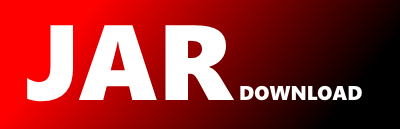
com.citrix.sharefile.api.models.SFRemoteUpload Maven / Gradle / Ivy
// ------------------------------------------------------------------------------
//
// This code was generated by a tool.
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
//
// Copyright (c) 2017 Citrix ShareFile. All rights reserved.
//
// ------------------------------------------------------------------------------
package com.citrix.sharefile.api.models;
import java.io.InputStream;
import java.util.ArrayList;
import java.net.URI;
import java.util.Date;
import java.util.Map;
import java.util.HashMap;
import com.google.gson.annotations.SerializedName;
import com.citrix.sharefile.api.*;
import com.citrix.sharefile.api.enumerations.*;
import com.citrix.sharefile.api.models.*;
public class SFRemoteUpload extends SFODataObject {
@SerializedName("Name")
private String Name;
@SerializedName("Uri")
private String Uri;
@SerializedName("IsPublic")
private Boolean IsPublic;
@SerializedName("RequireUserInfo")
private Boolean RequireUserInfo;
@SerializedName("SelectRecipient")
private Boolean SelectRecipient;
@SerializedName("Users")
private ArrayList Users;
/**
* The Name of this Remote Upload.
*/
public String getName() {
return this.Name;
}
/**
* The Name of this Remote Upload.
*/
public void setName(String name) {
this.Name = name;
}
/**
* Uri to access the Remote Upload through the Web portal
*/
public String getUri() {
return this.Uri;
}
/**
* Uri to access the Remote Upload through the Web portal
*/
public void setUri(String uri) {
this.Uri = uri;
}
/**
* Indicates whether the Remote Upload is public or not.
* One File Drop on your account can be marked as public, meaning that it is accessible from the short link https://example.sharefile.com/filedrop.
* Any additional File Drops on your account can be linked to on your web site using the link at the top of this page
*/
public Boolean getIsPublic() {
return this.IsPublic;
}
/**
* Indicates whether the Remote Upload is public or not.
* One File Drop on your account can be marked as public, meaning that it is accessible from the short link https://example.sharefile.com/filedrop.
* Any additional File Drops on your account can be linked to on your web site using the link at the top of this page
*/
public void setIsPublic(Boolean ispublic) {
this.IsPublic = ispublic;
}
/**
* Indicates whether the Remote Upload requires user information ( first name, last name, email , company).
*/
public Boolean getRequireUserInfo() {
return this.RequireUserInfo;
}
/**
* Indicates whether the Remote Upload requires user information ( first name, last name, email , company).
*/
public void setRequireUserInfo(Boolean requireuserinfo) {
this.RequireUserInfo = requireuserinfo;
}
/**
* When it's true, visitors of this Remote Upload can select a recipient from a list.
*/
public Boolean getSelectRecipient() {
return this.SelectRecipient;
}
/**
* When it's true, visitors of this Remote Upload can select a recipient from a list.
*/
public void setSelectRecipient(Boolean selectrecipient) {
this.SelectRecipient = selectrecipient;
}
/**
* Users who can have access to this Remote Upload.
*/
public ArrayList getUsers() {
return this.Users;
}
/**
* Users who can have access to this Remote Upload.
*/
public void setUsers(ArrayList users) {
this.Users = users;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy