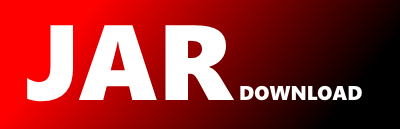
com.citrix.sharefile.api.models.SFPasswordPolicy Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFPasswordPolicy extends SFODataObject {
@SerializedName("MaxAgeDays")
private Integer MaxAgeDays;
@SerializedName("HistoryCount")
private Integer HistoryCount;
@SerializedName("MinimumLength")
private Integer MinimumLength;
@SerializedName("MinimumSpecialCharacters")
private Integer MinimumSpecialCharacters;
@SerializedName("MinimumNumeric")
private Integer MinimumNumeric;
@SerializedName("AllowedSpecialCharacters")
private String AllowedSpecialCharacters;
@SerializedName("ValidationRegEx")
private String ValidationRegEx;
@SerializedName("ValidationRegExFormula")
private String ValidationRegExFormula;
@SerializedName("ValidationRegExDescription")
private String ValidationRegExDescription;
/**
* Number of days a password is allowed to be used before being required to change it.
*/
public Integer getMaxAgeDays() {
return this.MaxAgeDays;
}
/**
* Number of days a password is allowed to be used before being required to change it.
*/
public void setMaxAgeDays(Integer maxagedays) {
this.MaxAgeDays = maxagedays;
}
/**
* The number of previously used passwords to disallow when a user updates their password.
*/
public Integer getHistoryCount() {
return this.HistoryCount;
}
/**
* The number of previously used passwords to disallow when a user updates their password.
*/
public void setHistoryCount(Integer historycount) {
this.HistoryCount = historycount;
}
/**
* The minimum length a password is required to be.
* Must be greater than or equal to 8.
* Cannot be smaller than the combination of required special characters + required numerics + 1 Upper Case character + 1 Lower Case character.
*/
public Integer getMinimumLength() {
return this.MinimumLength;
}
/**
* The minimum length a password is required to be.
* Must be greater than or equal to 8.
* Cannot be smaller than the combination of required special characters + required numerics + 1 Upper Case character + 1 Lower Case character.
*/
public void setMinimumLength(Integer minimumlength) {
this.MinimumLength = minimumlength;
}
/**
* The minimum number of special characters a password must contain.
* Special Characters are defined in the AllowedSpecialCharacters field.
*/
public Integer getMinimumSpecialCharacters() {
return this.MinimumSpecialCharacters;
}
/**
* The minimum number of special characters a password must contain.
* Special Characters are defined in the AllowedSpecialCharacters field.
*/
public void setMinimumSpecialCharacters(Integer minimumspecialcharacters) {
this.MinimumSpecialCharacters = minimumspecialcharacters;
}
/**
* The miminum number of numeric characters a password must contain.
* Must be greater than or equal to 1.
*/
public Integer getMinimumNumeric() {
return this.MinimumNumeric;
}
/**
* The miminum number of numeric characters a password must contain.
* Must be greater than or equal to 1.
*/
public void setMinimumNumeric(Integer minimumnumeric) {
this.MinimumNumeric = minimumnumeric;
}
/**
* Read only field - the special characters allowed in a password.
*/
public String getAllowedSpecialCharacters() {
return this.AllowedSpecialCharacters;
}
/**
* Read only field - the special characters allowed in a password.
*/
public void setAllowedSpecialCharacters(String allowedspecialcharacters) {
this.AllowedSpecialCharacters = allowedspecialcharacters;
}
/**
* Read only field - The full Regular Expression used to determine if a password meets the strength policy.
*/
public String getValidationRegEx() {
return this.ValidationRegEx;
}
/**
* Read only field - The full Regular Expression used to determine if a password meets the strength policy.
*/
public void setValidationRegEx(String validationregex) {
this.ValidationRegEx = validationregex;
}
/**
* Read only field - Colon separated regular expression rules.
*/
public String getValidationRegExFormula() {
return this.ValidationRegExFormula;
}
/**
* Read only field - Colon separated regular expression rules.
*/
public void setValidationRegExFormula(String validationregexformula) {
this.ValidationRegExFormula = validationregexformula;
}
/**
* Read only field - A localized description of the password strength policy
*/
public String getValidationRegExDescription() {
return this.ValidationRegExDescription;
}
/**
* Read only field - A localized description of the password strength policy
*/
public void setValidationRegExDescription(String validationregexdescription) {
this.ValidationRegExDescription = validationregexdescription;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy