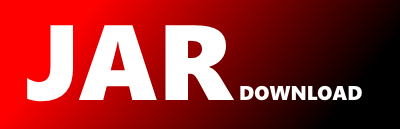
com.citrix.sharefile.api.models.SFOAuthClient Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFOAuthClient extends SFODataObject {
@SerializedName("ClientSecret")
private String ClientSecret;
@SerializedName("AccountID")
private String AccountID;
@SerializedName("Image")
private String Image;
@SerializedName("ImageSmall")
private String ImageSmall;
@SerializedName("State")
private SFSafeEnum State;
@SerializedName("Name")
private String Name;
@SerializedName("CompanyName")
private String CompanyName;
@SerializedName("ToolUrl")
private String ToolUrl;
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("LastModifiedDate")
private Date LastModifiedDate;
@SerializedName("ServerFlow")
private Boolean ServerFlow;
@SerializedName("ClientFlow")
private Boolean ClientFlow;
@SerializedName("UsernamePasswordFlow")
private Boolean UsernamePasswordFlow;
@SerializedName("SamlFlow")
private Boolean SamlFlow;
@SerializedName("IsQA")
private Boolean IsQA;
@SerializedName("Impersonation")
private Boolean Impersonation;
@SerializedName("DeviceRegistration")
private Boolean DeviceRegistration;
@SerializedName("CanCreateFreemiumAccount")
private Boolean CanCreateFreemiumAccount;
@SerializedName("IsInternalAdmin")
private Boolean IsInternalAdmin;
@SerializedName("DisableAuthCookie")
private Boolean DisableAuthCookie;
@SerializedName("AccessFilesFolders")
private SFSafeEnum AccessFilesFolders;
@SerializedName("ModifyFilesFolders")
private SFSafeEnum ModifyFilesFolders;
@SerializedName("AdminUsers")
private SFSafeEnum AdminUsers;
@SerializedName("AdminAccounts")
private SFSafeEnum AdminAccounts;
@SerializedName("ChangeMySettings")
private SFSafeEnum ChangeMySettings;
@SerializedName("WebAppLogin")
private SFSafeEnum WebAppLogin;
@SerializedName("AppCode")
private SFSafeEnum AppCode;
@SerializedName("RedirectUrls")
private ArrayList RedirectUrls;
public String getClientSecret() {
return this.ClientSecret;
}
public void setClientSecret(String clientsecret) {
this.ClientSecret = clientsecret;
}
public String getAccountID() {
return this.AccountID;
}
public void setAccountID(String accountid) {
this.AccountID = accountid;
}
public String getImage() {
return this.Image;
}
public void setImage(String image) {
this.Image = image;
}
public String getImageSmall() {
return this.ImageSmall;
}
public void setImageSmall(String imagesmall) {
this.ImageSmall = imagesmall;
}
public SFSafeEnum getState() {
return this.State;
}
public void setState(SFSafeEnum state) {
this.State = state;
}
public String getName() {
return this.Name;
}
public void setName(String name) {
this.Name = name;
}
public String getCompanyName() {
return this.CompanyName;
}
public void setCompanyName(String companyname) {
this.CompanyName = companyname;
}
public String getToolUrl() {
return this.ToolUrl;
}
public void setToolUrl(String toolurl) {
this.ToolUrl = toolurl;
}
public Date getCreationDate() {
return this.CreationDate;
}
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
public Date getLastModifiedDate() {
return this.LastModifiedDate;
}
public void setLastModifiedDate(Date lastmodifieddate) {
this.LastModifiedDate = lastmodifieddate;
}
public Boolean getServerFlow() {
return this.ServerFlow;
}
public void setServerFlow(Boolean serverflow) {
this.ServerFlow = serverflow;
}
public Boolean getClientFlow() {
return this.ClientFlow;
}
public void setClientFlow(Boolean clientflow) {
this.ClientFlow = clientflow;
}
public Boolean getUsernamePasswordFlow() {
return this.UsernamePasswordFlow;
}
public void setUsernamePasswordFlow(Boolean usernamepasswordflow) {
this.UsernamePasswordFlow = usernamepasswordflow;
}
public Boolean getSamlFlow() {
return this.SamlFlow;
}
public void setSamlFlow(Boolean samlflow) {
this.SamlFlow = samlflow;
}
public Boolean getIsQA() {
return this.IsQA;
}
public void setIsQA(Boolean isqa) {
this.IsQA = isqa;
}
public Boolean getImpersonation() {
return this.Impersonation;
}
public void setImpersonation(Boolean impersonation) {
this.Impersonation = impersonation;
}
public Boolean getDeviceRegistration() {
return this.DeviceRegistration;
}
public void setDeviceRegistration(Boolean deviceregistration) {
this.DeviceRegistration = deviceregistration;
}
public Boolean getCanCreateFreemiumAccount() {
return this.CanCreateFreemiumAccount;
}
public void setCanCreateFreemiumAccount(Boolean cancreatefreemiumaccount) {
this.CanCreateFreemiumAccount = cancreatefreemiumaccount;
}
public Boolean getIsInternalAdmin() {
return this.IsInternalAdmin;
}
public void setIsInternalAdmin(Boolean isinternaladmin) {
this.IsInternalAdmin = isinternaladmin;
}
public Boolean getDisableAuthCookie() {
return this.DisableAuthCookie;
}
public void setDisableAuthCookie(Boolean disableauthcookie) {
this.DisableAuthCookie = disableauthcookie;
}
public SFSafeEnum getAccessFilesFolders() {
return this.AccessFilesFolders;
}
public void setAccessFilesFolders(SFSafeEnum accessfilesfolders) {
this.AccessFilesFolders = accessfilesfolders;
}
public SFSafeEnum getModifyFilesFolders() {
return this.ModifyFilesFolders;
}
public void setModifyFilesFolders(SFSafeEnum modifyfilesfolders) {
this.ModifyFilesFolders = modifyfilesfolders;
}
public SFSafeEnum getAdminUsers() {
return this.AdminUsers;
}
public void setAdminUsers(SFSafeEnum adminusers) {
this.AdminUsers = adminusers;
}
public SFSafeEnum getAdminAccounts() {
return this.AdminAccounts;
}
public void setAdminAccounts(SFSafeEnum adminaccounts) {
this.AdminAccounts = adminaccounts;
}
public SFSafeEnum getChangeMySettings() {
return this.ChangeMySettings;
}
public void setChangeMySettings(SFSafeEnum changemysettings) {
this.ChangeMySettings = changemysettings;
}
public SFSafeEnum getWebAppLogin() {
return this.WebAppLogin;
}
public void setWebAppLogin(SFSafeEnum webapplogin) {
this.WebAppLogin = webapplogin;
}
public SFSafeEnum getAppCode() {
return this.AppCode;
}
public void setAppCode(SFSafeEnum appcode) {
this.AppCode = appcode;
}
public ArrayList getRedirectUrls() {
return this.RedirectUrls;
}
public void setRedirectUrls(ArrayList redirecturls) {
this.RedirectUrls = redirecturls;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy