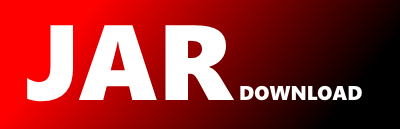
com.citrix.sharefile.api.models.SFFile Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFFile extends SFItem {
@SerializedName("FilePath")
private String FilePath;
@SerializedName("Hash")
private String Hash;
@SerializedName("HasPreview")
private Boolean HasPreview;
@SerializedName("VirusStatus")
private SFSafeEnum VirusStatus;
@SerializedName("DlpInfo")
private SFItemDlpInfo DlpInfo;
@SerializedName("Info")
private SFItemInfo Info;
@SerializedName("LockedBy")
private SFUser LockedBy;
@SerializedName("FileLockInfo")
private SFFileLock FileLockInfo;
@SerializedName("Version")
private Float Version;
@SerializedName("ESignatureDocument")
private SFESignature ESignatureDocument;
/**
* Represents the Object Storage Identifier for this File. This field is
* used in Object Storage providers - including sharefile.com and Storage Zones. Other
* providers like CIFS and SharePoint do not need external references for object
* blobs and do not populate this field.
*/
public String getFilePath() {
return this.FilePath;
}
/**
* Represents the Object Storage Identifier for this File. This field is
* used in Object Storage providers - including sharefile.com and Storage Zones. Other
* providers like CIFS and SharePoint do not need external references for object
* blobs and do not populate this field.
*/
public void setFilePath(String filepath) {
this.FilePath = filepath;
}
/**
* MD5 Hash of the File contents.
*/
public String getHash() {
return this.Hash;
}
/**
* MD5 Hash of the File contents.
*/
public void setHash(String hash) {
this.Hash = hash;
}
/**
* Indicates that the File has an image Preview.
*/
public Boolean getHasPreview() {
return this.HasPreview;
}
/**
* Indicates that the File has an image Preview.
*/
public void setHasPreview(Boolean haspreview) {
this.HasPreview = haspreview;
}
/**
* Current Anti-Virus scanning status for this file
*/
public SFSafeEnum getVirusStatus() {
return this.VirusStatus;
}
/**
* Current Anti-Virus scanning status for this file
*/
public void setVirusStatus(SFSafeEnum virusstatus) {
this.VirusStatus = virusstatus;
}
/**
* Data Loss Prevention information for this file.
*/
public SFItemDlpInfo getDlpInfo() {
return this.DlpInfo;
}
/**
* Data Loss Prevention information for this file.
*/
public void setDlpInfo(SFItemDlpInfo dlpinfo) {
this.DlpInfo = dlpinfo;
}
/**
* Effective Access Control permissions for this file
*/
public SFItemInfo getInfo() {
return this.Info;
}
/**
* Effective Access Control permissions for this file
*/
public void setInfo(SFItemInfo info) {
this.Info = info;
}
/**
* Indicates the user that has locked the file
*/
public SFUser getLockedBy() {
return this.LockedBy;
}
/**
* Indicates the user that has locked the file
*/
public void setLockedBy(SFUser lockedby) {
this.LockedBy = lockedby;
}
/**
* File lock info
*/
public SFFileLock getFileLockInfo() {
return this.FileLockInfo;
}
/**
* File lock info
*/
public void setFileLockInfo(SFFileLock filelockinfo) {
this.FileLockInfo = filelockinfo;
}
/**
* File version.
*/
public Float getVersion() {
return this.Version;
}
/**
* File version.
*/
public void setVersion(Float version) {
this.Version = version;
}
/**
* Electronic signature object associated with this item
*/
public SFESignature getESignatureDocument() {
return this.ESignatureDocument;
}
/**
* Electronic signature object associated with this item
*/
public void setESignatureDocument(SFESignature esignaturedocument) {
this.ESignatureDocument = esignaturedocument;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy