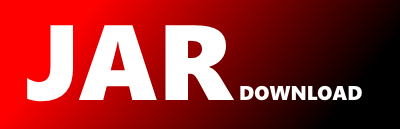
com.citrix.sharefile.api.models.SFNotification Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFNotification extends SFODataObject {
@SerializedName("NotificationType")
private String NotificationType;
@SerializedName("EventID")
private String EventID;
@SerializedName("SenderID")
private String SenderID;
@SerializedName("RecipientID")
private String RecipientID;
@SerializedName("FromName")
private String FromName;
@SerializedName("FromEmail")
private String FromEmail;
@SerializedName("ReplyTo")
private String ReplyTo;
@SerializedName("To")
private String To;
@SerializedName("CC")
private String CC;
@SerializedName("BCC")
private String BCC;
@SerializedName("Subject")
private String Subject;
@SerializedName("Message")
private String Message;
@SerializedName("PlainTextMessage")
private String PlainTextMessage;
@SerializedName("DateSent")
private Date DateSent;
@SerializedName("Status")
private String Status;
@SerializedName("IsImportant")
private Boolean IsImportant;
@SerializedName("ReadReceipt")
private Boolean ReadReceipt;
@SerializedName("AttachmentPaths")
private ArrayList AttachmentPaths;
@SerializedName("ForceEmailFromShareFile")
private Boolean ForceEmailFromShareFile;
@SerializedName("MergeNames")
private ArrayList MergeNames;
@SerializedName("MergeValues")
private ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy