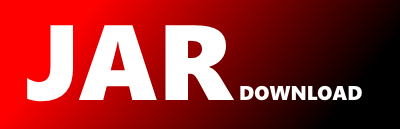
com.citrix.sharefile.api.models.SFOrderUpdateNotification Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFOrderUpdateNotification extends SFODataObject {
@SerializedName("AcknowledgementType")
private SFSafeEnum AcknowledgementType;
@SerializedName("Status")
private String Status;
@SerializedName("ErrorMessage")
private String ErrorMessage;
@SerializedName("AccountId")
private String AccountId;
@SerializedName("BillingLogId")
private String BillingLogId;
@SerializedName("SAPCustomerNumber")
private String SAPCustomerNumber;
@SerializedName("SAPOrderNumber")
private String SAPOrderNumber;
@SerializedName("SAPInvoiceNumber")
private String SAPInvoiceNumber;
@SerializedName("PaymentCurrency")
private String PaymentCurrency;
@SerializedName("PaymentDate")
private Date PaymentDate;
@SerializedName("PaymentAmount")
private Double PaymentAmount;
@SerializedName("ProcessedTimeStamp")
private Date ProcessedTimeStamp;
public SFSafeEnum getAcknowledgementType() {
return this.AcknowledgementType;
}
public void setAcknowledgementType(SFSafeEnum acknowledgementtype) {
this.AcknowledgementType = acknowledgementtype;
}
public String getStatus() {
return this.Status;
}
public void setStatus(String status) {
this.Status = status;
}
public String getErrorMessage() {
return this.ErrorMessage;
}
public void setErrorMessage(String errormessage) {
this.ErrorMessage = errormessage;
}
public String getAccountId() {
return this.AccountId;
}
public void setAccountId(String accountid) {
this.AccountId = accountid;
}
public String getBillingLogId() {
return this.BillingLogId;
}
public void setBillingLogId(String billinglogid) {
this.BillingLogId = billinglogid;
}
public String getSAPCustomerNumber() {
return this.SAPCustomerNumber;
}
public void setSAPCustomerNumber(String sapcustomernumber) {
this.SAPCustomerNumber = sapcustomernumber;
}
public String getSAPOrderNumber() {
return this.SAPOrderNumber;
}
public void setSAPOrderNumber(String sapordernumber) {
this.SAPOrderNumber = sapordernumber;
}
public String getSAPInvoiceNumber() {
return this.SAPInvoiceNumber;
}
public void setSAPInvoiceNumber(String sapinvoicenumber) {
this.SAPInvoiceNumber = sapinvoicenumber;
}
public String getPaymentCurrency() {
return this.PaymentCurrency;
}
public void setPaymentCurrency(String paymentcurrency) {
this.PaymentCurrency = paymentcurrency;
}
public Date getPaymentDate() {
return this.PaymentDate;
}
public void setPaymentDate(Date paymentdate) {
this.PaymentDate = paymentdate;
}
public Double getPaymentAmount() {
return this.PaymentAmount;
}
public void setPaymentAmount(Double paymentamount) {
this.PaymentAmount = paymentamount;
}
public Date getProcessedTimeStamp() {
return this.ProcessedTimeStamp;
}
public void setProcessedTimeStamp(Date processedtimestamp) {
this.ProcessedTimeStamp = processedtimestamp;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy