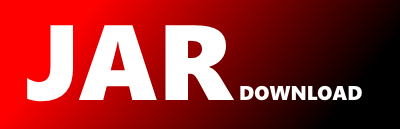
com.citrix.sharefile.api.models.SFQuote Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFQuote extends SFODataObject {
@SerializedName("AccountId")
private String AccountId;
@SerializedName("CreatedBy")
private String CreatedBy;
@SerializedName("QuoteJson")
private String QuoteJson;
@SerializedName("SentToEmailAddress")
private String SentToEmailAddress;
@SerializedName("PersonalMessage")
private String PersonalMessage;
@SerializedName("UpdatedBy")
private String UpdatedBy;
@SerializedName("CreatedDate")
private Date CreatedDate;
@SerializedName("UpdateDate")
private Date UpdateDate;
@SerializedName("ExpirationDate")
private Date ExpirationDate;
@SerializedName("AcceptedDate")
private Date AcceptedDate;
@SerializedName("ProcessedDate")
private Date ProcessedDate;
@SerializedName("IsValid")
private Boolean IsValid;
/**
* Account for which quote was created
*/
public String getAccountId() {
return this.AccountId;
}
/**
* Account for which quote was created
*/
public void setAccountId(String accountid) {
this.AccountId = accountid;
}
/**
* Creator user Id
*/
public String getCreatedBy() {
return this.CreatedBy;
}
/**
* Creator user Id
*/
public void setCreatedBy(String createdby) {
this.CreatedBy = createdby;
}
/**
* Json string capturing the account changes and other required information
*/
public String getQuoteJson() {
return this.QuoteJson;
}
/**
* Json string capturing the account changes and other required information
*/
public void setQuoteJson(String quotejson) {
this.QuoteJson = quotejson;
}
/**
* Email address of the recipient
*/
public String getSentToEmailAddress() {
return this.SentToEmailAddress;
}
/**
* Email address of the recipient
*/
public void setSentToEmailAddress(String senttoemailaddress) {
this.SentToEmailAddress = senttoemailaddress;
}
/**
* Message string that was send with quote
*/
public String getPersonalMessage() {
return this.PersonalMessage;
}
/**
* Message string that was send with quote
*/
public void setPersonalMessage(String personalmessage) {
this.PersonalMessage = personalmessage;
}
/**
* Id of the user making the update
*/
public String getUpdatedBy() {
return this.UpdatedBy;
}
/**
* Id of the user making the update
*/
public void setUpdatedBy(String updatedby) {
this.UpdatedBy = updatedby;
}
/**
* Time the quote was created
*/
public Date getCreatedDate() {
return this.CreatedDate;
}
/**
* Time the quote was created
*/
public void setCreatedDate(Date createddate) {
this.CreatedDate = createddate;
}
/**
* Last time when the quote was updated
*/
public Date getUpdateDate() {
return this.UpdateDate;
}
/**
* Last time when the quote was updated
*/
public void setUpdateDate(Date updatedate) {
this.UpdateDate = updatedate;
}
/**
* Time when the quote becomes invalid
*/
public Date getExpirationDate() {
return this.ExpirationDate;
}
/**
* Time when the quote becomes invalid
*/
public void setExpirationDate(Date expirationdate) {
this.ExpirationDate = expirationdate;
}
/**
* Time when the quote was accepted by the customer
*/
public Date getAcceptedDate() {
return this.AcceptedDate;
}
/**
* Time when the quote was accepted by the customer
*/
public void setAcceptedDate(Date accepteddate) {
this.AcceptedDate = accepteddate;
}
/**
* Time when the quote was processed by the bot
*/
public Date getProcessedDate() {
return this.ProcessedDate;
}
/**
* Time when the quote was processed by the bot
*/
public void setProcessedDate(Date processeddate) {
this.ProcessedDate = processeddate;
}
/**
* Flag to define if the quote is still valid
*/
public Boolean getIsValid() {
return this.IsValid;
}
/**
* Flag to define if the quote is still valid
*/
public void setIsValid(Boolean isvalid) {
this.IsValid = isvalid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy