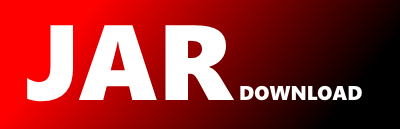
com.citrix.sharefile.api.models.SFPolicy Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFPolicy extends SFODataObject {
@SerializedName("Name")
private String Name;
@SerializedName("Category")
private SFSafeEnum Category;
@SerializedName("IsDefault")
private Boolean IsDefault;
@SerializedName("CreatedBy")
private SFUser CreatedBy;
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("UpdatedBy")
private SFUser UpdatedBy;
@SerializedName("UpdatedDate")
private Date UpdatedDate;
@SerializedName("Usage")
private SFPolicyUsage Usage;
/**
* Name of Policy
*/
public String getName() {
return this.Name;
}
/**
* Name of Policy
*/
public void setName(String name) {
this.Name = name;
}
/**
* UserAccess, FileAndFolder, Storage
*/
public SFSafeEnum getCategory() {
return this.Category;
}
/**
* UserAccess, FileAndFolder, Storage
*/
public void setCategory(SFSafeEnum category) {
this.Category = category;
}
/**
* Indicates this is the default policy for the category specified
*/
public Boolean getIsDefault() {
return this.IsDefault;
}
/**
* Indicates this is the default policy for the category specified
*/
public void setIsDefault(Boolean isdefault) {
this.IsDefault = isdefault;
}
/**
* Creator of Policy
*/
public SFUser getCreatedBy() {
return this.CreatedBy;
}
/**
* Creator of Policy
*/
public void setCreatedBy(SFUser createdby) {
this.CreatedBy = createdby;
}
/**
* Created Date
*/
public Date getCreationDate() {
return this.CreationDate;
}
/**
* Created Date
*/
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
/**
* User who last modified Policy or Policy Settings
*/
public SFUser getUpdatedBy() {
return this.UpdatedBy;
}
/**
* User who last modified Policy or Policy Settings
*/
public void setUpdatedBy(SFUser updatedby) {
this.UpdatedBy = updatedby;
}
/**
* Date Policy or Policy Settings were last modified
*/
public Date getUpdatedDate() {
return this.UpdatedDate;
}
/**
* Date Policy or Policy Settings were last modified
*/
public void setUpdatedDate(Date updateddate) {
this.UpdatedDate = updateddate;
}
/**
* Metrics of Users attached to policy
*/
public SFPolicyUsage getUsage() {
return this.Usage;
}
/**
* Metrics of Users attached to policy
*/
public void setUsage(SFPolicyUsage usage) {
this.Usage = usage;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy