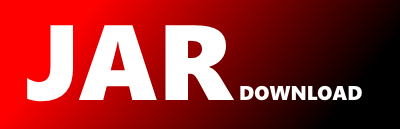
com.citrix.sharefile.api.models.SFEncryptedEmail Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFEncryptedEmail extends SFODataObject {
@SerializedName("Subject")
private String Subject;
@SerializedName("ToRecipients")
private ArrayList ToRecipients;
@SerializedName("CcRecipients")
private ArrayList CcRecipients;
@SerializedName("BccRecipients")
private ArrayList BccRecipients;
@SerializedName("OriginalEncryptedEmail")
private SFEncryptedEmail OriginalEncryptedEmail;
@SerializedName("InReplyTo")
private SFEncryptedEmail InReplyTo;
@SerializedName("Sender")
private SFUser Sender;
@SerializedName("Share")
private SFShare Share;
@SerializedName("ShareAlias")
private SFShareAlias ShareAlias;
@SerializedName("Uri")
private URI Uri;
@SerializedName("SentDate")
private Date SentDate;
/**
* Message subject
*/
public String getSubject() {
return this.Subject;
}
/**
* Message subject
*/
public void setSubject(String subject) {
this.Subject = subject;
}
/**
* Direct To recipients
*/
public ArrayList getToRecipients() {
return this.ToRecipients;
}
/**
* Direct To recipients
*/
public void setToRecipients(ArrayList torecipients) {
this.ToRecipients = torecipients;
}
/**
* Copied recipients
*/
public ArrayList getCcRecipients() {
return this.CcRecipients;
}
/**
* Copied recipients
*/
public void setCcRecipients(ArrayList ccrecipients) {
this.CcRecipients = ccrecipients;
}
/**
* Only available to the sender.
*/
public ArrayList getBccRecipients() {
return this.BccRecipients;
}
/**
* Only available to the sender.
*/
public void setBccRecipients(ArrayList bccrecipients) {
this.BccRecipients = bccrecipients;
}
/**
* Original encrypted email.
*/
public SFEncryptedEmail getOriginalEncryptedEmail() {
return this.OriginalEncryptedEmail;
}
/**
* Original encrypted email.
*/
public void setOriginalEncryptedEmail(SFEncryptedEmail originalencryptedemail) {
this.OriginalEncryptedEmail = originalencryptedemail;
}
/**
* Encrypted email that this message is in reply to.
*/
public SFEncryptedEmail getInReplyTo() {
return this.InReplyTo;
}
/**
* Encrypted email that this message is in reply to.
*/
public void setInReplyTo(SFEncryptedEmail inreplyto) {
this.InReplyTo = inreplyto;
}
/**
* Sender of the encrypted email
*/
public SFUser getSender() {
return this.Sender;
}
/**
* Sender of the encrypted email
*/
public void setSender(SFUser sender) {
this.Sender = sender;
}
/**
* Only available to the sender.
*/
public SFShare getShare() {
return this.Share;
}
/**
* Only available to the sender.
*/
public void setShare(SFShare share) {
this.Share = share;
}
/**
* Used to access the attachments. Only available for a recipient.
*/
public SFShareAlias getShareAlias() {
return this.ShareAlias;
}
/**
* Used to access the attachments. Only available for a recipient.
*/
public void setShareAlias(SFShareAlias sharealias) {
this.ShareAlias = sharealias;
}
/**
* Link to view the email in the web portal.
*/
public URI getUri() {
return this.Uri;
}
/**
* Link to view the email in the web portal.
*/
public void setUri(URI uri) {
this.Uri = uri;
}
/**
* Date the encrypted email was sent
*/
public Date getSentDate() {
return this.SentDate;
}
/**
* Date the encrypted email was sent
*/
public void setSentDate(Date sentdate) {
this.SentDate = sentdate;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy