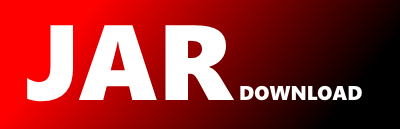
com.citrix.sharefile.api.models.SFEmailPluginSettings Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFEmailPluginSettings extends SFODataObject {
@SerializedName("AttachmentConversion")
private SFEditingProhibitedDefaultSetting AttachmentConversion;
@SerializedName("ShouldAttachPaperClip")
private SFEditingProhibitedDefaultSetting ShouldAttachPaperClip;
@SerializedName("FileAndLinkExpiration")
private SFEditingProhibitedDefaultSetting FileAndLinkExpiration;
@SerializedName("LinkInsertionMethod")
private SFEditingProhibitedDefaultSetting LinkInsertionMethod;
@SerializedName("BannerCustomization")
private SFEditingProhibitedDefaultSetting BannerCustomization;
@SerializedName("DownloadFilesText")
private SFEditingProhibitedDefaultSetting DownloadFilesText;
@SerializedName("RequestFilesText")
private SFEditingProhibitedDefaultSetting RequestFilesText;
@SerializedName("DownloadInfoLevel")
private SFEditingProhibitedDefaultSetting DownloadInfoLevel;
@SerializedName("ShouldNotifyOnDownload")
private SFEditingProhibitedDefaultSetting ShouldNotifyOnDownload;
@SerializedName("MaxDownloads")
private SFEditingProhibitedDefaultSetting MaxDownloads;
@SerializedName("UploadInfoLevel")
private SFEditingProhibitedDefaultSetting UploadInfoLevel;
@SerializedName("ShouldNotifyOnUpload")
private SFEditingProhibitedDefaultSetting ShouldNotifyOnUpload;
@SerializedName("EncryptedEmailAccessInfoLevel")
private SFEditingProhibitedDefaultSetting EncryptedEmailAccessInfoLevel;
@SerializedName("ShouldNotifyOnEncryptedEmailRead")
private SFEditingProhibitedDefaultSetting ShouldNotifyOnEncryptedEmailRead;
@SerializedName("EncryptedEmailExpiration")
private SFEditingProhibitedDefaultSetting EncryptedEmailExpiration;
/**
* Describes if any attachments added to an email will be auto converted to a Sharefile file. Values include Automatic, Always, Advanced.
* For Automatic, a size parameter can be set, measuring the smallest a file size in MB will be converted.
*/
public SFEditingProhibitedDefaultSetting getAttachmentConversion() {
return this.AttachmentConversion;
}
/**
* Describes if any attachments added to an email will be auto converted to a Sharefile file. Values include Automatic, Always, Advanced.
* For Automatic, a size parameter can be set, measuring the smallest a file size in MB will be converted.
*/
public void setAttachmentConversion(SFEditingProhibitedDefaultSetting attachmentconversion) {
this.AttachmentConversion = attachmentconversion;
}
/**
* Enable/Disable the paperclip icon on emails identifying the email has attachments.
*/
public SFEditingProhibitedDefaultSetting getShouldAttachPaperClip() {
return this.ShouldAttachPaperClip;
}
/**
* Enable/Disable the paperclip icon on emails identifying the email has attachments.
*/
public void setShouldAttachPaperClip(SFEditingProhibitedDefaultSetting shouldattachpaperclip) {
this.ShouldAttachPaperClip = shouldattachpaperclip;
}
/**
* By default, files and links will be available for 6 months. This setting will override that.
*/
public SFEditingProhibitedDefaultSetting getFileAndLinkExpiration() {
return this.FileAndLinkExpiration;
}
/**
* By default, files and links will be available for 6 months. This setting will override that.
*/
public void setFileAndLinkExpiration(SFEditingProhibitedDefaultSetting fileandlinkexpiration) {
this.FileAndLinkExpiration = fileandlinkexpiration;
}
/**
* Setting to determine how to display the link to the files. Values are Banner or TextLink.
*/
public SFEditingProhibitedDefaultSetting getLinkInsertionMethod() {
return this.LinkInsertionMethod;
}
/**
* Setting to determine how to display the link to the files. Values are Banner or TextLink.
*/
public void setLinkInsertionMethod(SFEditingProhibitedDefaultSetting linkinsertionmethod) {
this.LinkInsertionMethod = linkinsertionmethod;
}
/**
* Settings to customize the banner for displaying file links
*/
public SFEditingProhibitedDefaultSetting getBannerCustomization() {
return this.BannerCustomization;
}
/**
* Settings to customize the banner for displaying file links
*/
public void setBannerCustomization(SFEditingProhibitedDefaultSetting bannercustomization) {
this.BannerCustomization = bannercustomization;
}
/**
* The text to display for the Download Files link. Must contain two # symbols to identify that actual linked section of text.
*/
public SFEditingProhibitedDefaultSetting getDownloadFilesText() {
return this.DownloadFilesText;
}
/**
* The text to display for the Download Files link. Must contain two # symbols to identify that actual linked section of text.
*/
public void setDownloadFilesText(SFEditingProhibitedDefaultSetting downloadfilestext) {
this.DownloadFilesText = downloadfilestext;
}
/**
* The text to display for the Request Files link. Must contain two # symbols to identify that actual linked section of text.
*/
public SFEditingProhibitedDefaultSetting getRequestFilesText() {
return this.RequestFilesText;
}
/**
* The text to display for the Request Files link. Must contain two # symbols to identify that actual linked section of text.
*/
public void setRequestFilesText(SFEditingProhibitedDefaultSetting requestfilestext) {
this.RequestFilesText = requestfilestext;
}
/**
* Setting to determine the access level of the downloaded files.
*/
public SFEditingProhibitedDefaultSetting getDownloadInfoLevel() {
return this.DownloadInfoLevel;
}
/**
* Setting to determine the access level of the downloaded files.
*/
public void setDownloadInfoLevel(SFEditingProhibitedDefaultSetting downloadinfolevel) {
this.DownloadInfoLevel = downloadinfolevel;
}
/**
* Setting to enable/disable sending email notifications upon file download.
*/
public SFEditingProhibitedDefaultSetting getShouldNotifyOnDownload() {
return this.ShouldNotifyOnDownload;
}
/**
* Setting to enable/disable sending email notifications upon file download.
*/
public void setShouldNotifyOnDownload(SFEditingProhibitedDefaultSetting shouldnotifyondownload) {
this.ShouldNotifyOnDownload = shouldnotifyondownload;
}
/**
* Number of downloads available for each file.
*/
public SFEditingProhibitedDefaultSetting getMaxDownloads() {
return this.MaxDownloads;
}
/**
* Number of downloads available for each file.
*/
public void setMaxDownloads(SFEditingProhibitedDefaultSetting maxdownloads) {
this.MaxDownloads = maxdownloads;
}
/**
* Setting to determine the access level required to upload files.
*/
public SFEditingProhibitedDefaultSetting getUploadInfoLevel() {
return this.UploadInfoLevel;
}
/**
* Setting to determine the access level required to upload files.
*/
public void setUploadInfoLevel(SFEditingProhibitedDefaultSetting uploadinfolevel) {
this.UploadInfoLevel = uploadinfolevel;
}
/**
* Setting to enable/disable sending email notifications upon file upload.
*/
public SFEditingProhibitedDefaultSetting getShouldNotifyOnUpload() {
return this.ShouldNotifyOnUpload;
}
/**
* Setting to enable/disable sending email notifications upon file upload.
*/
public void setShouldNotifyOnUpload(SFEditingProhibitedDefaultSetting shouldnotifyonupload) {
this.ShouldNotifyOnUpload = shouldnotifyonupload;
}
/**
* Setting to determine the access level required to view encrypted email.
*/
public SFEditingProhibitedDefaultSetting getEncryptedEmailAccessInfoLevel() {
return this.EncryptedEmailAccessInfoLevel;
}
/**
* Setting to determine the access level required to view encrypted email.
*/
public void setEncryptedEmailAccessInfoLevel(SFEditingProhibitedDefaultSetting encryptedemailaccessinfolevel) {
this.EncryptedEmailAccessInfoLevel = encryptedemailaccessinfolevel;
}
/**
* Setting to enable/disable sending email notifications upon view of encrypted email.
*/
public SFEditingProhibitedDefaultSetting getShouldNotifyOnEncryptedEmailRead() {
return this.ShouldNotifyOnEncryptedEmailRead;
}
/**
* Setting to enable/disable sending email notifications upon view of encrypted email.
*/
public void setShouldNotifyOnEncryptedEmailRead(SFEditingProhibitedDefaultSetting shouldnotifyonencryptedemailread) {
this.ShouldNotifyOnEncryptedEmailRead = shouldnotifyonencryptedemailread;
}
/**
* By default, encrypted emails will be available for 6 months. This setting will override that.
*/
public SFEditingProhibitedDefaultSetting getEncryptedEmailExpiration() {
return this.EncryptedEmailExpiration;
}
/**
* By default, encrypted emails will be available for 6 months. This setting will override that.
*/
public void setEncryptedEmailExpiration(SFEditingProhibitedDefaultSetting encryptedemailexpiration) {
this.EncryptedEmailExpiration = encryptedemailexpiration;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy