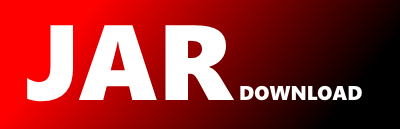
com.citrix.sharefile.api.models.SFQueueEntry Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFQueueEntry extends SFODataObject {
@SerializedName("CreationDate")
private Date CreationDate;
@SerializedName("ModifiedDate")
private Date ModifiedDate;
@SerializedName("Status")
private SFSafeEnum Status;
@SerializedName("IsLocked")
private Boolean IsLocked;
@SerializedName("WorkerName")
private String WorkerName;
@SerializedName("Payload")
private Map Payload;
@SerializedName("ErrorCount")
private Integer ErrorCount;
@SerializedName("ErrorCode")
private Integer ErrorCode;
@SerializedName("ErrorMessage")
private String ErrorMessage;
@SerializedName("Queue")
private SFQueue Queue;
public Date getCreationDate() {
return this.CreationDate;
}
public void setCreationDate(Date creationdate) {
this.CreationDate = creationdate;
}
public Date getModifiedDate() {
return this.ModifiedDate;
}
public void setModifiedDate(Date modifieddate) {
this.ModifiedDate = modifieddate;
}
public SFSafeEnum getStatus() {
return this.Status;
}
public void setStatus(SFSafeEnum status) {
this.Status = status;
}
public Boolean getIsLocked() {
return this.IsLocked;
}
public void setIsLocked(Boolean islocked) {
this.IsLocked = islocked;
}
public String getWorkerName() {
return this.WorkerName;
}
public void setWorkerName(String workername) {
this.WorkerName = workername;
}
public Map getPayload() {
return this.Payload;
}
public void setPayload(Map payload) {
this.Payload = payload;
}
public Integer getErrorCount() {
return this.ErrorCount;
}
public void setErrorCount(Integer errorcount) {
this.ErrorCount = errorcount;
}
public Integer getErrorCode() {
return this.ErrorCode;
}
public void setErrorCode(Integer errorcode) {
this.ErrorCode = errorcode;
}
public String getErrorMessage() {
return this.ErrorMessage;
}
public void setErrorMessage(String errormessage) {
this.ErrorMessage = errormessage;
}
public SFQueue getQueue() {
return this.Queue;
}
public void setQueue(SFQueue queue) {
this.Queue = queue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy