerializedName("IsLocked")
private Boolean IsLocked;
@SerializedName("IsDeleted")
private Boolean IsDeleted;
@SerializedName("Created")
private Date Created;
@SerializedName("LastLogin")
private Date LastLogin;
@SerializedName("LastWipe")
private Date LastWipe;
@SerializedName("WipeToken")
private String WipeToken;
@SerializedName("WipeInitiator")
private String WipeInitiator;
@SerializedName("WipeInitiatorRole")
private SFSafeEnum WipeInitiatorRole;
@SerializedName("LockInitiator")
private String LockInitiator;
@SerializedName("LockInitiatorRole")
private SFSafeEnum LockInitiatorRole;
@SerializedName("DeviceType")
private SFSafeEnum DeviceType;
@SerializedName("LastErrorMessage")
private String LastErrorMessage;
public SFAccount getAccount() {
return this.Account;
}
public void setAccount(SFAccount account) {
this.Account = account;
}
public SFUser getUser() {
return this.User;
}
public void setUser(SFUser user) {
this.User = user;
}
public SFDevice getDevice() {
return this.Device;
}
public void setDevice(SFDevice device) {
this.Device = device;
}
public Boolean getIsOwner() {
return this.IsOwner;
}
public void setIsOwner(Boolean isowner) {
this.IsOwner = isowner;
}
public String getFriendlyName() {
return this.FriendlyName;
}
public void setFriendlyName(String friendlyname) {
this.FriendlyName = friendlyname;
}
public Boolean getWipe() {
return this.Wipe;
}
public void setWipe(Boolean wipe) {
this.Wipe = wipe;
}
public Boolean getIsLocked() {
return this.IsLocked;
}
public void setIsLocked(Boolean islocked) {
this.IsLocked = islocked;
}
public Boolean getIsDeleted() {
return this.IsDeleted;
}
public void setIsDeleted(Boolean isdeleted) {
this.IsDeleted = isdeleted;
}
public Date getCreated() {
return this.Created;
}
public void setCreated(Date created) {
this.Created = created;
}
public Date getLastLogin() {
return this.LastLogin;
}
public void setLastLogin(Date lastlogin) {
this.LastLogin = lastlogin;
}
public Date getLastWipe() {
return this.LastWipe;
}
public void setLastWipe(Date lastwipe) {
this.LastWipe = lastwipe;
}
public String getWipeToken() {
return this.WipeToken;
}
public void setWipeToken(String wipetoken) {
this.WipeToken = wipetoken;
}
public String getWipeInitiator() {
return this.WipeInitiator;
}
public void setWipeInitiator(String wipeinitiator) {
this.WipeInitiator = wipeinitiator;
}
public SFSafeEnum getWipeInitiatorRole() {
return this.WipeInitiatorRole;
}
public void setWipeInitiatorRole(SFSafeEnum wipeinitiatorrole) {
this.WipeInitiatorRole = wipeinitiatorrole;
}
public String getLockInitiator() {
return this.LockInitiator;
}
public void setLockInitiator(String lockinitiator) {
this.LockInitiator = lockinitiator;
}
public SFSafeEnum getLockInitiatorRole() {
return this.LockInitiatorRole;
}
public void setLockInitiatorRole(SFSafeEnum lockinitiatorrole) {
this.LockInitiatorRole = lockinitiatorrole;
}
public SFSafeEnum getDeviceType() {
return this.DeviceType;
}
public void setDeviceType(SFSafeEnum devicetype) {
this.DeviceType = devicetype;
}
public String getLastErrorMessage() {
return this.LastErrorMessage;
}
public void setLastErrorMessage(String lasterrormessage) {
this.LastErrorMessage = lasterrormessage;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy